Nullish Coalescing operator
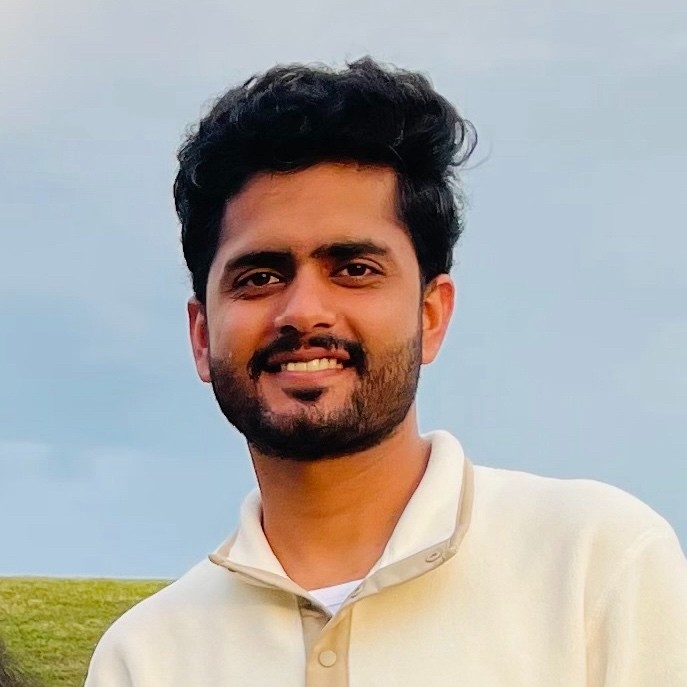
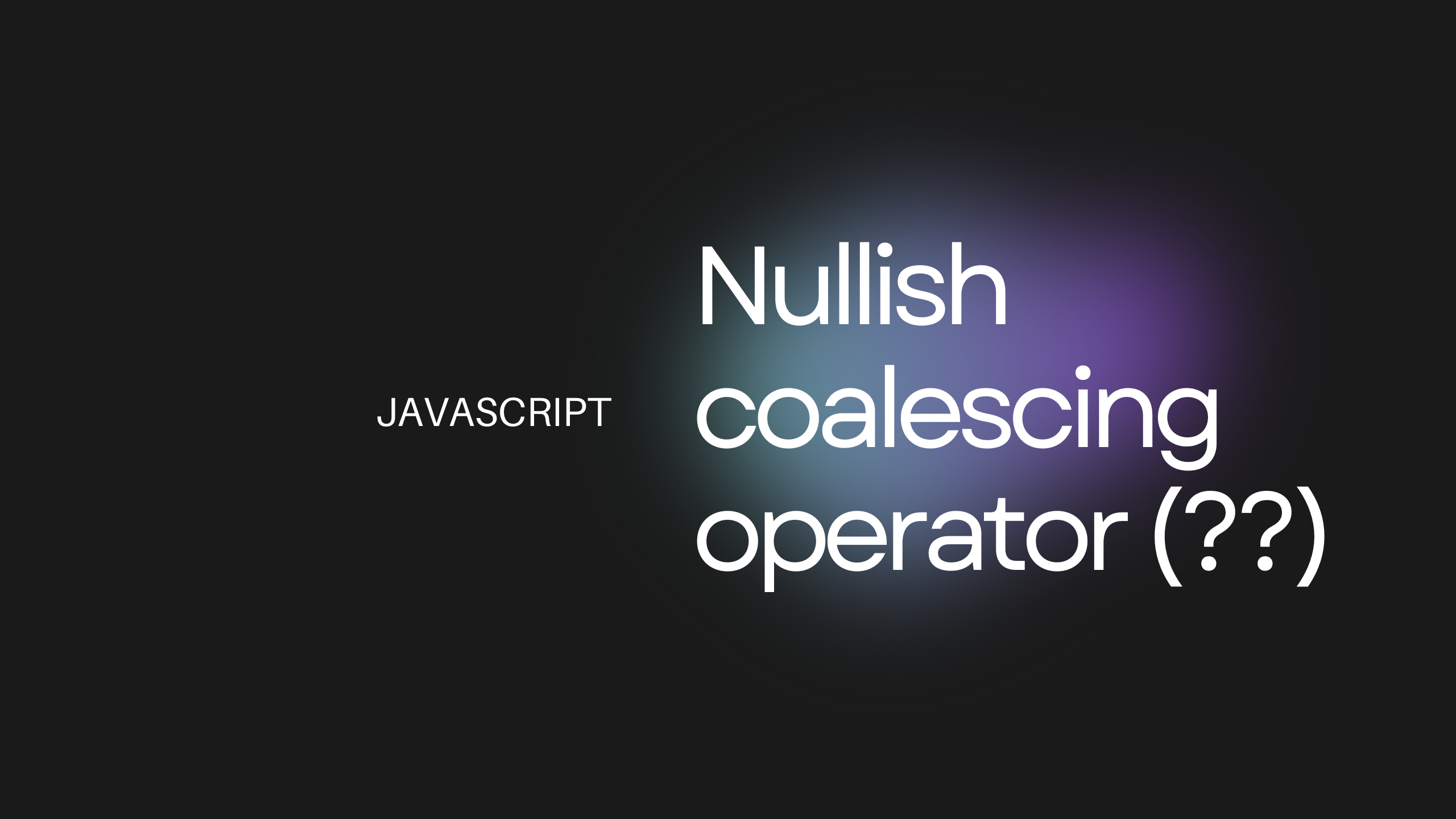
Understanding the Nullish Coalescing Operator in JavaScript
JavaScript is a flexible programming language that adds new capabilities every iteration (ECMAScript 2020 and up) to improve functionality and write more legible, concise code. The Nullish Coalescing Operator (??) is one such feature. We will go into the specifics of the Nullish Coalescing Operator, its function, and how it varies from other logical operators in this blog post.
What is the Nullish Coalescing Operator?
The Nullish Coalescing Operator (??) is a logical operator introduced in ECMAScript 2020. Its purpose is to handle default values for variables that may be null or undefined. Unlike the logical OR operator (||), the Nullish Coalescing Operator specifically checks for null or undefined values and assigns a default value only in those cases. It is designed to be more precise in scenarios where only explicit nullish values should trigger default assignments. The operator contributes to code conciseness and clarity when working with optional or potentially absent values.
Consider the following scenario:
// Using the || operator for default value
const result = b || 'Default';
In the example above, if b is falsy (e.g., null, undefined, false, 0, or an empty string), the right-hand side of the || operator (‘Default’) is assigned to the result. However, this approach has a drawback when b is a falsy value, but not necessarily null or undefined.
This is where the Nullish Coalescing Operator comes into play:
// Using the ?? operator for default value
const result = b ?? 'Default';
With the ?? operator, the right-hand side is only assigned to the result if b is null or undefined. Falsy values like 0 or an empty string won’t trigger the default assignment.
Key Characteristics of the Nullish Coalescing Operator:
- Specifically for Nullish Values:
The ?? operator checks for null or undefined values, and only in those cases does it assign the default value.
2. Difference from || Operator:
While the || operator checks for any falsy value, the ?? operator specifically checks for null or undefined, making it more precise in certain scenarios.
3. Common Use Cases:
The Nullish Coalescing Operator is commonly used when assigning default values to function parameters or when dealing with configuration options that might be null or undefined.
A useful feature to JavaScript is the Nullish Coalescing Operator (?? ), which offers a clear and methodical way to manage default values for variables that might be null or undefined. Cleaner and more predictable code results from its use, particularly in situations when false values other than null or undefined are not meant to cause default assignments.
In order to improve the quality and maintainability of their code, developers should keep up with the latest developments in JavaScript, including new features and recommended practices. When dealing with default values in a contemporary JavaScript codebase, the Nullish Coalescing Operator is a useful tool to have in your toolbox.
Note:
The Nullish Coalescing Operator (??) in JavaScript is used to provide a default value only when the variable is null or undefined, excluding other falsy values like 0 or empty strings.
Subscribe to my newsletter
Read articles from Vinay Chhabra directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
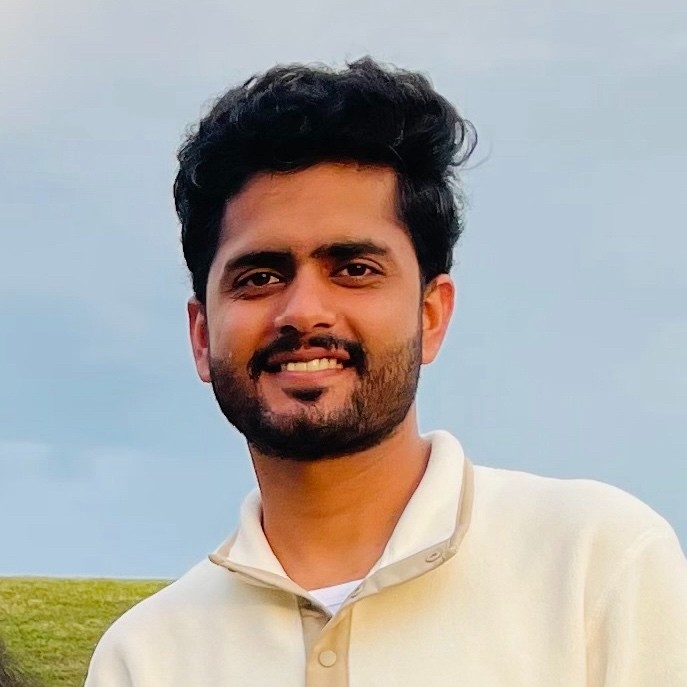
Vinay Chhabra
Vinay Chhabra
I'm Vinay Chhabra, a Full-Stack Developer and Software Engineer specializing in React.js, Node.js, and Magento 2. On Hashnode, I share insights on tech trends, development strategies, and e-commerce solutions.