Building a Meme Generator with React
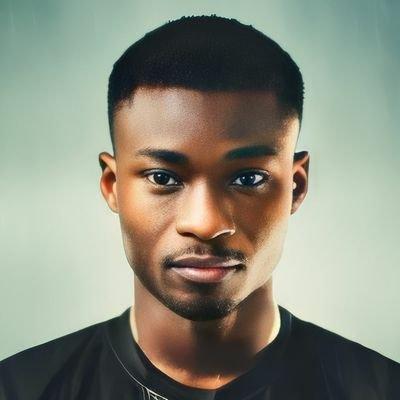
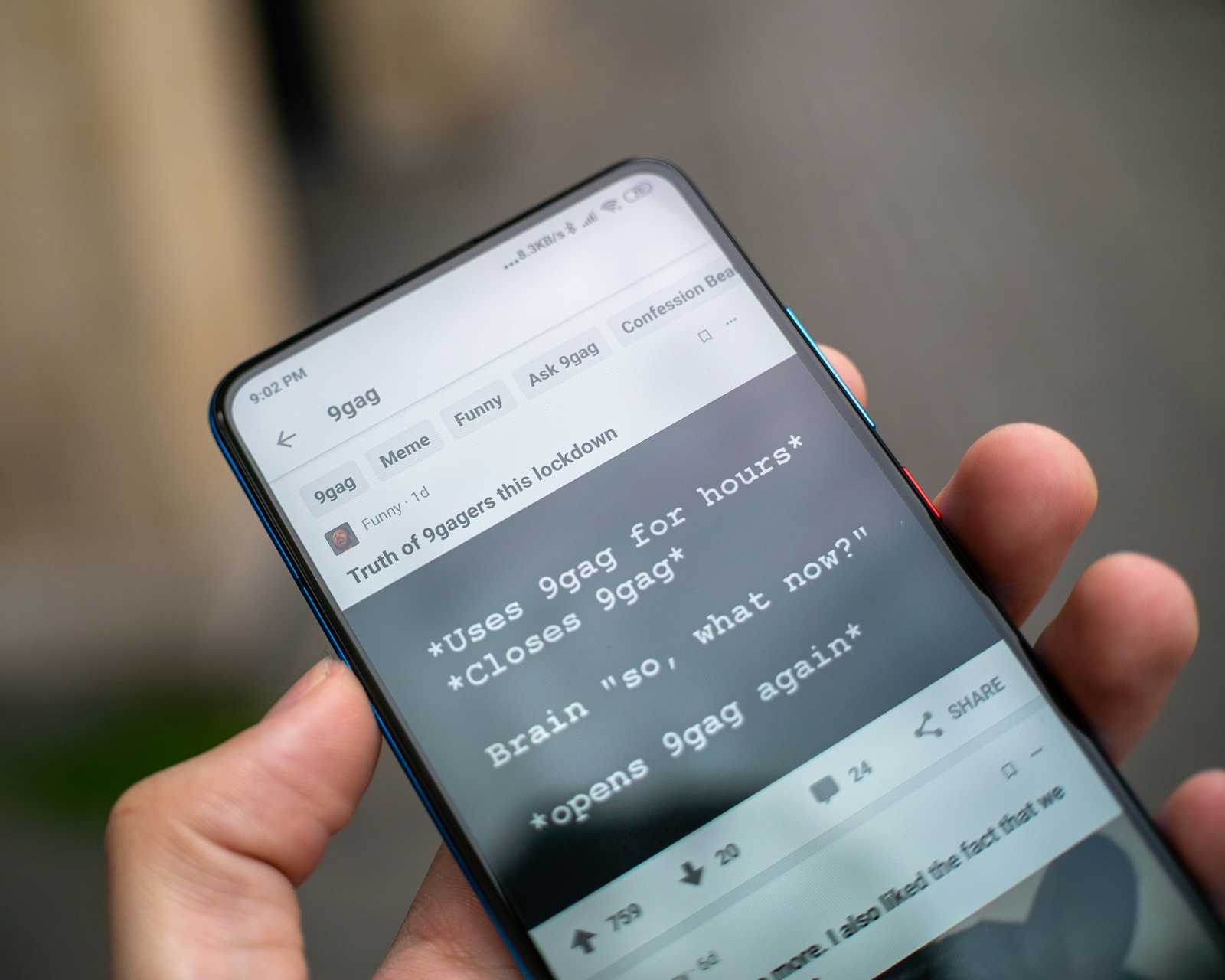
This week marks the culmination of my Web2Advance scholarship bootcamp with Web3Bridge, where I embarked on another journey to develop a meme generator using React. Let me take you through the highlights and the valuable lessons I learned along the way
Project Overview
In this project I built a meme generator from scratch. I began by splitting the app into components as seen in the code snippets below then further integrating the app to API and which would fetch memes at random for the app.
```jsx
// Meme.js Component
import React from "react"
import Header from "./components/Header"
export default function App() {
return (
<div>
<Header />
<Meme />
</div>
)
}
```
```jsx
// Meme.js Component
import React from "react"
export default function Meme() {
// Code for Meme Component
}
```
Exploring React Features
Throughout the project, I had the chance to explore several key aspects of React development:
1. Adding Events: I learned how to add event listeners to my app, enabling users to interact with the meme generator effortlessly.
```jsx
// Button for generating a new meme image
<button
className="form--button"
onClick={getMemeImage}
\>
Get a new meme image 🖼
</button>
```
2. Conditional Rendering: Implementing conditional rendering allowed me to dynamically display content based on user input, enhancing the overall user experience.
3. Utilizing Forms: From handling form inputs to updating state dynamically, I mastered the art of working with forms in React, making the meme generator interactive and user-friendly.
```jsx
// Input fields for top and bottom text
<input
type="text"
placeholder="Top text"
className="form--input"
name="topText"
value={meme.topText}
onChange={handleChange}
/>
<input
type="text"
placeholder="Bottom text"
className="form--input"
name="bottomText"
value={meme.bottomText}
onChange={handleChange}
/>
```
4. Harnessing useEffect: The useEffect hook became my best friend as I leveraged it to fetch meme data from an external API. This asynchronous operation seamlessly integrated new meme templates into the generator with each page load.
```jsx
// useEffect hook for fetching meme data
React.useEffect(() => {
async function getMemes() {
const res = await fetch("https://api.imgflip.com/get_memes")
const data = await res.json()
setAllMemes(data.data.memes)
}
getMemes()
}, [])
```
Lessons Learned
Building the meme generator was not only about coding; it was also about embracing the challenges and learning from them:
1. Problem-Solving Skills: I encountered various roadblocks along the way, but each obstacle presented an opportunity to sharpen my problem-solving skills and deepen my understanding of React.
2. Persistence Pays Off: There were moments of frustration, but I persevered, and the sense of accomplishment upon completing the project was unparalleled. It reminded me that persistence is key in mastering new skills.
Project Showcase
If you're curious to see the meme generator in action, I invite you to check out the live project. Feel free to explore and unleash your creativity!
Closing Thoughts
As I wrap up this incredible journey with Web3Bridge, I can't help but feel grateful for the knowledge gained and the friendships formed along the way. Building the meme generator was not just about coding; it was about pushing my limits, embracing challenges, and ultimately, realizing the power of perseverance and passion. A special shoutout to Scrimba for providing invaluable guidance and resources.
Thank you for joining me on this adventure, and stay tuned for more.
Until next time
Subscribe to my newsletter
Read articles from Jeremiah Samuel directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
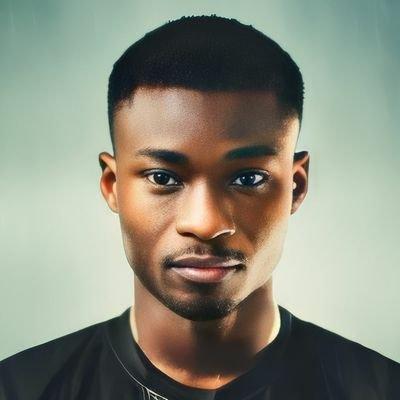