JavaScript Ternary Operator
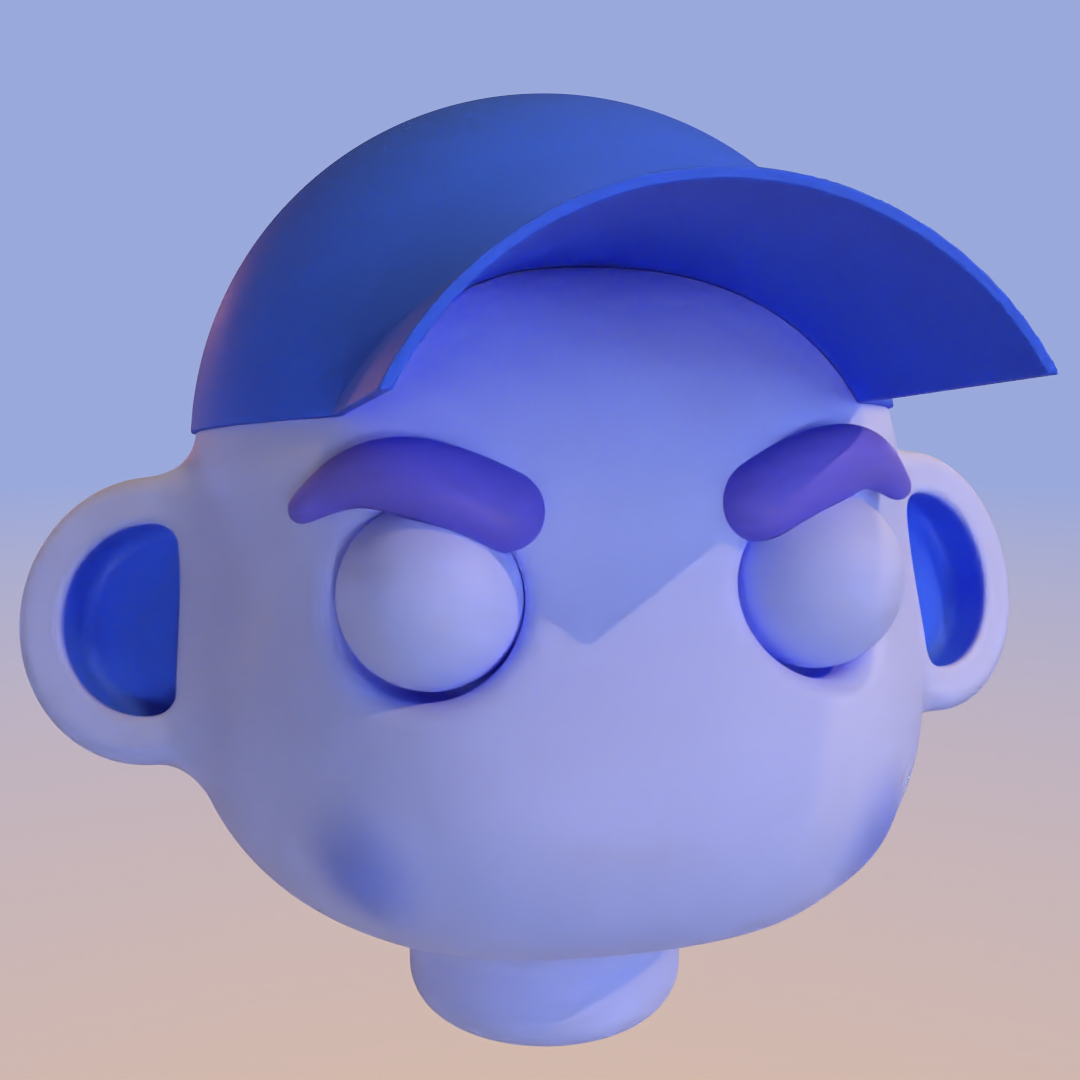
is a shorthand way of writing an if-else statement
it was used to make simple conditions simpler and more readable
for more complex conditions it is recommended to use traditional if-else
syntax
the ?
is equal to if
and :
is equal to else
const age = 20
age >= 18 ? console.log('You are eligible to vote') : console.log('You are not eligible to vote')
// Output: You are eligible to vote
example without using else
you don't need to write variable = true
, use variable
if the variable is true, you can write it as variable ?
if you don't want to do anything if it is false, you can use null
const variable = true
variable ? console.log('good') : null
or for more simpler you can use &&
to check if it's true without else
const variable = true
variable && console.log('good') // Output: good
use ?
to check if variables have value
if the variables were null or undefined, the code after ?
will not be executed
it was used to prevent error
const arr = [1, 2, 3]
console.log(arr?.length); // Output: 3
example in React
if using React, you can render the element inside the ()
directly
import React from 'react'
function TernaryOperator() {
const age = 18
return (
<p>
{age >= 18 ? ('You are eligible to vote') : ('You are not eligible to vote')}
</p>
)
}
export default TernaryOperator
so it makes the code more readable because you can put the operator inside the HTML element and render it there
Subscribe to my newsletter
Read articles from wisnu bayu directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
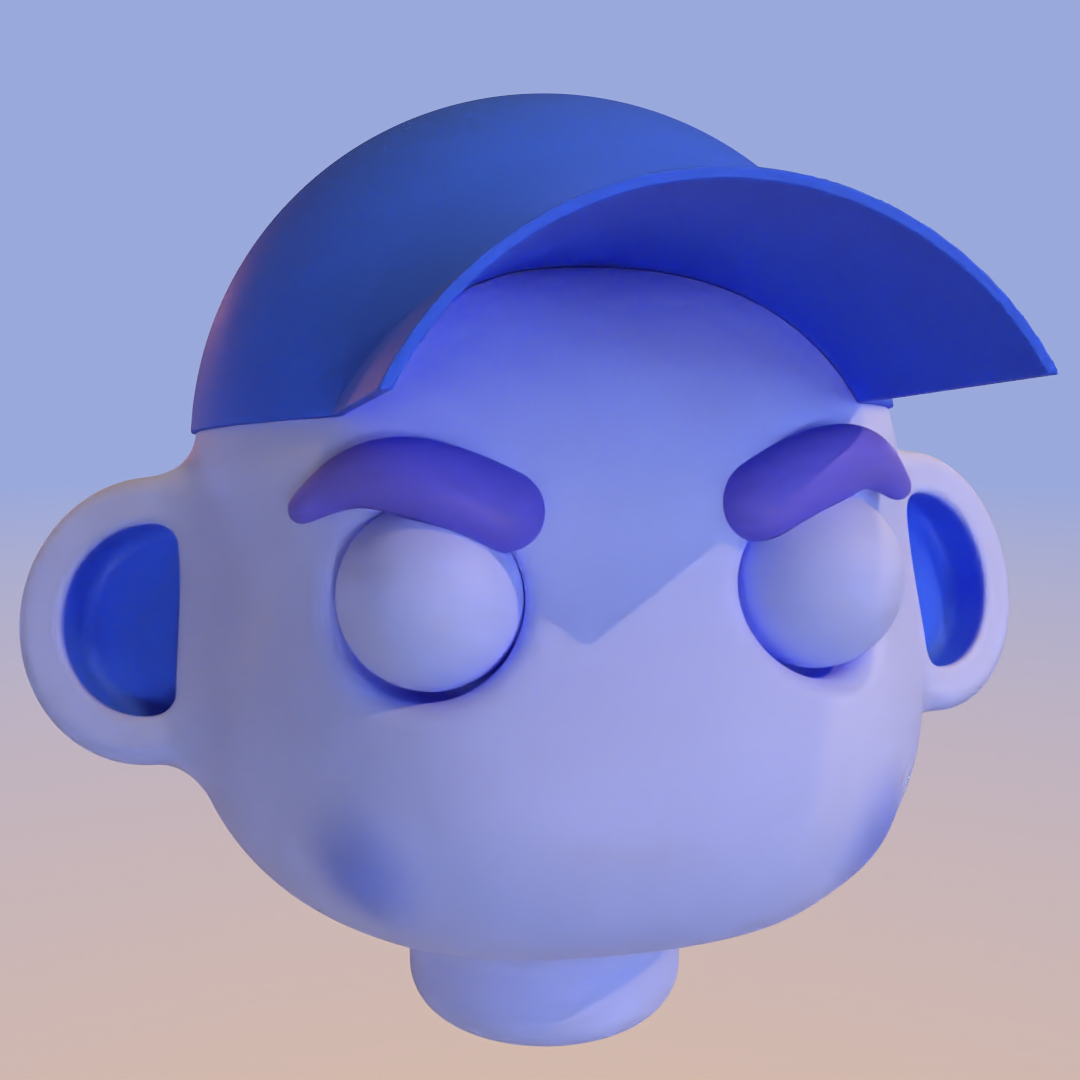