How to Implement Data Transfer Pattern in Laravel

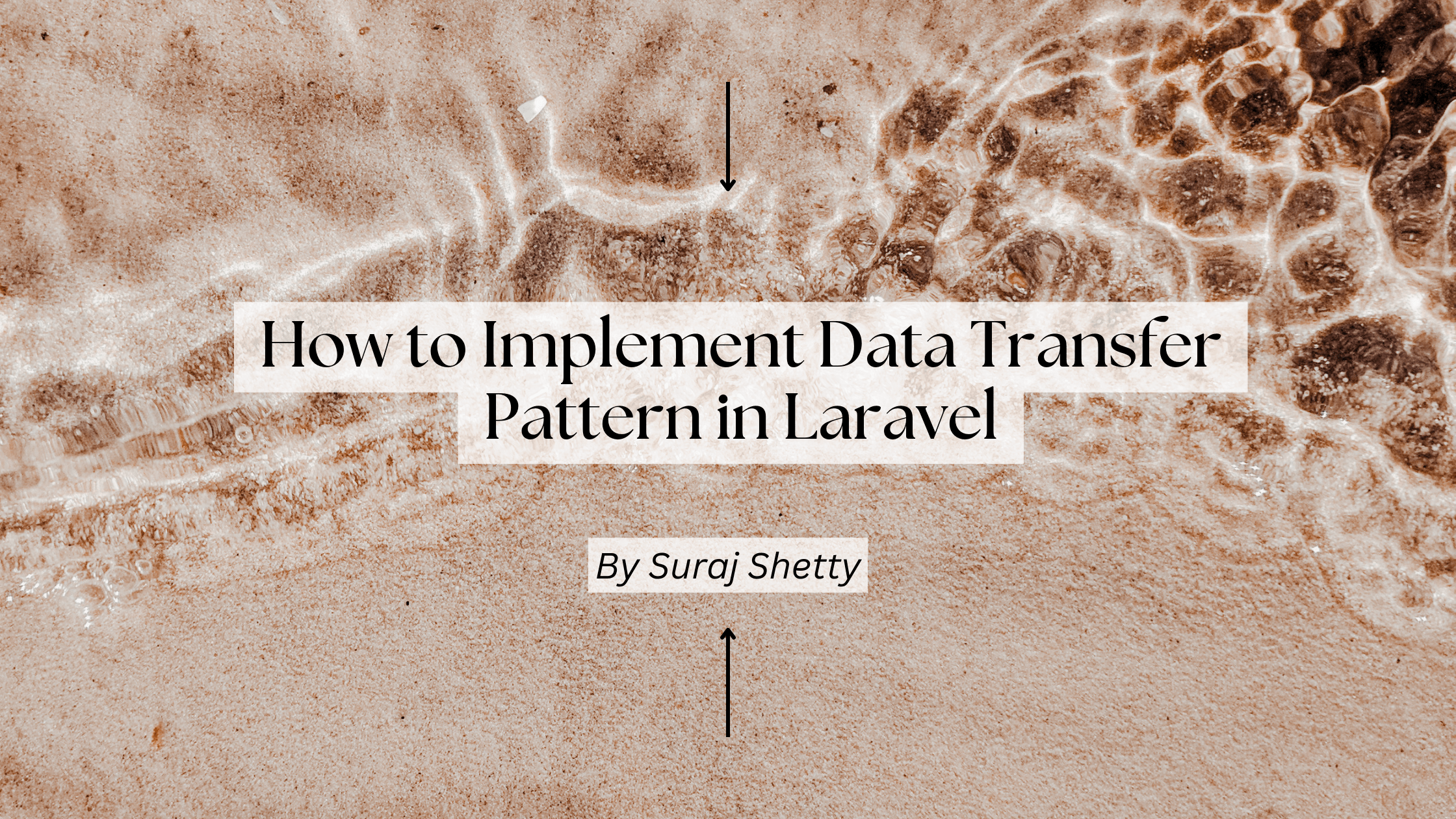
Prerequisites:
PHP
Basic of Laravel
Let's begin by exploring the Data Transfer Pattern in Laravel:
To illustrate, I'll use a CRUD application as a test case while implementing BO (Business Object), DAO (Data Access Object), and DTO (Data Transfer Object) patterns.
first, let's define what each of these patterns represents:
Business Object (BO): This represents the business logic of your application. It contains the methods and functions that perform operations on your data.
Data Access Object (DAO): This pattern is responsible for handling the interactions with the database. It abstracts away the database operations from the business logic.
Data Transfer Object (DTO): DTOs are used to transfer data between different layers of your application. They help in decoupling the layers and make your code more maintainable.
Now, let's create a simple CRUD application to manage "Products".
Create Laravel Project:
laravel new crud-app cd crud-app
Generate Model, Controller, and Migration:
php artisan make:model Product -m php artisan make:controller ProductController
Create Migration:
public function up() { Schema::create('products', function (Blueprint $table) { $table->id(); $table->string('name'); $table->text('description'); $table->decimal('price', 8, 2); $table->timestamps(); }); }
Run migration:
php artisan migrate
Create BO, DAO, and DTO:
Note: Create folders for BO, DAO, and DTO inside the
app
directory or according to your folder structure or design pattern, such as creating a BO folder in theService
folder.mkdir app/BO mkdir app/DAO mkdir app/DTO
Now, create the files ProductBO.php, ProductDAO.php, and ProductDTO.php inside their respective folders.
Implement BO (ProductBO.php):
namespace App\BO; use App\DAO\ProductDAO; use App\DTO\ProductDTO; class ProductBO { protected $productDAO; public function __construct(ProductDAO $productDAO) { $this->productDAO = $productDAO; } public function getAllProducts() { return $this->productDAO->getAll(); } // Implement other business logic methods like add, edit, delete. etc.. }
Implement the DAO (ProductDAO.php):
namespace App\DAO; use App\Models\Product; class ProductDAO { public function getAll() { return Product::all(); } // Implement other database operations like fetching, manipulation }
Implement DTO (ProductDTO.php):
namespace App\DTO; class ProductDTO { public $id; public $name; public $description; public $price; public function __construct($id, $name, $description, $price) { $this->id = $id; $this->name = $name; $this->description = $description; $this->price = $price; } }
Implement Controller (ProductController.php):
namespace App\Http\Controllers; use App\BO\ProductBO; use App\DTO\ProductDTO; class ProductController extends Controller { protected $productBO; public function __construct(ProductBO $productBO) { $this->productBO = $productBO; } public function index() { $products = $this->productBO->getAllProducts(); return response()->json($products); } // Implement other CRUD methods like add, update, delete. etc .. }
Conclusion:
Now you have a good understanding of data transfer patterns. This is a basic setup for implementing CRUD operations in Laravel using BO, DAO, and DTO patterns. You can expand this by adding methods for creating, updating, and deleting operations, as well as form validation, error handling, and more advanced features as needed. In upcoming blogs, we will cover the advanced implementation of data transfer patterns in Laravel.
Thanks For Reading This Blog.
Subscribe to my newsletter
Read articles from Suraj Shetty directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Suraj Shetty
Suraj Shetty
Passionate backend developer with a strong foundation in designing and implementing scalable and efficient server-side solutions. Specialized in creating robust APIs and database management. Committed to staying ahead in technology trends, I have a keen interest in DevOps practices, aiming to bridge the gap between development and operations for seamless software delivery. Eager to contribute to innovative projects and collaborate with like-minded professionals in the tech community. Let's connect and explore the possibilities of creating impactful solutions together! #BackendDevelopment #DevOps #TechInnovation