Dart Inheritance: Overriding Parent Method | Method Overriding
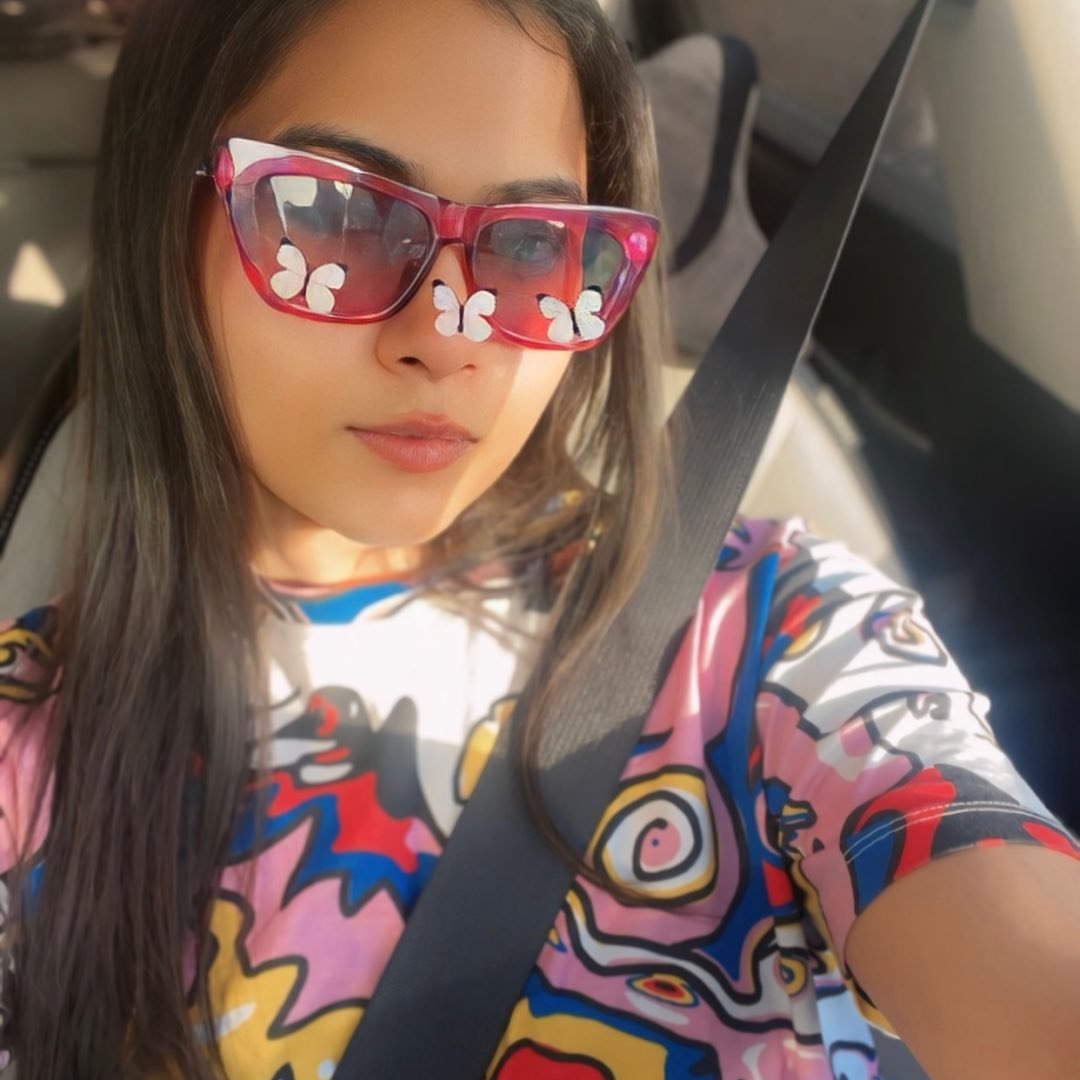
Table of contents
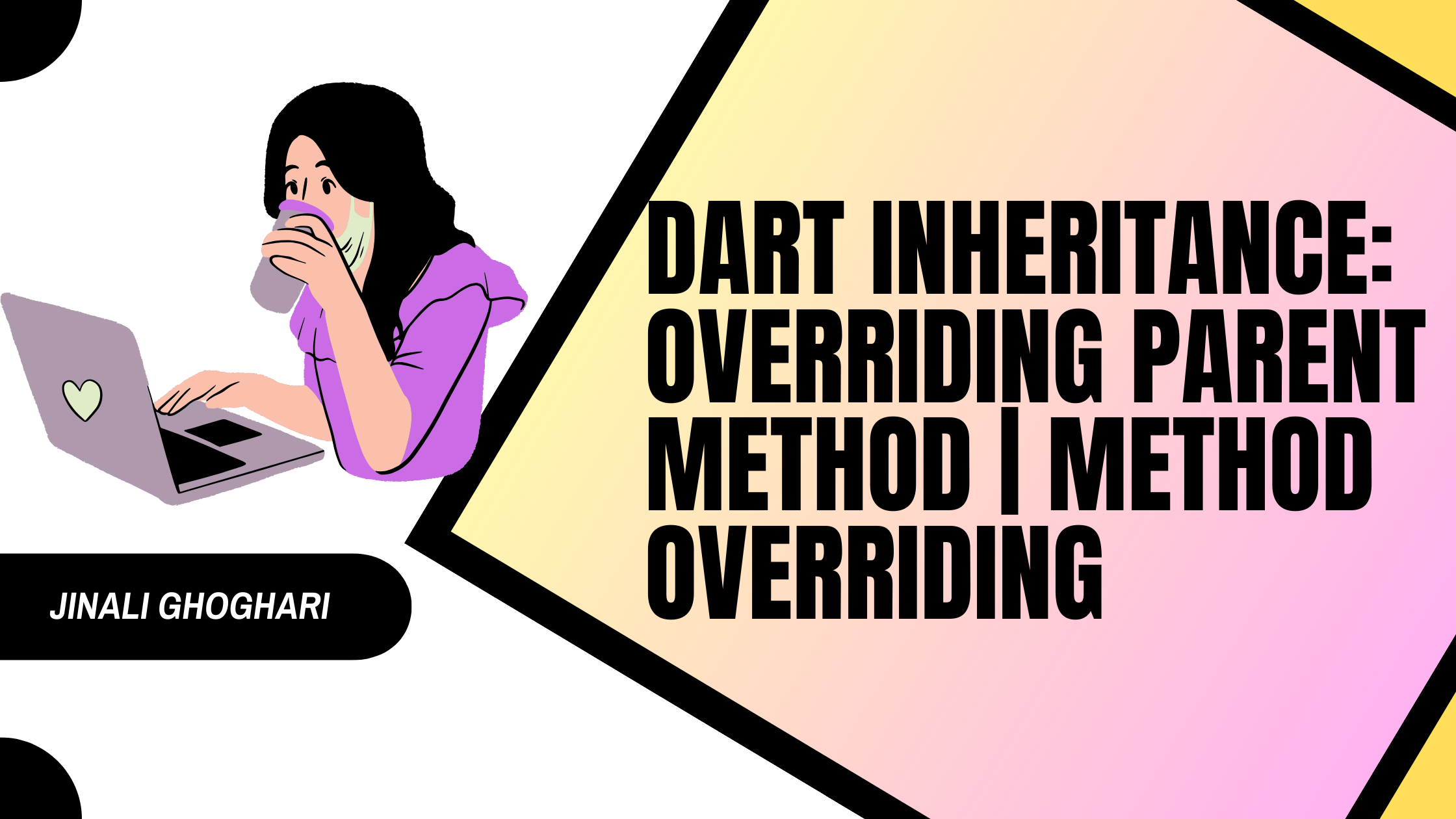
Method Overriding
Method overriding occurs in dart when a child class tries to override the parent class’s method.
When a child class extends a parent class, it gets full access to the methods of the parent class and thus it overrides the methods of the parent class.
This method is helpful when you have to perform different functions for a different child class, so we can simply re-define the content by overriding it.
Imagine you have a class called Animal
that has a method called makeSound()
which prints a generic sound like "Roar!". Now, you create a new class called Cat
that extends "Animal". But instead of making the same sound as all animals, you want your cat to make a different sound, like "Meow!". So, you override the makeSound()
method in the Cat
class to provide this new sound.
Important Points:
A method can be overridden only in the child class, not in the parent class itself.
Both the methods defined in the child and the parent class should be the exact copy, from name to argument list except the content present inside the method i.e. it can and can’t be the same.
A method declared final or static inside the parent class can’t be overridden by the child class.
Constructors of the parent class can’t be inherited, so they can’t be overridden by the child class.
Example
Let’s understand this concept by example. We have three classes here. One parent class is called Car and two child classes are called Jinali and Reet. Parent class has one method named showOwner().
So when showOwner() is called via the parent’s own object then it will have a different owner name. And when showOwner() is called via Jinali or Reet’s object then the same method has a different implementation. The simple example of method overriding… 😉
class Car {
void ShowOwner() => print("This is Parent's car");
}
class Jinali extends Car {
@override
void ShowOwner() {
// TODO: implement ShowOwner
print("This JG's car");
}
}
class Reet extends Car {
@override
void ShowOwner() {
// TODO: implement ShowOwner
print("This is Sanket's car");
}
}
main() {
Car parentCar = new Car();
Jinali jgCar = new Jinali();
Reet reetCar = new Reet();
parentCar.ShowOwner();
jgCar.ShowOwner();
reetCar.ShowOwner();
}
Output
This is Parent's car
This JG's car
This is Reet's car
Conclusion
So, method overriding allows you to customize or change the behavior of methods in child classes without altering the original code in the parent class. It's a way for child classes to give their own special twist to methods inherited from their parent class.
Subscribe to my newsletter
Read articles from Jinali Ghoghari directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
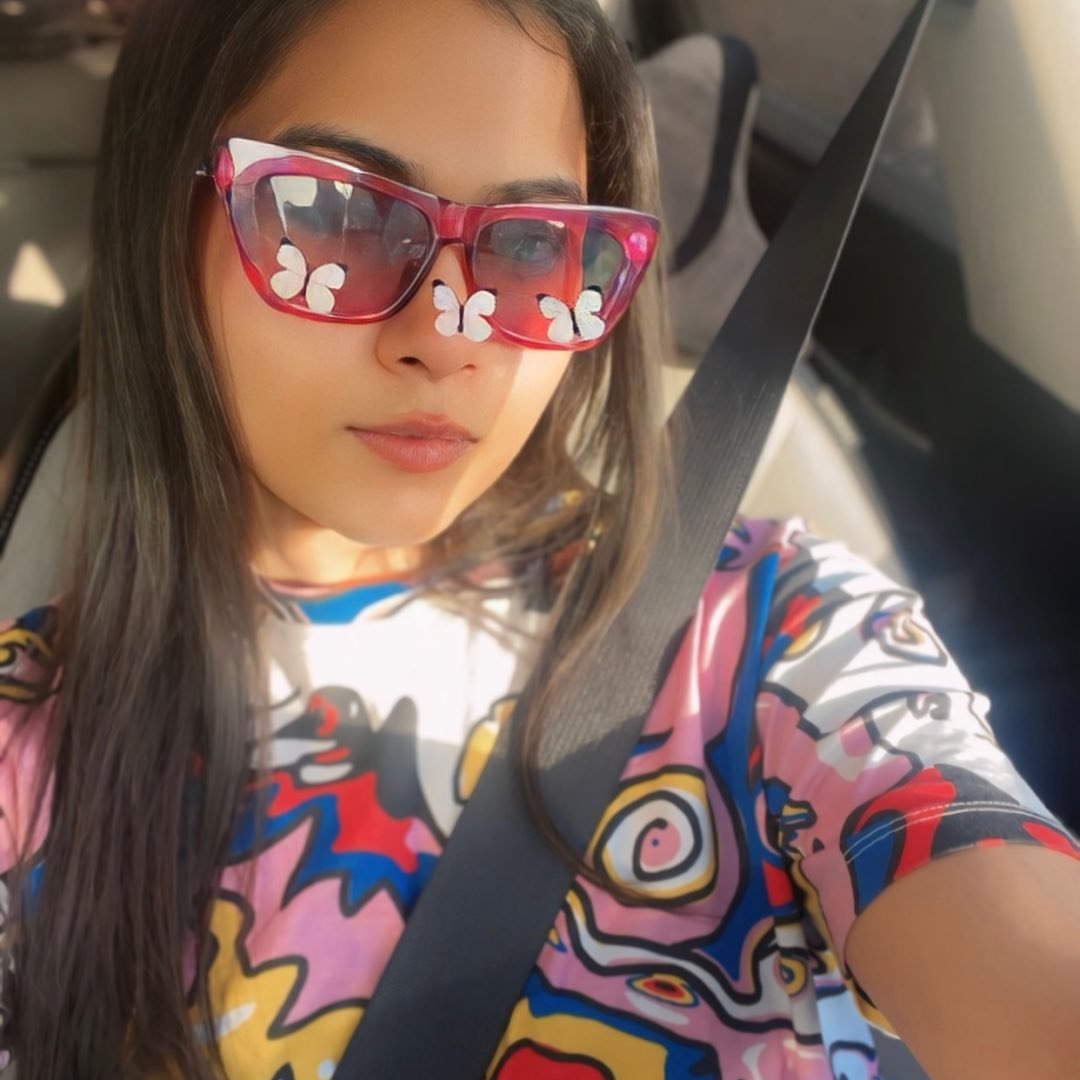