Resource utilization check using python Fabric
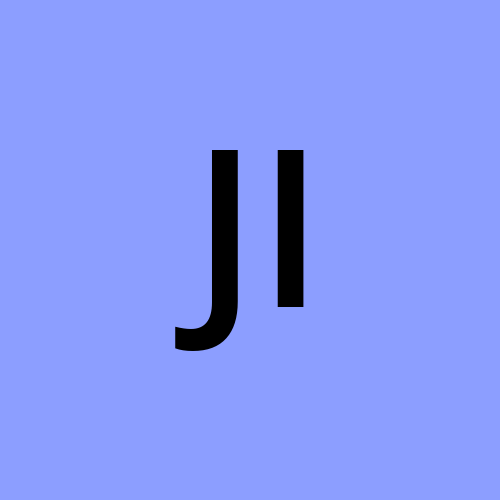
2 min read
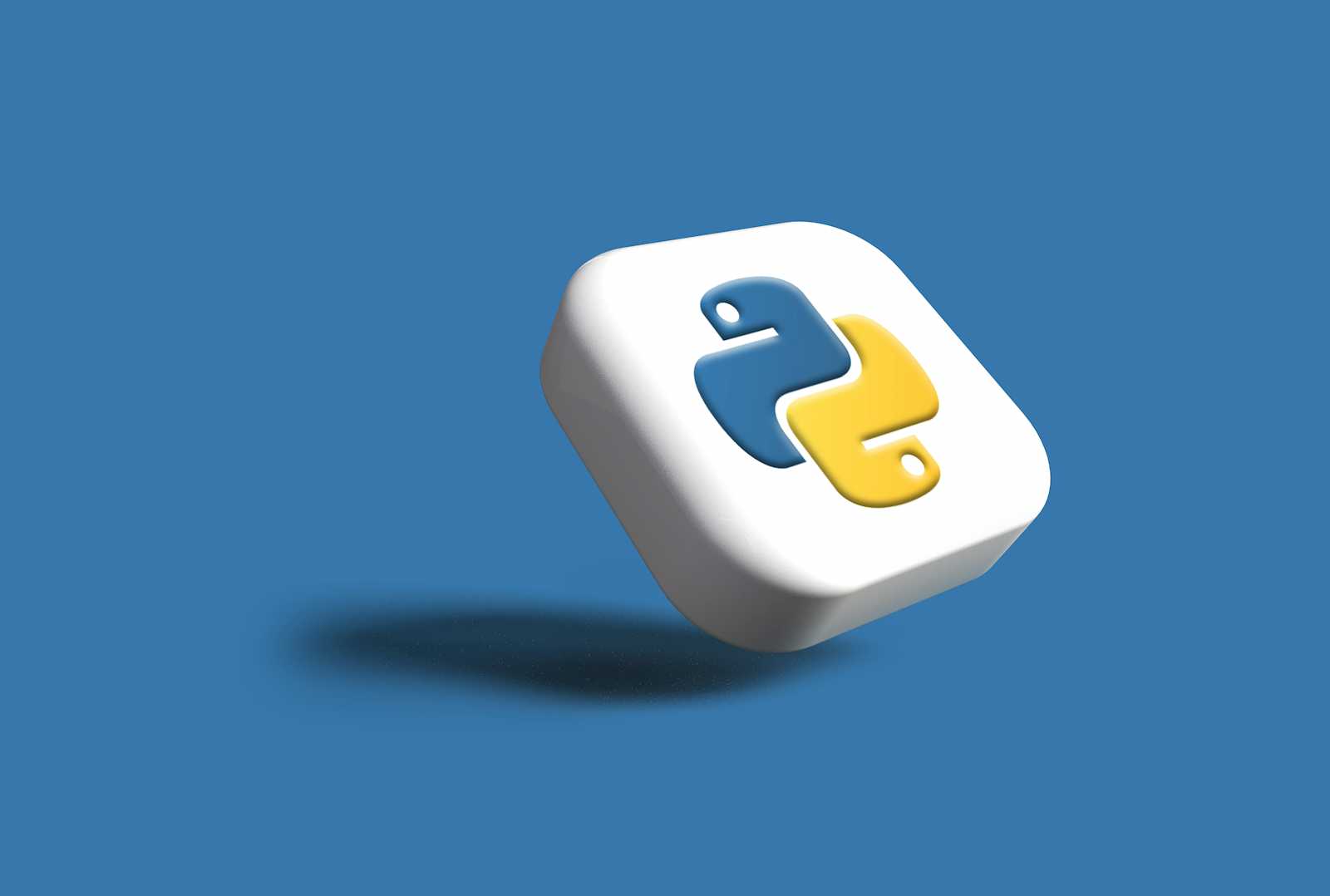
Python Fabric module can be used for checking the resource utilization in a server remotely.
# Import Modules
import argparse
from fabric import Connection
# Define user inputs
parser = argparse.ArgumentParser()
parser.add_argument("--hostname", required=True, help="Server IP or IP Address")
parser.add_argument("--username", required=True, help="Username")
parser.add_argument("--keyfile", required=True, help="Keyfile")
parser.add_argument("--option", required=True, type=int, choices=[1, 2, 3], help="[1] CPU [2] Memory [3] Disk")
args = parser.parse_args()
# Create a session
session = Connection(
host = args.hostname,
user = args.username,
connect_kwargs={
"key_filename": args.keyfile
},
connect_timeout=30,
)
# Define functions
def cpu_check(c):
command = "echo '-----CPU Load-----'; w"
return c.run(command, hide=True).stdout.strip()
def memory_check(c):
command = "echo '-----Memory Usage-----'; free -m"
return c.run(command, hide=True).stdout.strip()
def disk_check(c):
command = "echo '-----Disk Usage-----'; df -h"
return c.run(command, hide=True).stdout.strip()
# Get the operating system/kernel
uname = session.run('uname -s', hide=True)
# Execute functions based on the input option
if 'Linux' in uname.stdout:
if args.option == 1:
print(cpu_check(session))
elif args.option == 2:
print(memory_check(session))
elif args.option == 3:
print(disk_check(session))
else:
print("Please enter a valid option")
else:
print(f"Unable to get the details as the server has {uname} operating system!")
Inputs to the script:
Hostname : Server IP address or Hostname
Login username
SSH key file
Option (1. CPU, 2. Memory, 3. Disk)
$ python python-ssh-fabric.py --hostname <IP Address> --username ubuntu --keyfile <SSH key file> --option 1
-----CPU Load-----
18:43:06 up 10:48, 0 users, load average: 0.00, 0.00, 0.00
$ python python-ssh-fabric.py --hostname <IP Address> --username ubuntu --keyfile <SSH key file> --option 2
-----Memory usage-----
total used free shared buff/cache available
Mem: 949 172 265 0 511 620
Swap: 0 0 0
$ python python-ssh-fabric.py --hostname <IP Address> --username ubuntu --keyfile <SSH key file> --option 3
-----Disk usage-----
Filesystem Size Used Avail Use% Mounted on
/dev/root 7.6G 1.7G 6.0G 22% /
tmpfs 475M 0 475M 0% /dev/shm
tmpfs 190M 832K 190M 1% /run
tmpfs 5.0M 0 5.0M 0% /run/lock
/dev/xvda15 105M 6.1M 99M 6% /boot/efi
tmpfs 95M 4.0K 95M 1% /run/user/1000
Reference:
https://docs.fabfile.org/en/latest/api/connection.html#connect-kwargs-arg
https://docs.fabfile.org/en/latest/concepts/authentication.html
0
Subscribe to my newsletter
Read articles from Jithesh directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
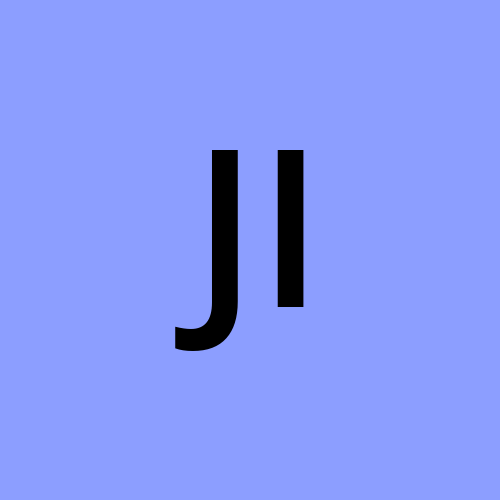