Understanding the Basic Principles of Laravel's Architecture

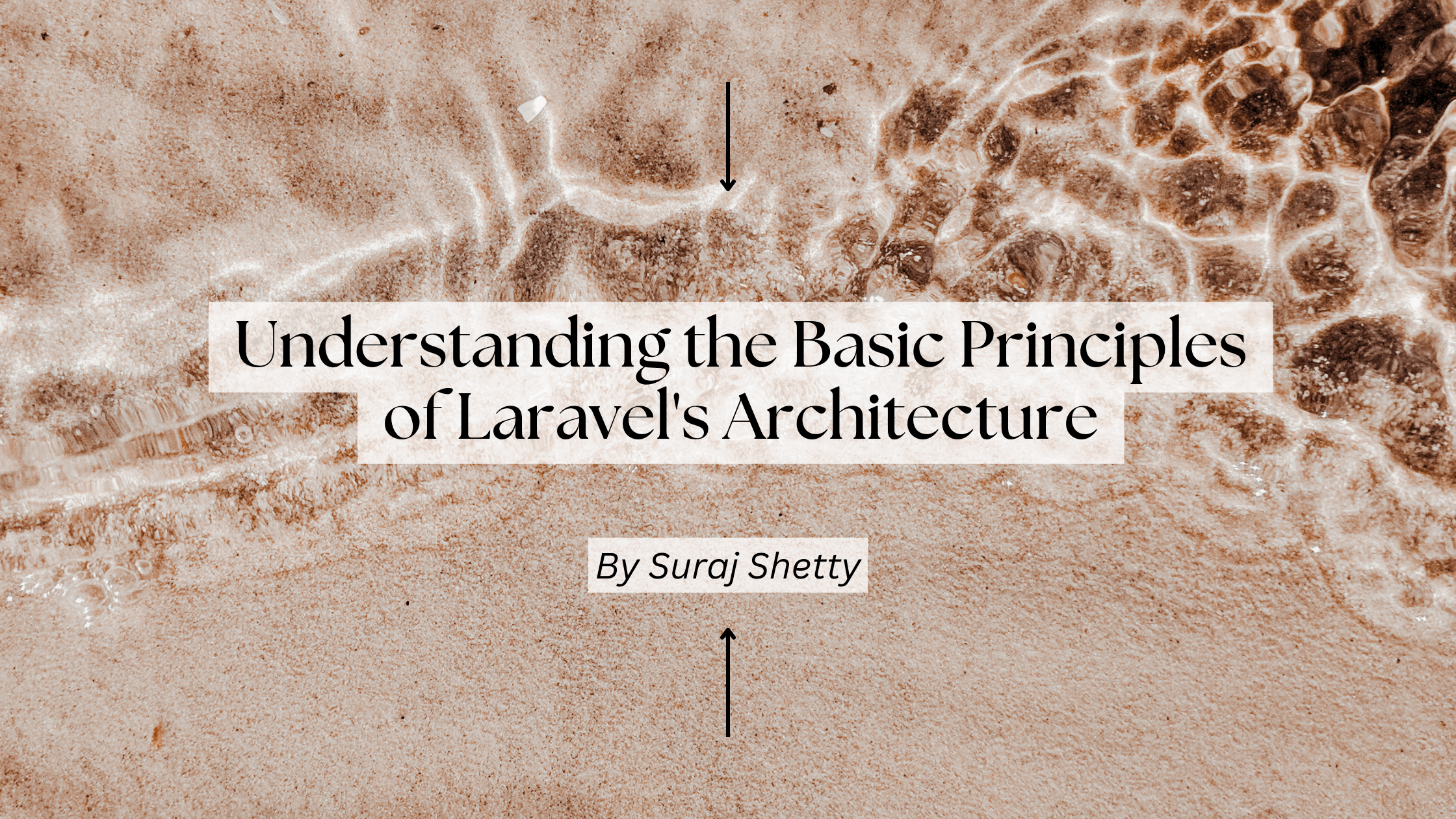
Prerequisites:
- PHP
- Basic of Laravel
Let's begin by exploring the architecture:
Model:
In Laravel, a model symbolizes your application's data structure and interacts with the database via Eloquent ORM, simplifying database operations with an ActiveRecord implementation, as demonstrated in creating a simple User model.
// app/Models/User.php
namespace App\Models;
use Illuminate\Database\Eloquent\Model;
class User extends Model
{
protected $fillable = ['name', 'email', 'password'];
}
View:
Views in Laravel, responsible for presenting data to users, are usually written in Blade, a powerful templating engine enabling clean and expressive PHP code in views.
<!-- resources/views/welcome.blade.php -->
<!DOCTYPE html>
<html>
<head>
<title>Welcome</title>
</head>
<body>
<h1>Welcome, {{ $user->name }}</h1>
</body>
</html>
Controller:
Controllers manage user requests, retrieve data from models, and transfer it to views for display, serving as a bridge between models and views; now, let's proceed to create a UserController.
// app/Http/Controllers/UserController.php
namespace App\Http\Controllers;
use App\Models\User;
use Illuminate\Http\Request;
class UserController extends Controller
{
public function show($id)
{
$user = User::findOrFail($id);
return view('welcome', compact('user'));
}
}
Routing:
Routing maps incoming HTTP requests to controller actions, and Laravel offers a clean, expressive method to define these routes in the routes/web.php
file.
// routes/web.php
use App\Http\Controllers\UserController;
Route::get('/user/{id}', [UserController::class, 'show']);
Middleware:
Middleware offers a handy way to filter HTTP requests coming into your application. It acts as a layer between the request and the core of your application, enabling you to carry out tasks like authentication, logging, and more. Laravel comes with several built-in middleware.
<?php
namespace App\Http\Middleware;
use Closure;
use Illuminate\Support\Facades\Log;
class LogHttpRequest
{
/**
* Handle an incoming request.
*
* @param \Illuminate\Http\Request $request
* @param \Closure $next
* @return mixed
*/
public function handle($request, Closure $next)
{
// Log the incoming request details
Log::info('Incoming request: ' . $request->method() . ' ' . $request->url());
// Proceed to the next middleware in the stack
return $next($request);
}
}
We've developed a middleware called LogHttpRequest
that intercepts incoming requests. The handle
method of this middleware takes the incoming request and closure, which is the next middleware in the stack. Within the handle
method, we use Laravel's Log
facade to record the details of the incoming request. After logging these details, we move forward to the next middleware in the stack by invoking $next($request)
. To make this middleware active, you must register it in the $middleware
property of your HTTP kernel located at app/Http/Kernel.php
.
protected $middleware = [
// Other middleware...
\App\Http\Middleware\LogHttpRequest::class,
];
Registering the middleware in the kernel ensures it runs for every incoming HTTP request, enabling logging of request details before they reach your application's core logic.
Let's delve deeper into the more advanced concepts of Laravel architecture:
Laravel Request life cycle:
Start
|
V
Incoming Request: The request is received by the server.
|
V
public/index.php: Laravel's front controller initializes the application.
|
V
app/Http/Kernel.php: Kernel Handles Request: The HTTP kernel processes the incoming request.
|
V
┌─────────────────────┐
│ Middleware │
│ │
│ Middleware Stack │
│ │
│ Route Dispatching │
│ │
│ Controller Method │
│ Execution │
└─────────────────────┘
|
V
Response Sent: The response is prepared and sent back to the client.
|
V
End: Request processing ends.
Incoming Request: The request is received by the server.
public/index.php: Laravel's front controller initializes the application. This file is located in the
public
folder and serves as the entry point for all requests.app/Http/Kernel.php: The HTTP kernel processes the incoming request. This file defines the middleware stack and handles the incoming request by dispatching it through the middleware layers.
Middleware: Middleware classes reside in the
app/Http/Middleware
directory. They intercept and process requests and responses as they pass through the middleware stack.Route Dispatching: Route dispatching is handled by the router, which maps incoming requests to controller methods based on the defined routes. Route definitions are typically located in the
routes
directory.Controller Method Execution: Controller methods are located in controller classes, usually stored in the
app/Http/Controllers
directory. These methods contain the main logic for handling the request and generating a response.Response Sent: Once the controller method has finished executing, the response is prepared and sent back to the client.
End: Request processing ends, and the server waits for the next incoming request.
This flowchart provides a structured overview of the Laravel request life cycle, incorporating the relevant folder structure and file names where each stage of request processing occurs.
Service Container & Service Provider:
Service Container:
Laravel's service container is a powerful tool for managing class dependencies and performing dependency injection. It automatically resolves dependencies and injects them into your classes, making your code more modular and easier to test.
Service Provider:
A Service Provider in Laravel is responsible for setting up and configuring services within the Laravel application. It serves as a link between the application and external services or components. Laravel's service providers play a crucial role in the framework's service container mechanism.
The Service Container and Service Provider are both fundamental components of Laravel's architecture, but they serve different purposes and operate at different levels within the framework. Let's break down the differences between them:
Aspect | Service Container | Service Provider |
Purpose | Manages class dependencies and performs dependency injection. | Bootstraps and configures services within the Laravel application. |
Responsibilities | - Resolving dependencies |
- Binding dependencies
- Singleton management | - Registering services
- Bootstrapping
- Binding aliases |
| Usage | Used throughout the Laravel framework to manage dependencies in controllers, middleware, service providers, and other components. | Typically used to integrate third-party libraries, configure database connections, define custom authentication drivers, and perform other setup tasks. |
In summary, both the Service Container and Service Provider are key parts of Laravel's architecture, but they have different roles. The Service Container handles class dependencies and carries out dependency injection. On the other hand, the Service Provider is in charge of starting up and setting up services in the application. Together, they help in building modular, easy-to-maintain, and expandable Laravel applications.
Laravel Facade:
A facade is a design pattern that offers a straightforward and static way to interact with complex systems or subsystems. In Laravel, facades give you an easy method to use services in the Service Container without the need to create or manually resolve them. They serve as static proxies to the classes registered in the Service Container.
Here's how Facades work in Laravel:
Facades in Laravel allow you to access services through static methods, acting as proxies to instances in the Service Container, correspond to registered services, use aliases for simplicity, resolve the underlying service upon method calls, and offer a clean, expressive syntax for easy interaction.
Here's an example of using the Auth
Facade to authenticate a user:
use Illuminate\Support\Facades\Auth;
// Attempt to authenticate the user
if (Auth::attempt(['email' => $email, 'password' => $password])) {
// Authentication successful
return redirect()->intended('dashboard');
} else {
// Authentication failed
return back()->withErrors(['email' => 'Invalid credentials']);
}
In this example, the Auth
Facade is used to access Laravel's authentication services, automatically linking to an instance of the Illuminate\Auth\AuthManager
class from the Service Container to handle the attempt()
method, showcasing how Facades offer a convenient and expressive approach to using Laravel's framework components.
Conclusion:
Now you understand MVC (Model View Controller), how to use routes and middleware, and primarily grasp the Laravel request lifecycle, service container, service provider, and Laravel facade. With a deep understanding of these concepts, you'll encounter no issues when working with Laravel projects. In upcoming projects, we will explore more examples related to Laravel architecture.
Thanks For Reading This Blog.
Subscribe to my newsletter
Read articles from Suraj Shetty directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Suraj Shetty
Suraj Shetty
Passionate backend developer with a strong foundation in designing and implementing scalable and efficient server-side solutions. Specialized in creating robust APIs and database management. Committed to staying ahead in technology trends, I have a keen interest in DevOps practices, aiming to bridge the gap between development and operations for seamless software delivery. Eager to contribute to innovative projects and collaborate with like-minded professionals in the tech community. Let's connect and explore the possibilities of creating impactful solutions together! #BackendDevelopment #DevOps #TechInnovation