Components In React

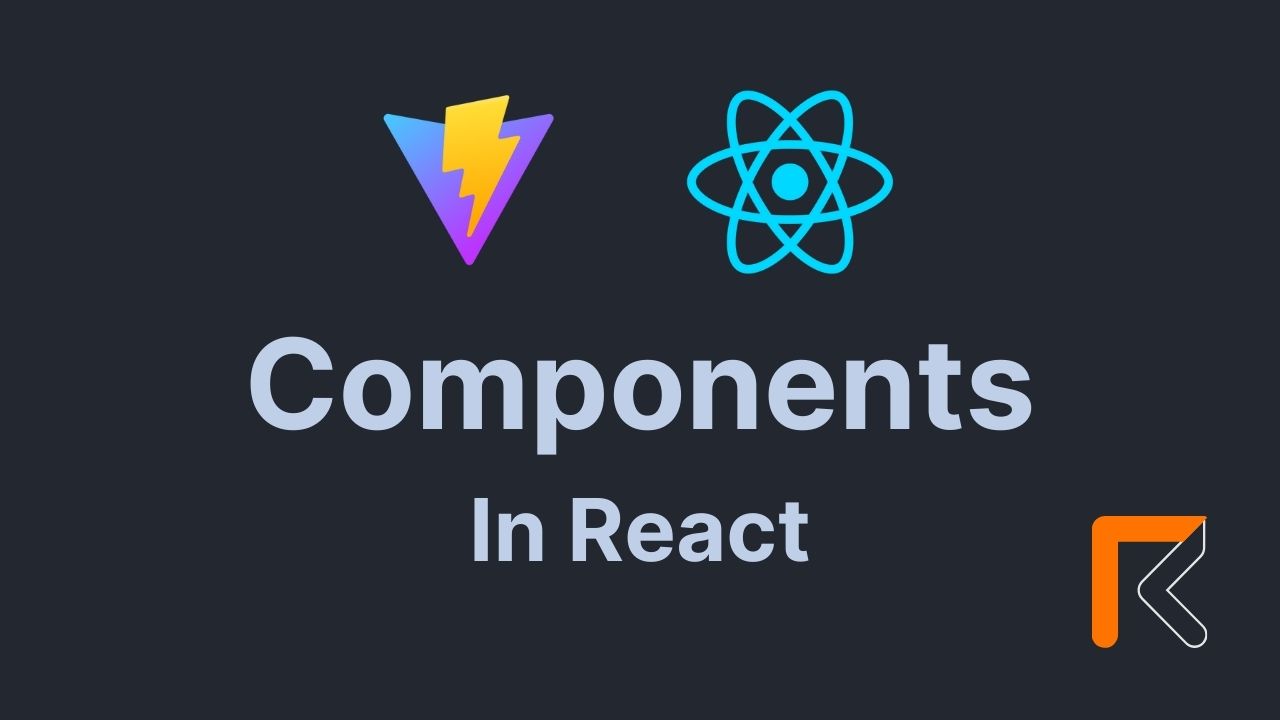
In this article, we are going to learn about the components of react
Components
What is a Component?
In React, components are reusable pieces of code that represent a part of a user interface. Components allow developers to organize and structure the UI in a modular way.
Components are small reusable blocks of web applications like navigation, hero banners, footers, cards, etc.
In React there are two types of components 1) Class Components and 2)Function Components.
Here we are focusing on Function Components
How To Create Component?
Let's consider we want to create a button component.
There are some rules to create components they are:
The component name should be capitalized
Use
.jsx
file extension to use HTML-like syntax.
The steps to create a button component are as:
Create a file in the
src folder
and named the file asBuyButton.jsx
Create a new component using the function
function Buybutton() { return( <button>Buy Now</button> ) } export default Buybutton;
Import Component
To display the button on the browser you need to import
button component
.Here in my case, i am importing
button component
inApp.jsx
fileimport Buybutton from "./Buybutton"; function App() { return( <Buybutton/> ) } export default App;
Multi-component import/export without default
Create a file in the
src folder
and named the file asShopbutton.jsx
Create a component
Here in
Shopbutton.jsx
file I have created two components 1) Buybutton 2) Cardbuttonexport function Buybutton() { return( <button>Buy Now</button> ) } export function Cardbutton() { return( <button>Add to Card</button> ) }
Import Component
To display the button on the browser you need to import these
button components
.Here in my case, i am importing these
button components
inApp.jsx
fileimport {Buybutton, Cardbutton} from "./Shopbutton"; function App() { return( <> <Buybutton/> <Cardbutton/> </> ) } export default App;
Multi-component import/export with default
After the component file created
Create a component
Here in
Shopbutton.jsx
file I have created two components 1) Buybutton 2) Cardbutton but I have export the Buybutton component defaultfunction Buybutton() { return( <button>Buy Now</button> ) } export function Cardbutton() { return( <button>Add to Card</button> ) } export default Buybutton;
Import Component
import {Cardbutton} from "./Shopbutton"; import Buybutton from "./Shopbutton"; function App() { return( <> <Buybutton/> <Cardbutton/> </> ) } export default App;
Output
Dynamic Component
In dynamic components, we can use the javascript expression directly in jsx. This includes variables, functions, etc. To use the javascript expression directly in jsx use parenthesis {}
in jsx.
function UserDetails() {
let firstName = "Mohammed Abdul";
let lastName = "Raheem";
return (
<>
<h1>
<span>{firstName}</span>
<span>{lastName}</span>
</h1>
</>
);
}
Reusable Component
In React we can reuse our components in different parts of our web application easily. Using Reusable components enhances UI consistency and reduces dependency and development time by writing duplicate code. Also, it is easy to maintain reusable components and easy to update and fix bugs.
Example:
Create a file called Random.jsx
//File Name: Random.jsx
function Random() {
let randomNumber = Math.random() * 100;
return(
<>
<p>This is a Random Number: {Math.floor(randomNumber)} </p>
</>
)
}
export default Random;
Imported Random.jsx
in App.jsx
file
import Random from "./Random";
function App() {
return(
<>
<Random/>
<Random/>
<Random/>
</>
)
}
export default App;
Thank you for reading
Subscribe to my newsletter
Read articles from Mohammed Abdul Raheem directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
