Laravel - Create a page to view records
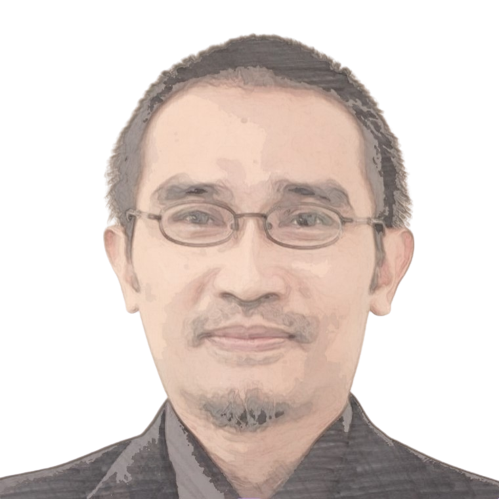
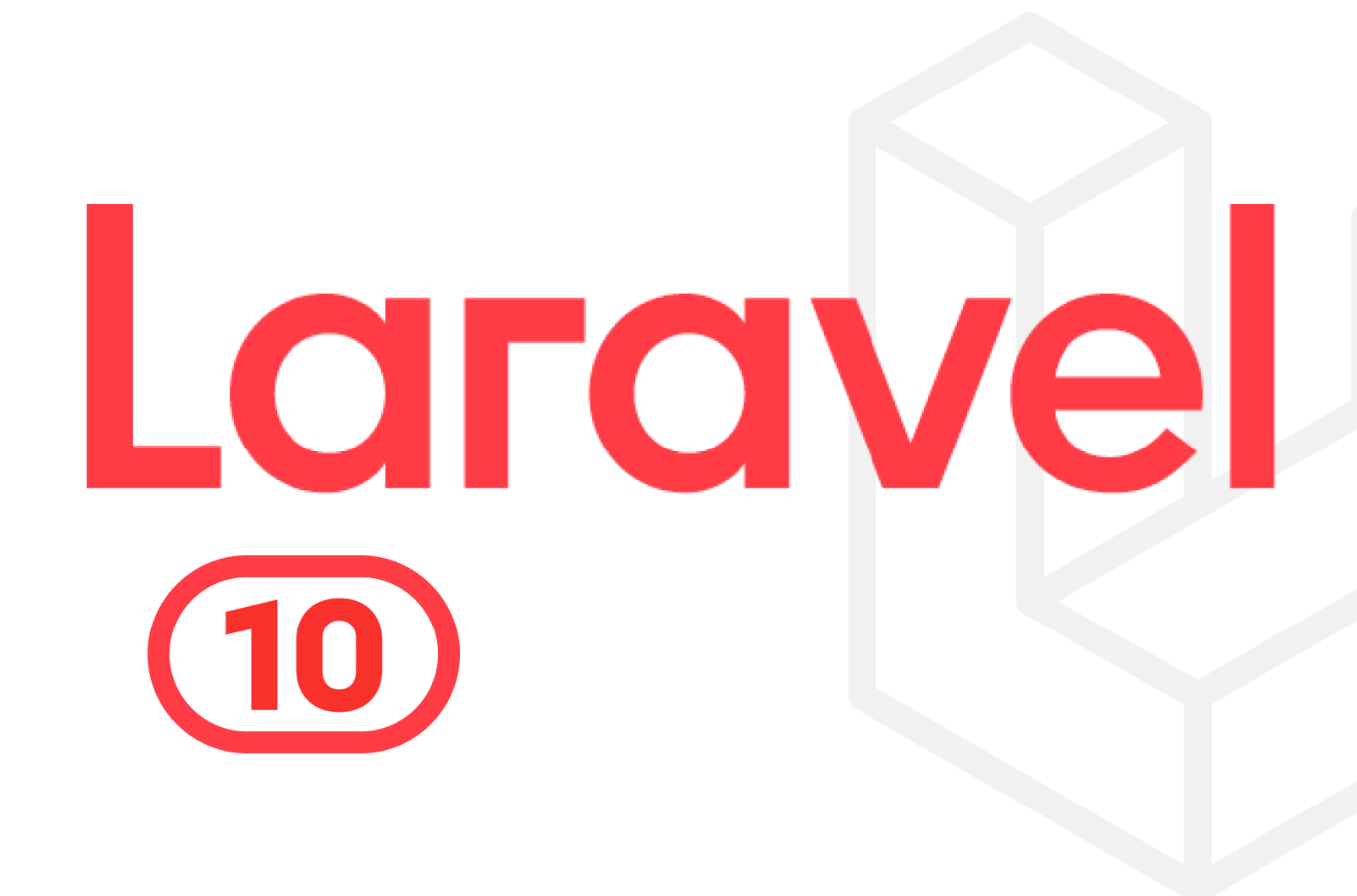
[0] Prep
Continue from previous article or download quickstart file.
[1] Add page to view records
Create a new route: Open your routes/web.php file and add a route that maps to a controller method or a closure.
use App\Http\Controllers\MemberController;
Route::get('/members', [MemberController::class, 'index'])->name('members.index');
Create a new controller: Run the following command to generate a new controller:
php artisan make:controller MemberController
This will create a new MemberController.php file in the app/Http/Controllers directory.
Define the index method: Open the MemberController.php file and define the index method. This method will handle the logic for retrieving all members and passing them to the view.
File C:\laragon\www\razzi\app\Http\Controllers\MemberController.php
:
<?php
namespace App\Http\Controllers;
use Illuminate\Http\Request;
use App\Models\Member;
class MemberController extends Controller
{
public function index()
{
$members = Member::all();
return view('members.index', compact('members'));
}
}
Create a view: Create a new file named index.blade.php in the resources/views/members directory. This file will contain the HTML markup for displaying the members.
File C:\laragon\www\razzi\resources\views\members\index.blade.php
:
<h1>Members</h1>
<table>
<thead>
<tr>
<th>Name</th>
<th>Email</th>
</tr>
</thead>
<tbody>
@foreach ($members as $member)
<tr>
<td>{{ $member->name }}</td>
<td>{{ $member->email }}</td>
</tr>
@endforeach
</tbody>
</table>
Browse:
[2] Integrate into Breeze dashboard
[2.1] Add the link into the layout file
<x-nav-link :href="route('members.index')" :active="request()->routeIs('dashboard')">
{{ __('Members') }}
</x-nav-link>
Outcome:
[2.2] Add the Breeze layout into the view file
File C:\laragon\www\razzi\resources\views\members\index.blade.php
:
<x-app-layout>
<x-slot name="header">
<h2 class="font-semibold text-xl text-gray-800 leading-tight">
{{ __('Members') }}
</h2>
</x-slot>
<div class="py-12">
<div class="max-w-7xl mx-auto sm:px-6 lg:px-8">
<div class="bg-white overflow-hidden shadow-sm sm:rounded-lg">
<div class="p-6 text-gray-900">
{{ __("You're logged in!") }}
<table>
<thead>
<tr>
<th>Name</th>
<th>Email</th>
</tr>
</thead>
<tbody>
@foreach ($members as $member)
<tr>
<td>{{ $member->name }}</td>
<td>{{ $member->email }}</td>
</tr>
@endforeach
</tbody>
</table>
</div>
</div>
</div>
</div>
</x-app-layout>
Outcome:
[3] Download example:
https://archive.org/download/laravelprojects/razzi_20240331_laravel10_view_records.zip
Subscribe to my newsletter
Read articles from Mohamad Mahmood directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
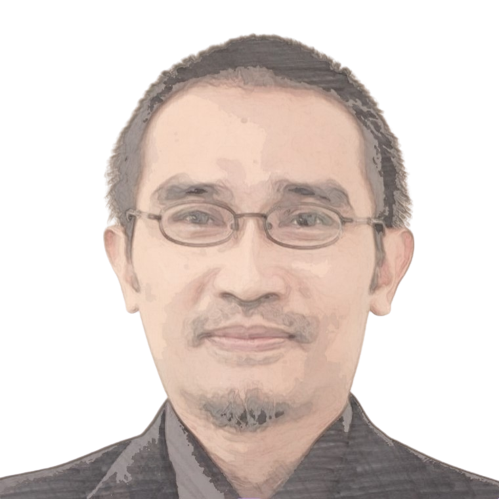
Mohamad Mahmood
Mohamad Mahmood
Mohamad's interest is in Programming (Mobile, Web, Database and Machine Learning). He studies at the Center For Artificial Intelligence Technology (CAIT), Universiti Kebangsaan Malaysia (UKM).