The Tale of Two Variables: Auto and Static
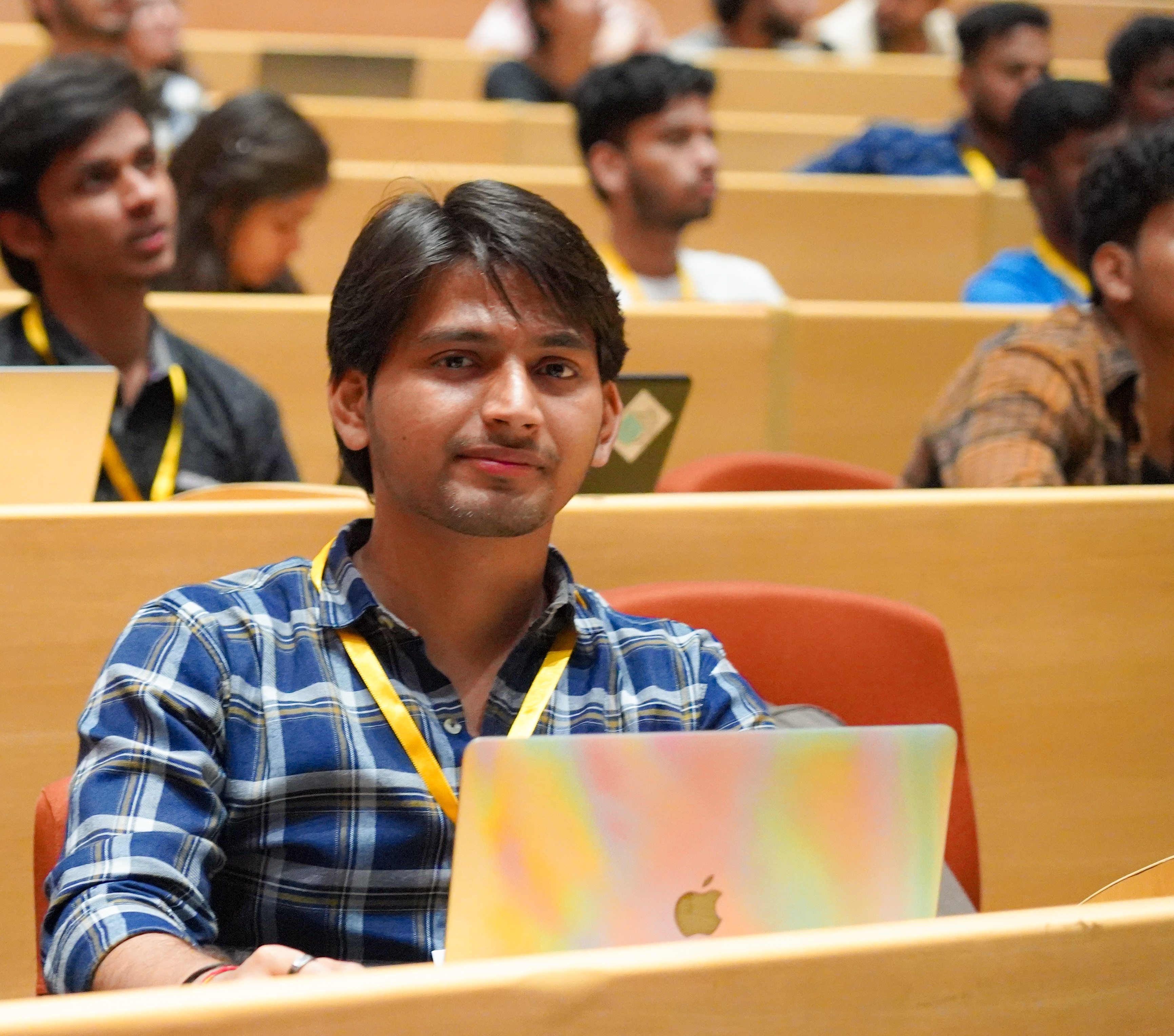
#include<stdio.h>
void increment (); //function decalaration
int main(){
increment (); // function call 3 times
increment();
increment();
return 0;
}
void increment (){ // function definition
auto int i=1;
static int j=1;
i=i+1;
j=j+1;
printf("%d %d\n",i,j);
}
Imagine you're working as a cashier at a store, helping customers with their purchases. Here's how auto
and static
variables would play out:
1. Auto (The Short-Term Helper):
An
auto
variable is like a temporary helper you hire for each customer.When a new customer arrives (function call), you create a new helper (variable) named
i
.You initialize
i
to 1, giving them a starting point (initializing the variable).You increment
i
by 1 to track the number of items the customer buys (incrementing the variable).You tell the customer the total items (printing
i
).Once the customer leaves, you dismiss the temporary helper
i
. They're no longer needed.This happens for every new customer (function call). So, each customer gets a fresh
i
that starts at 1 and goes up to 2.
2. Static (The Persistent Assistant):
A
static
variable is like a seasoned employee who stays on long-term.The first time a customer arrives (function call), you hire a new employee,
j
.You initialize
j
to 1 to keep track of the total customers served (initializing the variable).Every subsequent customer gets the same
j
employee, who remembers the previous number served.You increment
j
by 1 for each new customer.You tell the customer their total number (printing
j
).Unlike the temporary helper,
j
stays on even after the customer leaves (function ends). This meansj
remembers the total count between customers.
Why the Different Outputs?
Because
auto
variables are like temporary helpers, they start fresh every time (always 2 for each customer).static
variables, like the persistent employee, keep track of the overall count, leading to an increasing value (j keeps increasing).
I hope this story clarifies the difference between auto
and static
variables!
Subscribe to my newsletter
Read articles from SAHIL ALI directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
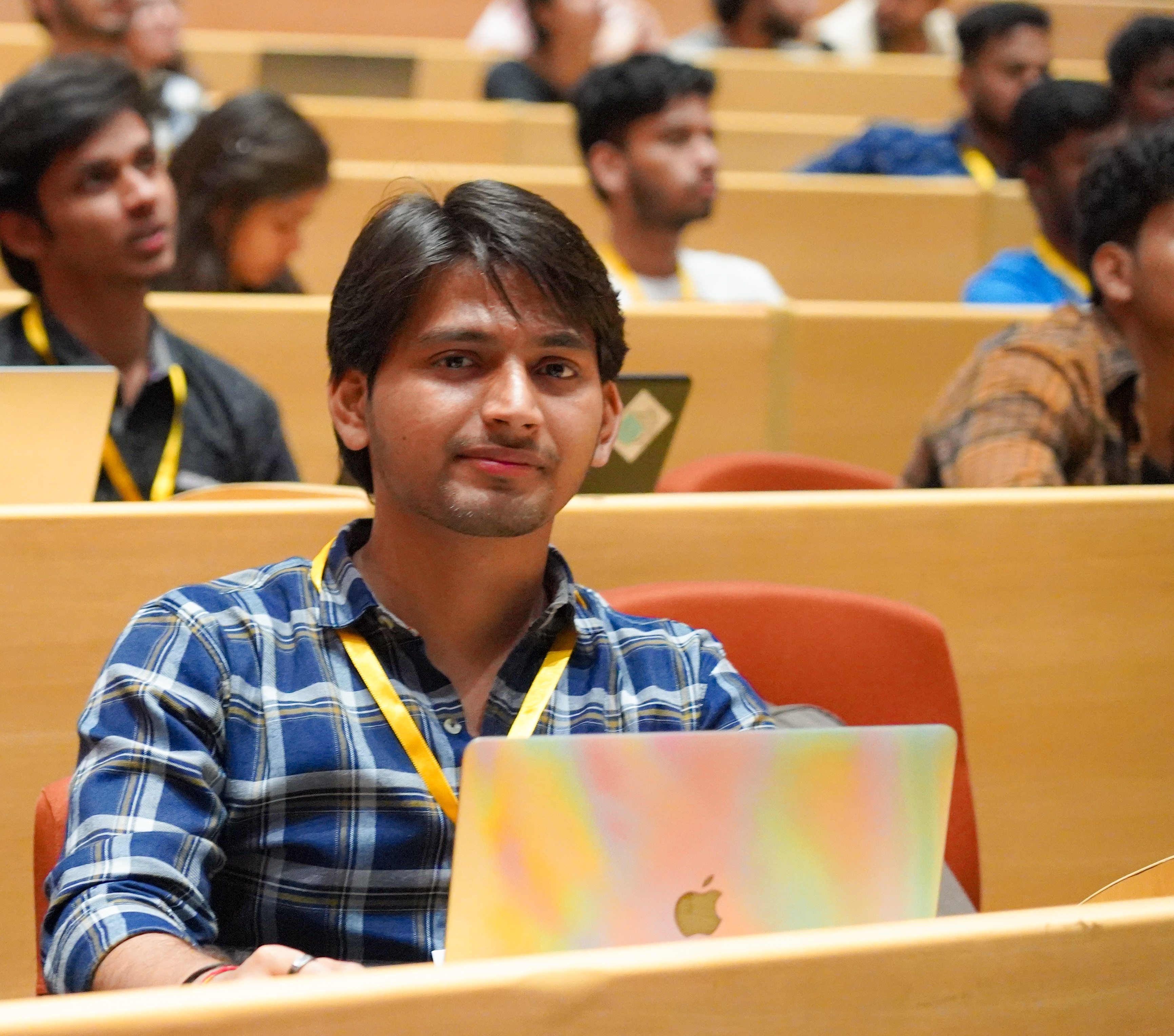
SAHIL ALI
SAHIL ALI
Full Stack Software Developer | Competitive Coder @Codeforces Open Source Contributor My major is Neural Network and Software Building.