React Lesson #3 - JSX Attributes & Styling React Elements
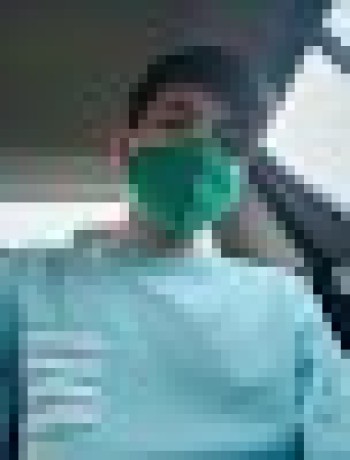
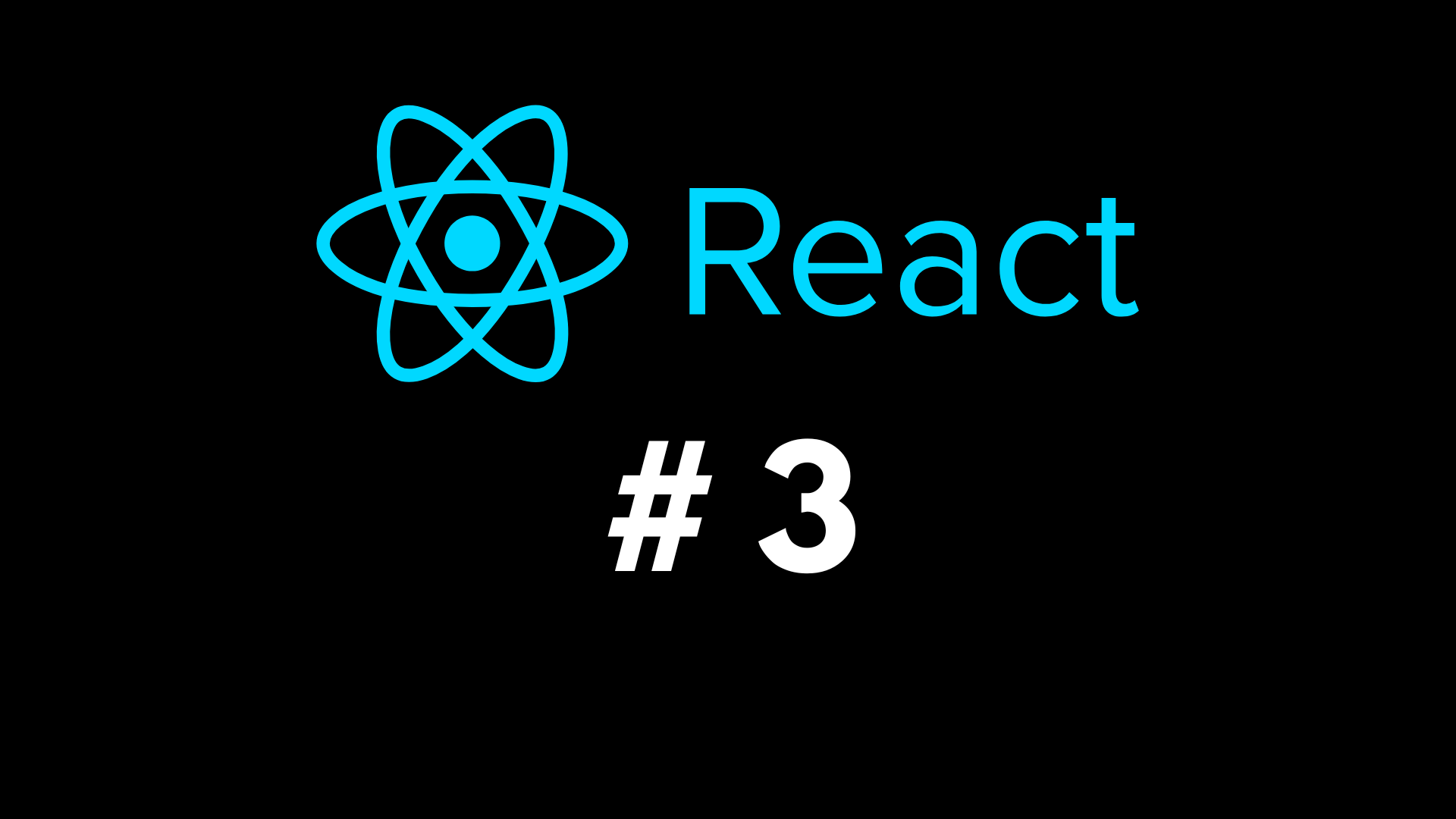
Our goal is to change the color of this heading to red:
Before moving forward, let's organize the file structure and the basic HTML structure.
index.html
<!DOCTYPE html>
<html lang="en">
<head>
<title>JSX</title>
<link rel="stylesheet" href="styles.css" />
</head>
<body>
<div id="root"></div>
<script src="../src/index.js" type="text/JSX"></script>
</body>
</html>
Here, notice that in the script tag, in the type attribute we have used "text/JSX". We use it to tell the browser that we are using a JSX script, so that it does not generate console errors.
styles.css
.heading {
color: red;
}
index.js
import React from "react";
import ReactDOM from "react-dom";
ReactDOM.render(
<div>
<h1 className="heading">My Favourite Foods</h1>
<ul>
<li>Bacon</li>
<li>Jamon</li>
<li>Noodles</li>
</ul>
</div>,
document.getElementById("root")
);
As we can see in h1 tag, we have used the attribute "className" instead of just "class". Because in JavaScript DOM, that is what we are supposed to use. However, you will find that just "class" attribute works too. But it is going to generate console errors. Hence to avoid that we write it according to JavaScript DOM.
Subscribe to my newsletter
Read articles from Ratan Sharma directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
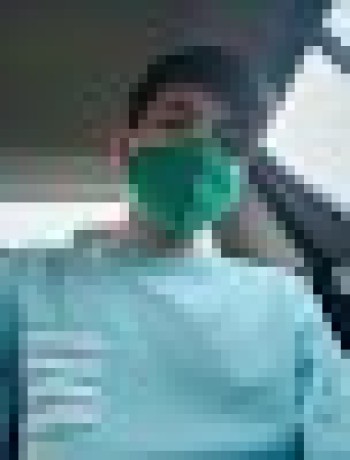