π¦ΈββοΈ Unveiling the Power of JavaScript Functions: Your Code's Superheroes
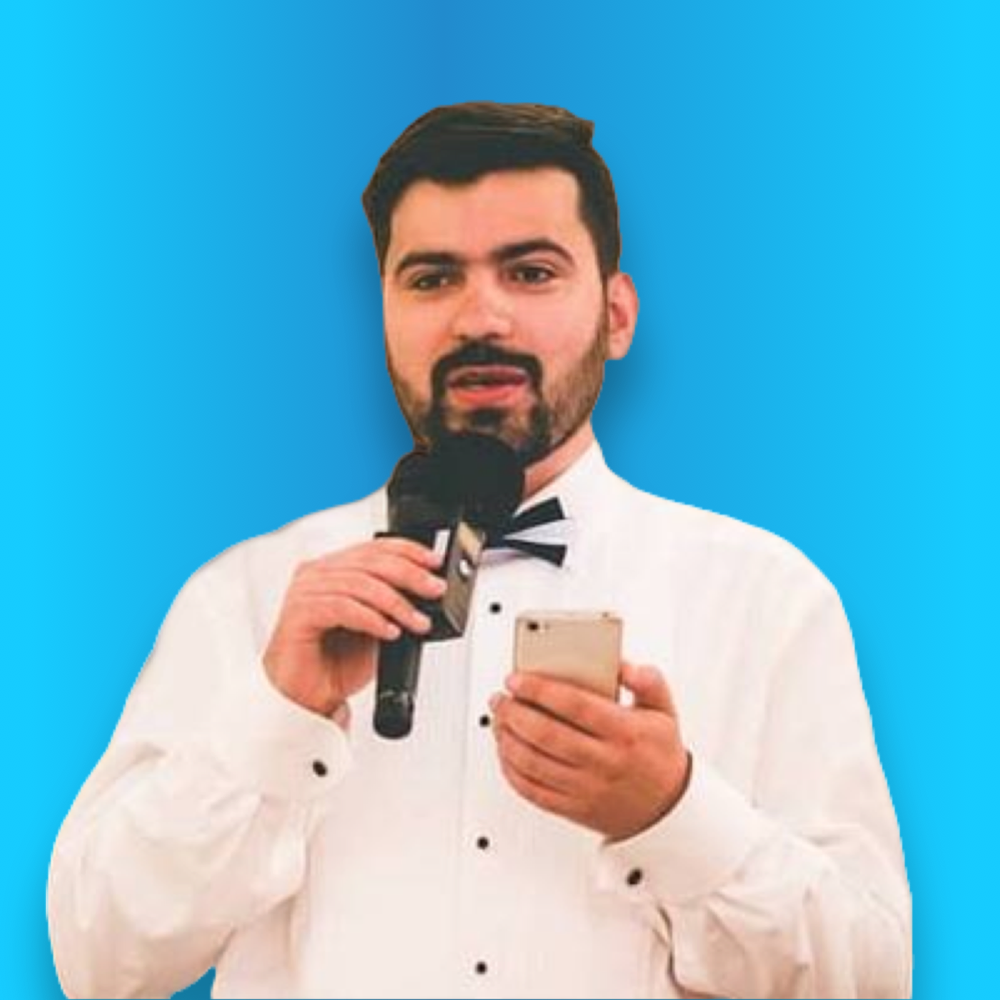
Table of contents
- π§ Function 101: The Building Block
- π οΈ Parameters and Arguments: The Function's Helpers
- β¬ οΈ Return Statement: The Messenger
- πΆοΈ Function Expressions: The Cool Kids
- β‘οΈ Arrow Functions: The Short and Sweet
- π© Function Scope: The VIP Access
- π Global Scope: The Big Picture
- π Callback Functions: The Team Players
- π Higher-Order Functions: The Managers
- π IIFE (Immediately Invoked Function Expression): The Instant Performer
- π Final thoughts
- βοΈ References
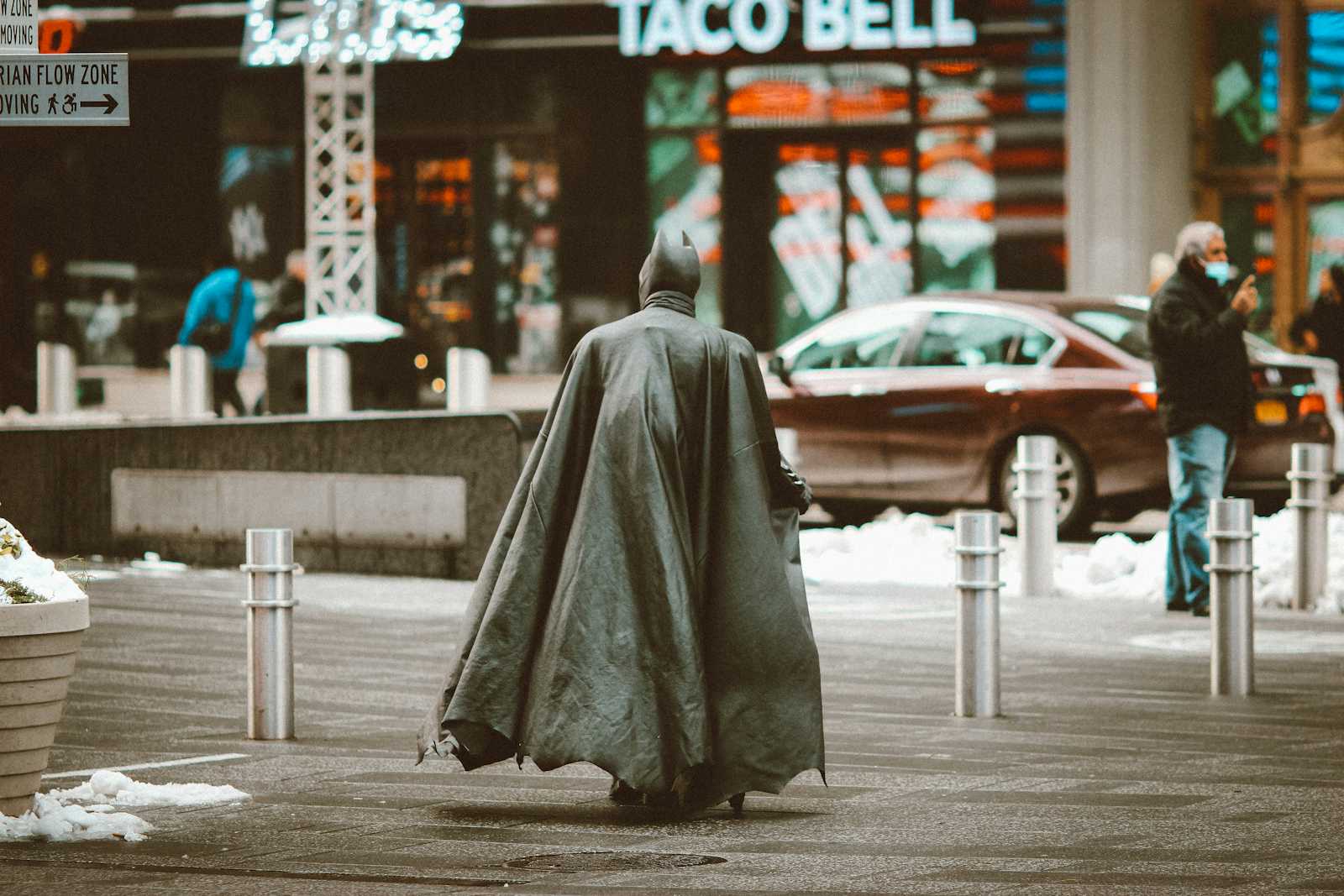
Let's dive into π€Ώ the wonderful world of JavaScript functions β those handy chunks of code that make your life easier. Think of them as your code's superheroes, ready to save the day whenever you call upon them!
π§ Function 101: The Building Block
So, what's a function? It's like a mini-program inside your program. You give it a name, and it can do specific tasks for you. It's like saying, "Hey function, go ahead and greet the world!" π
function greet() {
console.log('Hello, JavaScript functions!');
}
// Call the function
greet();
π οΈ Parameters and Arguments: The Function's Helpers
Functions can be even more powerful with parameters. They're like the helpers that bring information into the function πΈ. It's like saying, "Hey function, here's a name β say hello to Alice!"
function greetWithName(name) {
console.log(`Hello, ${name}!`);
}
// Call the function with an argument
greetWithName('Alice');
β¬ οΈ Return Statement: The Messenger
Functions can also send messages back using the return
statement. It's like the function saying, "I've got something valuable for you." It's like saying, "Hey function, add these numbers and tell me the result."
function addNumbers(a, b) {
return a + b;
}
// Use the function's return value
const sum = addNumbers(3, 7);
console.log(`The sum is: ${sum}`);
πΆοΈ Function Expressions: The Cool Kids
Function expressions are like the cool kids. You can assign a function to a variable. Flexibility at its finest! It's like saying, "Hey function, you're not just a function β you're a cool variable too!"
const multiply = function (x, y) {
return x * y;
};
// Use the function expression
const result = multiply(4, 5);
console.log(`The result is: ${result}`);
β‘οΈ Arrow Functions: The Short and Sweet
Arrow functions are the short and sweet versions of functions. They're like the rockstars of modern JavaScript. It's like saying, "Hey arrow function, show off your power β square this number!"
const powerUp = (x) => x ** 2;
// Use the arrow function
const squared = powerUp(3);
console.log(`The square is: ${squared}`);
π© Function Scope: The VIP Access
Functions have their own VIP section called scope. Variables inside a function stay there, like secret agents with exclusive access. It's like saying, "Hey variable, you're a secret agent β stay within your function's scope!"
function secretAgent() {
const codeName = '007';
console.log(`Agent ${codeName}, you have VIP access here.`);
}
// Can't access codeName here
π Global Scope: The Big Picture
Variables declared outside functions have global scope. They're like the big shots, accessible from anywhere. It's like saying, "Hey global variable, you're the big shot β everyone can see you!"
const globalVariable = "I'm everywhere!";
function showGlobal() {
// Can access globalVariable here
console.log(globalVariable);
}
showGlobal();
π Callback Functions: The Team Players
Callback functions are like team players. You pass them as arguments to other functions, and they join in on the action. It's like saying, "Hey function, here's a partner β celebrate when we're done!"
function doSomething(callback) {
console.log('Doing something...');
callback();
}
function celebrate() {
console.log('Yay, we did it!');
}
// Use a callback function
doSomething(celebrate);
π Higher-Order Functions: The Managers
Higher-order functions are like managers. They take functions as parameters or return them. They're the bosses in the world of functions. It's like saying, "Hey higher-order function, manage these operations β we'll call you when we need them!"
function managerFunction(operation) {
return function (a, b) {
return operation(a, b);
};
}
const multiply = managerFunction((x, y) => x * y);
// Use the higher-order function
const result = multiply(5, 4);
console.log(`The result is: ${result}`);
π IIFE (Immediately Invoked Function Expression): The Instant Performer
IIFE is like the instant performer. It executes immediately after being created. It's a one-time show. It's like saying, "Hey function, no need to wait for a call β perform now, right away!"
(function () {
console.log('IIFE is here for an instant performance!');
})();
π Final thoughts
Our journey into the captivating realm of JavaScript functions has unveiled these remarkable snippets of code as true superheroes in the coding universe. π¦ΈββοΈ Just like reliable companions, they stand ready to make our coding lives easier, swooping in to save the day whenever we summon their powers. So, whether it's simplifying tasks, enhancing organization, or streamlining processes, JavaScript functions are the unsung heroes behind the scenes, always poised to lend a helping hand in our coding adventures. Here's to the mighty world of functions, where every line of code tells a story of efficiency and empowerment! ππ»
βοΈ References
Subscribe to my newsletter
Read articles from Ricardo Rocha // π¨βπ» directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
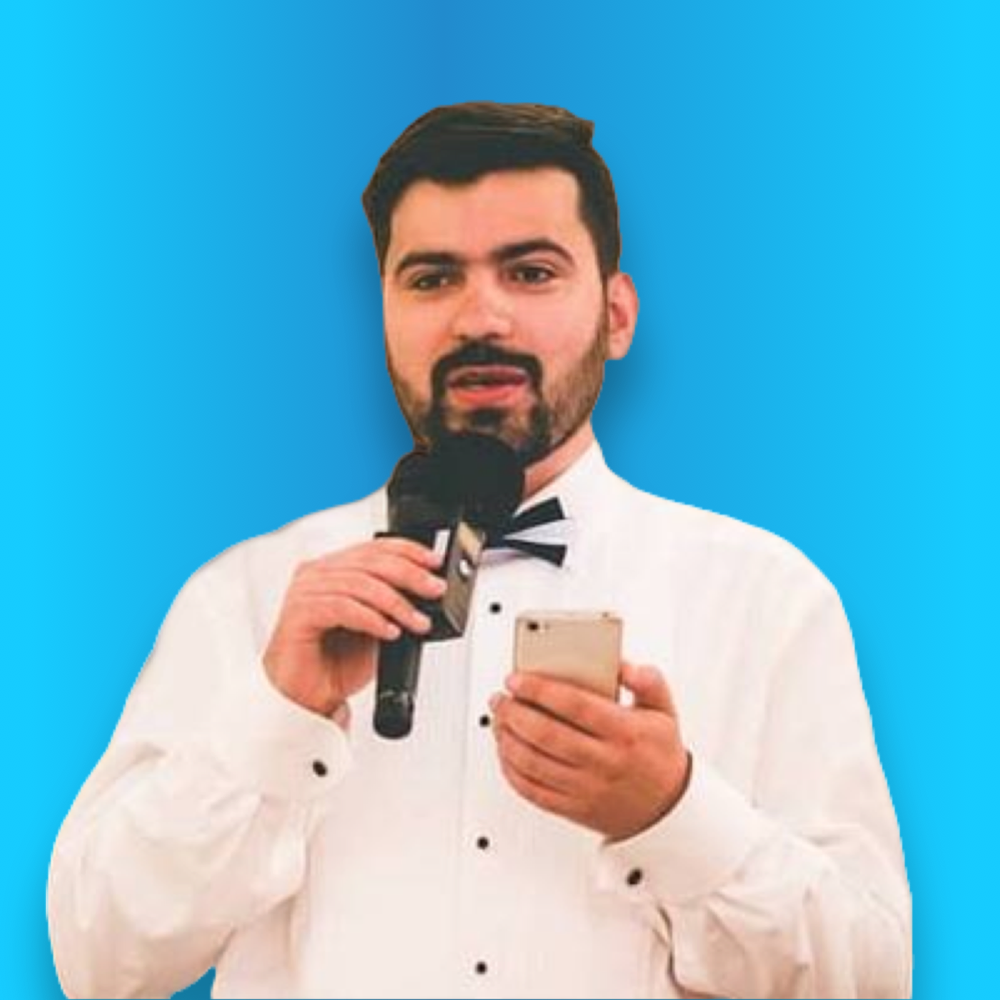
Ricardo Rocha // π¨βπ»
Ricardo Rocha // π¨βπ»
Hey there π! π So, here's the lowdown β I'm a full-stack web dev with a serious crush on front-end development. Armed with a master's in Software Engineering, I've been rocking the programming scene for a solid decade. I've got this knack for software architecture, team and project management, and even dabble in the magical realm of deep learning (yeah, AI, baby!). My coding toolbox π§° is stacked β JavaScript, TypeScript, React, Angular, C#, SQL, NoSQL - you name it. Nevertheless, learning is my best tool π! But here's the thing β I'm not just about the code. My soft skills game is strong β think big-picture pondering, critical thinking, and communication skills sharper than a ninja's blade. Leading, mentoring, and rocking successful projects? Yeah, that's my jam as well. Now, outside the coding dojo, I'm a music lover. Saxophone and piano are my instruments of choice, teaching me the art of teamwork and staying cool under pressure. I've got a soft spot for giving back too π₯°. I've lent a hand to the Jacksonville Human Society (dog shelter). And speaking of sharing wisdom, I also write blogs and buzz around on Twitter, LinkedIn, Stackoverflow and my own Blog. Go ahead and check me out: Linkedin (https://www.linkedin.com/in/ricardogomesrocha/) Stackoverflow (https://stackoverflow.com/users/5148197/ricardo-rocha) Twitter (https://twitter.com/RochaDaRicardo) Github (https://github.com/RicardoGomesRocha) Blog (https://ricardo-tech-lover.hashnode.dev/) Let's connect and dive into the exciting world of web development! Cheers π₯, Ricardo π