Solving CS50P - Problem Set 2
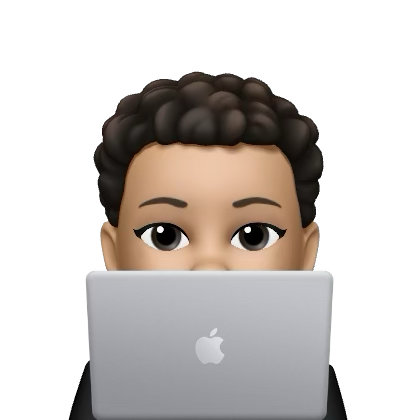
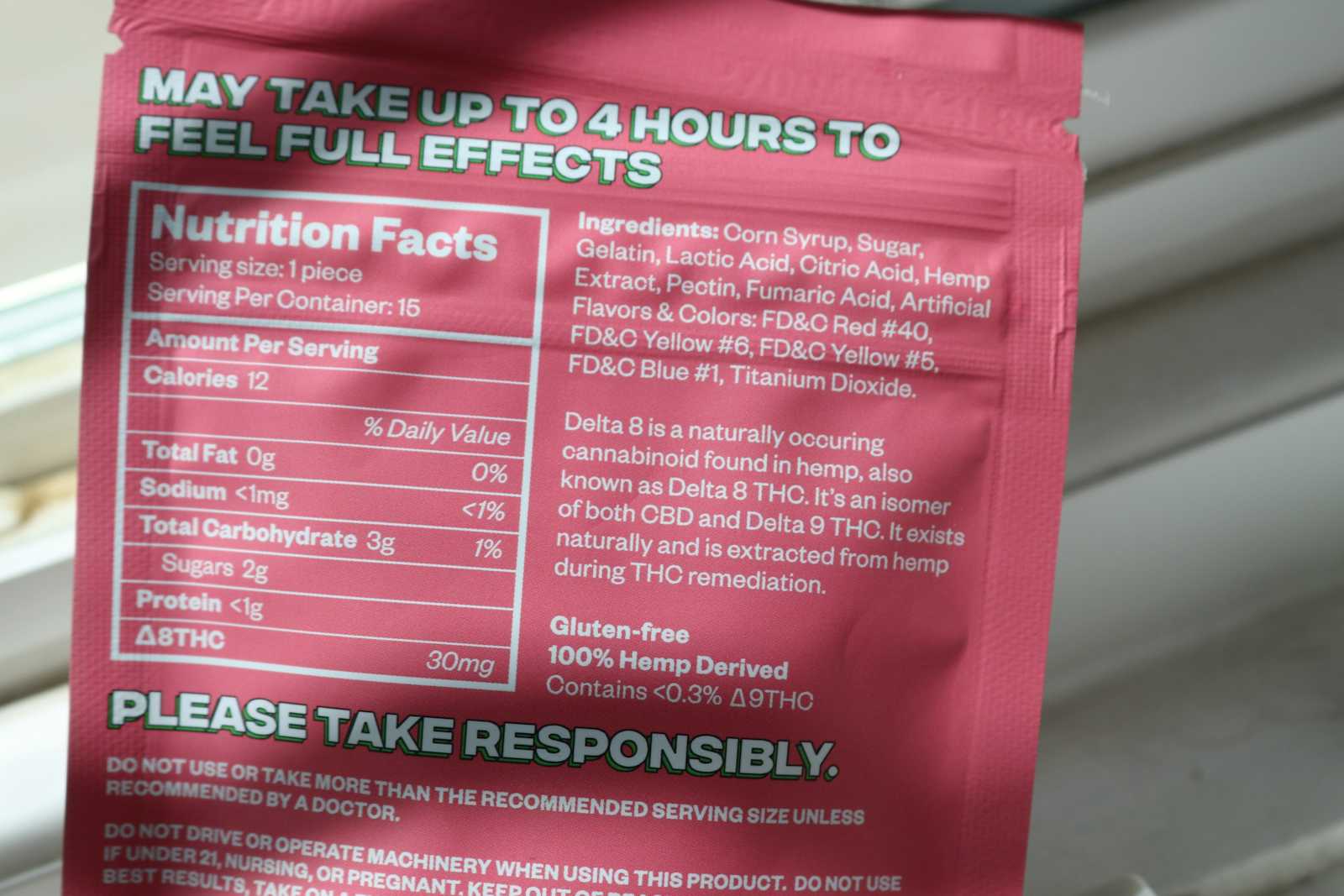
In this article, we will be delving into loops and dictionaries in Python. Loops are a fundamental aspect of programming, and there are various ways to use them to solve problems. Feel free to explore alternative approaches to the problems.
camelCase
I created a program that asks the user for input in camelCase and outputs that same input in snake_case. It prints each character in the input. If a character is uppercase, it prints an underscore followed by that character in lowercase.
camel_case = input("camelCase: ").strip()
for letter in camel_case:
if letter.islower():
print(letter, end="")
else:
print("_" + letter.lower(), end="")
print()
Coke Machine
This is a program designed for a vending machine that prompts the user to insert coins until they have inputted a minimum of 50 cents. The program verifies if the coin is valid and provides the user with feedback on the amount or change due after each coin insert.
valid_coins = [5, 10, 25,]
cost = 50
print("Amount Due: 50")
while True:
inserted_coin = int(input("Insert coin: "))
if inserted_coin in valid_coins:
# calculate change if coin is valid
cost -= inserted_coin
if cost < 0:
print(f"Change Owed: {cost * -1}")
break
elif cost == 0:
print(f"Change Owed: 0")
break
else:
print(f"Amount Due: {cost}")
else:
print("Amount Due: 50")
Nutrition Facts
This program prompts the user to input a fruit and outputs the corresponding calorie count of that fruit. The program checks if the input is a key in the dictionary and prints the corresponding value.
fruit = {
"Apple": 130,
"Avocado" : 50,
"Sweet Cherries" : 100,
"Kiwifruit" : 90,
"Pear" : 100,
}
user_input = input("Item: ").title()
if user_input in fruit:
print(f"Calories: {fruit[user_input]}")
else:
None
Vanity Plates
This program prompts the user to input a licence plate. It determines whether the input is valid by checking certain criteria, and outputs the result as: "Valid" or "Invalid."
def main():
plate = input("Plate: ")
if is_valid(plate):
print("Valid")
else:
print("Invalid")
def is_valid(s):
if s[0:2].isalpha() and \
2 <= len(s) <= 6 and \
first_not_zero(s) and \
number_at_end(s) and \
check_punctuation(s):
return True
else:
return False
def first_not_zero(n):
for char in n:
if char.isdigit():
break
if char != "0":
return True
def number_at_end(num):
if num.isalpha():
return True
else:
# initialize first digit
first_digit = None
# look for fist digit
for i in num:
if i.isdigit():
first_digit = i
break
_, end = num.split(first_digit, 1)
for j in end:
if j.isalpha():
return False
return True
def check_punctuation(p):
import string
for char in p:
if char in string.punctuation:
return False
return True
main()
Just setting up my twttr
This program prompts the user for input and iterates through each character in the input, omitting all vowels and printing only consonants. The continue keyword is used to skip the iteration of the vowels.
# ask user for input
user_input = input("Input: ")
vowels = ["A", "a", "E", "e", "I", "i", "O", "o", "U", "u"]
print("Output: ",end="")
for letter in user_input:
if letter in vowels:
continue
print(letter, end="")
print()
Conclusion
Initially, loops might seem daunting and frustrating, but with consistent practice, you will definitely get the hang of it and be able to harness their full power. Happy coding!
Subscribe to my newsletter
Read articles from Karabo Molefi directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
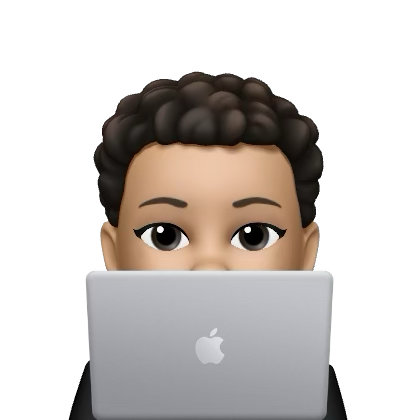