Understanding the Difference between State and Props in ReactJS

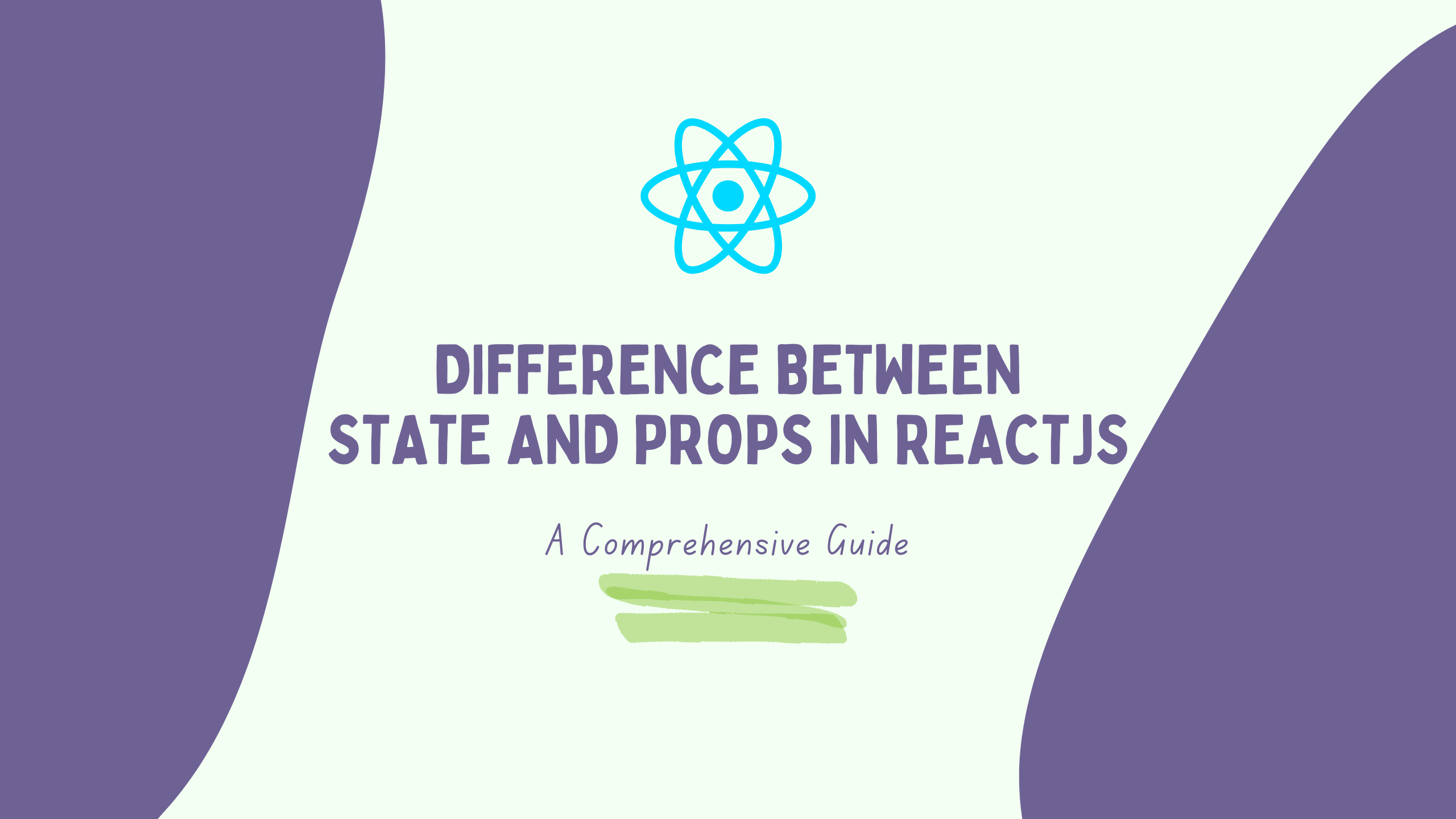
ReactJS is a powerful JavaScript library widely used for building user interfaces. One of its core concepts revolves around managing data through two fundamental mechanisms: state and props.
Understanding the difference between these two is crucial for developing React applications efficiently.
In this article, I'll dive into the difference between state and props, along with practical examples to illustrate their usage.
State in ReactJS:
State in React is a built-in object that represents the internal state of a component.
It holds data that influences the rendering and behavior of the component.
State is mutable, meaning it can be modified directly using the
setState()
method provided by React.
Example:
Consider a simple counter component:
import React, { useState } from 'react';
cosnt Counter = () => {
const [count, setCount] = useState(0);
const increment = () => {
setCount(count + 1);
};
return (
<div>
<p>Count: {count}</p>
<button onClick={increment}>Increment</button>
</div>
);
}
export default Counter;
In this example, the
useState
hook is used to declare a state variablecount
, initialized with a value of0
.The
increment
function updates the count state by callingsetCount
, which triggers a re-render of the component.
Props in ReactJS:
Props (short for properties) are inputs to React components.
They are immutable and are passed down from parent to child components.
Props allow components to be customizable and reusable by providing a way to pass data from the parent component to its children.
Example:
Consider a User
component that displays user information:
import React from 'react';
cosnt User = (props) => {
return (
<div>
<h2>Welcome, {props.name}</h2>
<p>Email: {props.email}</p>
</div>
);
}
export default User;
- Here, the
User
component receivesname
andemail
as props, which are then accessed usingprops.name
andprops.email
within the component.
Key Differences:
Mutability: State is mutable and can be modified using
setState()
, while props are immutable and cannot be changed within a component.Source: State is managed internally within a component, whereas props are passed from parent components.
Scope: State is local and specific to a component, while props are passed down through component hierarchies.
Usage: State is used for managing internal component state, such as user input, while props are used for passing data from parent to child components.
Conclusion:
Understanding the distinction between state and props is fundamental to building React applications efficiently. State is used for managing internal component data, while props facilitate communication between components by passing data from parent to child. By leveraging these mechanisms effectively, developers can create dynamic and reusable React components for building scalable applications. You can explore React Official Documentation.
Remember, while state and props serve different purposes, they often work together to create interactive and responsive user interfaces in ReactJS.
~Happy coding! ๐งโ๐ป
Hope you found this article helpful, don't forget to share & give feedback in the comment section! ๐
Subscribe to my newsletter
Read articles from Pranab K.S directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Pranab K.S
Pranab K.S
I'm a passionate web developer with a love for coding challenges and a knack for making well-informed decisions. Proficient in a variety of web technologies, including HTML, CSS, JavaScript, jQuery, Node.js, Express.js, MongoDB and React. I'm an avid open source enthusiast dedicated to both learning and contributing to the developer community.