Implementation of React Routers
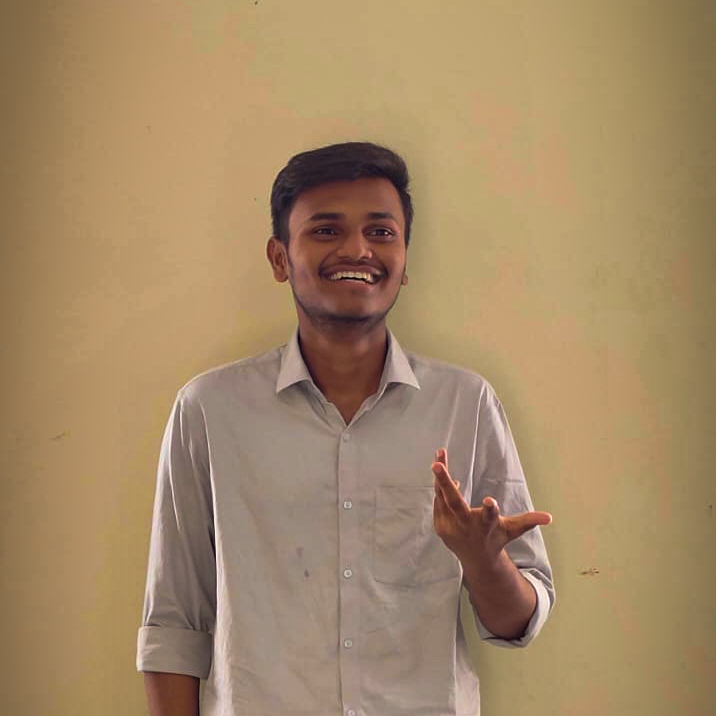
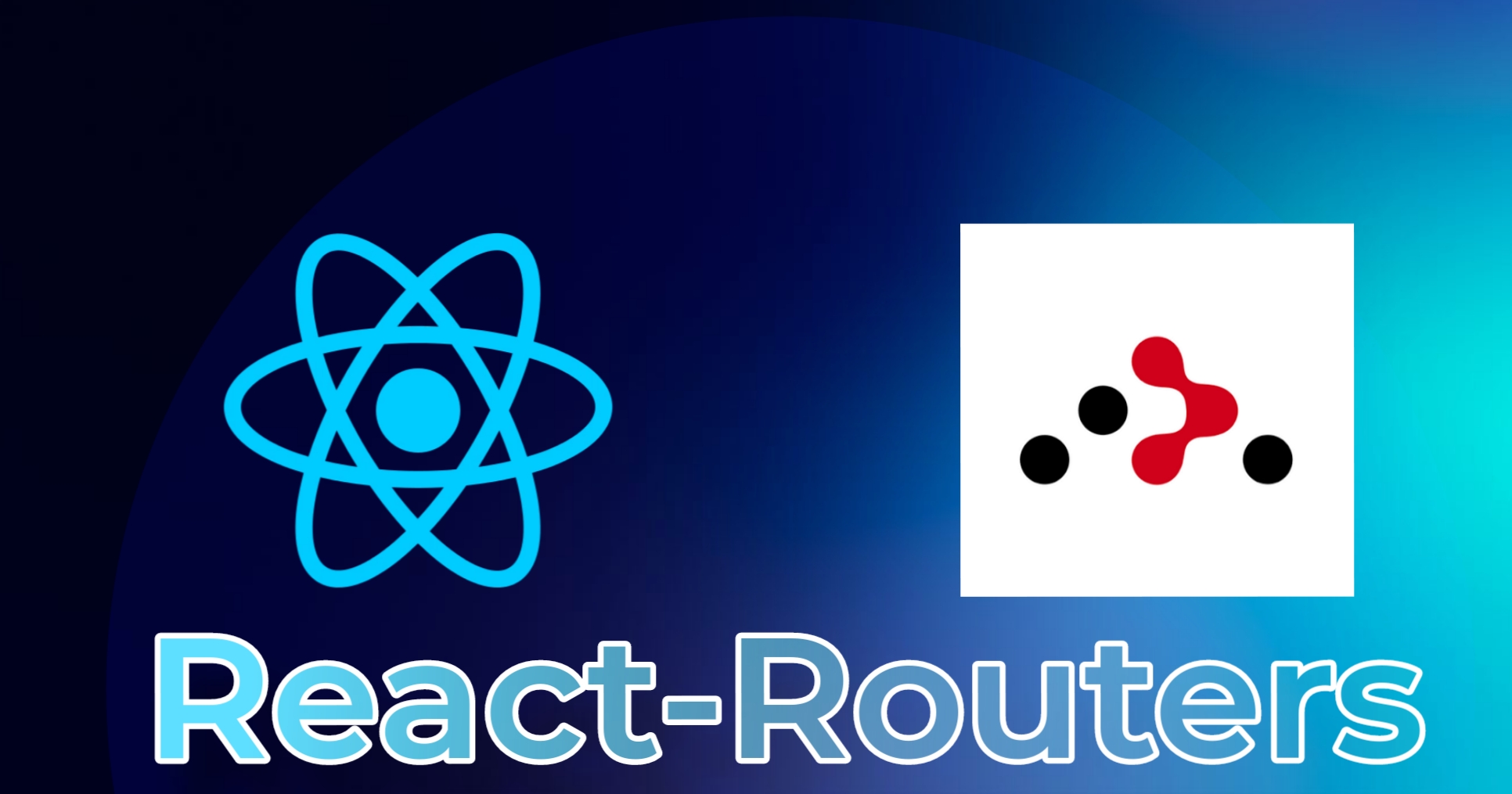
In this Blog we gonna learn about the react routers i.e. is what are the routers, it's uses, Their installation and their implementation with proper codes
let's Get Started....
what are the Routers??
In React applications, routers are libraries that manage the navigation between different components within a single-page application (SPA)..
The basic need of the react routers is to provide User-Friendly experience in the complex projects by providing the single page applications..
The Functionalities of React routers are...
URL Mapping : Routers map specific URLs to corresponding React components to be rendered.
Dynamic Rendering : Based on the current URL, the router dynamically renders the appropriate component responsible for that view.
Browser History Management*:* Routers integrate with the browser's history API to manage the navigation history stack.
The Famous library which is used for the react routers is react-router-dom
which is need to be installed separately along with the react application...
Installation...
First step we need to setup the react application run this below code in Terminal..
npm create vite@latest
After setting up react file in that file directory we need router packages
npm i react-router-dom
It is as simple as that to install the packages.
Implementation...
In this I'm gonna explain about 2 different ways to implement and explain why with the flow diagrams...
Method-1 :-
In This method we create all the components which are need to be there in the page like below diagram and import all these components into a Nav file and call that file in fixed.jsx file this is because as we click on components that components must be fixed so by this we can achieve that..
for this the code looks like..
Nav.jsx
import React from "react";
import { NavLink } from "react-router-dom";
import './Nav.css';
function Nav() {
return (
<>
<div>
<NavLink to="/">Home</NavLink>
<NavLink to="/Info">Info</NavLink>
<NavLink to="/Questions">Questions</NavLink>
<NavLink to="/Help">Help</NavLink>
</div>
</>
)}
export default Nav
for the single-page-applications links normal anchor tags <a href="#"></a>
will not work so we import <Link/>
tag or <NavLink/>
tags form the react-router-dom
library difference between we learn later in this....
Fixed.jsx
import React from "react";
import Nav from "./Nav";
import { Outlet } from "react-router-dom";
function Fixed(){
return(
<>
<Nav/>
<Outlet/>
</>
)}
export default Fixed
In this we see an import { Outlet }
from router library which helps user to keep the navBar constant and to keep changing with the components content....
Main.jsx
import React from 'react'
import ReactDOM from 'react-dom/client'
import { createBrowserRouter, RouterProvider } from 'react-router-dom'
import Home from './Home'
import Details from './Info'
import Questions from './Questions'
import Fixed from './Fixed'
import Help from './Help'
const router = createBrowserRouter([
{
path: "/",
element: <Fixed/>,
children: [
{
path: "",
element: <Home/>
},
{
path: "/info",
element: <Details/>
},
{
path: "/Questions",
element: <Questions/>
},
{
path: "/Help",
element: <Help/>
},
]}, ])
ReactDOM.createRoot(document.getElementById('root')).render(
<React.StrictMode>
<RouterProvider router={router} />
</React.StrictMode>
)
In this main.jsx file we import 2 methods from router library they are createBrowserRouter
and RouterProvider
here RouterProvider
which is called will take a prop router
which is a collection of objects of paths of components with the help of createBrowserRouter
method this contains 3 elements path
, element
and children
here children
contains all the components paths
Method-2:-
All the other file in this metho is same a method-1 except main.jsx in this we use other router methods they are
Main.jsx
import React from 'react'
import ReactDOM from 'react-dom/client'
import { createBrowserRouter, createRoutesFromElements, Route, RouterProvider } from 'react-router-dom'
import Home from './Home'
import Details from './Info'
import Questions from './Questions'
import Fixed from './Fixed'
import Help from './Help'
const router = createBrowserRouter(
createRoutesFromElements(
<Route path='/' element={<Layout/>}>
<Route path='/' element={<Home/>}/>
<Route path='/info' element={<Details/>}/>
<Route path='/Questions' element={<Questions/>}/>
<Route path='/help' element={<Help/>}/>
</Route>
)
)
ReactDOM.createRoot(document.getElementById('root')).render(
<React.StrictMode>
<RouterProvider router={router} />
</React.StrictMode>
)
In this method we use createRoutesFromElements
method which consists of nested Route
elements with the path
and the components
The difference between the boths methods is in method-1 we use children element which is an array of path objects but in the method-2 we use route element which is a good practice to use...
Link vs NavLink
Both these links will convert into Anchor tags after the rendering the components but the main difference is that NavLink
will take a class which calls a callBack function and contains a method called isActive
which is used to find whether the component is active or not...
<NavLink className=" ()=> isActive ? "condt1":"condt2";></NavLink>
For more about the router visit the documented site of the reactRouter
Thanks for allocating your time for reading this for more follow me on Twitter .
Plz... kindly share the feedback which helps me to write more and correct myself...
Subscribe to my newsletter
Read articles from Jayakrishna directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
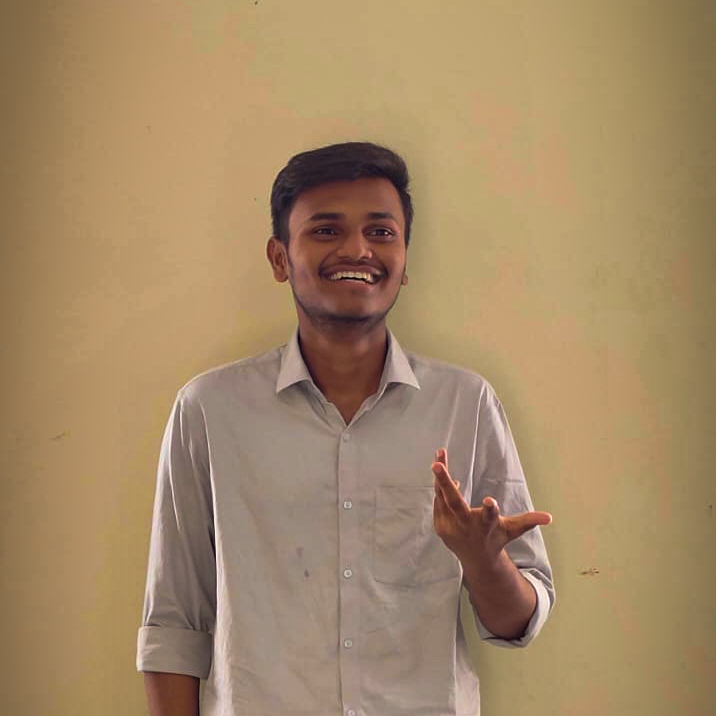