FlotaingActionButton Widget and Attributes

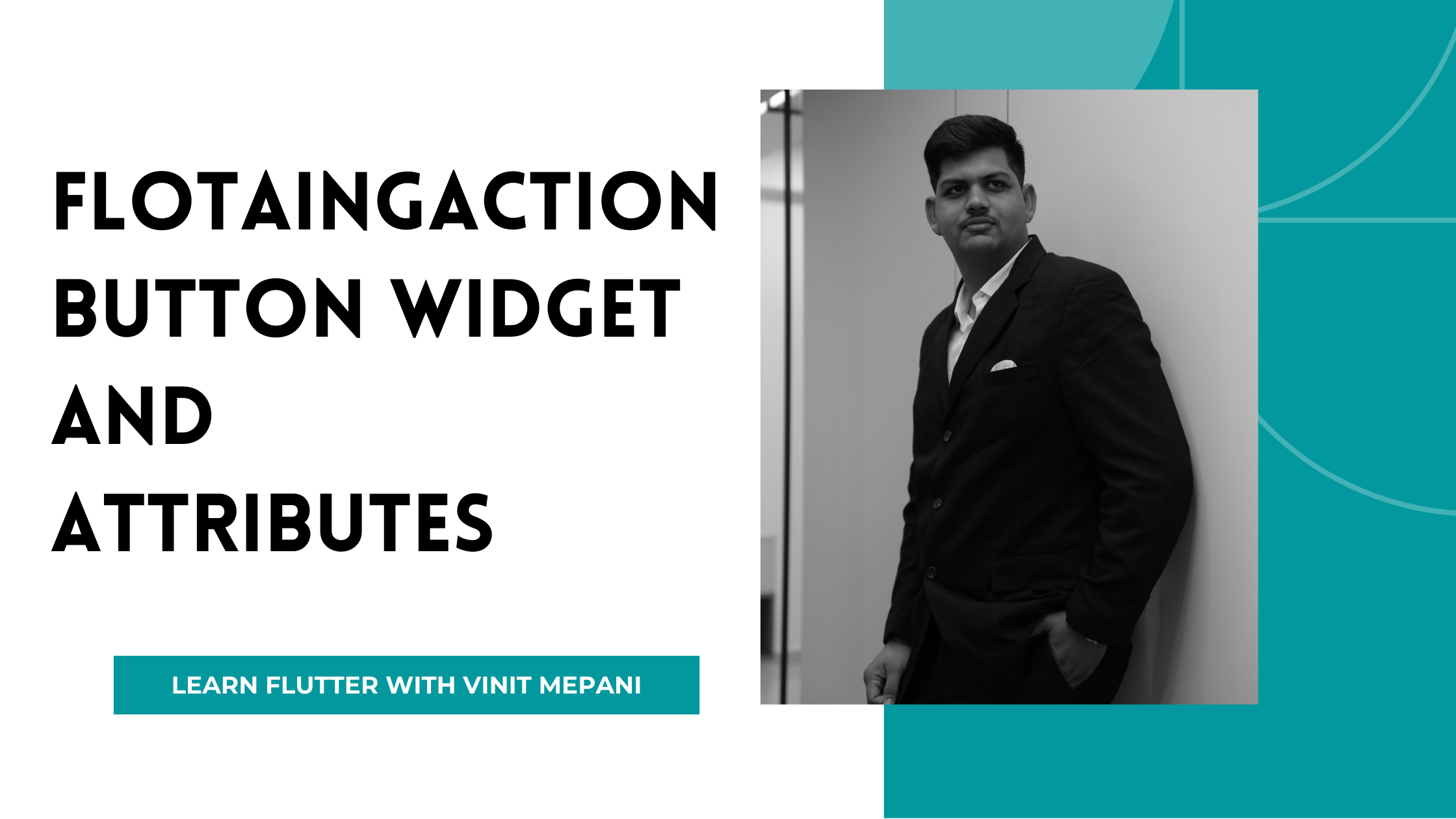
The FloatingActionButton widget in Flutter is a circular button typically placed in the bottom-right corner of the screen. It's commonly used to trigger the most important action in the application, such as adding a new item or navigating to a primary destination.
Attributes:
onPressed (Function):
- The callback function that is called when the button is pressed. This attribute is required.
child (Widget):
- The widget to be displayed inside the button. It's typically an Icon or Text widget.
tooltip (String):
- The tooltip message to be displayed when the user long-presses the button. It's an optional attribute.
backgroundColor (Color):
- The background color of the button. It defaults to the accent color of the current theme if not specified.
foregroundColor (Color):
- The foreground color of the button, which affects the color of the child widget. It defaults to white if not specified.
elevation (double):
- The elevation of the button, which affects its shadow. The default value is 6.0.
shape (ShapeBorder):
- The shape of the button. It defaults to a circular shape.
heroTag (Object):
- An optional tag for identifying the button in hero animations. If omitted, a unique tag will be generated automatically.
Example:
import 'package:flutter/material.dart';
class FloatingActionButtonExample extends StatelessWidget {
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: Text('FloatingActionButton Widget Example'),
),
body: Center(
child: Text('Press the FloatingActionButton'),
),
floatingActionButton: FloatingActionButton(
onPressed: () {
// Add your action here
print('FloatingActionButton pressed');
},
child: Icon(Icons.add),
tooltip: 'Add',
backgroundColor: Colors.blue,
),
);
}
}
void main() {
runApp(MaterialApp(
home: FloatingActionButtonExample(),
));
}
Explanation:
In this example, a FloatingActionButton widget is placed inside a Scaffold widget.
The onPressed attribute is set to a callback function that prints a message when the button is pressed.
The child attribute is set to an Icon widget with the "add" icon to represent the button.
The tooltip attribute is set to "Add" to provide a tooltip message when the button is long-pressed.
The backgroundColor attribute is set to Colors.blue to specify the background color of the button.
When you run this code, you'll see an app bar with a title and a centered text widget. Pressing the floating action button will print a message to the console.
Subscribe to my newsletter
Read articles from Vinit Mepani directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Vinit Mepani
Vinit Mepani
"Hello World, I'm Vinit Mepani, a coding virtuoso driven by passion, fueled by curiosity, and always poised to conquer challenges. Picture me as a digital explorer, navigating through the vast realms of code, forever in pursuit of innovation. In the enchanting kingdom of algorithms and syntax, I wield my keyboard as a magical wand, casting spells of logic and crafting solutions to digital enigmas. With each line of code, I embark on an odyssey of learning, embracing the ever-evolving landscape of technology. Eager to decode the secrets of the programming universe, I see challenges not as obstacles but as thrilling quests, opportunities to push boundaries and uncover new dimensions in the realm of possibilities. In this symphony of zeros and ones, I am Vinit Mepani, a coder by passion, an adventurer in the digital wilderness, and a seeker of knowledge in the enchanting world of code. Join me on this quest, and let's create digital wonders together!"