Create Your Own Chatbot with Gemini API

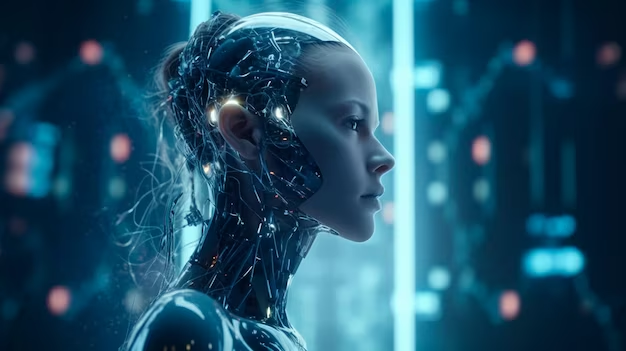
In last blog, we discussed how can we use Images in API on ExpressJs with Help of Gemini. In this Blog, We will learn how can we implement chat functionality into our Node Application.
Prerequisites
- Refer this for setting up the base for this Article: Gemini API: Prompts with Image
Introduction
Until this point we were using Express to create basic REST APIs. But, we will be learning to use and test Socket.io to implement chat systems. Socket.io ensures Bidirectional and low-latency communication for every platform.
1. Setting Up the Environment:
1a. Package Installations
Firstly, we will need to install socket.io package to enable our server to provide socket.io connections.
npm install socket.io
1b. Testing Extension Installations
I will be using Green Client Extension for VS Code to test the Socket.io connections. You may use any testing suite including API Clients like Postman.
2. Implementing Socket Communication
2a. Creating a template for listening events on Socket.
Lets start by understanding simple socket events.
connection
: Called when user wants to connect to the socket
disconnect
: Called when user gets disconnected. (default)
chat message
: Called when user wants to prompt
Note: You can use your own event names except for disconnect
that is called when user gets disconnected. For example, instead of chat message
, we can call the event prompt
.
Create a file router/geminichat.js
and put the following code:
let chat_functions=(io)=>{
//when connection is created
io.on('connection', (socket) => {
console.log('a user connected');
//Initialize Chat Connection
//When socket connection is disconnected
socket.on('disconnect',()=>{
console.log('user disconnected');
});
//When We Send a 'chat message'
socket.on('chat message', (msg) => {
console.log('message: ' + msg);
});
});
}
module.exports= chat_functions;
2b. Adding Socket Support to Server.
We will need to update code for index.js as follows:
i. Add Declarations for new dependencies
ii. Create Socket.io connections.
iii. Update app.listen
to server.listen
as we want to listen the socket.io connections as well.
//Add the following to initail declartations with express and dotenv
const { Server } = require("socket.io");
const { createServer } = require('node:http');
const chat_functions=require('./router/geminichat');
const app=express();
const port=8000;
const server = createServer(app); //TO Add
const io=new Server(server);//To Add
chat_functions(io);
//update app.listen to server.listen
server.listen(port,function(err){
console.log("Server is running on port: ",port);
});
2c. Testing Sockets
Now that we have implemented the sockets, we need to test if it works correctly. You can use any testing suite, here I am using Green Client
Vs Code Extension for testing.
You can click Save Connection. After saving, You can see a screen like this:
i. You can use plug icon to connect the server. After connection is established, we can use Emit Message Function to communicate with the server
ii. Event Name is used to further emit and communicate with the server. In our case, chat message
event is used to chat with Gen AI.
iii. You can also verify the Connection on terminal as it provides logs:
3. Creating the Chat System
Now that we have a robust Socket Connection, we can start our implementation for chatting with Gemini!
3a. The Gemini Chat System
i. Lets start with Initializing the chat with gemini. In geminichat.js
update the code as follows:
const { GoogleGenerativeAI } = require("@google/generative-ai");
const genAI = new GoogleGenerativeAI(process.env.API_KEY);
let chat_functions=(io)=>{
io.on('connection', (socket) => {
console.log('a user connected');
//Initialize Chat Connection
const model = genAI.getGenerativeModel({ model: "gemini-pro"});
const chat = model.startChat();
//....Rest of the code
});
}
module.exports= chat_functions;
ii. Now that we have initialized the chat, we can update the chat message
event function as follows:
socket.on('chat message', async(msg) => {
console.log('message: ' + msg);
const result = await chat.sendMessage(msg);
const response = await result.response;
const text = response.text();
socket.emit('gemini-response', response.text());
});
Here, we wait for gemini to send a result after sending the message. Once the result is received, we emit the response on the socket notifying the user about the response that is received.
3b. (Optional) Lets Test
All the steps remains the same as previous test. But this time, we get a response!
As You see, we receive response based on the prompt we put.
Lets Summarize
We started with base template that we created in last blog. First we implemented sockets using Socket.io to ensure fast and reliable communication. Next we created events to listen on prompt. Finally, we used gemini-API and sent the response to the user.
Subscribe to my newsletter
Read articles from Tanmai Kamat directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
