Solving CS50 Python - Problem Set 1
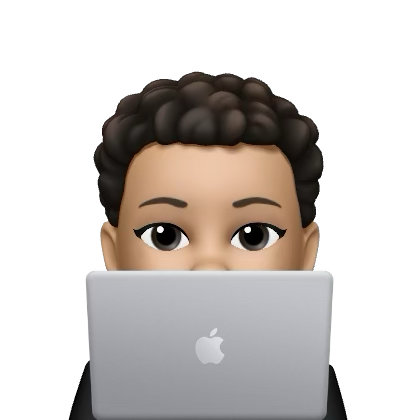
Table of contents
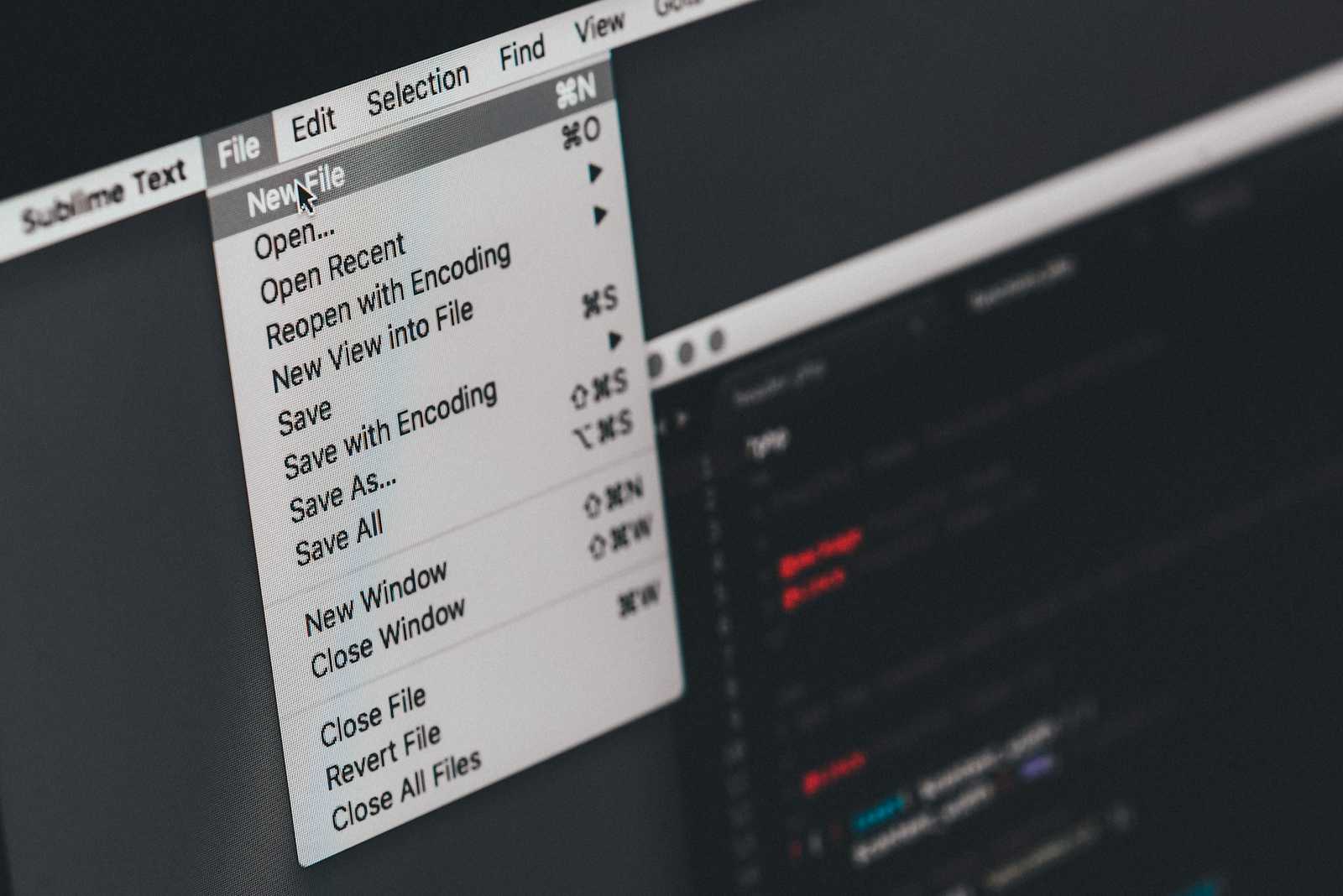
Hi everyone. Today, I present to you my solutions to CS50P Problem Set 1. I hope you utilize them to explore different approaches to the same problem.
Home Federal Savings Bank
I implemented a program that prompts the user for a greeting as input. It evaluates if the greeting is “hello” or starts with an “h” (but not hello) or otherwise. It then prints the amount of money according to the greeting.
Solution:
greeting = input("Greeting: ").lower().strip()
if greeting.startswith("hello"):
print("$0")
elif greeting.startswith("h"):
print("$20")
else:
print("$100")
Deep Thought
I implemented a program that prompts the user for input, asking the question: "What is the Answer to the Great Question of Life, the Universe and Everything?". If the user inputs “42”, forty-two, or “forty two” case-insensitively. The program outputs “yes”, otherwise “no”.
Solution:
def main():
answer = input("What is the Answer to the Great Question of Life, the Universe and Everything? ").lower().strip()
if check_answer(answer):
print("Yes")
else:
print("No")
# check if answer is correct or not
def check_answer(a):
if a == "42":
return True
elif a == "forty-two":
return True
elif a == "forty two":
return True
else:
return False
main()
File Extensions
I created a program that asks the user to input a file name. It identifies the suffix of the input and outputs which type of file it is.
Solution:
file_name = input("File name: ").strip().lower()
if file_name.endswith("gif"):
print("image/gif")
elif file_name.endswith("jpg") or file_name.endswith("jpeg"):
print("image/jpeg")
elif file_name.endswith("png"):
print("image/png")
elif file_name.endswith("pdf"):
print("application/pdf")
elif file_name.endswith("txt"):
print("text/plain")
elif file_name.endswith("zip"):
print("application/zip")
else:
print("application/octet-stream")
Math Interpreter
I created a program that asks the user to input an arithmetic expression and calculates and outputs the result.
Solution:
# prompt user for arithemtic expression
def main():
expression = input("Expression: ")
#split expression to 3 parts
x,y,z = expression.split(" ")
# convert to float
x = float(x)
z = float(z)
# convert str operator to operator
operator = str_to_operator(y)
if operator:
ans = operator(x, z)
print(ans)
else:
None
import operator
def str_to_operator(o):
if o == "+":
return operator.add
elif o == "-":
return operator.sub
elif o == "*":
return operator.mul
elif o == "/":
return operator.truediv
elif o == "^":
return operator.xor
elif o == "%":
return operator.mod
else:
return None
main()
Subscribe to my newsletter
Read articles from Karabo Molefi directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
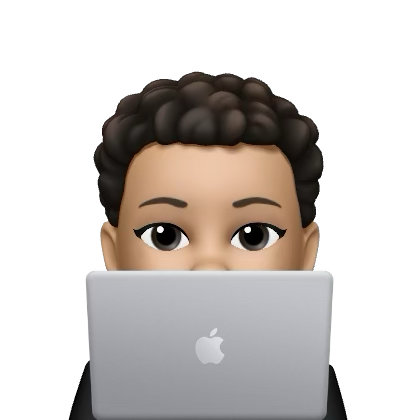