Dart Core Libraries 'dart:core'
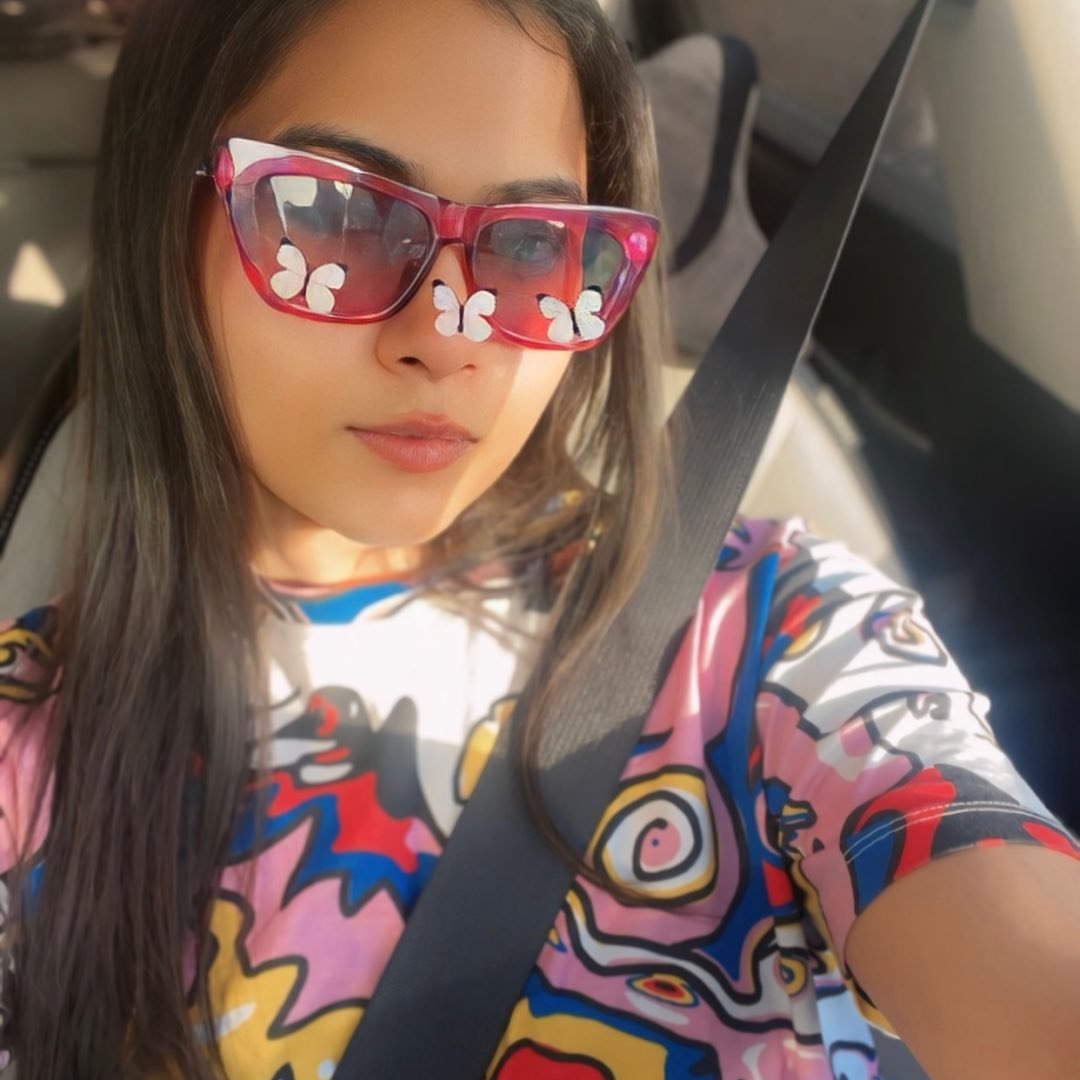
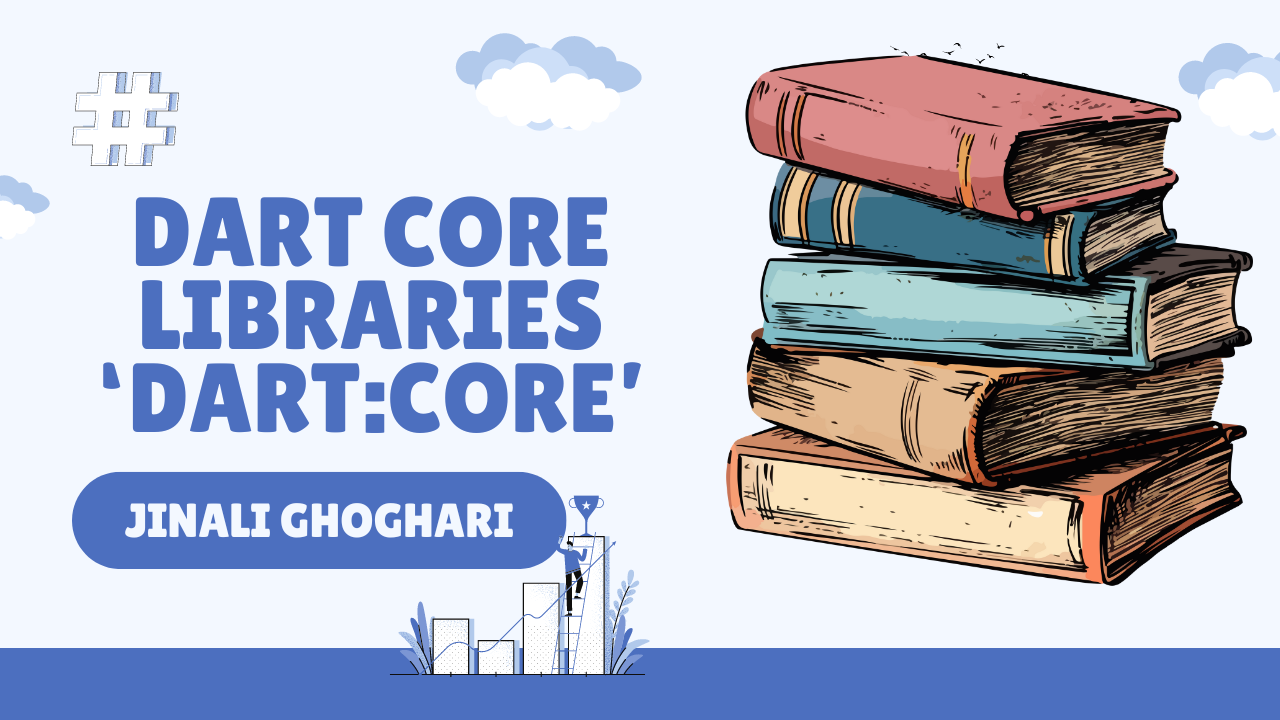
The dart:core
library is automatically imported into every Dart program and contains fundamental types, utilities, and functionalities that are essential for Dart programming.
t includes core types, collections, utilities, and annotations that are essential for everyday programming tasks. Here are some of the key components of dart:core
:
1. Core Types
Object
: The root of the Dart class hierarchy. All Dart classes implicitly extendObject
.String
: Represents a sequence of characters used for text manipulation and formatting.int
,double
,num
: Dart provides different numeric types for integers, floating-point numbers, and a common superclassnum
.bool
: Represents boolean values (true
orfalse
) used for logical operations.
2. Collections
List
: Represents an ordered collection of elements.Set
: Represents a collection of unique elements, without duplicates.Map
: Represents a collection of key-value pairs.
3. Utilities and Functions
print()
: Outputs text or values to the console for debugging and logging purposes.String Interpolation: Allows embedding expressions within strings using
${}
syntax.DateTime
: Represents dates and times.Iterables and Iterators: Provides iteration capabilities for collections.
4. Annotations
@override
: Indicates that a method overrides a superclass method.@deprecated
: Marks a program element as deprecated (discouraged from use).
Practical Examples
Let's demonstrate how these core components of dart:core
are used in practice:
void main() {
// Core types
String message = 'Hello, Dart!';
int number = 42;
double piValue = 3.14;
bool isDartFun = true;
// Collections
List<int> numbers = [1, 2, 3];
Set<String> names = {'Jinali', 'Moksha', 'Reet'};
Map<String, int> ages = {'Jinali': 21, 'Moksha': 20, 'Reet': 14};
// Utilities and Functions
print(message);
print('Square root of $number: ${number.sqrt()}');
print('PI value: $piValue');
print('Is Dart fun? $isDartFun');
// Using DateTime
DateTime now = DateTime.now();
print('Current date and time: $now');
}
Benefits of Understanding dart:core
Foundational Knowledge: Mastering
dart:core
provides a solid foundation for Dart programming, enabling developers to write concise and efficient code.Standardized APIs: Dart's core libraries follow standardized APIs, making it easier to collaborate on projects and understand existing codebases.
Enhanced Productivity: Leveraging the utilities and collections in
dart:core
allows developers to focus on building features rather than implementing basic functionalities from scratch.
Conclusion
In conclusion, the dart:core
library is a critical component of Dart programming that offers essential tools and utilities for developers.
Subscribe to my newsletter
Read articles from Jinali Ghoghari directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
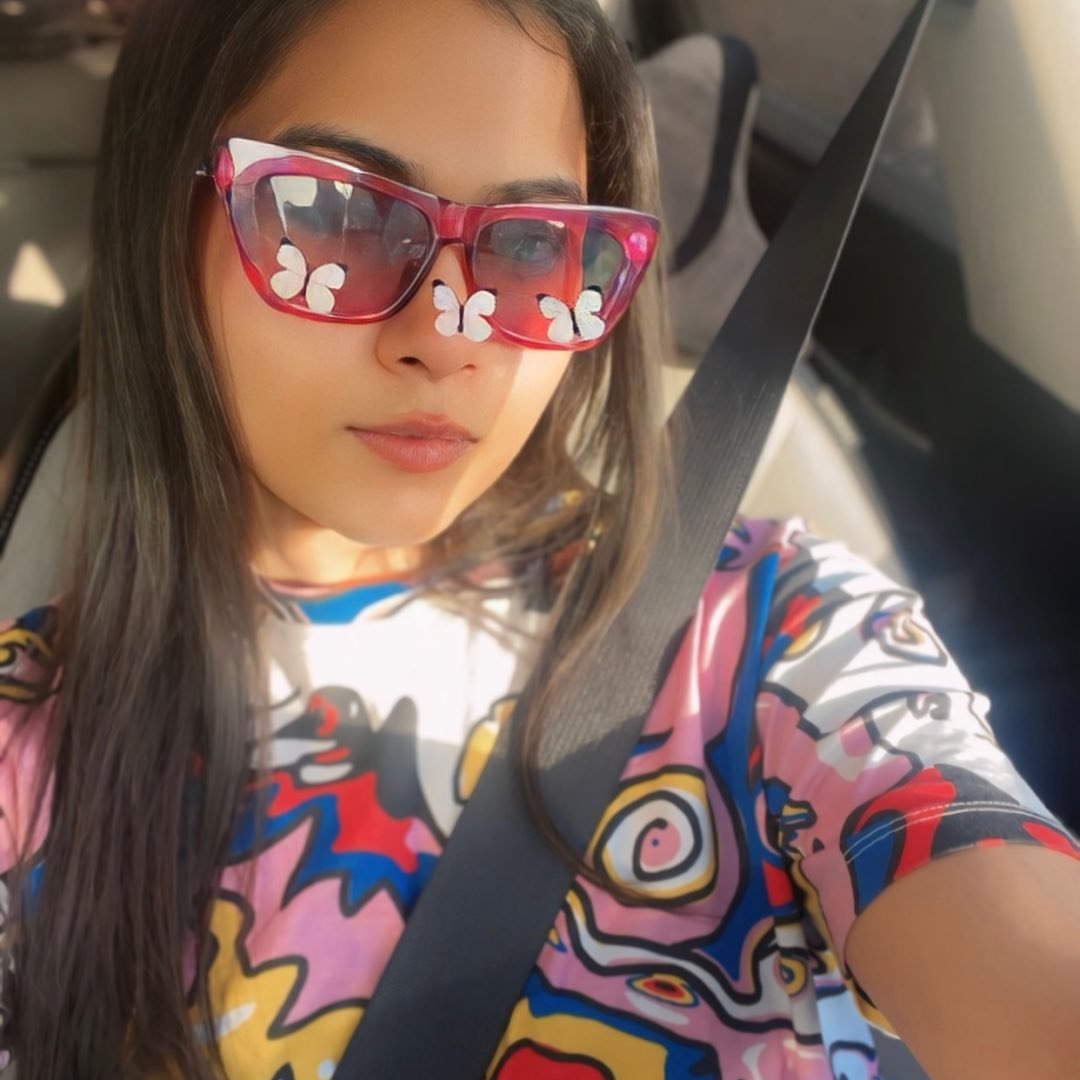