SendGrid with Ruby on Rails and NameCheap, step-by-step
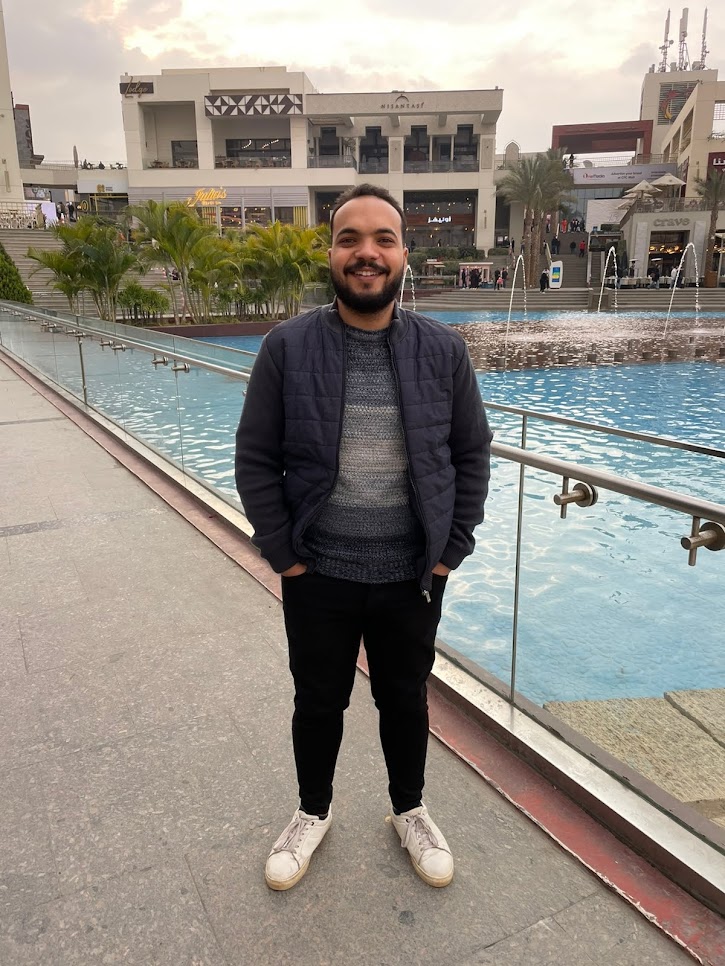
SendGrid is a robust email delivery service businesses and developers use to streamline email communication processes. It simplifies the task of sending transactional and marketing emails at scale. With its intuitive API and easy-to-use interface, we can seamlessly integrate SendGrid into applications, websites, or marketing automation platforms.
In this article, we will explore how to integrate SendGrid with Ruby on Rails to facilitate seamless email delivery, leveraging its robust features to enhance communication with users.
Getting Started with SendGrid:
After creating an account, obtaining an API key, and connecting the domain to SendGrid, Let's start with integrating with our rails application:
add
sendgrid-ruby
gem inGemfile
and runbundle install
source 'https://rubygems.org' git_source(:github) { |repo| "https://github.com/#{repo}.git" } # Bundle edge Rails instead: gem 'rails', github: 'rails/rails', branch: 'main' gem 'rails', '~> 6.1.3' # Use postgresql as the database for Active Record gem 'pg', '~> 1.1' gem 'sendgrid-ruby'
add SendGrid environment variables
SENDGRID_KEY=Blablabla SENDGRID_DOMAIN=yourdomain.com SENDGRID_ADDRESS=smtp.sendgrid.net SENDGRID_PORT=587
configure
ActionMailer
settingsconfig.action_mailer.delivery_method = :smtp ActionMailer::Base.smtp_settings = { :user_name => "apikey", # DO NOT CHANGE :password => ENV["SENDGRID_KEY"] :domain => ENV["SENDGRID_DOMAIN"], :address => ENV["SENDGRID_ADDRESS"], :port => ENV["SENDGRID_PORT"], :authentication => :plain, :enable_starttls_auto => true }
create new mailer
rails g mailer UserMailer
add
welcome_email
to our newly created userclass UserMailer < ApplicationMailer def welcome_email(user_id) @user = User.find_by(id: user_id) mail(to: @user.email, subject: "Welcome To Our Application") end end
create
User
model and controller ( I'll scaffold it)rails g scaffold User email name
call
UserMailer#welocme_email
after creating a new userclass UsersController < ApplicationController def create @user = User.new(user_params) if @user.save UserMailer.welcome_email(@user.id).perform_later render json: @user, status: :created, location: @user else render json: @user.errors, status: :unprocessable_entity end end end
create a view that outputs HTML
<!--/app/views/user_mailer/welcome_email.html.erb--> <h2> Welcome <%= @user.name %></h2> <p> Thanks for applying to our application </p>
And Voila!
Subscribe to my newsletter
Read articles from Mohamed Abd El-Meged directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
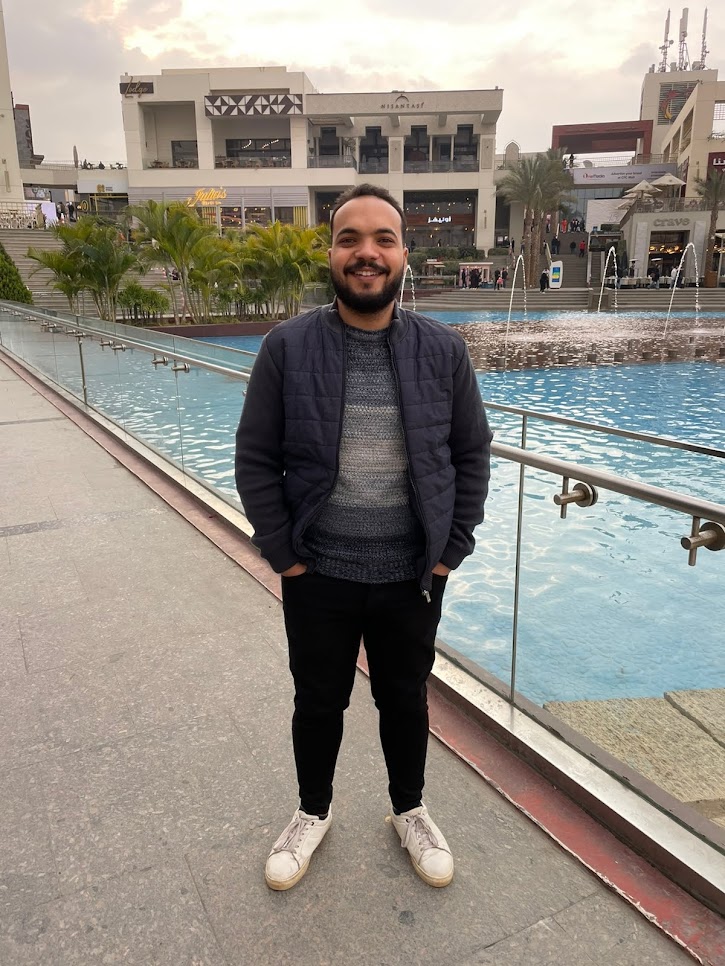
Mohamed Abd El-Meged
Mohamed Abd El-Meged
Hello! My name is Mohamed Abd El-Meged and I am an experienced backend engineer. Over the past four years, I have specialized in working with frameworks such as Ruby on Rails and Django, and have successfully utilized multiple types of databases, including MySQL, PostgreSQL, and in-memory datastore such as Redis. Additionally, I have experience with message brokers such as RabbitMQ and Apache Kafka, and GRPC. I am also well-versed in working with search engines such as Elasticsearch and Algolia. Throughout my career, I had the opportunity to work across a variety of industries, including IoT, healthcare solutions, e-learning, and food delivery I am also well-versed in writing unit tests using rspec for rails and pytest for Django , which ensures that my code is of the highest quality and produces the best possible results In addition to my expertise in backend engineering, I also have knowledge of other technologies such as ReactJS and AWS basics, which enables me to contribute to various projects in a more comprehensive and holistic manner.