Transactions in Spring Boot
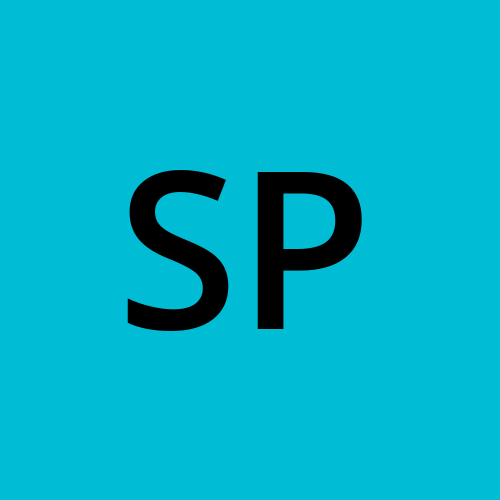
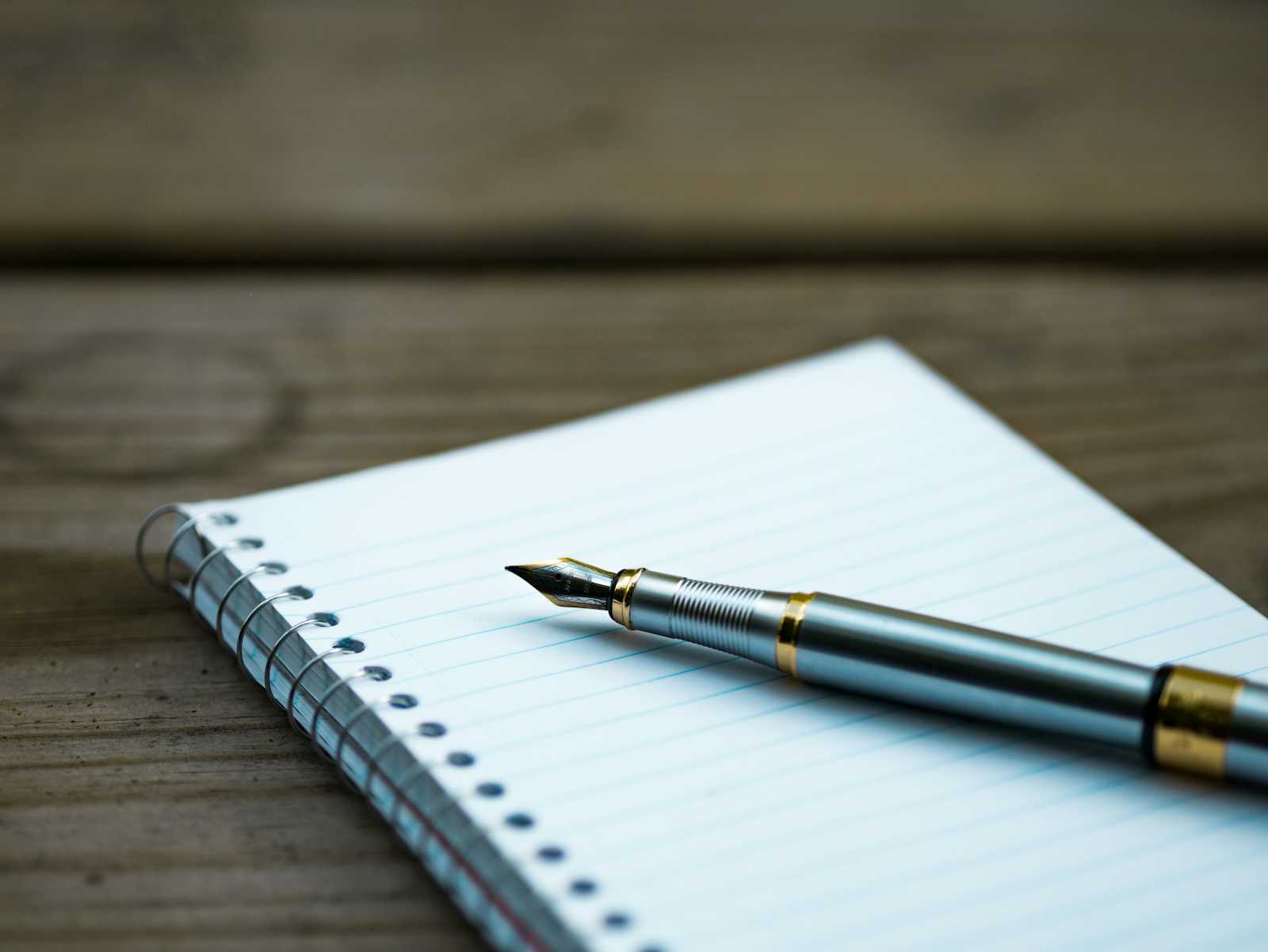
In Spring Boot, transactions are managed using the Spring Framework's transaction management capabilities. Transactions are essential for ensuring data consistency and integrity in database operations. Spring Boot provides several ways to manage transactions, including annotation-based and programmatic approaches.
Here's an overview of how transactions can be handled in Spring Boot:
Declarative Transaction Management with Annotations: Spring Boot supports declarative transaction management using annotations such as
@Transactional
. By annotating methods or classes with@Transactional
, you can specify the transactional behavior for those methods or classes.import org.springframework.transaction.annotation.Transactional; @Service public class MyService { @Autowired private MyRepository myRepository; @Transactional public void performTransactionalOperation() { // Database operations } }
Programmatic Transaction Management: Spring Boot also allows programmatic transaction management using the
TransactionTemplate
class or directly accessing thePlatformTransactionManager
. This approach is less common but offers flexibility in specific scenarios.import org.springframework.transaction.support.TransactionTemplate; @Service public class MyService { @Autowired private TransactionTemplate transactionTemplate; public void performTransactionalOperation() { transactionTemplate.execute(status -> { // Database operations return null; // Return value if needed }); } }
Transaction Propagation: Spring Boot supports different propagation behaviors for transactions, such as
REQUIRED
,REQUIRES_NEW
,NESTED
, etc., which define how transactions are managed in nested method calls.Transaction Isolation Levels: Isolation levels determine the degree to which a transaction is isolated from the effects of other transactions. This can be configured using annotations or programmatically.
Transaction Rollback: Spring Boot automatically rolls back transactions when exceptions occur within a transactional method unless explicitly configured otherwise.
Integration with JPA and Spring Data: Transactions are automatically managed by using JPA or Spring Data JPA in Spring Boot application. Repository methods are transactional by default unless marked otherwise.
Transaction Management in Testing: Spring Boot provides utilities for testing transactional behavior, allowing us to rollback transactions after each test method execution to keep the test environment clean.
Overall, Spring Boot simplifies transaction management by providing comprehensive support for handling transactions in various scenarios, whether it's through annotations or programmatic approaches.
Subscribe to my newsletter
Read articles from Sonalika Patel directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
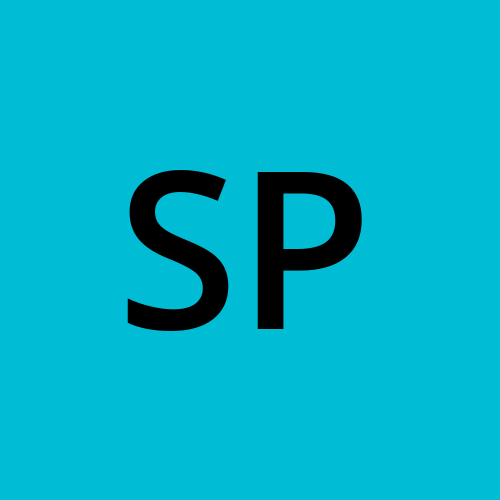