Dart: Difference between static method and instance method
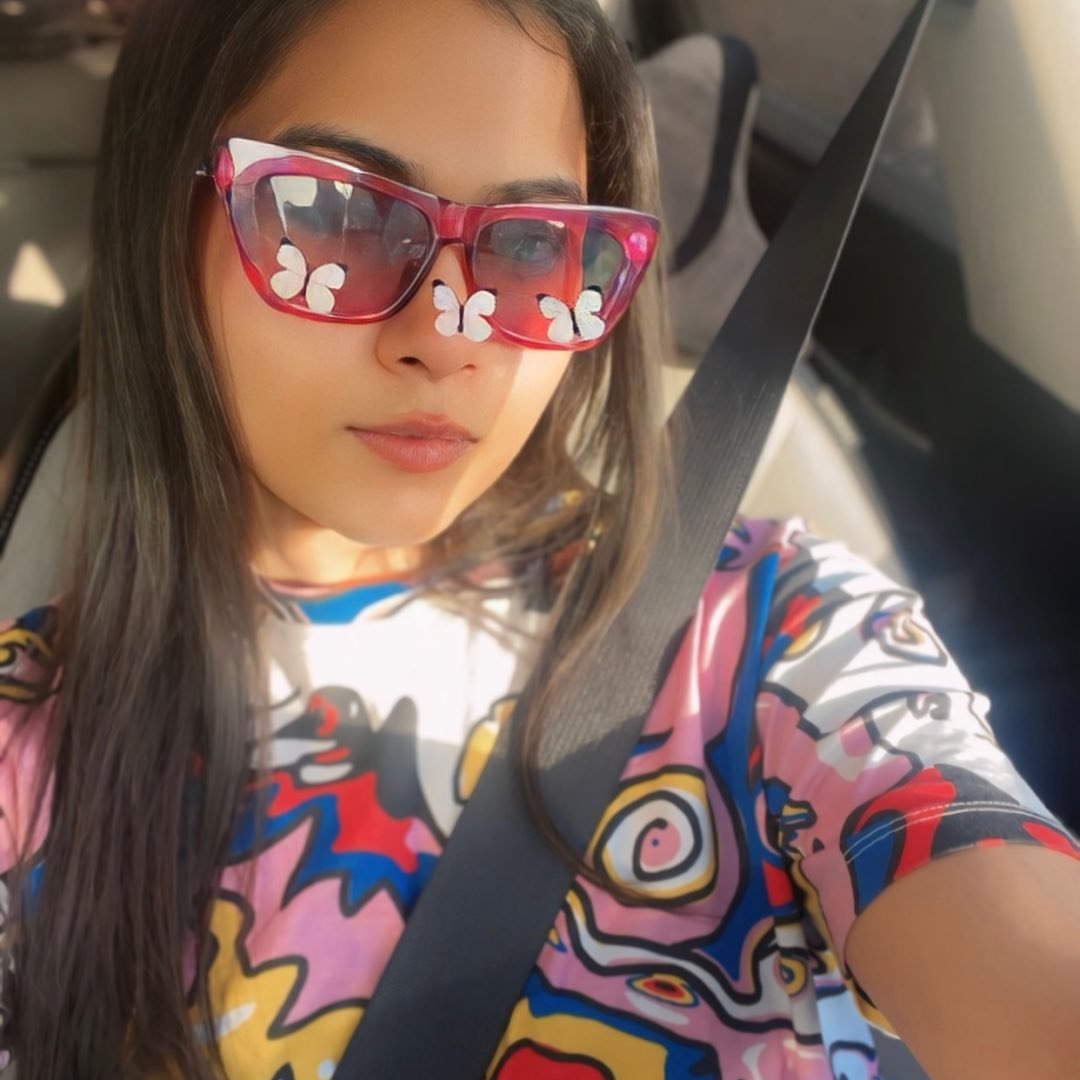
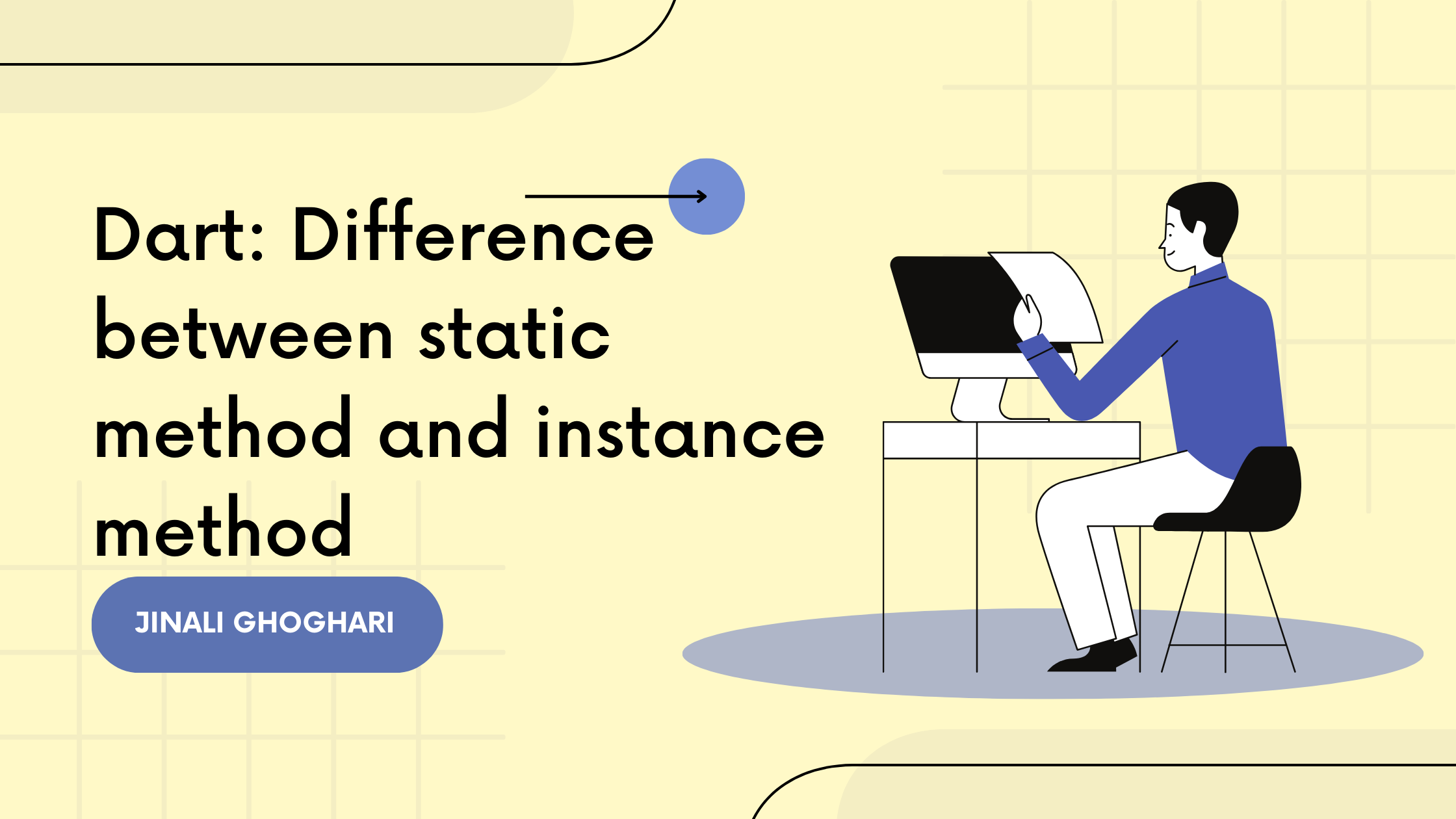
Let's compare static methods and instance methods in Dart and illustrate their differences in a table:
Instance Method:
Instance methods are associated with an instance of a class. They have access to the instance's properties and can modify them.
These methods are invoked on an object of the class using dot notation.
Instance methods can be overridden in subclasses.
They can access instance variables and other instance methods directly.
Here's a simple example:
// Define a class named Counter
class Counter {
// Instance variable to hold the counter value
int value = 0;
// Instance method to increment the counter value
void increment() {
value++; // Incrementing the counter value
}
}
// Main function, the entry point of the program
void main() {
// Creating an instance of the Counter class
var myCounter = Counter();
// Invoking the increment method on the instance
myCounter.increment(); // Incrementing the counter value
// Printing the current value of the counter
print(myCounter.value); // Output: 1
}
Static Method:
Static methods are associated with the class itself rather than any particular instance.
They cannot access instance variables or methods directly because they are not tied to any specific instance.
Invoked using the class name directly, without creating an instance.
Static methods can be used for utility functions or operations that don't rely on instance-specific data.
Here's an example:
dartCopy code// Define a class named MathUtils
class MathUtils {
// Static method to add two numbers
static int add(int a, int b) {
return a + b; // Returning the sum of the two numbers
}
}
// Main function, the entry point of the program
void main() {
// Calling the static add method of MathUtils class
var result = MathUtils.add(2, 3); // Adding 2 and 3
// Printing the result of the addition
print(result); // Output: 5
}
In summary, instance methods are tied to instances of a class and can access instance variables, while static methods belong to the class itself and cannot access instance-specific data. They are useful for different purposes and contexts within a program.
Subscribe to my newsletter
Read articles from Jinali Ghoghari directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
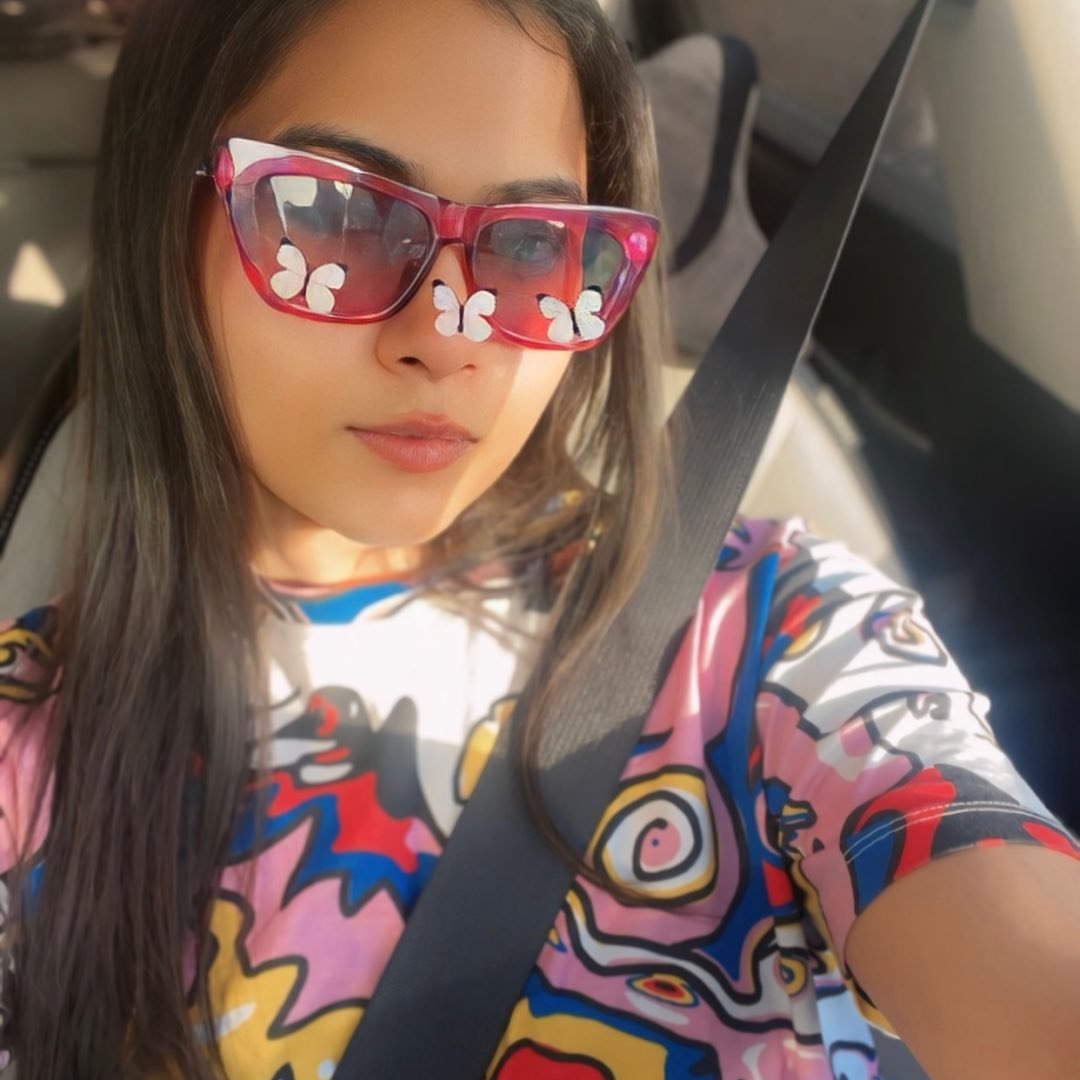