My Journey with JavaScript

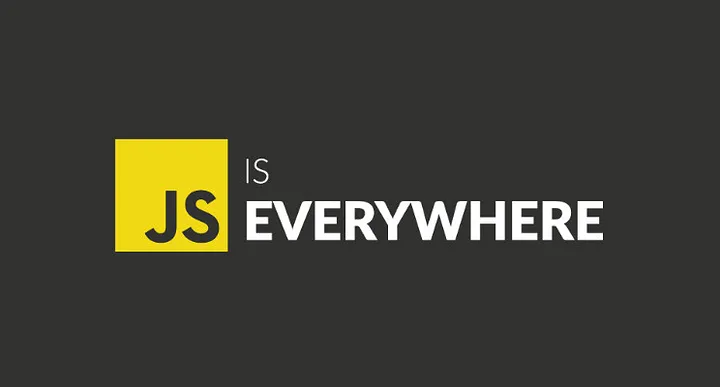
Hello everyone! I am thrilled to embark on this blogging journey and share my experiences with JavaScript. This marks the beginning of a series where I will walk you through my learning process and discoveries in the world of JavaScript.I'm learning JavaScript from the documentary, not from MDN or Ecma-262. I'm learning my javascript fromjavascript.info
.
Whyjavascript.info?
You might wonder why I chose javascript.info as my primary learning resource over other popular sources like MDN (Mozilla Developer Network) or the official ECMAScript documentation (Ecma-262). Well, I found javascript.info to be a comprehensive and beginner-friendly resource that offers clear explanations, practical examples, and interactive exercises. It has been a valuable companion in my learning journey, guiding me through the fundamentals and beyond.
So let us start with Javascript, it fun language to learn and to start your coding journey or web development journey.JavaScript, often abbreviated as JS, is a dynamic and versatile programming language that powers the web.
I started learning JS with Hello World I know it is basic but we start with basic right? So to get Hello World. we use alert("Hello World!") .
alert("Hello World!")
These are the topics I have learned so far:
"use strict"
Variables
Data types
Interaction
Type conversion
Comparisons
Conditional statements
Nullish Coalescing operator
Functions
"use strict"
The "use strict" directive is written on the top of the script, and then the whole script works in a modern way.
"use strict"; //on the top of the script
alert("hello world!")
it should be written only on the top otherwise it will not work. And the fun thing is you don't need to write it every time if you are using classes and modules (as modern js supports them it will enable "use strict" automatically.
Variables
Variables are named containers used to store data. In JavaScript, variables can be declared using var
, let
, and const
.
let: This is a modern way of declaring variables.
const: Similar to, but the value assigned to a variable declared
const
cannot be changed after declared.var: This was used before the introduction of
let
. However, it had issues with variable scope, which is whylet
andconst
were introduced.
Data Type
Primitive Data Types:
String: Represents a sequence of characters.
Number: Represents numeric values.
BigInt: Represents integers of arbitrary length.
Boolean: Represents true or false values.
Symbol: Represents unique identifiers.
Undefined: Represents a variable that has been declared but not assigned a value.
Null: Represents a deliberate absence of any value.
Non-Primitive Data Type:
- Object: Represents collections of key-value pairs.
Interaction
There are 3 browser-specific functions to interact with us:
alert: shows a message.
prompt: shows a message asking the user to input text, it contains ok with a text or cancel to send null as a value.
confirm: shows a message and asks you a question if you click ok that returns true and false for cancel.
Type conversion
These are the most widely used :
String(): Converts a value to a string.
Number(): Converts a value to a number.
let value = true; value = String(value); alert(typeof value);
Boolean(): Converts a value to a boolean (true or false).
We can use typeof for checking the data type of the variable.
Comparisons
Here the comparison operators are similar to maths. We use them to compare two numbers, variables, or strings. And we always get Boolean as the return value(true or false). We use them for conditional operators.
Greater/less than:
a > b
,a < b
.Greater/less than or equals:
a >= b
,a <= b
.Equals:
a == b
, please note the double equality sign==
means the equality test, while a single onea = b
means an assignment.Not equals: In maths the notation is
≠
, but in JavaScript, it’s written asa != b
.
alert( 2 > 1 ); // true (correct)
alert( 'Z' > 'A' ); // true
alert( '2' > 1 ); // true, string '2' becomes a number 2
alert( true == 1 ); // true
alert( false == 0 ); // true
Conditional statements
Conditional statements in JavaScript allow you to execute different blocks of code based on specified conditions.
Types of Conditional Statements in JavaScript:
if Statement: The
if
statement is the most basic type of conditional statement. It executes a block of code if the specified condition is true.if (condition) { // code to execute if condition is true }
if...else Statement: The
if...else
statement extends theif
statement by providing an alternative block of code to execute if the condition is false.if (condition) { // code to execute if condition is true } else { // code to execute if condition is false }
else if Statement: The
else if
statement allows you to check multiple conditions sequentially and execute different blocks of code based on which condition is true.if (condition1) { // code to execute if condition1 is true } else if (condition2) { // code to execute if condition2 is true } else { // code to execute if all conditions are false }
switch Statement: The
switch
statement provides a way to execute different blocks of code based on the value of an expression. It is particularly useful when you have multiple possible values to check.switch (expression) { case value1: // code to execute if expression equals value1 break; case value2: // code to execute if expression equals value2 break; default: // code to execute if expression doesn't match any case }
ternary Operator: It is a shorthand alternative to the
if...else
statement and allows you to evaluate a condition and return a value based on whether the condition is true or false.condition ? expressionIfTrue : expressionIfFalse
Nullish Coalescing operator
The Nullish Coalescing Operator (??
) is a logical operator in JavaScript that that are used to handle null or undefined values by returning the default value when the value is null
or undefined
.
let value1 = null;
let value2 = undefined;
let value3 = "Hello";
console.log(value1 ?? "Default Value"); // Output: "Default Value"
console.log(value2 ?? "Default Value"); // Output: "Default Value"
console.log(value3 ?? "Default Value"); // Output: "Hello"
Functions
Functions are like magic tools in JavaScript! You write them once and use them over and over again.
How to Write:
function functionName(parameters) {
// do something
return value; // optional
}
functionName(arguments); //calling function
Types:
Named Function:
function add(a, b) { return a + b; }
Anonymous Function:
let subtract = function(a, b) { return a - b; };
Arrow Function:
let multiply = (a, b) => a * b;
One of the best I like to use is the arrow function. It is use easy to use.
Well, there are a few things to know:
Parameter: When you are declaring a function what you write in parentheses
(...)
is called a parameter.Argument: Arguments are the same thing but instead of being passed to a function declaration it is passed at the time of calling a function.
return: Keyword used to return a value from a function.
As I continue my journey with JavaScript, I look forward to exploring its features, mastering its concepts, and building exciting projects. I invite you to join me on this adventure, learn alongside me, and share your thoughts, questions, and experiences in the comments below. Stay tuned for the next part of my JavaScript journey!
Subscribe to my newsletter
Read articles from Gaurav Suman directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
