Entity Extraction from Text with Python
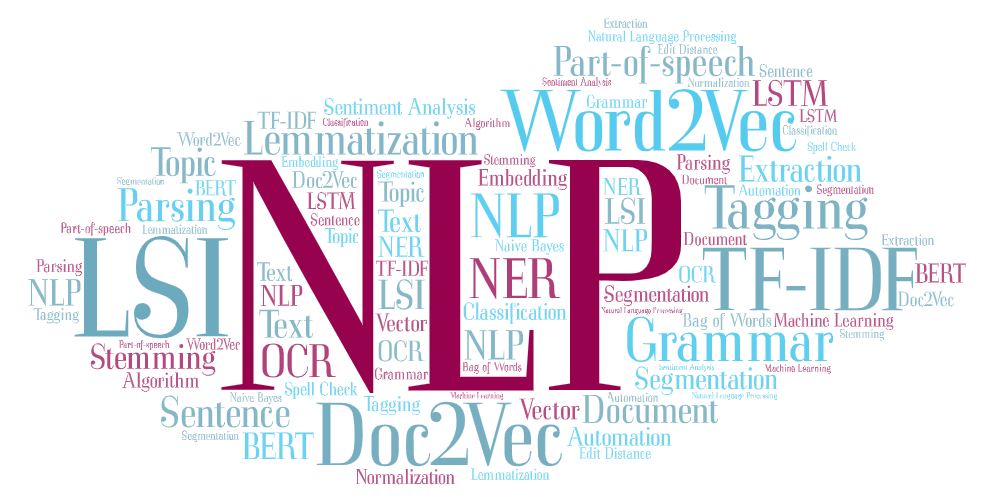
Introduction: Entity extraction, also known as named entity recognition (NER), is a vital task in natural language processing (NLP) that involves identifying and categorizing named entities such as persons, organizations, locations, dates, and more within text data. In this tutorial, we'll delve into various techniques and Python libraries for efficient entity extraction.
Understanding Entity Extraction: Named entities refer to specific elements in text that have a unique identity, such as names of people, places, companies, dates, and monetary values. Entity extraction aims to automatically detect and classify these entities, providing valuable insights for text analysis, information retrieval, and data mining tasks.
Python Libraries for Entity Extraction: Python offers powerful libraries and tools for implementing entity extraction techniques:
NLTK (Natural Language Toolkit): NLTK provides functionalities for tokenization, part-of-speech tagging, and named entity recognition using machine learning models like CRFs (Conditional Random Fields) and MaxEnt (Maximum Entropy).
spaCy: spaCy is a popular NLP library that offers fast and accurate entity recognition capabilities, including pre-trained models for various languages and domains.
Stanford NER: The Stanford NER (Named Entity Recognizer) is a Java-based tool that can be integrated with Python using libraries like
nltk_contrib
.Hugging Face Transformers: Transformers library offers state-of-the-art pre-trained models like BERT, RoBERTa, and GPT-3, which can be fine-tuned for entity extraction tasks.
Techniques for Entity Extraction:
Rule-based Approaches: Define patterns and rules using regular expressions or linguistic rules to extract entities based on specific patterns or contexts.
Machine Learning Models: Train supervised machine learning models such as CRFs or deep learning models like BERT for automatic entity recognition from text data.
Dictionary-based Methods: Utilize dictionaries or knowledge bases containing entity names and categories to match and extract entities from text.
Hybrid Approaches: Combine rule-based methods with machine learning models or ensemble different models for improved accuracy and coverage.
let's dive deeper into entity extraction and provide a few examples using different techniques and Python libraries.
1. Rule-Based Entity Extraction with Regular Expressions: Rule-based entity extraction involves defining patterns using regular expressions to identify entities based on specific formats or contexts. For example, let's consider extracting email addresses from a text:
pythonCopy codeimport re # Sample text containing email addresses text = "Contact us at support@example.com or info@company.com for inquiries." # Define a regular expression pattern for email addresses email_pattern = r"\b[A-Za-z0-9._%+-]+@[A-Za-z0-9.-]+\.[A-Z|a-z]{2,}\b" # Extract email addresses using the regular expression pattern email_addresses = re.findall(email_pattern, text) # Print extracted email addresses print("Extracted Email Addresses:") for email in email_addresses: print(email)
In this example, we use a regular expression pattern (
r"\b[A-Za-z0-9._%+-]+@[A-Za-z0-9.-]+\.[A-Z|a-z]{2,}\b"
) to match email addresses in the text.2. Named Entity Recognition (NER) with spaCy: Named Entity Recognition (NER) is a machine learning-based approach to entity extraction, where pre-trained models are used to identify and classify entities in text. Let's extract named entities from a news article using spaCy:
pythonCopy codeimport spacy # Load spaCy's English NER model nlp = spacy.load("en_core_web_sm") # Sample news article text text = """ Google announced plans to invest $1 billion in renewable energy projects. The CEO, Sundar Pichai, stated that sustainability is a top priority for the company. """ # Process the text with spaCy's NER model doc = nlp(text) # Extract named entities entities = [(entity.text, entity.label_) for entity in doc.ents] # Print extracted entities and their labels print("Extracted Entities:") for entity, label in entities: print("Entity:", entity) print("Label:", label)
In this example, spaCy's pre-trained NER model identifies and categorizes entities such as organizations (Google), monetary values ($1 billion), persons (Sundar Pichai), and other entities mentioned in the text.
3. Custom Entity Extraction with Dictionary-Based Approach: A dictionary-based approach involves using predefined dictionaries or knowledge bases to match and extract specific entities from text. Let's extract programming languages mentioned in a blog post using a custom dictionary:
pythonCopy code# Sample text containing programming languages text = "The blog post discusses Python, Java, and JavaScript programming languages." # Define a dictionary of programming languages programming_languages = ["Python", "Java", "JavaScript"] # Extract programming languages using the dictionary approach extracted_languages = [lang for lang in programming_languages if lang in text] # Print extracted programming languages print("Extracted Programming Languages:", extracted_languages)
In this example, we manually define a dictionary of programming languages and extract those mentioned in the text.
These examples illustrate different approaches to entity extraction using Python, including rule-based methods, machine learning-based NER with spaCy, and custom dictionary-based approaches. Depending on the task and context, developers can choose the most suitable technique for their entity extraction needs.
Conclusion: Entity extraction is a crucial task in NLP, enabling automated analysis and understanding of textual data. Python's versatile libraries like spaCy, NLTK, and Hugging Face Transformers offer efficient solutions for entity extraction, from rule-based methods to state-of-the-art machine learning models. By mastering entity extraction techniques and leveraging Python's capabilities, developers can enhance text processing applications, extract valuable information, and gain deeper insights from textual data sources.
Subscribe to my newsletter
Read articles from Prakhar Kumar directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by