Sort the People
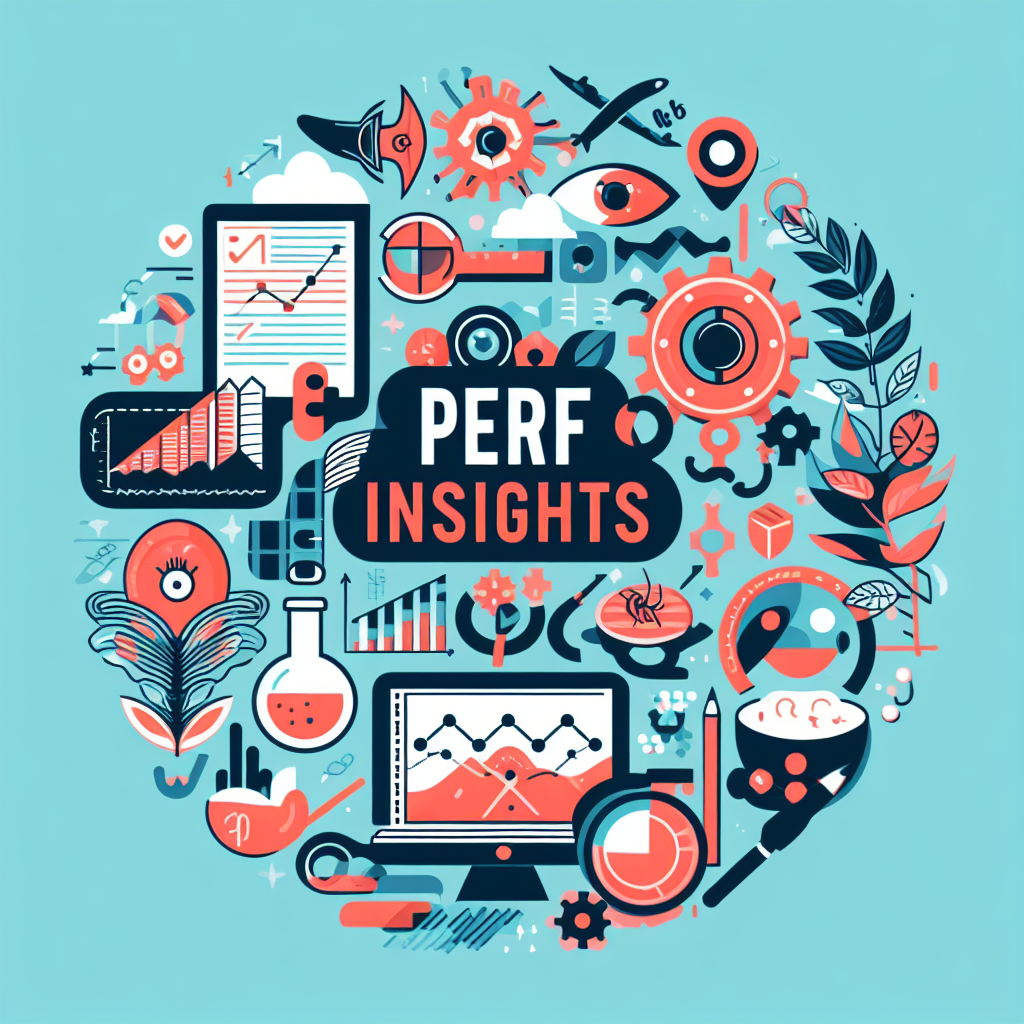
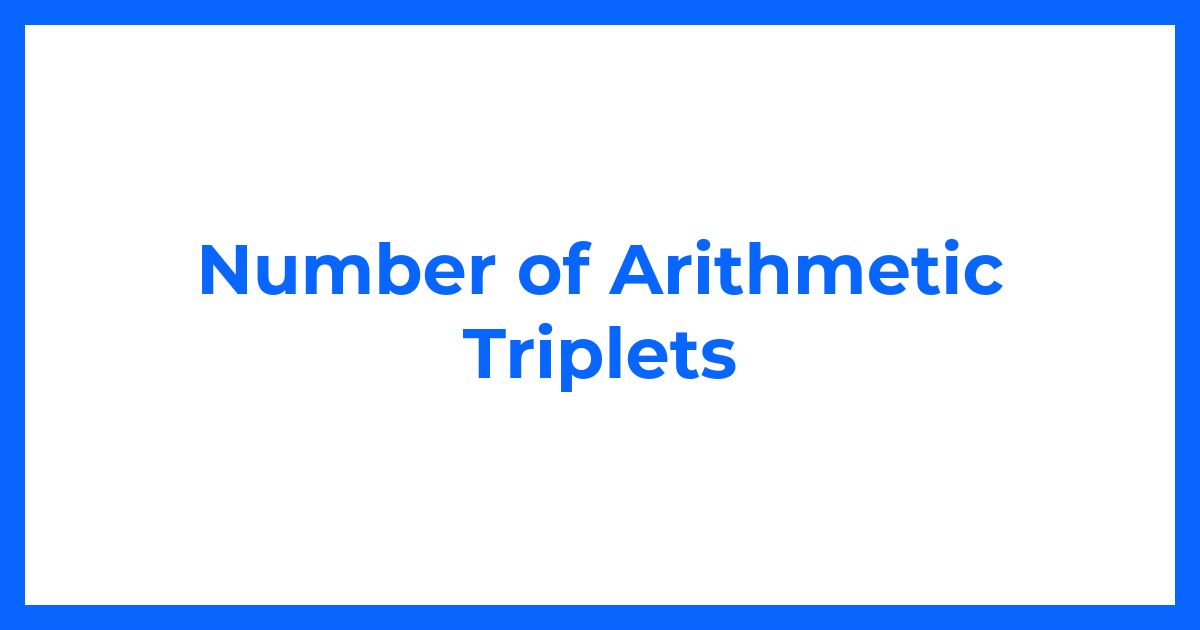
You are given an array of strings names
, and an array heights
that consists of distinct positive integers. Both arrays are of length n
.
For each index i
, names[i]
and heights[i]
denote the name and height of the i<sup>th</sup>
person.
Return names
sorted in descending order by the people's heights.
LeetCode Problem - 2418
import java.util.Arrays;
import java.util.HashMap;
import java.util.Map;
class Solution {
// Method to sort people by their heights
public String[] sortPeople(String[] names, int[] heights) {
// Create a HashMap to store height as key and name as value
Map<Integer, String> map = new HashMap<>();
// Populate the HashMap with heights and corresponding names
for (int i = 0; i < heights.length; i++) {
map.put(heights[i], names[i]);
}
// Sort the heights array
Arrays.sort(heights);
// Create a result array to store sorted names
String[] resultArr = new String[heights.length];
// Traverse the sorted heights array in reverse order
for (int z = 0, i = heights.length - 1; i >= 0; i--) {
// Retrieve the name corresponding to the current height
resultArr[i] = map.get(heights[z]);
// Increment the index to get the next name
z++;
}
// Return the sorted array of names
return resultArr;
}
}
Subscribe to my newsletter
Read articles from Gulshan Kumar directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
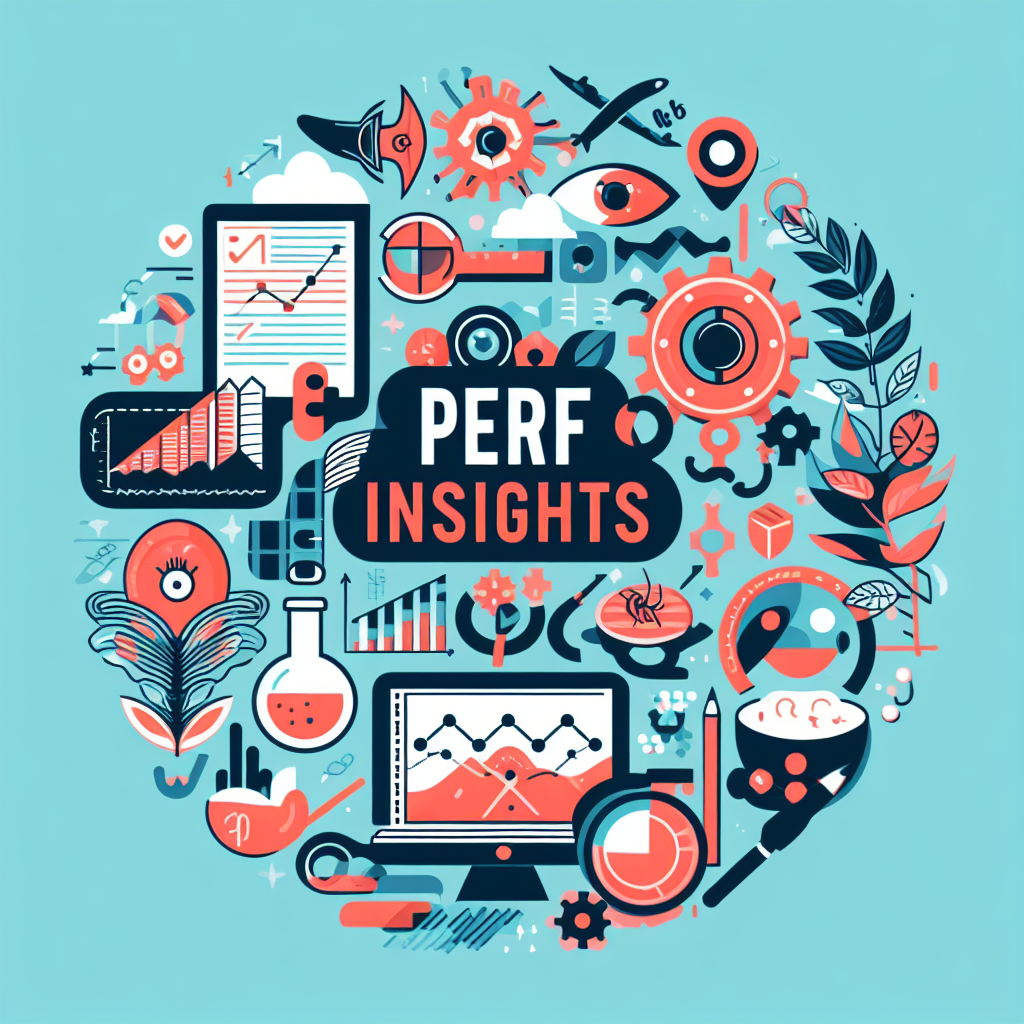
Gulshan Kumar
Gulshan Kumar
As a Systems Engineer at Tata Consultancy Services, I deliver exceptional software products for mobile and web platforms, using agile methodologies and robust quality maintenance. I am experienced in performance testing, automation testing, API testing, and manual testing, with various tools and technologies such as Jmeter, Azure LoadTest, Selenium, Java, OOPS, Maven, TestNG, and Postman. I have successfully developed and executed detailed test plans, test cases, and scripts for Android and web applications, ensuring high-quality standards and user satisfaction. I have also demonstrated my proficiency in manual REST API testing with Postman, as well as in end-to-end performance and automation testing using Jmeter and selenium with Java, TestNG and Maven. Additionally, I have utilized Azure DevOps for bug tracking and issue management.