Dart: Getter & Setter Method | Why do we use getter & setter
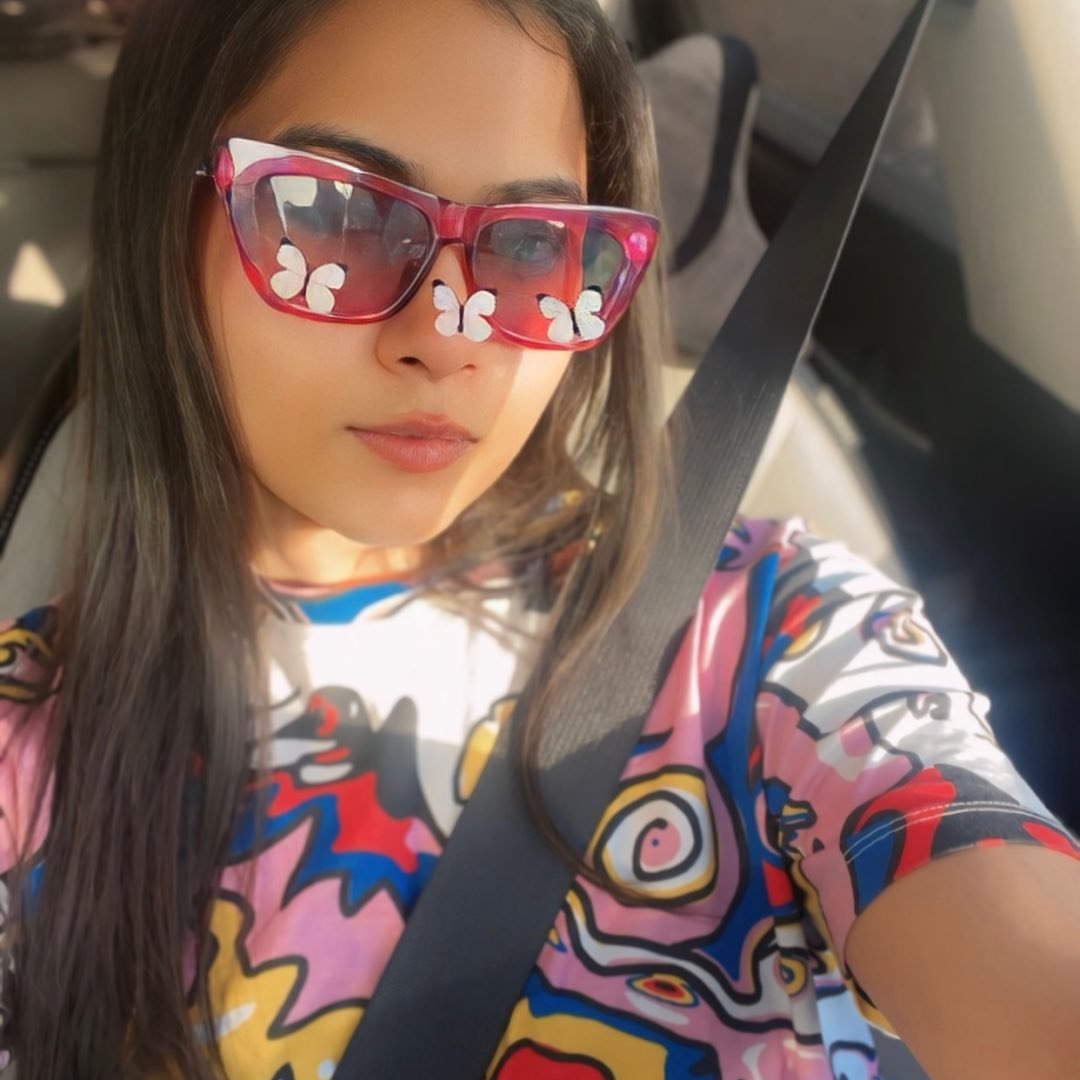
Table of contents
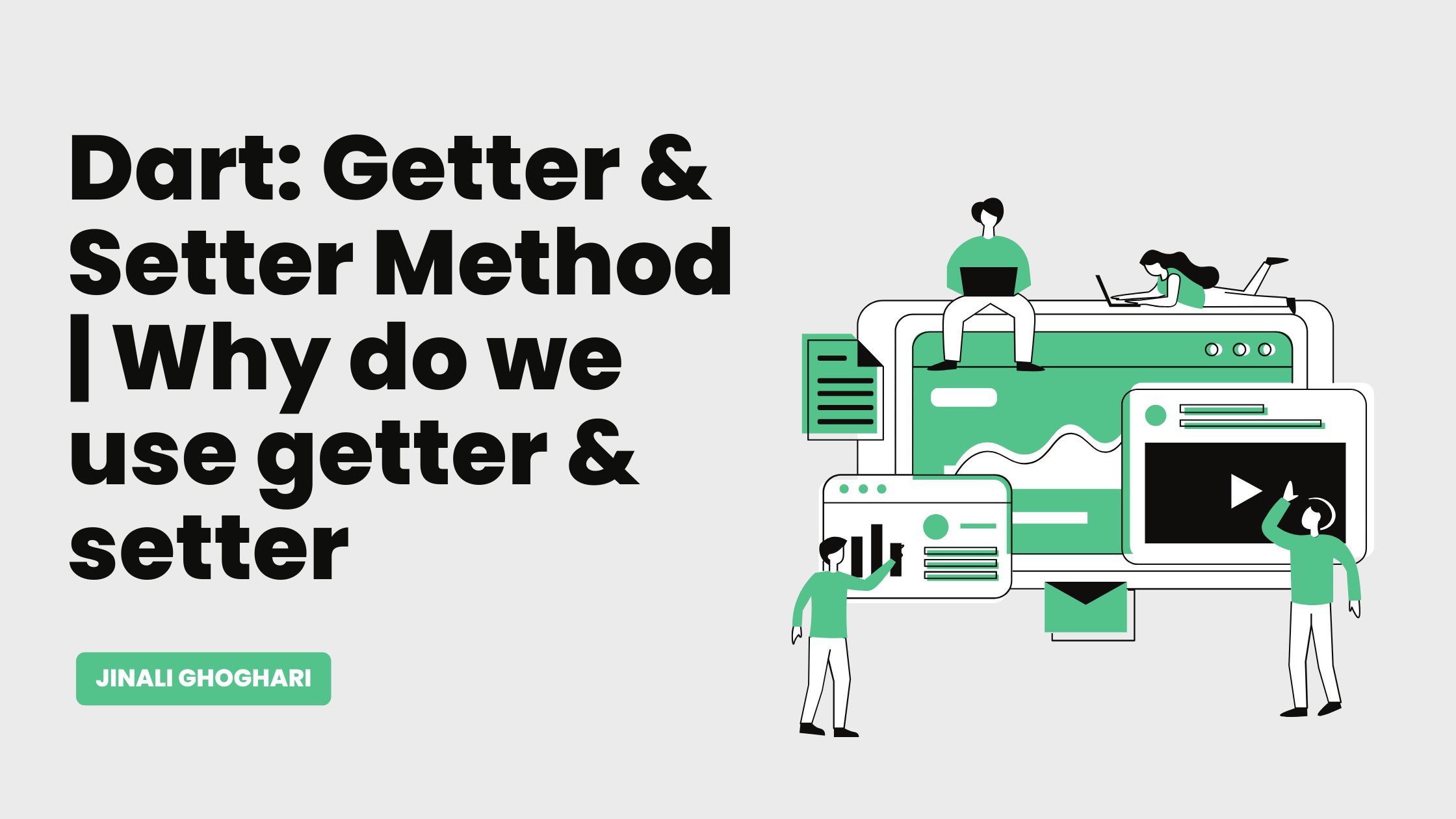
Getter and setter methods are the class methods used to manipulate the data of the class fields.
Getter is used to read or get the data of the class field whereas setter is used to set the data of the class field to some variable.
Getter Method in Dart:
It is used to retrieve a particular class field and save it in a variable. All classes have a default getter method but it can be overridden explicitly.
The getter method can be defined using the get keyword as:
return_type get field_name{
...
}
It must be noted we have to define a return type but there is no need to define parameters in the above method.
Setter Method in Dart:
It is used to set the data inside a variable received from the getter method. All classes have a default setter method but it can be overridden explicitly.
The setter method can be defined using the set keyword as:
set field_name{
...
}
Example:
class Person {
String _name; // Private field
int _age; // Private field
// Constructor
Person(this._name, this._age);
// Getter for _name
String get name => _name;
// Setter for _name
set name(String newName) => _name = newName;
// Setter for _age
set age(int newAge) => _age = newAge;
// Getter for _age
int get age => _age;
// Method to print the person's details
void printDetails() {
print('Name: $_name, Age: $_age');
}
}
void main() {
var person = Person('Jinali', 21);
// Accessing private fields using getters
print(person.name); // Output: Jinali
print(person.age); // Output: 21
// Using setters to update private fields
person.name = 'Reet';
person.age = 14;
person.printDetails(); // Output: Name: Reet, Age: 14
}
Why do we use getter & setter
They allow you to define custom behavior when getting (reading) or setting (writing) the value of an object's property.
Encapsulation: Getters and setters provide a way to encapsulate the internal representation of an object's state. By using getters and setters, you can hide the implementation details of how a property is stored or computed, and expose a clean interface for interacting with the object.
Controlled Access: Getters and setters allow you to control access to class fields. For example, you can perform validation or additional logic when setting a value, ensuring that it meets certain criteria before allowing it to be stored.
Computed Properties: Getters can be used to compute property values dynamically rather than storing them directly. This is useful for properties that depend on other properties or need to be calculated at runtime.
Data Binding and Observables: Getters and setters are commonly used in frameworks like Flutter for implementing data binding and observables. By using getters and setters, you can listen for changes to a property and trigger updates in the UI or other parts of the application.
Backward Compatibility: Getters and setters provide a way to evolve the API of a class without breaking existing code. If you later need to add validation or additional logic to a property, you can do so by converting the field to a getter/setter pair without changing the external interface of the class.
Subscribe to my newsletter
Read articles from Jinali Ghoghari directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
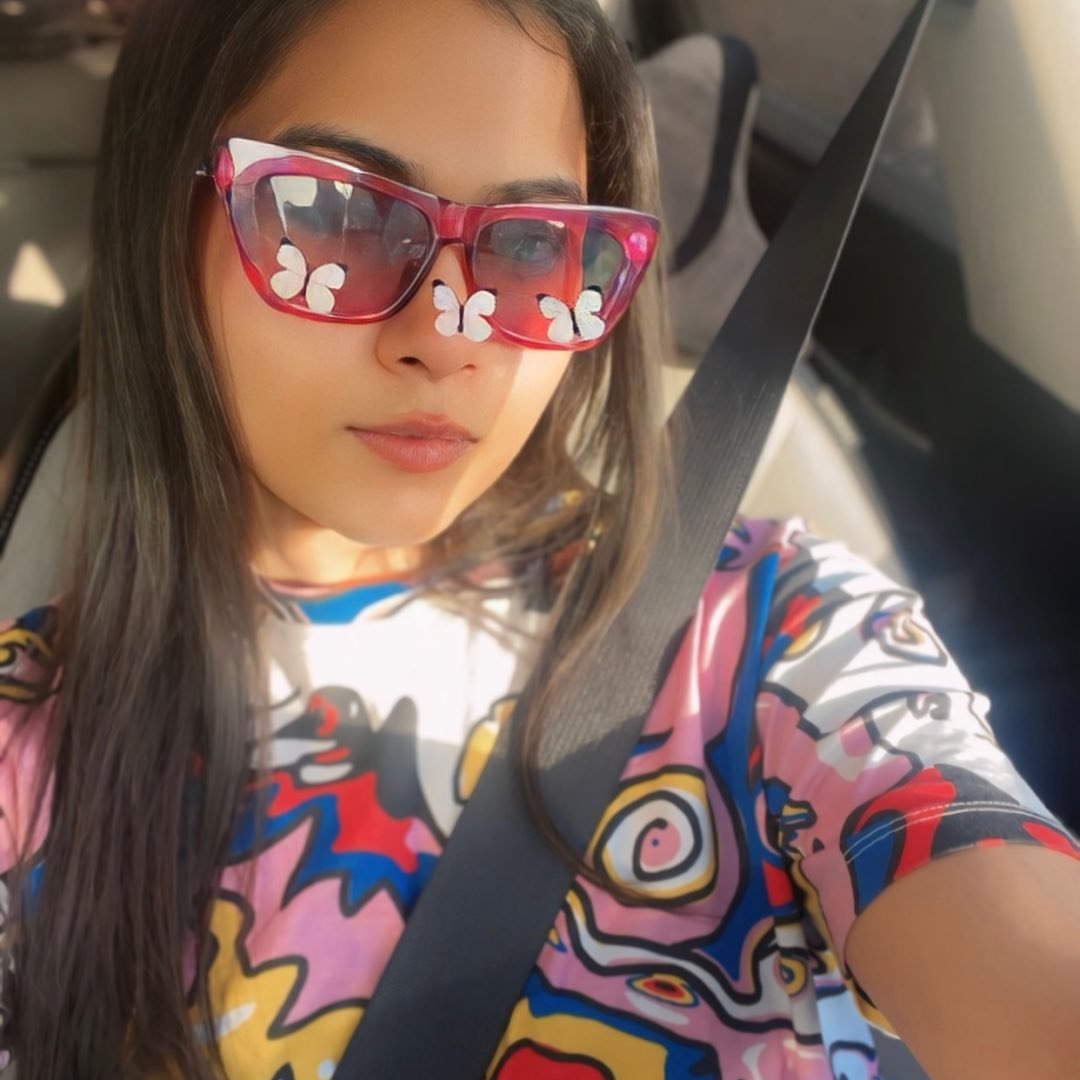