JavaScript - Quick Introduction
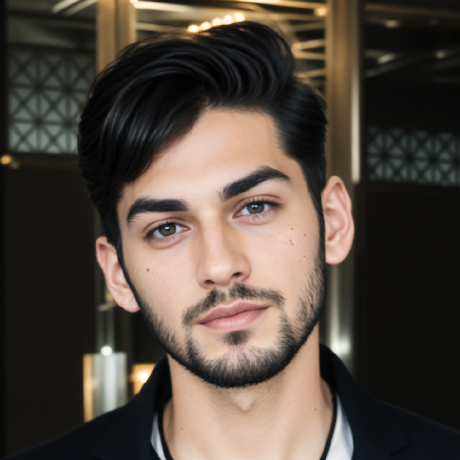
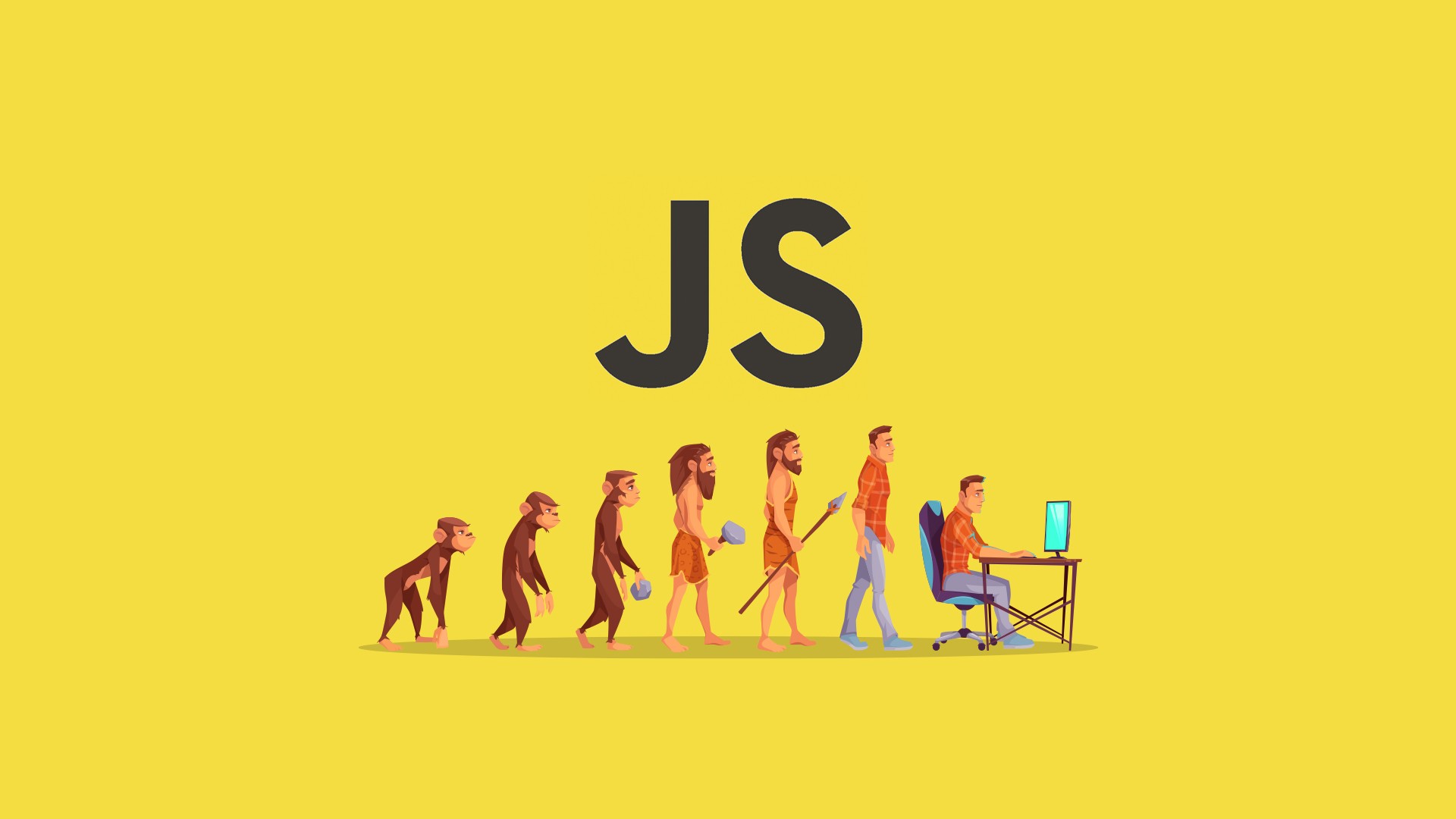
What is JavaScript?
- JavaScript is a scripting language that enables you to create dynamically updating content, control multimedia, animate images, and pretty much everything else. (Okay, not everything, but what you can achieve with a few lines of JavaScript code is amazing.)
Why Do We Need JS?
To Provide Functionalities to Our Websites! Like HTML gives structure, CSS Gives Styling, But what would happen if we press a Button? --> JavaScript will take care of it!
But Why JS and Not any other Language? --> To create a Web App or a Website the browser needs to Know the Particular Language in which we are writing our web app
This Web Browser has an Engine Built-in
Such as Chrome have V8, Edge have Chakra, Firefox have SpiderMonkey, Safari have JavaScriptCore
Node.JS
A runtime environment to Execute JS code outside the Browser
To run JS code in Terminal --> Install Node.js --> use
node <filename>.js
in your terminal to run
Interpretation and Just-in-Time Compilation (JIT)
Technically Interpreted
- At its code, JS is Technically interpreted which means The code will be executed line by line by the interpreter Like Chrome V8 Engine or Chakra etc.
But Modern JIT compilation is Faster
Modern JavaScript engines use a Technique called Just in Time Compilation. Here Frequently Executed code Blocks get Converted into machine code for faster performance on subsequent runs.
This Compilation happens at runtime not before execution (That is why it's called JIT, not AOT [Ahead of Time] where compilation happens Before execution).
So is JS a Compiled Language?
It is and It's Not
Traditionally Compiled languages Like C, C++, C#, Go, and Rust translate the whole code base into machine code. JS doesn't do this.
But it does convert some parts of the code into machine code for better performance.
So, it's your choice to Call it Compiled or Interpreted.
Steps in JIT
Parsing: JavaScript engines first parse your code, and convert it into an internal representation line Abstract Syntax Tree or AST
Initial Interpretation: The engine Might Initially interpret the code line by line for the first run
JIT Compilation(optional): This would happen based on how often your code block is executed.
Variables
A container where we can Store the Data
in JS variables Can be Declared using the
var
let
andconst
keywordTo access the Data we point to that container (variable) to access that data. This is called passing or accessing by reference.
Why do we Need variables? In JS there is a saying or Best Practice called "Do Not Repeat Yourself" and variables will help you follow that best Practice.
In JS we Declare, Assign and Reassign
Declaration -
var myVariable
Assignment -
myVariable = "JavaScript is a Good Language"
ormyVariable = 12345
once you have declared a variable, assign the value. There is no need to use the var keyword on the same variable again and again. Unless you want to Redeclare it AgainReAssignment: -
myVariable = "Well, It's not as good as I thought"
ormyVariable = "Just Kidding, It's my Favourite! Love You JavaScript.
Best Naming Practices
Rule: Variable Names Should always start with letters (a-z or A-Z), underscore(_) or $ sign followed by letters number or underscore.
Descriptive and Meaningfulness: Use Self Descriptive and Meaningful names such as
customerName
instead ofname
orx
whose name is it your name? My name? Whose name? Give your variables a meaningful name from now on.Camel Case and Consistency: Use camel case to define a variable where the first letter is lowercase and subsequent words start with upper case (looks like a camel bump) such as
creditCardgroceryStoreshoppingCart
. For function Naming you can usePascalCase
where every word starts with an uppercase. But, Always remember. Stick to your naming convention whether you are using PascalCase, camelCase or snake_case. STICK TO IT!!!!.
Data Types in JS
Primitive
Numbers
Strings
Booleans
Null
Undefined (Declared but to defined)
Symbols(Special Types of Data types introduced in ES6, Uniques and Immutable, Ofter used as object property key)
Composite
Objects
Arrays
Functions
Special Data Types
- BigInt - Introduced in ES10, used for working with arbitrarily large integers
Operators in Js
Arithmetic Operators:
Addition (+): Adds two values or concatenates strings.
Subtraction (-): Subtracts one value from another.
Multiplication (*): Multiplies two values.
Division (/): Divides one value by another.
The remainder (%): Returns the remainder of a division.
Exponentiation (**): Raises a number to a power.
Comparison Operators:
Equal ( == ): Checks if two values are equal.
Strict Equal ( === ): Checks if two values are equal without type conversion.
Not Equal ( != ): Checks if two values are not equal.
Strict Not Equal ( !== ): Checks if two values are not equal without type conversion.
Greater Than (>): Checks if one value is greater than another.
Less Than (<): Checks if one value is less than another.
Greater Than or Equal (>=): Check if one value is greater than or equal to another.
Less Than or Equal ( <= ): Check if one value is less than or equal to another.
Logical Operators:
Logical AND (&&): Returns true if both operands are true.
Logical OR (||): Returns true if at least one operand is true.
Increment(++) & Decrement(--)
Increases or Decreases the Value by 1
In this, we have something called Pre and Post increment
let num = 10
Preincrement increases the value first and then uses it. So
console.lot(++num)
will output 11Whereas Postincreament uses the value first and then increases it. So, console.log(num++) will output 10 then we again
console.log(num)
it will output 11
Subscribe to my newsletter
Read articles from Akshay Parihar directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
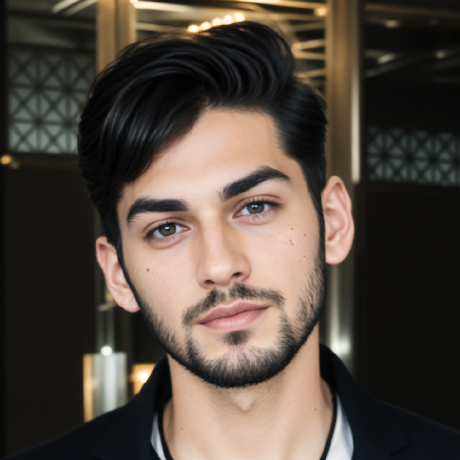