Dart : Super Keyword
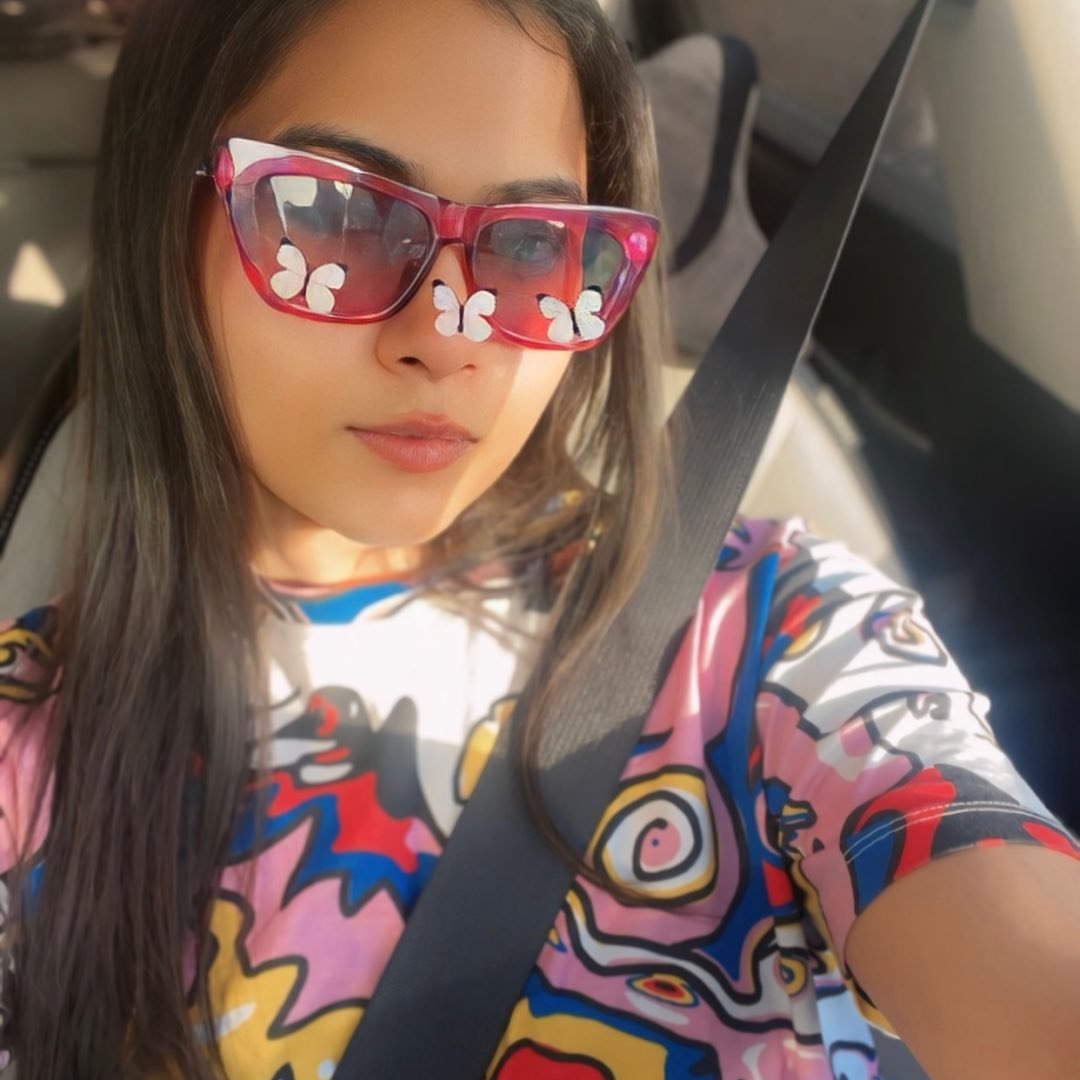
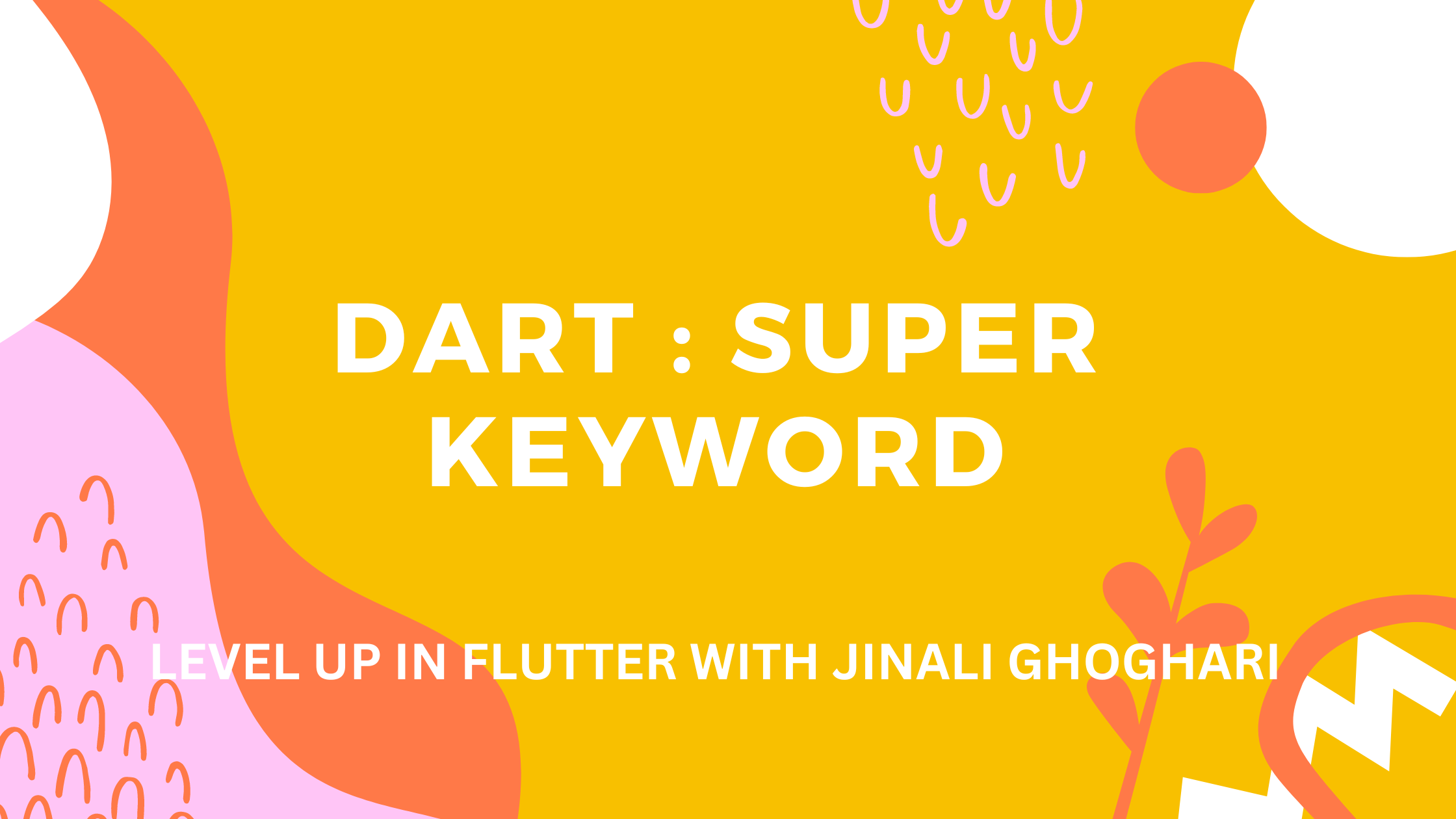
In Dart, when you're working with classes, sometimes you have a class that inherits from another class.
The class that does the inheriting is called the child class, and the class it inherits from is called the parent class.
Now, let's say both the parent and child classes have a method or a property with the same name.
When you're inside the child class and you want to specifically refer to the method or property of the parent class, you use the super
keyword.
So, in simple words, super
in Dart is like saying "Hey, go to the parent class and do this." It's a way to access and use things from the parent class when you're working inside the child class.
When both the parent and child classes have things with the same name (like variables or functions), using super
helps you access the version of that thing that belongs to the parent class, not the child class.
Syntax
// To access parent class variables
super.variable_name;
// To access parent class method
super.method_name();
Example:
In this example, we use the super
keyword to access the parent class method.
When the parent and child classes have the same name, we can use the super keyword to call the parent class method from the child class.
class ParentClass {
// Creating a method in Parent class
void showMessage()
{
print("This is parent class method.");
}
}
class SubClass extends ParentClass {
void showMessage()
{
print("This is child class method.");
// Calling parent class method
super.showMessage();
}
}
void main(){
// Creating the child class instance
SubClass myClass = new SubClass();
myClass.showMessage();
}
Output
//This is child class method.
//This is parent class method.
Subscribe to my newsletter
Read articles from Jinali Ghoghari directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
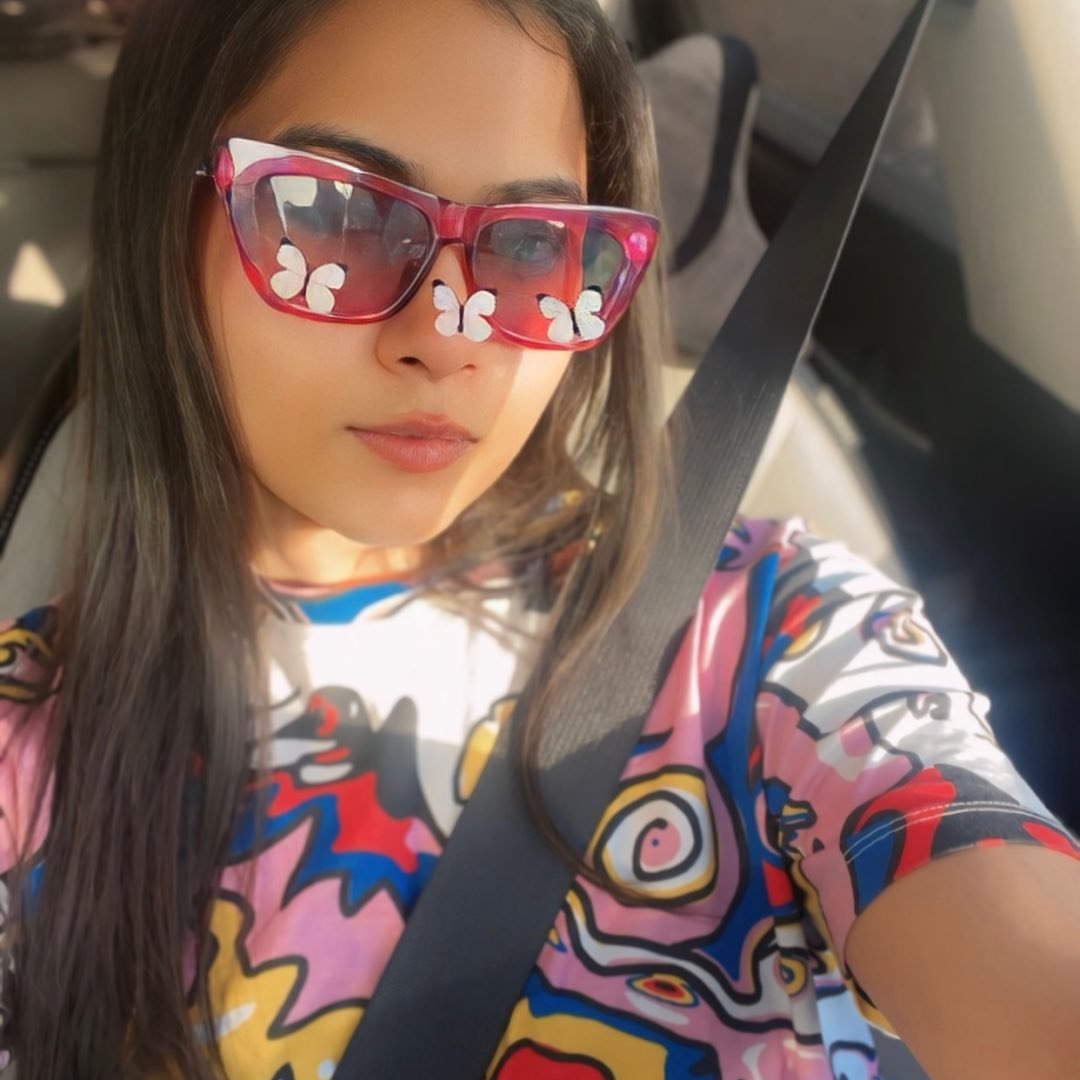