Understanding SQL Injection: Risks, Prevention, and Best Practices
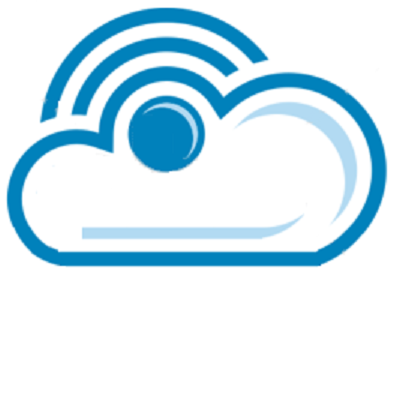
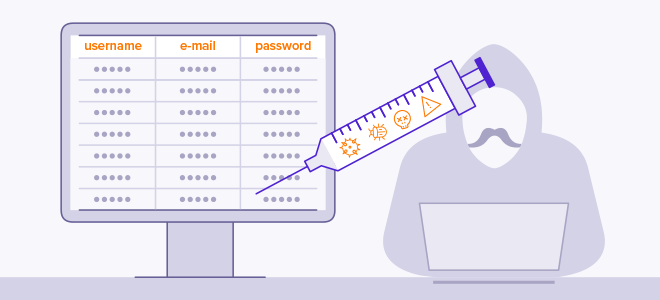
Understanding SQL Injection: Risks, Prevention, and Best Practices
In the realm of web application security, SQL injection (SQLi) remains one of the most prevalent and damaging vulnerabilities. Exploiting SQL injection vulnerabilities can lead to unauthorized access, data leakage, and potentially devastating consequences for organizations and users alike. In this article, we'll explore what SQL injection is, how it works, and best practices for prevention.
What is SQL Injection?
SQL injection is a type of security vulnerability that occurs when an attacker injects malicious SQL code into input fields of a web application, which is then executed by the underlying SQL database. This can happen when user input is not properly validated or sanitized before being used in SQL queries.
How Does SQL Injection Work?
The process of exploiting a SQL injection vulnerability typically involves the following steps:
Identifying Vulnerable Input: The attacker identifies input fields within a web application, such as login forms, search boxes, or URL parameters, that are susceptible to SQL injection.
Crafting Malicious Input: The attacker constructs malicious SQL queries or payloads, such as UNION SELECT statements or SQL comments, and submits them through the vulnerable input fields.
Executing the Attack: The malicious SQL code is concatenated with the legitimate SQL query executed by the application's database server, resulting in unintended behavior. This could include bypassing authentication, retrieving sensitive data, modifying database contents, or even executing system commands.
Exploiting the Results: The attacker leverages the results of the SQL injection attack to gain unauthorized access or perform further malicious actions within the application or database.
Risks of SQL Injection
SQL injection vulnerabilities pose significant risks to web applications and organizations, including:
Data Leakage: Attackers can extract sensitive information from databases, such as usernames, passwords, credit card numbers, and personal identifiable information (PII).
Data Manipulation: Attackers can modify or delete data stored in databases, leading to data loss, integrity breaches, and system downtime.
Unauthorized Access: Attackers can bypass authentication mechanisms and gain unauthorized access to restricted areas of the application or administrative interfaces.
Denial of Service (DoS): Attackers can execute resource-intensive SQL queries, leading to performance degradation, server overload, and denial of service for legitimate users.
Preventing SQL Injection
Preventing SQL injection requires a combination of secure coding practices, input validation, and parameterized queries. Some best practices for preventing SQL injection vulnerabilities include:
Input Validation: Validate and sanitize user input to ensure that it conforms to expected formats and does not contain malicious characters or SQL code.
Parameterized Queries: Use parameterized queries or prepared statements with bound parameters to separate SQL code from user input, preventing injection attacks.
Least Privilege Principle: Restrict database privileges and access rights to minimize the impact of successful SQL injection attacks.
Input Escaping: Escape special characters in user input before incorporating them into SQL queries, using libraries or frameworks that provide built-in escaping mechanisms.
Web Application Firewall (WAF): Implement a WAF to monitor and filter incoming HTTP traffic, detecting and blocking SQL injection attempts in real-time.
Security Testing: Conduct regular security assessments, code reviews, and penetration testing to identify and remediate SQL injection vulnerabilities proactively.
Examples
Example 1: SQL Injection in Login Form
Consider a login form where the username and password are checked against a database to authenticate users:
username = request.POST['username']
password = request.POST['password']
# SQL query to authenticate user
sql = "SELECT * FROM users WHERE username = '%s' AND password = '%s'" % (username, password)
# Execute SQL query and check if user exists
result = execute_sql_query(sql)
if result:
# User authenticated, grant access
login_user(username)
else:
# Invalid credentials, deny access
show_error_message("Invalid username or password")
In this example, if the attacker submits the following input as the password:
password: ' OR '1'='1
The resulting SQL query becomes:
SELECT * FROM users WHERE username = 'attacker' AND password = '' OR '1'='1'
This query always evaluates to true ('1'='1'), allowing the attacker to bypass authentication and log in as any user.
Example 2: SQL Injection in Search Query
Consider a search feature where users can search for products based on keywords:
search_query = request.GET['query']
# SQL query to search for products
sql = "SELECT * FROM products WHERE name LIKE '%{}%'".format(search_query)
# Execute SQL query and fetch results
results = execute_sql_query(sql)
If the attacker submits the following input as the search query:
query: ' OR 1=1 --
The resulting SQL query becomes:
SELECT * FROM products WHERE name LIKE '%' OR 1=1 -- %'
This query will return all products in the database, as the condition 1=1 always evaluates to true, effectively bypassing the search filter.
Example 3: SQL Injection in URL Parameter
Consider a URL parameter used to filter data in an API endpoint:
category_id = request.GET['category_id']
# SQL query to retrieve products based on category
sql = "SELECT * FROM products WHERE category_id = {}".format(category_id)
# Execute SQL query and fetch results
results = execute_sql_query(sql)
If the attacker modifies the URL parameter to the following:
/category?category_id=1%20OR%201=1
The resulting SQL query becomes:
SELECT * FROM products WHERE category_id = 1 OR 1=1
This query will return all products in the database, as the condition 1=1 always evaluates to true, effectively bypassing the category filter.
These examples are only a small show case of how SQL injection vulnerabilities can be exploited to manipulate SQL queries and gain unauthorized access to sensitive data or perform unauthorized actions within a web application. It's essential for developers to implement secure coding practices, such as parameterized queries and input validation, to mitigate the risk of SQL injection attacks and protect their applications against exploitation.
Conclusion
SQL injection remains a pervasive and dangerous vulnerability that can have severe consequences for web applications and organizations. By understanding the risks associated with SQL injection and implementing best practices for prevention, developers and organizations can mitigate the likelihood of exploitation and safeguard their applications against malicious attacks. Effective measures such as input validation, parameterized queries, and security testing are essential components of a comprehensive security strategy to defend against SQL injection and other common web application vulnerabilities.
Subscribe to my newsletter
Read articles from Cloud Tuned directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
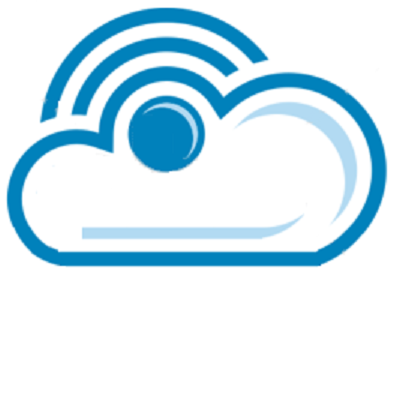