Day 10 Task: Advance Git & GitHub

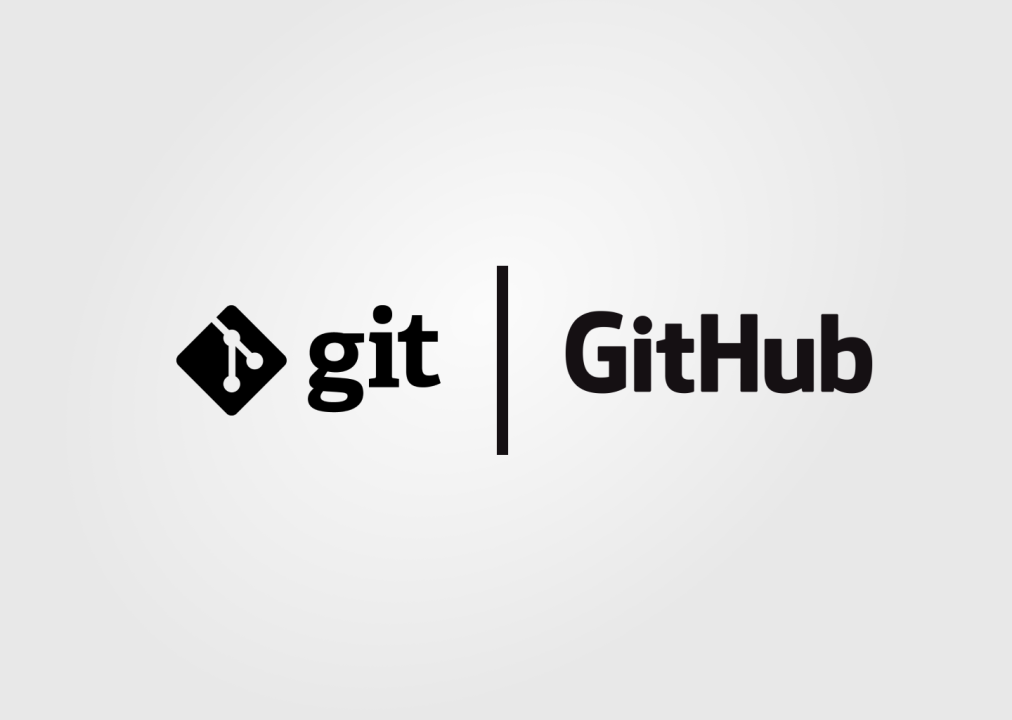
Git Branching
Use a branch to isolate development work without affecting other branches in the repository. Each repository has one default branch, and can have multiple other branches. You can merge a branch into another branch using a pull request.
Branches allow you to develop features, fix bugs, or safely experiment with new ideas in a contained area of your repository.
Git Revert and Reset
Two commonly used tools that git users will encounter are those of git reset and git revert . The benefit of both of these commands is that you can use them to remove or edit changes you’ve made in the code in previous commits.
Git Rebase and Merge
What Is Git Rebase?
Git rebase is a command that lets users integrate changes from one branch to another, and the logs are modified once the action is complete. Git rebase was developed to overcome merging’s shortcomings, specifically regarding logs.
What Is Git Merge?
Git merge is a command that allows developers to merge Git branches while the logs of commits on branches remain intact.
The merge wording can be confusing because we have two methods of merging branches, and one of those ways is actually called “merge,” even though both procedures do essentially the same thing.
Task 1 : Playing with git revert , git reset
git revert
undoes changes by creating new commits, preserving the commit history and avoiding conflicts in shared repositories.git reset
modifies the commit history by moving the branch pointer to a specified commit, potentially leading to data loss if used carelessly, and is typically used for local changes.
In summary, git revert
is safer for shared branches as it creates new commits to undo changes, while git reset
is more aggressive and can rewrite history, making it suitable for local changes or branches.
ubuntu@ip-172-31-2-228:~/Devops/Git$ git checkout -b dev
Switched to a new branch 'dev'
The command git checkout -b dev
creates a new branch named 'dev' and immediately switches your working directory to this newly created branch. It's a concise way to start working on a new branch in Git.
ubuntu@ip-172-31-2-228:~/Devops/git$ vi Version01.txt
ubuntu@ip-172-31-2-228:~/Devops/git$ git add Version01.txt
ubuntu@ip-172-31-2-228:~/Devops/git$ git commit -m "Added new feature"
[dev c54209c] Added new feature
2 files changed, 2 insertions(+)
create mode 100644 Git/file.txt
create mode 100644 git/Version01.txt
Here, added a new feature to the Version01.txt
file, staged the changes, and committed them to the Git repository with a descriptive commit message.
ubuntu@ip-172-31-2-228:~/Devops/git$ git push origin dev
Username for 'https://github.com': PrinceMeenia
Password for 'https://PrinceMeenia@github.com':
Enumerating objects: 56, done.
Counting objects: 100% (56/56), done.
Compressing objects: 100% (45/45), done.
Writing objects: 100% (56/56), 5.32 KiB | 680.00 KiB/s, done.
Total 56 (delta 13), reused 3 (delta 0), pack-reused 0
remote: Resolving deltas: 100% (13/13), done.
remote:
remote: Create a pull request for 'dev' on GitHub by visiting:
remote: https://github.com/PrinceMeenia/Devops/pull/new/dev
remote:
To https://github.com/PrinceMeenia/Devops.git
* [new branch] dev -> dev
The command git push origin dev
is pushing the commits from your local dev
branch to the remote repository's dev
branch on GitHub. You're prompted to enter your GitHub username and password for authentication. Then, Git enumerates the objects (commits, trees, and blobs) to be pushed, indicating the progress of the push operation.
Here, Local dev is pushed to a remote repository by creating a dev branch in the remote :
ubuntu@ip-172-31-2-228:~/Devops/git$ vi Version01.txt
ubuntu@ip-172-31-2-228:~/Devops/git$ git add .
ubuntu@ip-172-31-2-228:~/Devops/git$ git commit -m "Added feature2 in development branch”
> ^C
ubuntu@ip-172-31-2-228:~/Devops/git$ git commit -m "Added feature2 in development branch"
[dev 4dc57b1] Added feature2 in development branch
1 file changed, 3 insertions(+)
ubuntu@ip-172-31-2-228:~/Devops/git$ vi Version01.txt
ubuntu@ip-172-31-2-228:~/Devops/git$ git add .
ubuntu@ip-172-31-2-228:~/Devops/git$ git commit -m "Added feature3 in development branch"
[dev ed7de1f] Added feature3 in development branch
1 file changed, 2 insertions(+)
ubuntu@ip-172-31-2-228:~/Devops/git$ vi Version01.txt
ubuntu@ip-172-31-2-228:~/Devops/git$ git add .
ubuntu@ip-172-31-2-228:~/Devops/git$ git commit -m "Added feature4 in development branch"
[dev 4af31e0] Added feature4 in development branch
1 file changed, 3 insertions(+)
ubuntu@ip-172-31-2-228:~/Devops/git$
In the provided sequence of commands:
You made changes to
Version01.txt
and committed those changes with the message "Added feature2 in development branch."You made further changes to
Version01.txt
, committed them with the message "Added feature3 in development branch."More changes were made to
Version01.txt
, and you committed them with the message "Added feature4 in development branch."
Each commit represents the addition of a new feature in the development branch (dev
).
ubuntu@ip-172-31-2-228:~/Devops/git$ git log --oneline
4af31e0 (HEAD -> dev) Added feature4 in development branch
ed7de1f Added feature3 in development branch
4dc57b1 Added feature2 in development branch
c54209c (origin/dev) Added new feature
This command displays a simplified view of the commit history, showing each commit in a single line
ubuntu@ip-172-31-2-228:~/Devops/git$ git reset ed7de1f
Unstaged changes after reset:
D Git/Day-02.txt
D Git/file.txt
D Git/version01.txt
M git/Version01.txt
ubuntu@ip-172-31-2-228:~/Devops/git$ git log --oneline
ed7de1f (HEAD -> dev) Added feature3 in development branch
4dc57b1 Added feature2 in development branch
c54209c (origin/dev) Added new feature
The git reset ed7de1f
command undoes commits on the dev
branch up to the specified commit ed7de1f
, removing changes made in those commits. After the reset, there are unstaged changes indicating file deletions and modifications. The git log --oneline
command shows the updated commit history, where the dev
branch now points to the commit before the reset.
Task 2: Playing with git merge and git rebase
Here, we have switched to newly created branch dev
The commands executed are:
touch file3.txt
: This command creates a new file namedfile3.txt
in the current directory.git add .
: This command stages all changes in the working directory for the next commit.git commit -m "added file3.txt"
: This command commits the staged changes with the message "added file3". It records the addition of thefile.txt
file to the repository.created a new file in the dev branch
All the file including newly create file3.txt is also present in branch dev
After listing the directory contents and switching to the master
branch, you performed a merge from the dev
branch to the master
branch. This resulted in a fast-forward merge, where Git simply moved the master
branch pointer to the same commit as dev
. Consequently, the file3.txt
from dev
was incorporated into master
, and you have a consistent state across both branches.
A fast-forward merge occurs when the target branch (master
in this case) has not diverged from the source branch (dev
) since the time of the last commit on the target branch. This results in a linear history without a merge commit. The file3.txt
was successfully merged into master
, and now both branches are in sync
Git Rebase
Here, you can see with git merge , the history of commits you cannot see both dev and master branch synching , but in git rebase both the branches are properly synched with each other as the head is on dev, master both
Difference between Git rebase and Git merge
Merge: Use when you want to preserve the branching history and are working on a shared repository where rewriting history might cause conflicts for other collaborators.
Rebase: Use when you want to maintain a clean and linear commit history, especially for feature branches, and are sure that rebasing won't cause conflicts for other collaborators.
In general, both git merge
and git rebase
have their use cases, and the choice between them depends on your workflow preferences and the requirements of your project.
Subscribe to my newsletter
Read articles from prince meenia directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
