⚛️ Folder Structures in React Projects

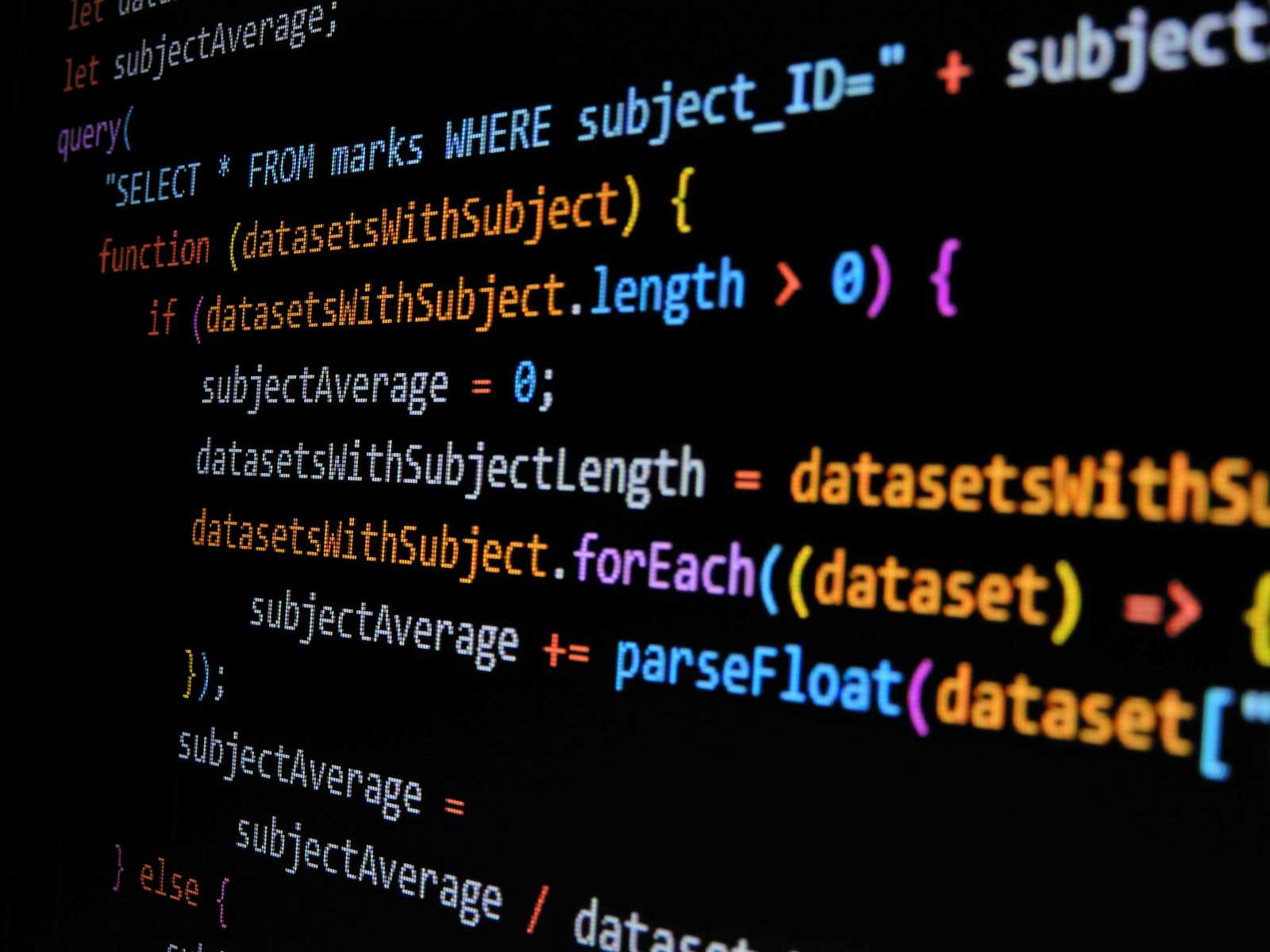
#react#webdev#javascript#typescript
Organizing files and directories within a React project is crucial for maintainability, scalability, and ease of navigation. This article explores the general architecture and folder structures across different scales of React projects, providing clear demonstrations for each level.
1️⃣ Level 1: Grouping by "File Types"
This structure is characterized by its simplicity - grouping files by their type:
└── src/
├── assets/
├── api/
├── configs/
├── components/
│ ├── SignUpForm.tsx
│ ├── Employees.tsx
│ ├── PaymentForm.tsx
│ └── Button.tsx
├── hooks/
│ ├── usePayment.ts
│ ├── useUpdateEmployee.ts
│ ├── useEmployees.ts
│ └── useAuth.tsx
├── lib/
├── services/
├── states/
└── utils/
Let's say youhave a lot of code revolving around payment. One day the whole business changes or is no longer needed, how easy is it to replace or remove it? With this folder structure, you'll have to go through every folder and the files inside it to make the necessary changes. And if the project keeps growing larger, it'll soon grow into a maintenance hell that will only get worse over time.
2️⃣ Level 2: Grouping by "File Types" and Features
As projects grow, the "Level 2" structure introduces grouping by feature within each type:
└── src/
├── assets/
├── api/
├── configs/
├── components/
│ ├── auth/
│ │ └── SignUpForm.tsx
│ ├── payment/
│ │ └── PaymentForm.tsx
│ ├── common/
│ │ └── Button.tsx
│ └── employees/
│ ├── EmployeeList.tsx
│ └── EmployeeSummary.tsx
├── hooks/
│ ├── auth/
│ │ └── useAuth.ts
│ ├── payment/
│ │ └── usePayment.ts
│ └── employees/
│ ├── useEmployees.ts
│ └── useUpdateEmployee.ts
├── lib/
├── services/
├── states/
└── utils/
-
- Logic relatedto a feature is stillspread across multiple folder types
Now let's come back to the problem statement where the payment module needs to be modified or removed. With this structure, it's a lot easier to do that now.
The "Level 2"folder structure is the one that I'd recommend if you don't know what to choose.
3️⃣ Level 3: Grouping by Features/Modules
For larger projects, the "Level 3" structure offers a highly modular approach, defining clear boundaries for different aspects of the application within each module:
└── src/
├── assets/
├── modules/
| ├── core/
│ │ ├── components/
│ │ ├── design-system/
│ │ │ └── Button.tsx
│ │ ├── hooks/
│ │ ├── lib/
│ │ └── utils/
│ ├── payment/
│ │ ├── components/
│ │ │ └── PaymentForm.tsx
│ │ ├── hooks/
│ │ │ └── usePayment.ts
│ │ ├── lib/
│ │ ├── services/
│ │ ├── states/
│ │ └── utils/
│ ├── auth/
│ │ ├── components/
│ │ │ └── SignUpForm.tsx
│ │ ├── hooks/
│ │ │ └── useAuth.ts
│ │ ├── lib/
│ │ ├── services/
│ │ ├── states/
│ │ └── utils/
│ └── employees/
│ ├── components/
│ │ ├── EmployeeList.tsx
│ │ └── EmployeeSummary.tsx
│ ├── hooks/
│ │ ├── useEmployees.ts
│ │ └── useUpdateEmployee.ts
│ ├── services/
│ ├── states/
│ └── utils/
└── ...
-
Features/Modules are clear representations of objects in the real world
Disadvantages:
- You'll have tobe well-aware of thebusiness logic to make the right grouping decisions
With this, ifyou are to remove or modify the payment logic, you'll know right away where to start.
Give Consistent Meanings to Folder Names
Regardless ofthe structure level, certain folder names should carry specific meanings. What a folder name means may vary based on your preferences or the project's conventions.
Here's what Iusually think about folder names:
UI Components
components: React components - the main UI building blocks.
design-system: Fundamental UI elements and patterns based on the design system.
icons: SVG icons that are meant to be used inline.
React Specific
hocs: React Higher-order Components.
contexts/providers: Contains React Contexts and Providers.
Utilities & External Integrations
utils:Utilities for universal logicthat is not related to business logic or any technologies, e.g. string manipulations, mathematic calculations, etc.
lib: Utilities that are related to certain technologies, e.g. DOM manipulations, HTML-related logic, localStorage, IndexedDB, etc.
plugins: Third-party plugins (e.g. i18n, Sentry, etc.)
Business Logic
services: Encapsulates main business& application logic.
helpers: Provides business-specificutilities.
Styles
TypeScript and Configurations
configs: Configs for the application(e.g. environment variables)
constants: Constant, unchanged values (e.g.
exp
ort const MINUTES_PER_HOUR = 60
).
Server Communication
State Management
states/store:Globalstate management logic (Zustand, Valtio, Jotai, etc.)
reducers, store, actions, selectors:Redux-specific logic
Routing
routes/router: Defining routes (if you're using React Router or the like).
pages: Defining entry-point components for pages.
Testing
Subscribe to my newsletter
Read articles from Syed Maaz Saeed directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Syed Maaz Saeed
Syed Maaz Saeed
Front-End Web Developer | MERN Stack Enthusiast | 🎓Software Engineer | 🐧 Linux Lover| Programmer | offering services based on the MERN technology stack.