Week 0 Functions
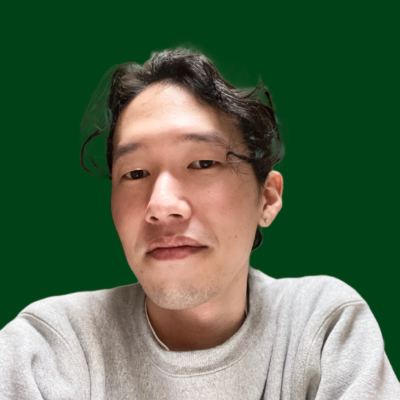
4 min read
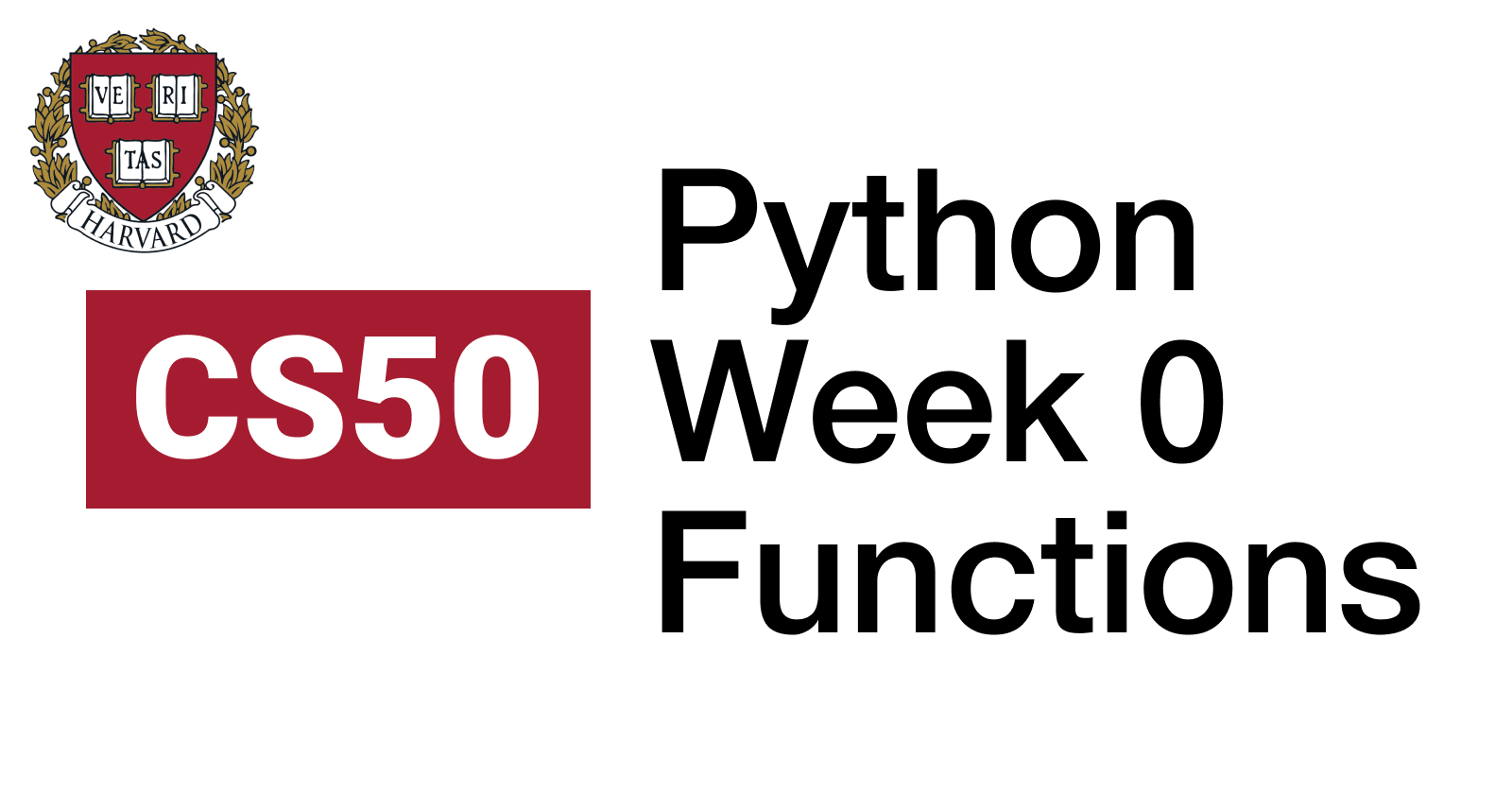
hello.py
code hello.py
print("hello, world")
Command-line Interface
python hello.py
Functions, Arguments, Side Effects
functions print() is a function
arguments inside of () a argument
side effects hello, world is a side effect
Bugs and Debugging
- bugs a mistake is a bug
Return Values and Variables
input("What's your name? ")
print("hello, world")
input("What's your name? ")
print("hello, David")
name = input("What's your name? ")
print("hello, David")
name = input("What's your name? ")
print("hello, name")
name = input("What's your name? ")
print("hello,")
print(name)
comments
# Ask user for their name
name = input("What's your name? ")
# Say hello to user
print("hello,")
print(name)
pseudocode
# Ask user for their name
# Say hello to user
multiline comments
#
#
#
```
```
# Ask user for their name
name = input("What's your name? ")
# Say hello to user
print("hello, " + name)
# Ask user for their name
name = input("What's your name? ")
# Say hello to user
print("hello,", name)
str = string
print(*objects, sep=’ ‘, end=’\n’)
docs.python.org/3/library/functions.html#print
# Ask user for their name
name = input("What's your name? ")
# Say hello to user
print("hello,", end="")
print(name)
# Ask user for their name
name = input("What's your name? ")
# Say hello to user
print("hello,", name, sep="???")
print(’hello, “friend”’)
print(”hello, \”friend\””)
# Ask user for their name
name = input("What's your name? ")
# Say hello to user
print(f"hello, {name}")
docs.python.org/3/library/stdtypes.html#string-methods
# Ask user for their name
name = input("What's your name? ")
# Remove whitespace from str
name = name.strip()
# Capitalize user's name
name = name.capitalize()
# another way and better way to capitalize
name = name.title()
# Say hello to user
print(f"hello, {name}")
# Ask user for their name
name = input("What's your name? ")
# Remove whitespace from str and capitalize user's name
name = name.strip().title()
# Say hello to user
print(f"hello, {name}")
# Ask user for their name
name = input("What's your name? ").strip().title()
# Say hello to user
print(f"hello, {name}")
# Ask user for their name
name = input("What's your name? ").strip().title()
# Split user's name into first name and last name
first, last = name.split(" ")
# Say hello to user
print(f"hello, {first}")
int = integer
+, -, *, /, %
interactive mode
python
exit()
code calculator.py
x = 1
y = 2
z = x + y
print(z)
python calculator.py
x = input("What's x? ")
y = input("What's y? ")
z = x + y
print(z)
python calculator.py
What's x? 1
What's y? 2
12
x = input("What's x? ")
y = input("What's y? ")
z = int(x) + int(y)
print(z)
x = int(input("What's x? "))
y = int(input("What's y? "))
print(x + y)
x = float(input("What's x? "))
y = float(input("What's y? "))
print(x + y)
python calculator.py
What's x? 1.2
What's y? 3.4
4.6
docs.python.org/3/library/functions.html#round
round(number[, ndigits])
x = float(input("What's x?"))
y = float(input("What's y?"))
z = round(x + y)
print(z)
python calculator.py
What's x? 1.2
What's y? 3.4
5
python calculator.py
What's x? 999
What's y? 1
1000
x = float(input("What's x?"))
y = float(input("What's y?"))
z = round(x + y)
print(f"{z:,}")
python calculator.py
What's x? 999
What's y? 1
1,000
x = float(input("What's x?"))
y = float(input("What's y?"))
z = x / y
print(z)
x = float(input("What's x?"))
y = float(input("What's y?"))
z = round(x / y, 2)
print(z)
x = float(input("What's x?"))
y = float(input("What's y?"))
z = x / y
print(f"{z:.2f}")
def
name = input("Whats's your name? ")
hello()
print(name)
def hello():
print("hello")
name = input("Whats's your name? ")
hello()
print(name)
def hello(to="world"):
print("hello,", to)
hello()
name = input("Whats's your name? ")
hello(name)
def main():
name = input("Whats's your name? ")
hello(name)
def hello(to="world"):
print("hello,", to)
main()
def main():
name = input("Whats's your name? ")
hello()
def hello():
print("hello,", name)
main()
def main():
x = int(input("What's x? "))
print("x sqaured is", square(x))
def square(n):
return n * n
return n ** 2 # same
return pow(n, 2) # same
main()
0
Subscribe to my newsletter
Read articles from Natsuki directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
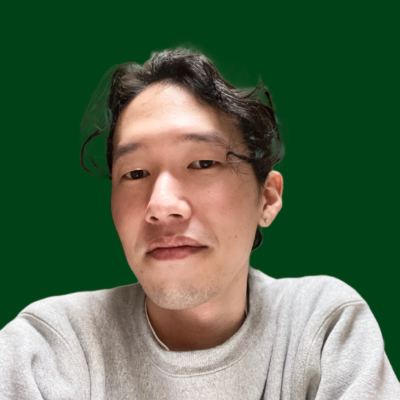