All about Laravel PHP code style fixer - Laravel Pint
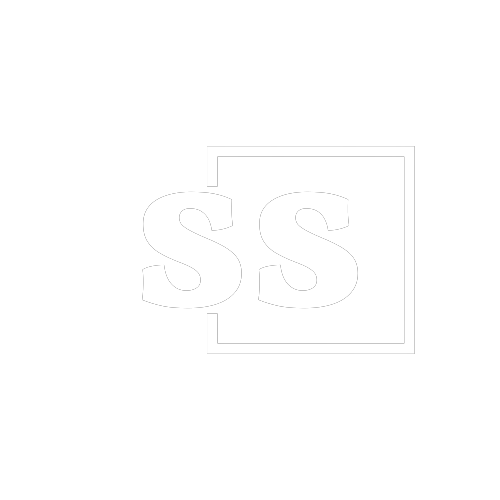
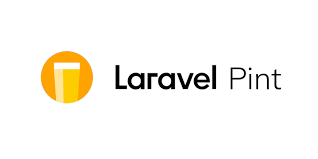
Maintaining code consistency and cleanliness is a common challenge for many developers. When working within a team, it becomes essential to uphold code consistency as a mandatory practice.
For Laravel, we have a package called Pint.
Pint is a Laravel package that comes pre-installed with Laravel 9 and later versions, aiding in PHP code style correction for Laravel through a straightforward command.
Pint is built on top of PHP-CS-Fixer which means you can add you own rules for your project inside the pint.json
file.
Prior to beginning, I'll be utilizing Laravel 11, assuming you have the same setup with all necessary dependencies installed and your project set up. If not, ensure your PHP version exceeds 8.2 (check with php -V
), Composer is installed (composer --version
), and all required PHP extensions are present.
Let's dive in.
Installation
Pint is included in recent releases of the Laravel framework, so installation is typically unnecessary. However, for older applications, you may install Laravel Pint via Composer:
composer require laravel/pint --dev
Running Pint
To run the pint, It provides pint binary inside the vendor/pint directory:
./vendor/bin/pint
You may also run Pint on specific files or directories using:
./vendor/bin/pint app/
./vendor/bin/pint app/Models/Post.php
Pint offers several options for usage:
-v
: Provides detailed information about Pint's changes.--test
: Allows testing of code styles without making actual alterations.--dirty
: Applies Pint only to uncommitted (git) changes.
To utilize these options, simply append them to the command accordingly.
./vendor/bin/pint -v
./vendor/bin/pint --test
./vendor/bin/pint --dirty
Configuration
While not required, you have the option to customize Pint's configuration. To do this, create a pint.json
file in your project's root directory. Inside this file, you can specify different presets, which represent sets of rules for your code. By default, Pint utilizes the Laravel preset.
{
"preset": "laravel"
}
You have the flexibility to switch to another preset, such as psr12, by simply replacing laravel
with psr12
in the pint.json
file.
{
"preset": "psr12"
}
Rules
Now, while you can configure the preset with default rules, you have the option to modify them by adding specific rules alongside the preset inside the JSON file.
{
"preset": "psr12",
"rules": {
"no_empty_comment": true,
"concat_space": {
"spacing": "one"
},
"array_push": true,
}
}
As demonstrated above, additional rules have been included alongside the preset. You can further customize these rules by visiting the PHP-CS-fixer-configurator and adding your own as needed.
It's important to note that if you're implementing Pint for an older project, it's advisable to carefully review the CS-Fixer documentation and understand its potential impact on your existing codebase.
Other configuration
You have the option to exclude specific folders or files from Pint's operation by specifying them to be ignored during the execution.
{
"exclude": [
"my-specific/folder"
]
}
You can ignore the file pattern inside the project:
{
"notName": [
"*-my-file.php"
]
}
Or you can use the exact path as well:
{
"notPath": [
"path/to/excluded-file.php"
]
}
These represent the key configurations and rules offered by Laravel Pint. For comprehensive information, refer to the official documentation.
These are the major configuration and rules provided by the Laravel pint. For more details visit official documentation.
Example configuration/rules
Pint configuration with psr12 and custom rules.
{
"preset": "psr12",
"rules": {
"align_multiline_comment": true,
"array_indentation": true,
"array_syntax": true,
"blank_line_after_namespace": true,
"blank_line_after_opening_tag": true,
"combine_consecutive_issets": true,
"combine_consecutive_unsets": true,
"concat_space": {
"spacing": "one"
},
"declare_parentheses": true,
"declare_strict_types": true,
"explicit_string_variable": true,
"final_class": false,
"fully_qualified_strict_types": true,
"global_namespace_import": {
"import_classes": true,
"import_constants": true,
"import_functions": true
},
"is_null": true,
"lambda_not_used_import": true,
"logical_operators": true,
"mb_str_functions": false,
"method_chaining_indentation": true,
"modernize_strpos": true,
"new_with_braces": true,
"no_empty_comment": true,
"not_operator_with_space": true,
"ordered_traits": false,
"protected_to_private": false,
"simplified_if_return": true,
"strict_comparison": false,
"ternary_to_null_coalescing": true,
"trim_array_spaces": true,
"use_arrow_functions": false,
"void_return": true,
"yoda_style": false,
"array_push": true,
"assign_null_coalescing_to_coalesce_equal": true,
"explicit_indirect_variable": false,
"modernize_types_casting": true,
"no_superfluous_elseif": true,
"no_useless_else": true,
"nullable_type_declaration_for_default_null_value": true,
"ordered_imports": {
"sort_algorithm": "alpha"
},
"ordered_class_elements": {
"order": [
"use_trait",
"case",
"constant",
"constant_public",
"constant_protected",
"constant_private",
"property_public",
"property_protected",
"property_private",
"construct",
"destruct",
"magic",
"phpunit",
"method_abstract",
"method_public_static",
"method_public",
"method_protected_static",
"method_protected",
"method_private_static",
"method_private"
],
"sort_algorithm": "none"
}
}
}
Conclusion
In conclusion, Laravel Pint emerges as a vital asset for developers seeking to uphold code consistency and cleanliness within their Laravel projects. With its integration into the Laravel framework and seamless compatibility with PHP-CS-Fixer, Pint offers a straightforward yet powerful solution for enforcing code style standards. Through simple commands and customizable configurations, developers can effortlessly apply code styling corrections, ensuring readability and maintainability across their codebase. By leveraging Pint, teams can enhance collaboration, streamline development processes, and elevate the overall quality of their Laravel applications, ultimately driving greater efficiency and success in their projects.
Happy Coding !!
Subscribe to my newsletter
Read articles from ssbhattarai directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
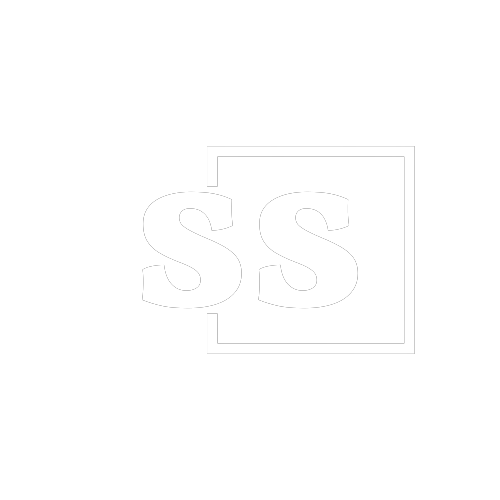