Part 3: Finalizing the Email Registration System and Deployment
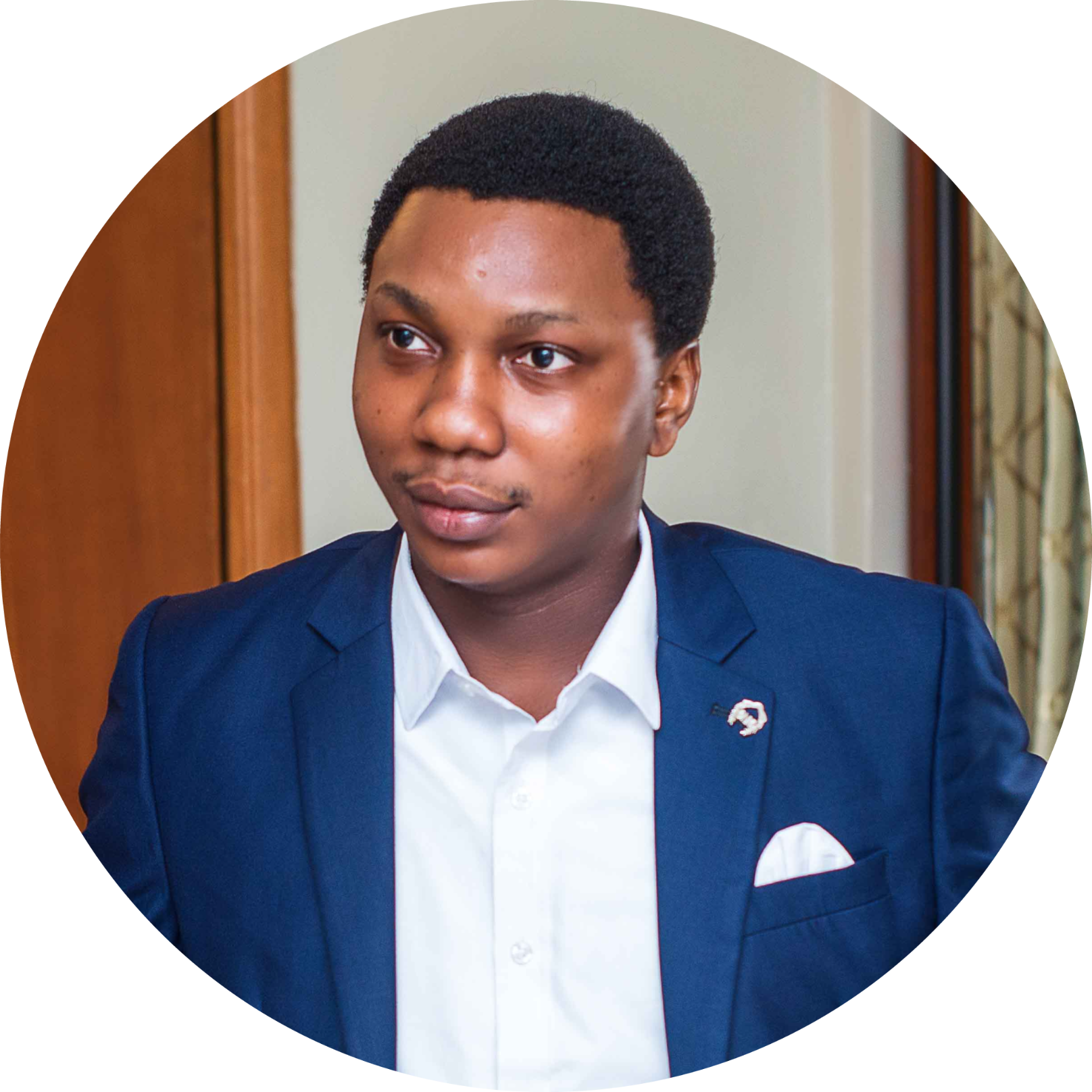
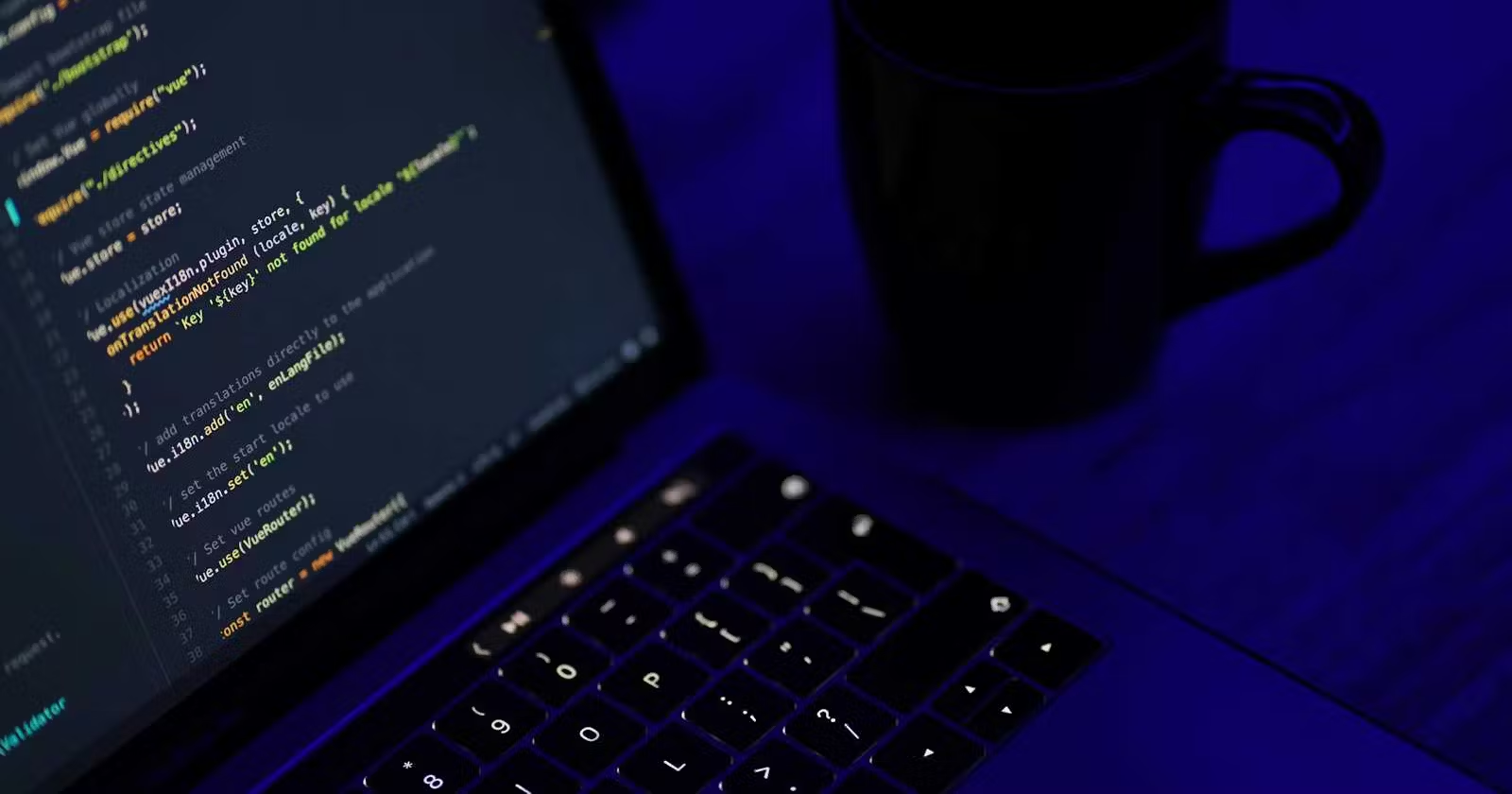
Looking back on our path, Parts 1 and 2 of our series carefully established the groundwork and guided us through the detailed creation of an automated email registration system using Azure Functions. From the initial setup of our Node.js project to carefully integrating the capabilities of Azure Functions with SendGrid's robust email service, we've tackled the challenges of coding the registration logic, conducting local testing, and ensuring seamless integration for effective email automation.
In Part 2, we explored the creation of a dynamic registration function customized to process user registrations efficiently, highlighted by our case study on managing workshop registrations for a tech conference. This practical application showcased the versatility and power of Azure Functions and SendGrid and emphasized the importance of comprehensive validation and personalized communication in enhancing user experience.
As we progress to Part 3, our focus shifts to deploying the system to Azure—a step we’ve intentionally moved ahead in our journey. This early deployment is a milestone and a strategic decision to set the stage for the subsequent in-depth discussion on securing user information with Azure Table Storage and ensuring our system’s readiness through comprehensive testing in a live environment. This approach mirrors a real-world development workflow, emphasizing the significance of establishing a working environment early to understand better and address the challenges of storage solutions and final testing strategies.
This final section aims to finalize and reinforce our project, equipping it with the robustness needed to handle real-world scenarios effectively. Join us as we explore the concluding phases of our journey. We aim to ensure the smooth deployment of our system, carry out focused testing within the Azure environment, and investigate possibilities for additional improvements. Our goal is to guarantee that the automated email registration system exemplifies the potential of serverless architecture and is prepared to provide scalable, efficient, and user-focused solutions.
Finalizing the Registration Logic
As we near the completion of our journey to implement a state-of-the-art email registration system, a crucial task awaits us: refining our registration logic. This step is a review and development driven by insights from testing and feedback gathered in Part 2. Our goal is to improve the scalability and security of our registration process, ensuring it can handle an increase in user sign-ups smoothly while keeping user data highly secure and protected.
Enhancing Scalability and Performance
Our initial design established a foundational registration logic capable of handling user sign-ups. As we progress, our focus shifts toward optimizing this system to meet the demands of real-world applications, particularly in managing a significant influx of registrations. A vital aspect of this optimization is our strategic implementation of asynchronous processing, demonstrated in our sendRegistrationEmail
function. This function is engineered to send confirmation emails without blocking the execution thread, allowing our Azure Function to manage other tasks concurrently and efficiently.
async function sendRegistrationEmail(userDetails) {
let sessionDetailsString = userDetails.sessions.map(session => `${session.title} - ${session.timeSlot}`).join("\n");
const msg = {
to: userDetails.email,
from: 'lokosman5@hotmail.com',
subject: 'Registration Confirmation',
text: `Hello ${userDetails.name}, thank you for registering. Here are your session details: \n${sessionDetailsString}`
};
try {
await sgMail.send(msg);
console.log('Email sent successfully');
} catch (error) {
console.error('Error sending email: ', error);
}
}
To elevate our system's capacity for scalability and performance, we incorporate this:
- Asynchronous Processing: By implementing Node.js's non-blocking nature and the async/await syntax, our Azure Function can handle multiple registration requests in parallel. This approach minimizes latency and enhances the user experience by guaranteeing a quick and smooth registration process.
Strengthening Security Measures
The core of our system's architecture places security as a feature and the foundation of our integrity and trustworthiness. As we refine our registration logic, a critical step involves enhancing our security measures to protect user data against unauthorized access and manipulation. Central to this effort is our validateRegistrationDetails
function, which employs thorough validation checks to prevent malicious data inputs, serves as our first defense in securing user information.
function validateRegistrationDetails(details, schedule) {
let isValid = true;
let message = 'Registration details are valid.';
if (!details.name || !details.email || !details.sessions || details.sessions.length === 0) {
isValid = false;
message = 'Missing required registration details: name, email, or sessions.';
return { isValid, message };
}
let sessionTimes = details.sessions.map(s => s.timeSlot);
let sessionIds = details.sessions.map(s => s.id);
if (new Set(sessionTimes).size !== sessionTimes.length) {
isValid = false;
message = 'Selected sessions have overlapping times.';
return { isValid, message };
}
const sessionExists = sessionIds.every(id => schedule.some(session => session.id === id));
if (!sessionExists) {
isValid = false;
message = 'One or more selected sessions do not exist in the conference schedule.';
return { isValid, message };
}
return { isValid, message };
}
Building upon this foundation, we extend our security strategy to encompass:
- Secure Coding Practices: Our development process complies with secure coding guidelines, ensuring that each line of code is designed to reduce vulnerabilities. Through regular code reviews, we actively detect and address potential security weaknesses.
Deploying to Azure
The email registration system's deployment is a critical point in our journey, where our conceptual design becomes a functional reality within the Azure ecosystem. This section carefully walks you through deploying the Azure Function to a production environment, aiming for a smooth transition from development to deployment. Furthermore, we'll delve into recommended strategies for handling and securing application settings, essential for preserving the system's integrity.
Step-by-Step Deployment Guide
Deploying our Azure Function entails deliberate steps to ensure a smooth and error-free transition to Azure's cloud infrastructure. Here's how to navigate this crucial phase:
Preparing Your Code for Deployment:
Before logging into the Azure Portal and creating a new Function App, preparing and pushing your function code to GitHub is essential. This simplifies the deployment process by making your code easily accessible for deployment from your GitHub repository.
Pushing Code to GitHub Using Visual Studio Code:
Open your Azure Function project in Visual Studio Code.
Ensure all changes are saved and ready for deployment.
Open the Source Control panel in Visual Studio Code.
Stage your changes, commit them with a meaningful message, and push your commits to your GitHub repository. For reference or to follow along with a real example, you can access the GitHub repository here: Azure-SendGridApp
Login to the Azure Portal:
Go to the Azure Portal and log in using your Microsoft account credentials.
Create a New Function App:
Once you are logged in, select Create a resource
In the Azure Portal, search for and select "Function Apps".
Click on "Create" to initiate the setup process.
Configure App Settings:
Fill in the details as per your specific project configuration. Here's an example based on a real-world Azure Function deployment:
Resource Group: EmailRegSystem
Location: North Europe
Subscription: Azure subscription 1
Operating System: Windows
Runtime stack: Node.js
Adjust settings to match your project needs, focusing on application settings and connection strings. Ensure these settings are aligned with the requirements of your email registration system.
Review and Create:
- After filling in the necessary information, click the "Review + Create" button. This action will navigate you to a summary page of your configurations. Then, click deployed.
Deploy Using Visual Studio Code:
Ensure you have the Azure Functions extension installed in Visual Studio Code. This extension simplifies the process of deploying directly from the IDE
Sign into your Azure account via the Azure Functions extension to sync with your Azure portal
Click on your subscription, select the Azure Functions App, and Right-click your existing function app
AzureSendGridRegApp
and select Deployments.Select Connect to a GitHub repository. Follow the prompts to Log in to your GitHub account and select the repository of your existing Azure repo created to deploy to Azure directly.
Once deployed, verify the function's status through the Azure Portal.
Securing Application Settings
When deploying your email registration system, ensuring the security of sensitive information, such as your SendGrid API key, is essential. While Azure Functions provide "App keys" for function-level access control, for securing external service credentials like the SendGrid API key, it's necessary to follow best practices:
Best Practices for Managing Your SendGrid API Key:
Use Application Settings for Storing API Keys:
In the Azure Portal, navigate to your Function App and the "Configuration" section under "Settings." Here, you can store your SendGrid API key as an application setting. This approach keeps it out of your codebase and securely stores the key within the Azure infrastructure.
Accessing Your API Key in Code:
Within your Azure Function, access the SendGrid API key through the application settings using environment variables. This method prevents hard-coding of sensitive information in your function's code.
const sendGridApiKey = process.env['SENDGRID_API_KEY'];
Keep Your Keys Out of Source Control:
Ensure that any
local.settings.json
files or other configuration files containing sensitive information are added to your.gitignore
file to prevent them from being pushed to source control.Regular Key Rotation:
Update and rotate your SendGrid API key through the SendGrid dashboard, and reflect these changes in the Azure Function App's application settings to minimize the risk of unauthorized access.
Sticking to these practices can enhance the security of your email registration system by securely managing and accessing your SendGrid API key, ensuring that sensitive information remains protected.
Storing User Information Securely with Azure Table Storage
Safeguarding user information requires careful attention and efficiency regarding user data storage. Azure offers a solution that meets these requirements and simplifies the process: Azure Table Storage. This part of our journey focuses exclusively on leveraging Azure Table Storage for our email registration system, ensuring a blend of functionality, security, and ease of use.
Why Choose Azure Table Storage?
Azure Table Storage is an exceptional service for storing extensive quantities of structured, non-relational data. It offers simplicity, cost-effectiveness, high availability, and scalability. It is an ideal choice for applications requiring straightforward, durable storage without the need for complex database operations. It provides fast and easy access to data, making it perfect for securely and efficiently storing user registration information.
Setting Up Azure Table Storage
Configuring Azure Table Storage for your project involves a few straightforward steps:
Creating a Storage Account:
Navigate to the Azure Portal.
Click on "Create a resource" and select "Storage account."
Fill in the necessary details (subscription, resource group, storage account name, and location).
Navigate to the advanced section and click on: Allow enabling anonymous access on individual containers.
Review and create your storage account.
Take note of your storage account name
Accessing and Configuring Table Storage:
Once the storage account is created, navigate to it within the Azure Portal.
Find the "Tables service" section under data storage and create a new table to store your user information. Name the table according to your application, but we are going to name ours
EventRegistration
Integrating Azure Table Storage into Your Node.js Project
Since you've created the EventRegistration table in Azure Table Storage, it's time to modify your code to interact with this table to store and retrieve event registration details. The Azure Data Tables client library simplifies this process in a Node.js environment. Ensure the @azure/data-tables
package is installed in your project:
npm install @azure/data-tables
Next, integrate Azure Table Storage operations into your existing code base.
Import the Azure Data Tables Client Library:
At the beginning of your file, add the following import statement:
const { TableClient, AzureNamedKeyCredential } = require("@azure/data-tables");
Configure the Table Client:
Set up the
TableClient
to connect to yourEventRegistration
Table, using environment variables to store your Azure Storage account name and key:const tableName = "EventRegistration"; const account = process.env.AZURE_STORAGE_ACCOUNT_NAME; const accountKey = process.env.AZURE_STORAGE_ACCOUNT_KEY; const credential = new AzureNamedKeyCredential(account, accountKey); const tableClient = new TableClient(`https://${account}.table.core.windows.net`, tableName, credential);
Insert Registration Details into Azure Table Storage:
After validating the registration details, insert them into the table before sending the confirmation email:
async function insertRegistrationDetails(details) { const entity = { partitionKey: "Registration", rowKey: `${details.email}`, name: details.name, email: details.email, organization: details.organization, position: details.position, sessions: JSON.stringify(details.sessions) }; await tableClient.createEntity(entity); console.log(`Registration details for ${details.email} inserted into Table Storage.`); }
Call the
insertRegistrationDetails
Function:In our function, call
insertRegistrationDetails
After validating the registration details and before sending the confirmation email:// After validating registration details await insertRegistrationDetails(registrationDetails); // Then send registration confirmation email await sendRegistrationEmail(registrationDetails);
This integration allows you to store user registration details securely in Azure Table Storage, leveraging the scalability and efficiency of Azure's cloud services. Ensure that exceptions and errors are handled gracefully, providing a smooth experience for your users.
You can find the complete code of the entire application in this GitHub Repo link: AzureSendGridApp
Final Testing: Validating the Email Registration System
The next critical step is to conduct final testing after deploying our email registration system to Azure. Our focus is to ensure the system operates flawlessly in the live environment, providing a seamless experience for users from the moment they register.
Simplified Approach for Effective Validation
Our testing strategy at this stage is concentrated on verifying the core functionality—specifically, the registration process and the email delivery mechanism. This approach ensures that the integration with SendGrid and the Azure Functions environment performs as expected under real-world conditions.
Testing Email Functionality in Production:
Simulating User Registrations: Use a tool like Postman to send registration requests to the deployed Azure Function. These simulated requests should mirror actual user input to test the system comprehensively. This step is crucial for verifying that the system correctly processes registrations and triggers the email-sending functionality.
Verifying Email Delivery: The primary goal is to ensure that upon successful registration, the system sends out confirmation emails to users without a hitch, and the registration details are saved automatically to the Azure storage account. Check the inbox of the test accounts to confirm that emails are not only sent but are correctly formatted and contain the appropriate registration information with your Azure storage account via the Azure portal if the details are saved.
Reviewing Function Logs for Errors: Inspect the logs generated by the Azure Function during the test to ensure no unexpected errors or issues. Any log entries indicating successful execution or potential errors with the SendGrid API will be especially pertinent for identifying areas requiring immediate attention.
This final round of testing is instrumental in affirming the system's readiness for user engagement. We can efficiently validate the system's performance and reliability by focusing solely on the critical components of the registration and email notification process.
Conclusion
As we bring our series to a close, it's essential to reflect on the journey we've embarked upon, from the initial exploration of serverless architecture and Azure Functions to the complex deployment and optimization of our automated email registration system. This series has not only illuminated the transformative potential of combining Azure Functions with SendGrid for email automation but also offered a practical blueprint for navigating the complexities of modern software development.
In Part 1, we explore serverless computing, uncovering the foundational elements that underpin Azure Functions. We delved into SendGrid's role in email automation, setting the stage for an innovative approach to handling email registrations. This part was crucial in establishing a solid understanding of the technologies and preparing the ground for practical application.
Part 2 transitioned from theory to action, guiding us through the development of the registration logic within Azure Functions. Through a case study focused on a tech conference, we demonstrated the system's versatility and impact in managing workshop registrations, showcasing the seamless integration with SendGrid for personalized email communication. This phase was instrumental in bridging the gap between conceptual understanding and practical implementation, emphasizing the importance of local testing and debugging to refine our system.
The Final part of our project—deploying to Azure, securing application settings, managing user information with Azure Table Storage, and thoroughly validating our system—represents the culmination of our efforts to create a robust, scalable, and secure email registration system. These steps ensure that our system is not merely a theoretical construct but a practical, deployable solution ready to meet the demands of real-world applications.
Throughout this journey, several key learnings have stood out:
Early Deployment and Testing: Deploying our system early in the development process allowed us to address real-world challenges head-on, making securing and scaling our application more informed and effective.
Security as a Priority: By incorporating secure coding practices, validating input data rigorously, and managing sensitive information carefully, we've laid a strong foundation for maintaining the integrity and trustworthiness of our system.
Scalability through Asynchronous Processing: Adopting asynchronous processing and non-blocking operations is important to ensure our system can handle a significant influx of user registrations without compromising performance or user experience.
Simplicity and Efficiency with Azure Table Storage: Our choice to store user information using Azure Table Storage reaffirms that simplicity and efficiency do not have to come at the expense of functionality and security. This approach has enabled us to manage data effectively, keeping our system agile and responsive.
As we conclude this series, it is clear that our technology and strategies are not merely tools but enablers of innovation and excellence in software development. The automated email registration system proves what is achievable when cutting-edge technology meets strategic thinking and a user-focused design.
The insights from this series equip us to navigate future projects confidently, inspiring us to continue pushing the boundaries of what's possible. As we close this chapter, let's carry forward the lessons learned, ready to tackle new challenges and seize opportunities for innovation in software development.
Subscribe to my newsletter
Read articles from lokosman directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
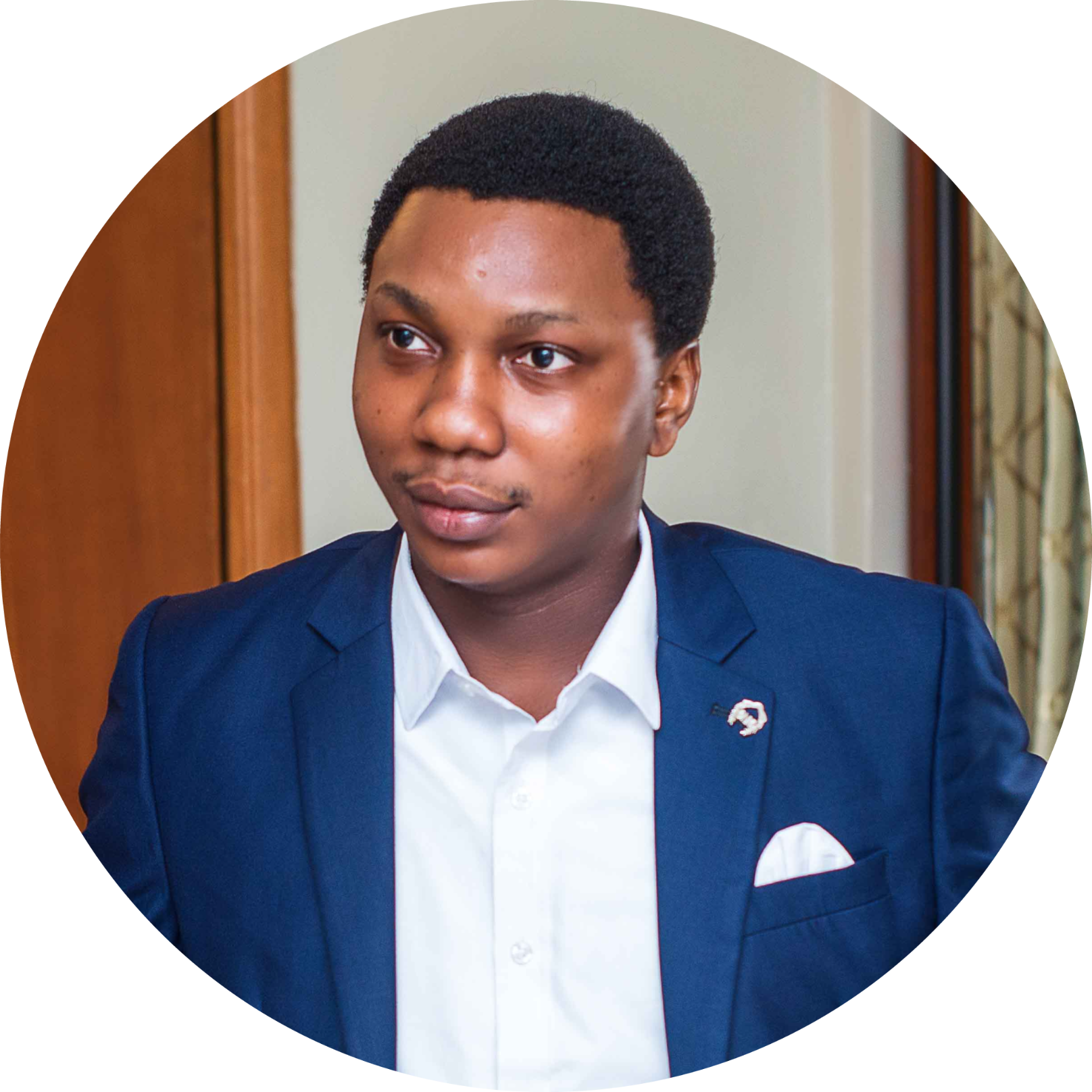
lokosman
lokosman
👋 Greetings, tech lovers! My expertise lies in Java backend engineering, focusing on refining microservices architecture. Web development, for me, is an endless expedition of discovery 🌌. When I share insights through my writings ✍️, it's a beacon of my passion. Each piece is a guiding lighthouse 🗼, hoping to enlighten your path and inspire your tech journey.