Stumped on Generating Data for Your Project?
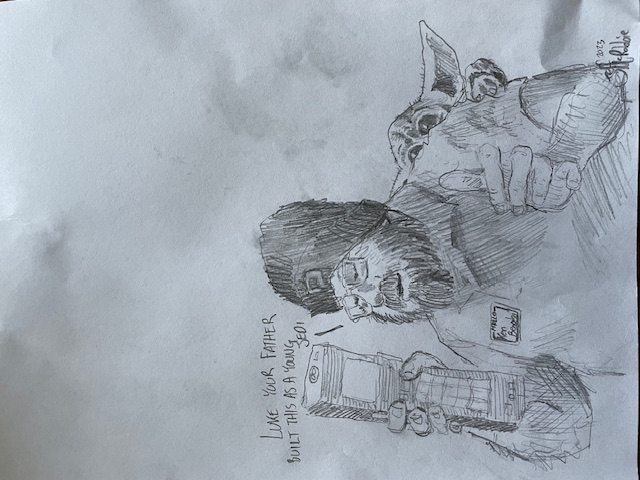
Have you ever worked on a coding project and you needed to seed your database, but couldn't think of how to come up with your data? Instead of using the same things over and over, there is a Python package called Faker that can generate fake data for almost anything you need! Faker is heavily inspired by PHP Faker, Perl Faker and Ruby Faker.
Faker Version 5.0.0 supports only supports Python 3.7 and above.
How-to-use:
From your terminal you will want to install Faker with pip install faker
Now you can use faker in your coding. Remember to import it as you normally would by from faker import Faker
and then you can use fake = Faker()
to create and initialize a faker generator, which will be able to generate the data you want by naming the type of data you want, and there can be a lot of things you can think of!
Here are some examples:
What is really cool is that each call to the method fake.name()
will result in a different (random) result! This happens because Faker forwards calls from faker.Generator.method_name()
to faker.Generator.format(method_name)
so if you needed 5 random names:
You can also use Faker's pytest plugin that will provide a faker fixture that you can use in your own tests. There is more information on this at
Welcome to Faker’s documentation! — Faker 24.4.0 documentationpytest fixture docs
faker.Faker
can also take in a locale as an argument, to return localized data. If a localized provider cannot be found, it goes back to the default LCID (Locale Indentifier or Locale ID) string for US English (en_US). This can also support multiple locales. (It was new in v3.0.0) here is an example:
*snippet credit to faker.readthedoc.io
The localization of Faker is ongoing, so don't hesitate to create a localized provider for your own locale and submit a Pull Request.
Faker also can optimize real-world frequencies by taking a performance-related argument called use_weighting
. For example: the English name Harry would be much more frequent than Severus. If use_weighting
was set to False
, then all items would have an equal chance of being selected, and the selection process is faster. The default is set to True
.
Once installed, you can use Faker from the command line:
So lets break this down:
faker
: is the script when installed in your environment, in development you could usepython -m faker
instead-h
,--help
: shows a help message--version
: shows the program’s version number-o FILENAME
: redirects the output to the specified filename-l {bg_BG,cs_CZ,...,zh_CN,zh_TW}
: allows use of a localized provider-r REPEAT
: will generate a specified number of outputs-s SEP
: will generate the specified separator after each generated output-i {my.custom_provider other.custom_provider}
list of additional custom providers to use. Note that is the import path of the package containing your Provider class, not the custom Provider class itself.fake
: is the name of the fake to generate an output for, such asname
,address
, ortext
[fake argument ...]
: optional arguments to pass to the fake (e.g. the profile fake takes an optional list of comma separated field names as the first argument)
You can use for loops, and iterate through arrays to generate unique values with the use of the unique property on the generator:
Here is another way you can use Faker for your projects:
Overall, Faker can be a great tool in your coding projects, and there is even more tools available both in creating providers and dynamic providers. Customizing your desired results and also more with setting up your own tests. You can read more in depth if you are interested at https://faker.readthedocs.io
I hope you enjoyed this read and hopefully this will help you in your coding adventures!
Subscribe to my newsletter
Read articles from Kenny McClelland directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
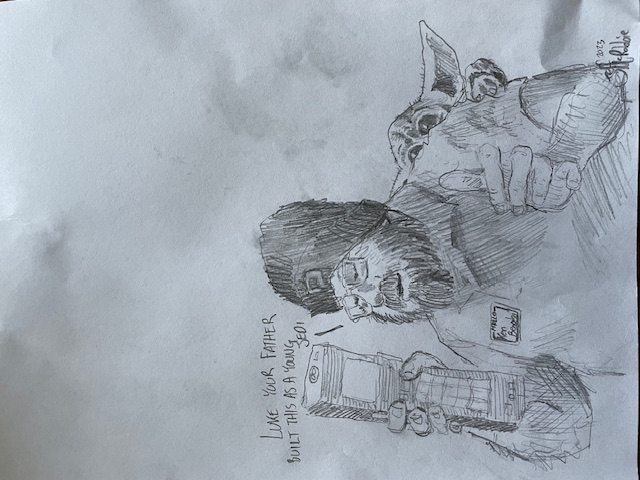
Kenny McClelland
Kenny McClelland
I am a full-time Software Engineering student with Flatiron school, and part-time CSA with Lowe's.