Dart Interfaces: Extending vs Implementing | Difference between Extends & Implements keyword
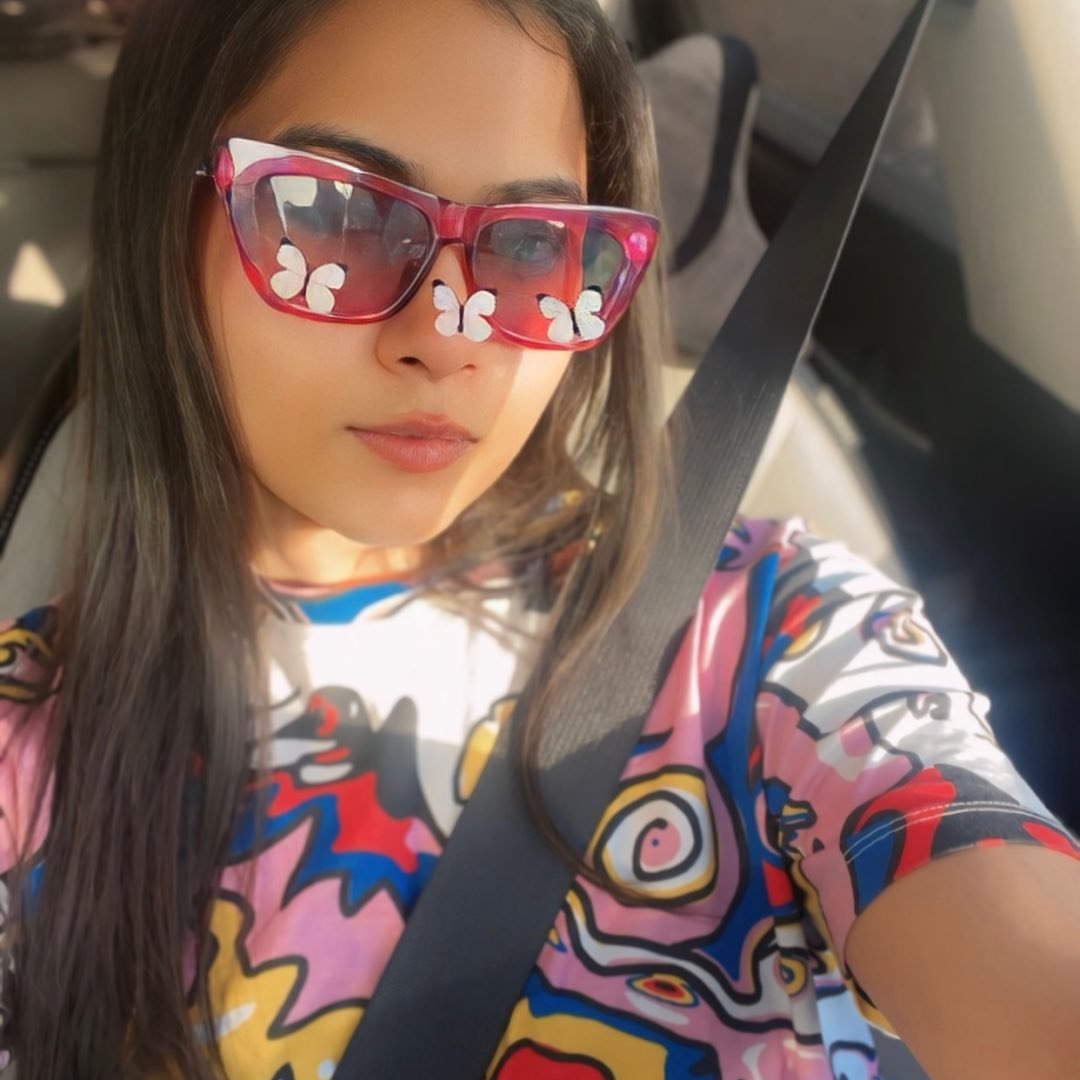
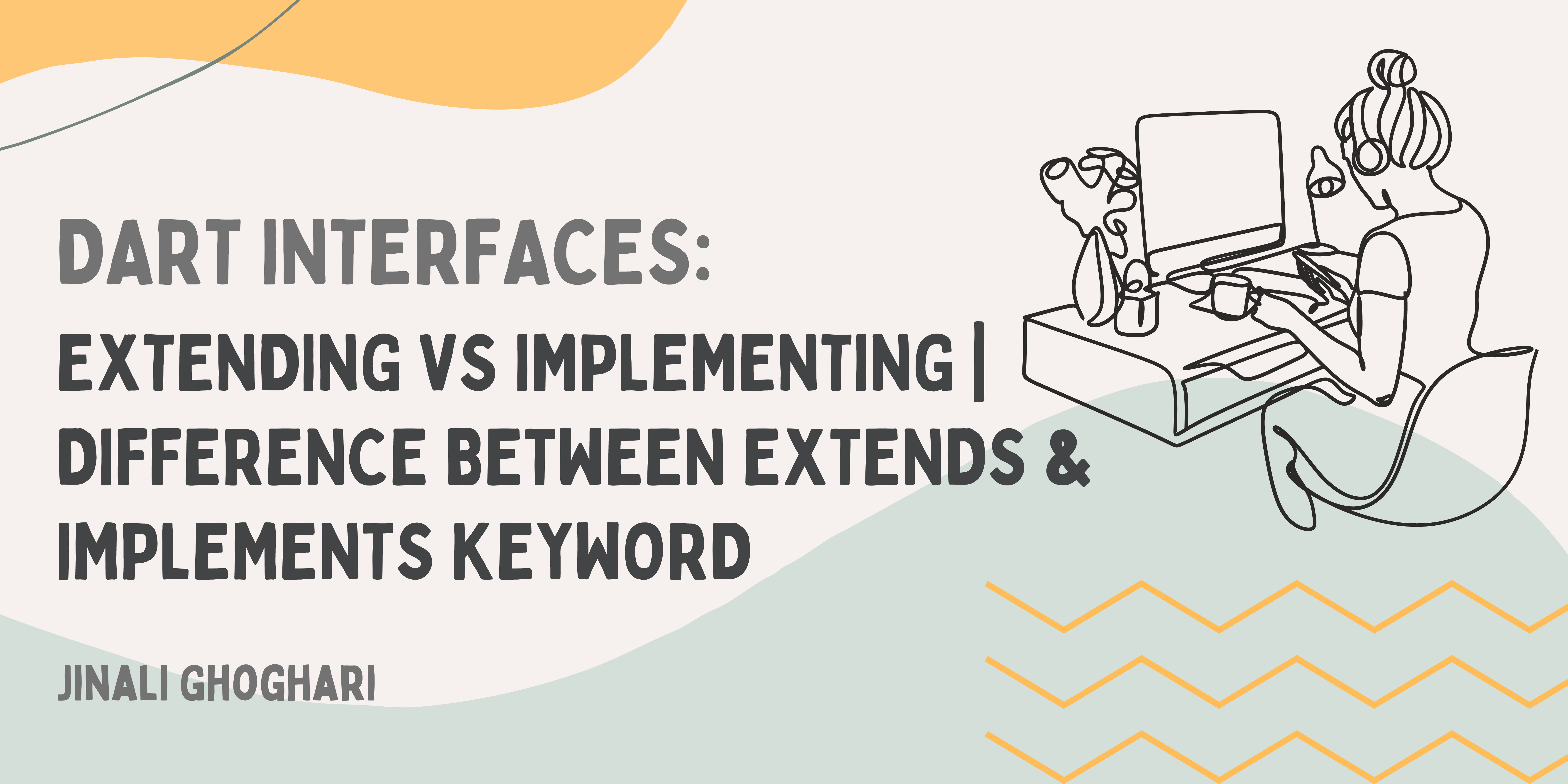
1. Extending (Extends keyword)
Extending is used to create a subclass that inherits the properties and methods of a superclass.
It establishes an "is-a" relationship between the subclass and the superclass. For example, if you have a class
Animal
and another classDog
that extendsAnimal
, you're saying thatDog
is a specific type ofAnimal
.The subclass can access all non-private members (fields and methods) of the superclass.
You use the
extends
keyword to indicate that a class inherits from another class.Example:
class Animal { void eat() { print('Animal is eating'); } } class Dog extends Animal { void bark() { print('Dog is barking'); } }
In this example,
Dog
extendsAnimal
, indicating that aDog
is a specific type ofAnimal
and inherits theeat()
method.
2. Implementing (implements keyword)
Implementing is used to declare that a class will provide specific behavior as defined by an interface.
It establishes a "can-do" relationship between the implementing class and the interface.
An interface in Dart is effectively a class with abstract methods and possibly no implementation.
A class can implement multiple interfaces.
You use the
implements
keyword to declare that a class implements one or more interfaces.Example:
abstract class Flyable { void fly(); } // Define a class named Bird which implements the Flyable interface class Bird implements Flyable { @override void fly() { print('Bird is flying'); } }
In this example, the
Bird
class implements theFlyable
interface, which means that it agrees to provide an implementation for thefly()
method defined in theFlyable
interface.- In summary, extending is used for inheritance, where a subclass inherits properties and methods from a superclass, while implementing is used to fulfill a contract defined by an interface, providing specific behavior as required by that interface.
Subscribe to my newsletter
Read articles from Jinali Ghoghari directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
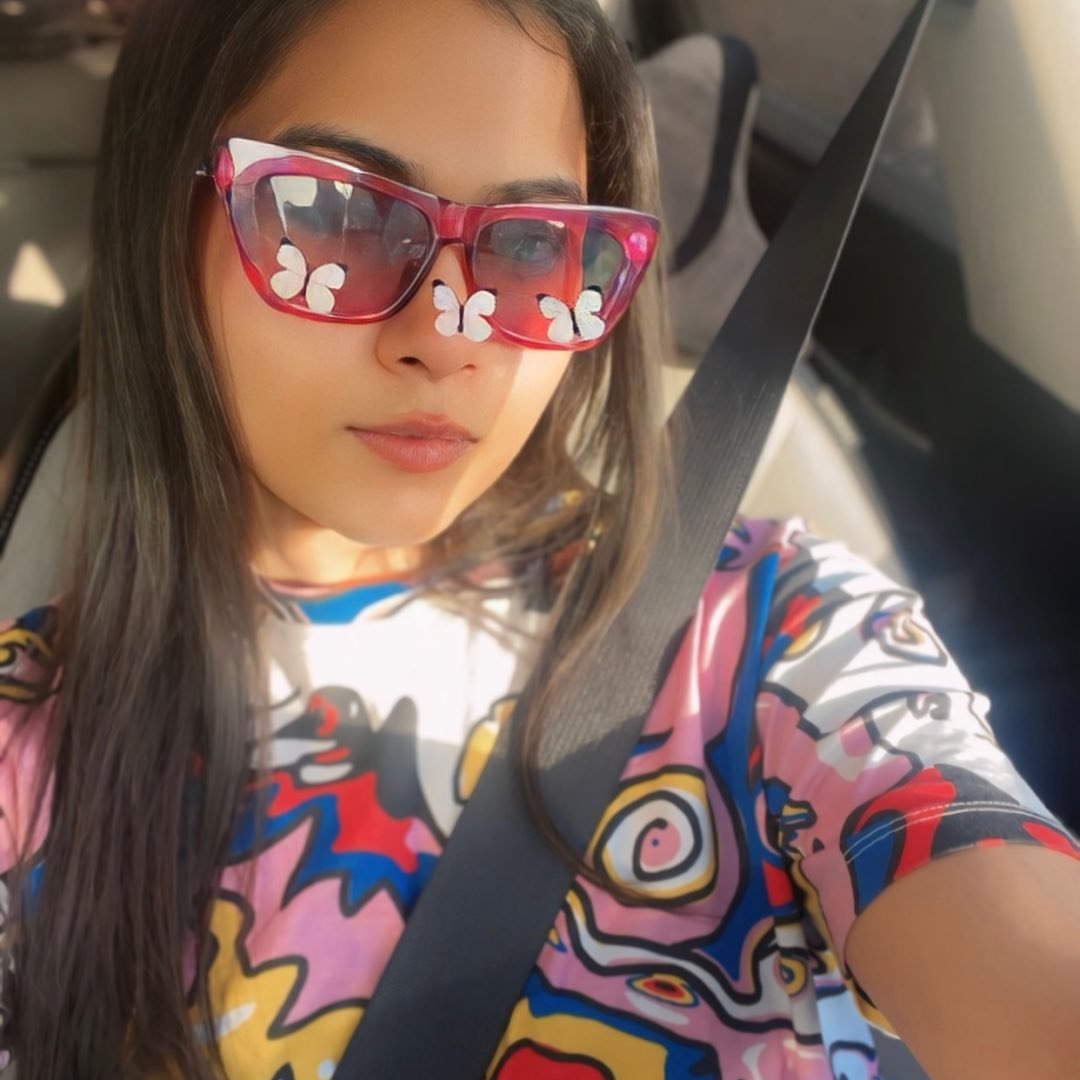