Introduction to String Matching Algorithms
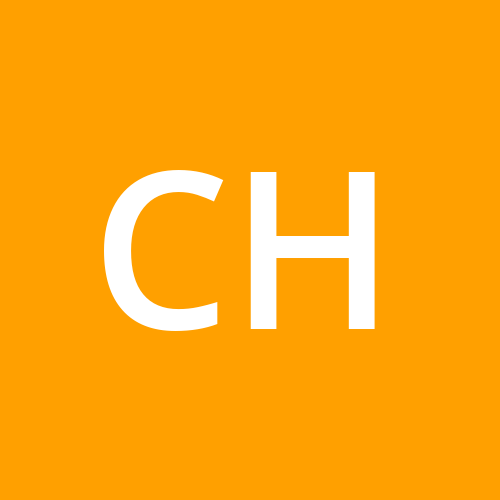
String matching is a fundamental problem in computer science. Simply put, string matching involves searching for patterns within a text. String-matching algorithms are used to find occurrences of a specified pattern (or substring) within a larger text (or string). These algorithms can be found in fields such as:
Information retrieval: String matching algorithms are used in search engines, databases, and information retrieval systems to efficiently locate relevant documents, records, or data based on user queries or patterns.
Text Processing: Many text-processing tasks, such as parsing, syntax highlighting, spell checking, and natural language processing, rely on string-matching algorithms to identify and manipulate specific patterns or language constructs within text.
Bioinformatics: String matching algorithms are used to analyse biological sequences (e.g., DNA, RNA, protein sequences) for sequence alignment, similarity search, and gene pattern identification.
Data mining and Pattern Recognition: String matching algorithms are used to identify trends, patterns, or anomalies within large datasets, enabling insights and decision-making.
Network Security: String matching plays a crucial role in network security, intrusion detection, and firewall systems by inspecting network traffic, identifying malicious patterns (e.g., virus signatures, attack patterns), and enforcing security policies.
Understanding how string matching works is essential for anyone working in a field that requires manipulating and analysing large amounts of data.
Now that we know what string-matching is and its significance to computer science out of the way, welcome to the first instalment in our series on string-matching algorithms! Over the upcoming articles, we'll delve into a variety of algorithms each with its advantages and drawbacks.
This article marks the beginning of our exploration into various types of string-matching algorithms. To start, let's dive into the naive string-matching algorithm.
Naive String Matching algorithm
The naive string-matching algorithm is the most simple and intuitive string-matching algorithm. No wonder we are starting our exciting ride into the wonders of string-matching here.
This straightforward approach checks for a match with the target string at every possible position in the text. Simple, right? Right.
Find the Index of the First Occurrence in a String.
Given two strings, text and pattern, return the index of the first occurrence of pattern in the text, or -1 if the pattern is not part of the text.
def find_first_index(text: str, pattern: str) -> int:
text_len, pattern_len = len(text), len(pattern)
for i in range(text_len - pattern_len + 1):
for j in range(pattern_len):
if pattern[j] != text[i + j]:
break
if j == pattern_len - 1:
return i
return -1
text = ‘ABCBADC’
pattern = ‘BAD’
index = find_first_index(text, pattern) # 3
Pattern found at the 3rd index
text = 'mississippi’
pattern = ‘issip’
index = find_first_index(text, pattern) # 4
Pattern found at the 4th index
Time and Space complexity
In the worst-case scenario, the time complexity of the naive string-matching algorithm is O((m - n + 1) * n).
Where m
is the length of the text and n
is the length of the pattern.
The worst-case scenario occurs when the pattern is not found in the text or is found at the end of the text. In this case, the algorithm will make comparisons for each position of the text with the pattern, resulting in a quadratic time complexity.
The space complexity is O(1).
The naive string-matching algorithm operates in place, meaning it does not require any additional space proportional to the input size. It only uses a constant amount of space for variables and temporary storage.
Despite its simplicity, checking for a match this way is NOT efficient for large text and pattern sizes.
More Efficient String Matching Algorithms
There are more efficient string-matching algorithms such as:
Exact String-matching algorithms: such as
Rabin-Karp Algorithm: which efficiently utilises hashing for pattern matching.
Knuth-Morris-Pratt (KMP) Algorithm: which employs a failure function to avoid unnecessary comparisons.
Boyer-Moore Algorithm: which uses mismatched character information to skip unnecessary comparisons.
Approximate String Matching Algorithms: such as Levenshtein distance.
Advanced String Matching Algorithms such as Aho-Corasick Algorithm, Suffix Trees and Suffix Arrays.
Conclusion
In this article, we covered the basics of string matching algorithms, their importance, and where they are used, such as in information retrieval, text processing, bioinformatics, data mining, pattern recognition, and network security.
We learned that string matching involves searching for a pattern within a larger text, and understanding this is essential for anyone working with large amounts of data.
We explored the naive string-matching algorithm, its simplicity, and how it operates, as well as its time and space complexity.
We also briefly mentioned more efficient string-matching algorithms like the Rabin-Karp, Knuth-Morris-Pratt, Boyer-Moore, Levenshtein distance, Aho-Corasick, Suffix Trees and Suffix Arrays.
This understanding lays the groundwork for our further exploration into more complex string-matching algorithms.
References
Subscribe to my newsletter
Read articles from Chidinma directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
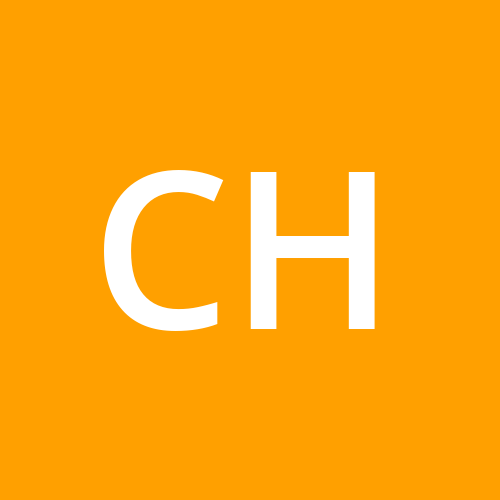
Chidinma
Chidinma
Software engineer in the Netherlands. I am committed to continuous learning and sharing knowledge. I write about topics related to data structures -•- algorithms -•- distributed systems -•- software architecture -•- and more.