Understanding Python and Data Types and Structures in it

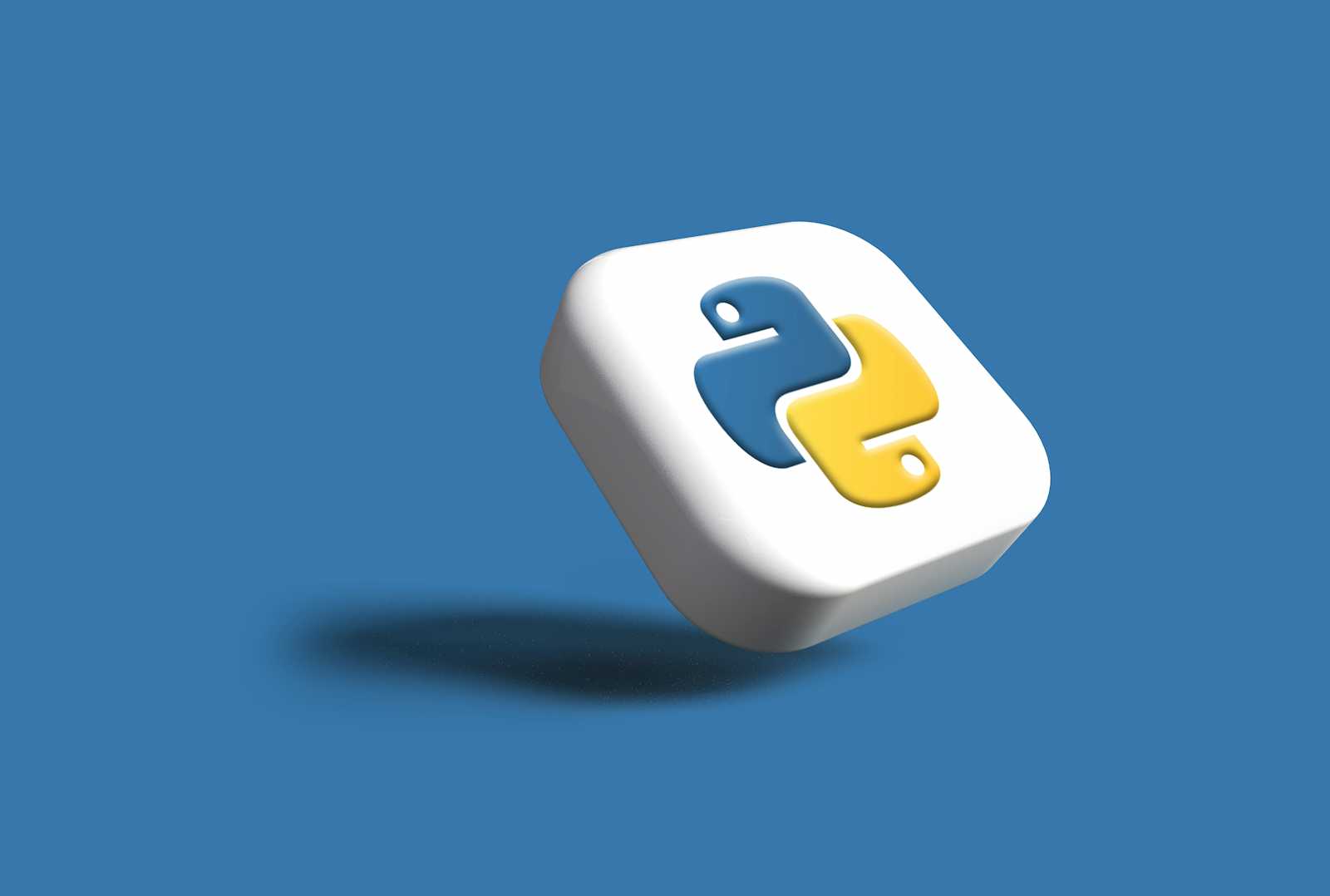
Python, the versatile and powerful programming language, is a go-to choice for developers worldwide. From web development to machine learning, its simplicity and readability make it a top contender in the tech space.
Did you know that Python was created by Guido van Rossum and boasts a rich ecosystem of libraries and frameworks like Django, TensorFlow, Flask, Pandas, and Keras?
Recently, I embarked on my Python journey by installing it on my system. Here are the installation steps I followed:
Windows Installation:
Visit the official Python website: python.org
Download the latest Python installer for Windows.
Run the installer and follow the on-screen instructions.
During installation, ensure to check the option to add Python to PATH.
Once installed, open Command Prompt and type
python --version
to verify the installation and check the Python version.
Ubuntu Installation:
Open Terminal.
Run the command: to update the package list.
sudo apt-get update
Then, install Python 3 by running:
sudo apt-get install python3
After installation, verify Python version by typing
python3 --version
in the Terminal.
Now that python is installed, let's delve into some fundamental concepts such as data types, data structures, and perform hands-on tasks to better understand Lists, Tuples, Sets, and Dictionaries in Python.
1. Data Types: Data types are classifications of data items that represent the kind of value and operations that can be performed on them. Python provides several built-in data types:
Numeric: Integers, Floats, Complex numbers
Sequential: Strings, Lists, Tuples
Boolean
Set
Dictionary
2. Data Structures: Data structures organize and store data in memory to enable efficient access and manipulation. Python offers various data structures:
Lists: Ordered collection, mutable
Tuple: Ordered collection, immutable
Dictionary: Key-value pairs, unordered
Set: Unordered collection of unique elements
Difference between List, Tuple, and Set:
Lists:
Ordered collection
Mutable (Elements can be added, removed, or modified)
Syntax:
my_list = [1, 2, 3, 4]
Tuple:
Ordered collection
Immutable (Cannot be modified after creation)
Syntax:
my_tuple = (1, 2, 3, 4)
Set:
Unordered collection of unique elements
Mutable (Elements can be added or removed)
Syntax:
my_set = {1, 2, 3, 4}
Basic Hands-on Tasks:
1. Create Dictionary:
Excited to dive into the world of Python development and explore its endless possibilities!
fav_tools = {
1: "Linux",
2: "Git",
3: "Docker",
4: "Kubernetes",
5: "Terraform",
6: "Ansible",
7: "Chef"
}
# Print favorite tool using key
print(fav_tools[2]) # Output: Git
2. Create List of Cloud Service Providers:
cloud_providers = ["AWS", "GCP", "Azure"]
# Add Digital Ocean and sort alphabetically
cloud_providers.append("Digital Ocean")
cloud_providers.sort()
print(cloud_providers) # Output: ['AWS', 'Azure', 'Digital Ocean', 'GCP']
In this post, we understood what is python, how it is installed and explored the fundamental concepts of Python data types and structures, including Lists, Tuples, Sets, and Dictionaries. We also performed basic hands-on tasks to solidify our understanding. Python's versatility in data handling makes it a powerful tool for various applications ranging from data analysis to web development.
Subscribe to my newsletter
Read articles from Rutuja Deodhar directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
