Laravel - Import Existing Records
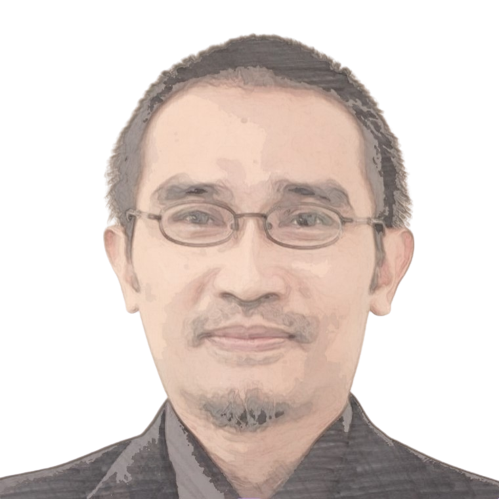
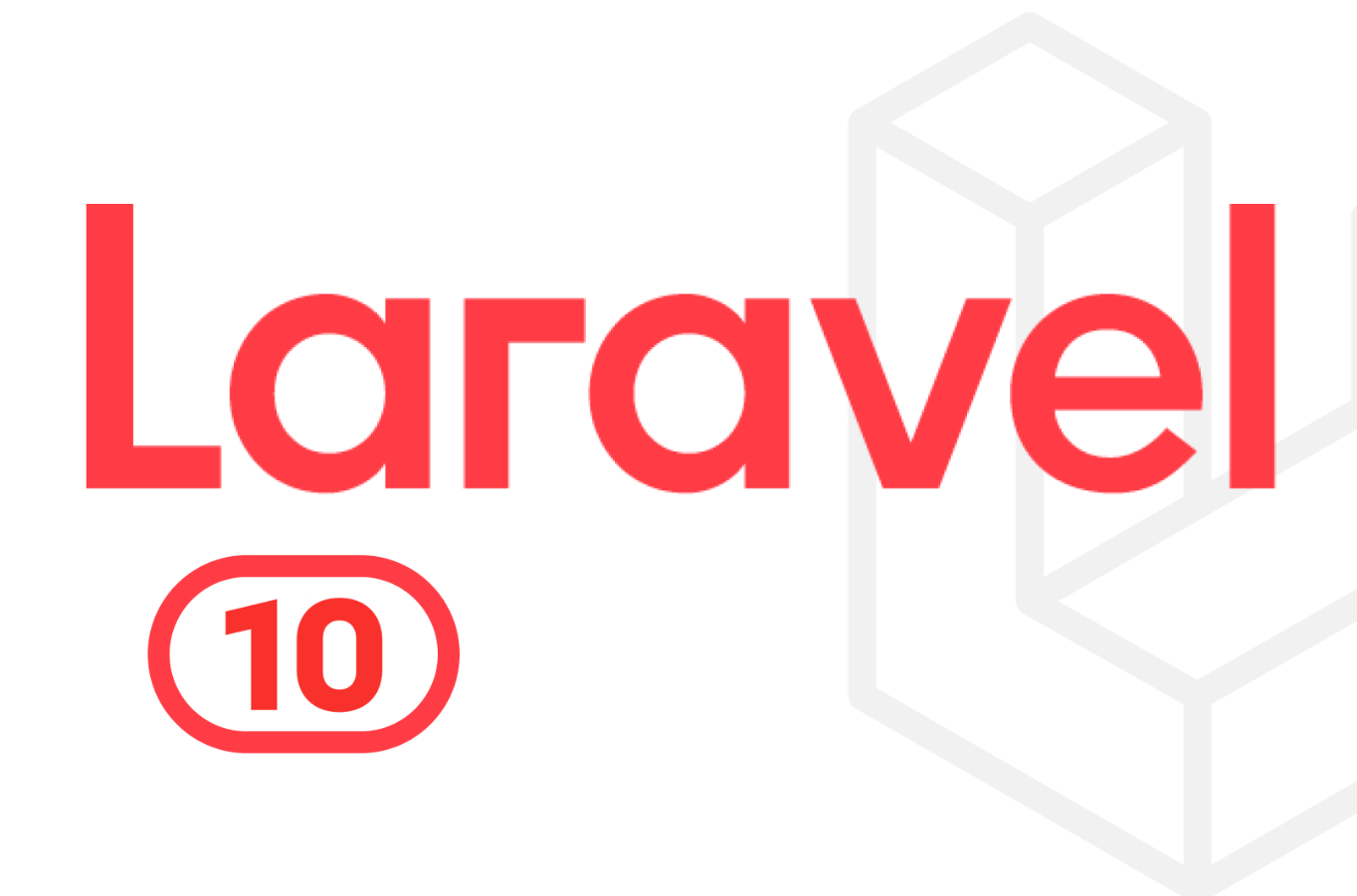
Some records may have existed prior to the project creation. This tutorial demonstrates the steps to import the records and build a page to access them.
[0] Prep
Continue from previous article or download quickstart file.
[1] Migration Tasks
[1.1] Create migration file
php artisan make:migration create_members_table --create=members
Output:
[1.2] Edit migration file
Assuming we have the following DDL:
CREATE TABLE "members" (
"id" INTEGER,
"phid" VARCHAR,
"hid" VARCHAR,
"name" VARCHAR,
"email" VARCHAR NOT NULL,
"phone" VARCHAR,
"admn" INTEGER,
"mngr" INTEGER,
"cord" INTEGER,
"modr" INTEGER,
"oper" INTEGER,
PRIMARY KEY("id" AUTOINCREMENT)
)
Edit migration file as follows:
File C:\laragon\www\razzi\database\migrations\2024_03_31_062201_create_members_table.php
:
<?php
use Illuminate\Database\Migrations\Migration;
use Illuminate\Database\Schema\Blueprint;
use Illuminate\Support\Facades\Schema;
return new class extends Migration
{
/**
* Run the migrations.
*/
public function up()
{
Schema::create('members', function (Blueprint $table) {
$table->id();
$table->string('phid')->nullable();
$table->string('hid')->nullable();
$table->string('name')->nullable();
$table->string('email')->nullable(false);
$table->string('phone')->nullable();
$table->integer('admn')->nullable();
$table->integer('mngr')->nullable();
$table->integer('cord')->nullable();
$table->integer('modr')->nullable();
$table->integer('oper')->nullable();
$table->timestamps();
});
}
/**
* Reverse the migration.
*
* @return void
*/
public function down()
{
Schema::dropIfExists('members');
}
};
[1.2] Create model
php artisan make:model Member
Output:
Edit
File C:\laragon\www\razzi\app\Models\Member.php
:
<?php
namespace App\Models;
use Illuminate\Database\Eloquent\Factories\HasFactory;
use Illuminate\Database\Eloquent\Model;
class Member extends Model
{
use HasFactory;
/* specify table name (optional). */
protected $table = 'members';
/* specify fillabel fields. */
protected $fillable = [
'phid',
'hid',
'name',
'email',
'phone',
'admn',
'mngr',
'cord',
'modr',
'oper',
];
}
[1.3] Run migration
php artisan migrate
Output:
[2] Import CSV data
Generate a new command using the Artisan command:
php artisan make:command ImportCsvData
Output:
Edit the import command:
File C:\laragon\www\razzi\app\Console\Commands\ImportCsvData.php
:
<?php
namespace App\Console\Commands;
use Illuminate\Console\Command;
use Illuminate\Support\Facades\DB;
class ImportCsvData extends Command
{
/**
* The name and signature of the console command.
*
* @var string
*/
protected $signature = 'app:import-csv-data {file} {--table=members}';
/**
* The console command description.
*
* @var string
*/
protected $description = 'Import csv data';
/**
* Execute the console command.
*/
public function handle()
{
$file = $this->argument('file');
$table = $this->option('table');
if (!file_exists($file)) {
$this->error('The specified file does not exist.');
return 1;
}
$data = array_map('str_getcsv', file($file));
$headers = array_shift($data);
DB::table($table)->insert($this->combineHeadersAndData($headers, $data));
$this->info($table . ' imported successfully.');
return 0;
}
/**
* Combine headers and data into associative arrays.
*
* @param array $headers
* @param array $data
* @return array
*/
private function combineHeadersAndData(array $headers, array $data)
{
$combinedData = [];
foreach ($data as $row) {
$combinedData[] = array_combine($headers, $row);
}
return $combinedData;
}
}
Run console command:
php artisan app:import-csv-data C:\laragon\z\razzi\members.csv --table=members
Output:
Check via Tinker commands:
php artisan tinker
App\Models\Member::count();
exit
Output:
Check via HeidiSQL (bundled in Laragon):
Download example:
https://archive.org/download/laravelprojects/razzi_20240331_laravel10_import_csv.zip
Subscribe to my newsletter
Read articles from Mohamad Mahmood directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
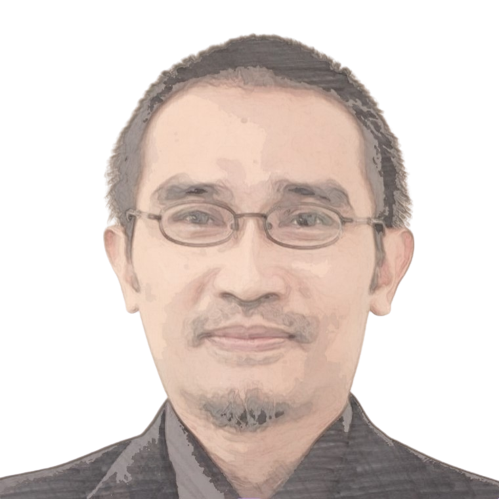
Mohamad Mahmood
Mohamad Mahmood
Mohamad's interest is in Programming (Mobile, Web, Database and Machine Learning). He studies at the Center For Artificial Intelligence Technology (CAIT), Universiti Kebangsaan Malaysia (UKM).