Translator API
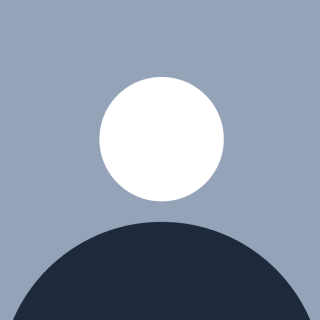

Today we will be adding a translator to my 1248 Learning application. The app is a spaced repetition flash card system. It can be used to study for exams or personal learning goals. One of those goals might be to learn a foreign language. This app is using the Google API for translating text.
First you will create an account with Google Cloud. Add a project. Then, enable Cloud Translation API. You will need to hold onto your API key and project code. I am also providing the API url that I used in this project.
const API_KEY = "YOUR_API_KEY"
const API_URL = `https://translation.googleapis.com/language/translate/v2`
Here are the imports needed in this component.
import React, {useState} from "react";
import { useFormik } from "formik";
import * as yup from "yup"
import Select from "react-select"
import axios from "axios";
const TranslateForm = ({sentence})=>{
...
}
export default TranslateForm
We will be using some state variables in this component. isTranslate is used to display the translated sentence.
const [isTranslate, setIsTranslate]=useState(false)
const [translated, setTranslated]=useState("")
First we will start off with the form needed. There are some language options available for the dropdown menu. We are using Select from "react-select" to help us with a dropdown menu in the formik form.
const TranslateForm = ({sentence}) => {
const languageOptions = [{value: "fr", label: "French"}, {value: "pl", label: "Polish"}, {value: "es", label: "Spanish"}]
const formSchema = yup.object().shape({
language: yup.string().required("A translating language is required.")
})
const formik = useFormik({
initialValues: {
language: ""
},
validationSchema: formSchema,
onSubmit: handleSubmit
})
function handleSubmit(values){
...
}
const defaultValue = (options, value) => {
return options ? options.find(option=>option.value===value):""
}
return (
<div>
<h3>Translate Front Sentence</h3>
<form onSubmit={formik.handleSubmit}>
<label htmlFor="language">Language</label>
<Select
name="language"
id="language"
value={defaultValue(languageOptions, formik.values.language)}
options={languageOptions}
onChange={value=>formik.setFieldValue('language', value.value)}
/>
{formik.errors.language ? <div className="errors">{formik.errors.language}</div> : null}
<button type="Submit">Translate</button>
{isTranslate ? <div>Translated sentence: {translated}</div> : null}
</form>
</div>
)
}
Next we will work on the handleSubmit function. This will use axios and the API information. Notice that we are setting our state variables here.
function handleSubmit(values){
setIsTranslate(true)
async function doTranslation(){
const {data}=await axios.post(API_URL, {}, {
params: {
q: sentence,
target: values.language,
key: API_KEY
}
})
setTranslated(data.data.translations[0].translatedText)
}
doTranslation()
}
The sentence in the props being past down to TranslateForm is from the CardForm and it is from the formik value front_sentence.
Final code all put together.
import React, {useState} from "react";
import { useFormik } from "formik";
import * as yup from "yup"
import Select from "react-select"
import axios from "axios";
const API_KEY = "YOUR_API_KEY"
const project_number = "learning-418920"
const TranslateForm = ({sentence}) => {
const [isTranslate, setIsTranslate]=useState(false)
const [translated, setTranslated]=useState("")
const languageOptions = [{value: "fr", label: "French"}, {value: "pl", label: "Polish"}, {value: "es", label: "Spanish"}]
const API_URL = `https://translation.googleapis.com/language/translate/v2`
const formSchema = yup.object().shape({
language: yup.string().required("A translating language is required.")
})
const formik = useFormik({
initialValues: {
language: ""
},
validationSchema: formSchema,
onSubmit: handleSubmit
})
function handleSubmit(values){
setIsTranslate(true)
async function doTranslation(){
const {data}=await axios.post(API_URL, {}, {
params: {
q: sentence,
target: values.language,
key: API_KEY
}
})
setTranslated(data.data.translations[0].translatedText)
}
doTranslation()
}
const defaultValue = (options, value) => {
return options ? options.find(option=>option.value===value):""
}
return (
<div>
<h3>Translate Front Sentence</h3>
<form onSubmit={formik.handleSubmit}>
<label htmlFor="language">Language</label>
<Select
name="language"
id="language"
value={defaultValue(languageOptions, formik.values.language)}
options={languageOptions}
onChange={value=>formik.setFieldValue('language', value.value)}
/>
{formik.errors.language ? <div className="errors">{formik.errors.language}</div> : null}
<button type="Submit">Translate</button>
{isTranslate ? <div>Translated sentence: {translated}</div> : null}
</form>
</div>
)
}
export default TranslateForm
Subscribe to my newsletter
Read articles from Nicole Listerfelt directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by