Safeguard Your Code: 6 Basic Vulnerabilities to Avoid and Tips to Improve Your Code Security
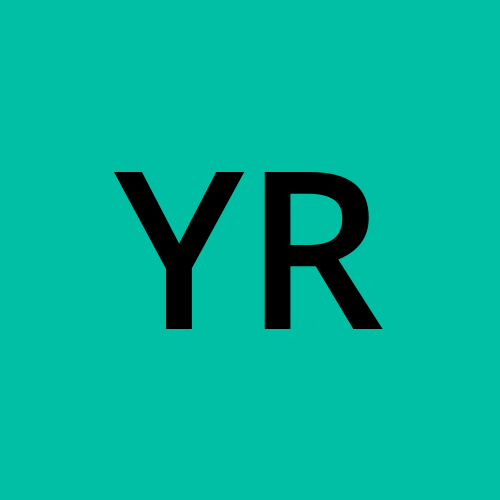
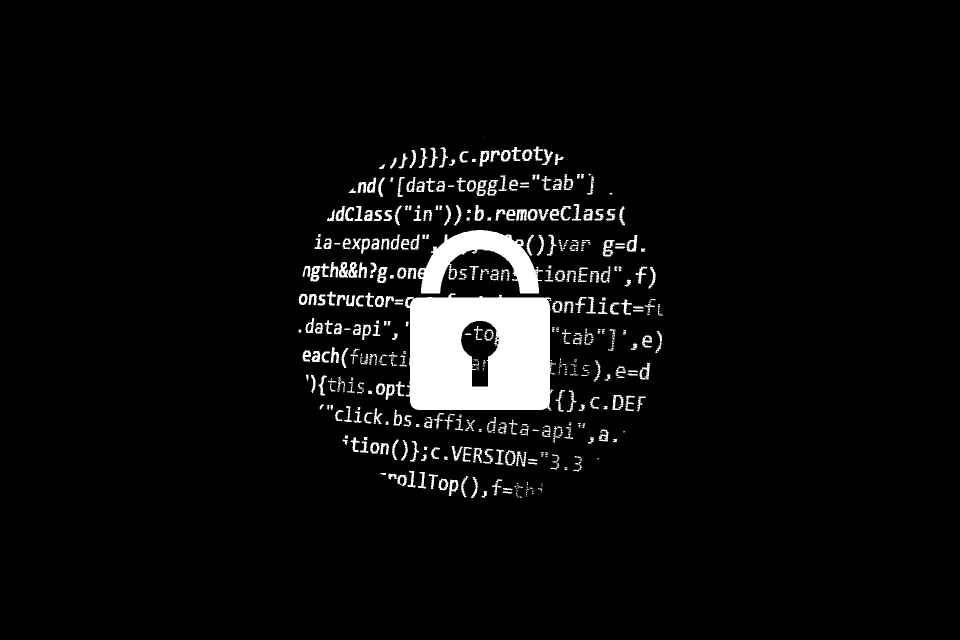
In the coding world, even the smallest oversight can pave the way for security vulnerabilities, leaving your application susceptible to exploitation by malicious actors. Cybersecurity incidents are rising and will continue as long as there is vulnerable code. It is impossible to write 100% safe code; after all, all programmers are human, and everyone makes mistakes regardless of their level of expertise. We must recognize this while also improving the code security. We can achieve it by being mindful of common pitfalls and best practices and prioritizing security.
Here are six essential vulnerabilities that I've learned in my ongoing Cybersecurity journey that I hope can help you with your code. Although the following examples are in C code, these vulnerabilities are not attached to a specific language:
Unsanitized Inputs: Trusting user inputs blindly is equal to leaving the front door of your application wide open. Therefore, it is crucial to validate and sanitize inputs to prevent injection attacks like SQL injection or cross-site scripting (XSS). Input validation ensures that only expected and safe data enters your system, protecting it from malicious payloads.
Example:
char username[50]; printf("Enter your username: "); fgets(username, sizeof(username), stdin); // Use fgets() for safer input reading
Explanation:
fgets()
is used to read user input into theusername
array. Unlike other input functions likescanf()
,fgets()
allows specifying the maximum number of characters to read, thereby preventing buffer overflow. This ensures safer input handling and reduces the risk of vulnerabilities. Note:scanf()
opens your code to malicious inputs. Why? It doesn't check if the user input is within the data bytes allocated for an input. Therefore, whatever the amount of data the user inputs, C will put that data at the memory address, even if there isn't enough space for it. This can cause the program to crash and introduce a known exploit: buffer overflow. This is whyfgets()
is recommended.Improper Error Handling: Neglecting error handling might seem inconsequential until it leads to information leakage or system crashes. Avoid verbose error messages that disclose sensitive information and opt for graceful error-handling mechanisms. Logging errors securely can help you identify and mitigate issues without exposing your system's inner workings.
Example:
FILE *file = fopen("example.txt", "r"); if (file == NULL) { fprintf(stderr, "Error: Unable to open file 'example.txt' for reading.\n"); exit(EXIT_FAILURE); }
Explanation:
fprintf()
is used to print a custom error message to the standard error stream (stderr
) if the file opening fails. This custom error message provides more descriptive information about the error and the specific file that couldn't be opened, enhancing clarity for the user or the developer. Finding a balance between providing informative error messages for troubleshooting purposes and safeguarding confidential data from potential misuse by malicious individuals is crucial.Using Deprecated or Vulnerable Libraries: Relying on outdated libraries is akin to building your castle on a crumbling foundation. Regularly update dependencies to patch security vulnerabilities and benefit from new features and optimizations. Utilize tools like dependency checkers to stay informed about the latest updates and security advisories.
Example:
// Example of using outdated OpenSSL library function #include <openssl/sha.h>
Explanation:
In this example, the code includes a header file from the OpenSSL library, which may contain functions that are deprecated or vulnerable to security exploits. This practice can introduce potential security risks into the codebase. Outdated libraries may have known vulnerabilities that could be exploited. To mitigate these risks, it's important to regularly update dependencies and use the latest versions of libraries, ensuring that security patches are applied and known vulnerabilities are addressed.
Using deprecated or vulnerable libraries like OpenSSL can introduce significant security risks into your codebase. It's crucial to stay informed about security advisories and regularly update dependencies to mitigate these risks. OpenSSL has encountered notable vulnerabilities in the past, including:
Heartbleed (CVE-2014-0160): A critical vulnerability in OpenSSL's TLS Heartbeat extension, allowing attackers to read sensitive information from server memory, potentially exposing private keys, user credentials, and other confidential data.
POODLE (CVE-2014-3566): This attack exploited a vulnerability in SSL 3.0, supported by OpenSSL, allowing attackers to decrypt encrypted data by exploiting the padding behavior of SSL 3.0 cipher suites.
DROWN (CVE-2016-0800): A vulnerability affecting OpenSSL and other TLS implementations, enabling attackers to decrypt encrypted communications by exploiting servers supporting SSLv2 and modern TLS protocols.
BLEichenbacher's ROBOT Attack (CVE-2017-3737): This vulnerability allowed attackers to decrypt encrypted communications by exploiting a flaw in the TLS protocol's RSA decryption process, affecting servers using OpenSSL.
While OpenSSL has tried to address these vulnerabilities, it's essential to consider alternatives like wolfSSL. WolfSSL offers a lightweight, portable, and secure SSL/TLS library for resource-constrained environments like embedded systems and IoT devices, prioritizing security and performance.
Insecure Data Storage: Storing sensitive data like passwords or API keys in plaintext is like storing a treasure in a glass jar. Employ cryptographic techniques like hashing and encryption to secure sensitive information. Additionally, implement proper access controls and utilize secure storage solutions to mitigate the risk of data breaches.
Example:
// Storing password in plaintext char password[50] = "mysupersecretpassword"; // Storing API Keys in plaintext char WeatherAPI[200] = "eyJhbGciOiJIUzI1NiIsInR5cCI6IkpXVCJ9.eyJzdWIiOiIxMjM0NTY3ODkwIiwibmFtZSI6IkpvaG4gRG9lIiwiaWF0IjoxNTE2MjM5MDIyfQ5SflKxwRJSMeKKF2QT4fwpMeJf36POk6yJV_adQssw5c"
Explanation:
These examples highlight a common security flaw where sensitive information, such as passwords or API keys, is stored in plaintext within the code. It's crucial to exercise caution when handling such sensitive data, especially when considering scenarios like uploading code to public repositories like GitHub. Storing data as plaintext means storing it without any form of protection, leaving it vulnerable to unauthorized access.
Developers should prioritize the adoption of cryptographic techniques such as hashing and encryption to safeguard sensitive information effectively.
Hashing: involves converting data into a fixed-size string of characters, known as a hash, using a mathematical algorithm. Unlike encryption, hashing is a one-way process, meaning it cannot be reversed to retrieve the original data. By hashing passwords before storing them, even if the hashed passwords are compromised, the original password cannot be retrieved by attackers.
Encryption, on the other hand, entails transforming data into a scrambled format using an encryption algorithm and a secret key. Unlike hashing, encryption can be reversed with the decryption key to retrieve the original data. Encrypting sensitive data like passwords or API keys ensures that even if unauthorized users gain access to the data, they cannot decipher it without the decryption key.
Moreover, it's essential to implement proper access controls to restrict unauthorized access to sensitive data. Access controls delineate who can access what data and what actions they can perform. By enforcing stringent access controls, developers can significantly mitigate the risk of data breaches and unauthorized access attempts.
It's worth noting that storing passwords in plaintext is highly discouraged in professional environments. The best practice is to hash passwords and compare the hash value for authentication. However, instances of insecure code like storing passwords in plaintext may still be encountered as developers continue to learn and adopt best practices.
Ignoring Security Best Practices: Disregarding established security best practices is like navigating a ship without a compass in an unfamiliar sea. Embrace principles like the principle of least privilege, secure by default, and defense in depth. Regularly conduct security audits, penetration testing, and code reviews to fortify your defenses against evolving threats.
Example:
// Not validating user permissions before accessing sensitive data if (userRole == ADMIN) { // Allow access to admin panel without proper authorization check }
Explanation:
In this example, the code fails to validate user permissions before granting access to sensitive data or functionalities. Without proper authorization checks, any user with the ADMIN role can gain unauthorized access to the admin panel, potentially compromising the security of the application. To mitigate this risk, ensure your code implements robust authorization checks based on user roles or specific permissions. Consider using role-based access control (RBAC), access control lists (ACLs), or other authorization mechanisms to restrict access to sensitive functionalities only to authorized users. Regularly audit and review access controls to maintain the integrity and security of your application.
Mindful Usage of
printf()
:When working with user input in C, it’s crucial to exercise caution, especially when using functions like
printf()
that support format specifiers such as%s
,%d
, etc. Mishandling user input usingprintf()
can lead to vulnerabilities known as format string vulnerabilities.
#include <stdio.h>
int main() {
char user_input[50];
// Unsafe usage of printf with format specifiers
printf("Enter your name: ");
fgets(user_input, sizeof(user_input), stdin);
printf("Hello, %s!\n", user_input); // Potential format string vulnerability
return 0;
}
Explanation:
In the example, if an attacker inputs a string containing format specifiers, they could exploit this vulnerability to compromise the program's security and potentially leak sensitive information or execute arbitrary code.
When using printf()
with user_input
obtained from fgets()
without proper validation, it can result in potential format string vulnerabilities. Format string vulnerabilities occur when an attacker provides input that includes format specifiers (e.g., %s
) to printf()
or similar functions. If the code fails to properly sanitize or validate the user input, the attacker can manipulate the format string to read or write arbitrary memory, potentially leading to unauthorized access or code execution.
Examples of Potential Vulnerabilities:
Password Leak: An attacker could input a format string containing
%s
to read sensitive information stored in memory, such as passwords or other confidential data. This information could then be leaked to the attacker, compromising the security of the system.Arbitrary Code Execution: By crafting a carefully constructed format string, an attacker could overwrite critical memory addresses and inject malicious code into the program's execution flow. This could lead to arbitrary code execution, allowing the attacker to take control of the system, escalate privileges, or perform other malicious actions.
Safe Usage of printf():
To use printf()
safely, it’s essential to properly sanitize the input to prevent format string vulnerabilities. In this example, the user input is sanitized by replacing the newline character (\n
) added by fgets()
with a null terminator (\0
), ensuring that the input is properly null-terminated before being used with printf()
.
#include <stdio.h>
int main() {
char user_input[50];
// Safe usage of printf with proper input sanitization
printf("Enter your name: ");
fgets(user_input, sizeof(user_input), stdin);
for (int i = 0; i < sizeof(user_input); i++) {
if (user_input[i] == '\n') {
user_input[i] = '\0';
break;
}
}
printf("Hello, %s!\n", user_input); // Safe usage with sanitized input
return 0;
}
Consideration for Simple String Output:
Additionally, if you're only printing a string without any format specifiers or user input, it’s safer to use puts()
instead of printf()
. puts()
automatically appends a newline character to the output and doesn’t support format specifiers, reducing the risk of format string vulnerabilities. It’s a straightforward and safer alternative for outputting simple strings.
#include <stdio.h>
int main() {
// Safer alternative with puts() for simple string output
puts("Good luck in your security journey!"); // No format specifiers used
return 0;
}
These are only six vulnerabilities that I thought would be helpful to share. However, there are many more! Keep learning and researching how to improve your code. I will continue my journey and share new things I learn along the way.
Now that you've gained insights into common vulnerabilities and best practices for securing your code, it's time to take action. Here's what you can do next:
Audit Your Code: Conduct a thorough review of your existing codebases to identify any potential security vulnerabilities. Look for areas where unsanitized inputs, improper error handling, or insecure data storage practices may be present.
Implement Security Measures: Take proactive steps to address the vulnerabilities you've identified. Update your code to incorporate input validation, proper error handling, and secure data storage techniques such as hashing and encryption.
Stay Informed: Stay abreast of the latest developments in cybersecurity and coding best practices. Follow reputable sources, participate in online communities, and continue learning to enhance your knowledge and skills in code security.
Share Your Knowledge: Spread awareness about the importance of code security within your professional network and community. Share this article or other resources with colleagues and peers to help them improve their coding practices.
Remember, proactive security measures are essential for safeguarding your code and protecting your applications from potential threats. By taking these steps, you contribute to building a more secure and resilient software ecosystem.
Keep learning, stay vigilant, and prioritize security in your coding journey.
Subscribe to my newsletter
Read articles from Yarelys Rivera directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
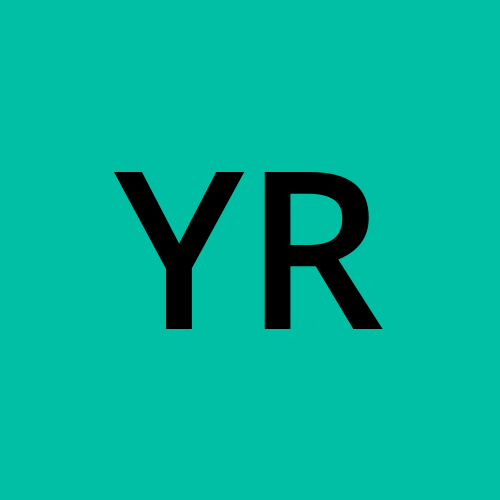
Yarelys Rivera
Yarelys Rivera
Welcome to CyberYara! I created this space to share insights on cybersecurity, programming, and the latest in tech. With a background in cybersecurity, leadership, and technology, I enjoy breaking down complex topics and making them accessible to a wider audience. My journey into cybersecurity began when I witnessed firsthand how frequent phishing attacks disrupted an organization I worked for. That experience led me to dive deep into security practices, earning certifications like GFACT, GSEC, and GCIH after intensive training at SANS Cyber Academy. I also completed the Google Cybersecurity Certificate and hold a Scrum Master Certification (PSM I). Beyond cybersecurity, I enjoy learning and sharpening my technical skills in Python, SQL, HTML & CSS, and AI. I also have extensive experience in operations and leadership, having managed diverse teams and ensured compliance across multiple projects. My background in journalism and psychology gives me a unique perspective on tech—how we communicate it, how we secure it, and how it impacts people. On this blog, you’ll find practical cybersecurity tips, programming tutorials, discussions on tech trends, and more. Whether you’re a beginner or a seasoned professional, I hope CyberYara sparks curiosity, learning, and meaningful conversations. Thanks for stopping by!