Array ? Arrays ? In Java
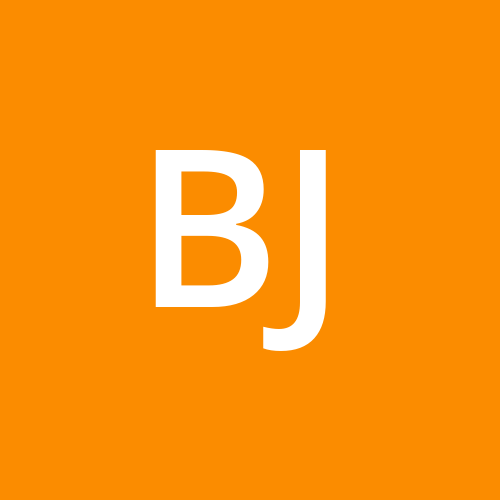
Foreword
# I am not a professional developer, which means it's for my logging point to look back at myself.
As I delve deeper into the world of Java Programming, I find myslelf repeately encountering arrays in various forms that got me confused. So, I've come to realize that my understanding of arrays is somewhat lacking, and arrays seem indispensable. What's more, I've noticed gaps in my knowledge, especially when it comes to choosing between arrays and ArrayList. By outlining these concepts, I aim to understand a simple concept like clone and more complex concept like Time Complexity. I hope to not only enhance my skills but also inspire someone who may be on a similar path. Let this document be a testament to my long-term jouney as A Backend Developer and first step.
#Method to sort Array after Cloning Array
package org.example;
import java.util.Arrays;
public class SortingArrayAfterCloning {
public static void main(String[] args) {
int[] arr = {1,5,3,2,7};
int[] sorted = solution(arr);
System.out.println("arr = " + Arrays.toString(arr));
// Prints the Original Array => arr = [1, 5, 3, 2, 7]
System.out.println("sorted = " + Arrays.toString(sorted));
// Prints the Sorted Array => sorted = [1, 2, 3, 5, 7]
}
private static int[] solution(int[] arr) {
int[] clone = arr.clone(); // Cloning the original Array
Arrays.sort(clone); // Sorting the Cloned Array
return clone;
}
}
Explanation: The 'solution' method clones the original Array before sorting it,
This ensures that the original array remains unchanged when printed directly.
so we can print the Sorted Array seperately.
#Method to sort Array without Cloning Array
import java.util.Arrays;
public class SortingArrayWithoutCloning {
public static void main(String[] args) {
int[] arr = {1,5,3,2,7};
int[] sorted = solution(arr);
System.out.println("arr = " + Arrays.toString(arr));
// Prints the Sorted Array => arr = [1, 2, 3, 5, 7]
System.out.println("sorted = " + Arrays.toString(sorted));
// Prints the Sorted Array => sorted = [1, 2, 3, 5, 7]
}
private static int[] solution(int[] arr) {
int[] clone = arr; // Pointing clone to the original array
Arrays.sort(arr); // sorting the original Array
return clone;
}
}
Explanation: The 'solution' method sorts the original array directly
without cloning it first, which means the original array is modified.
This results in the original array being modified, and thus, both the
original and sorted arrays are identical when printed.
(Cloning the original array in the solution method can lead to
them being different.)
#Printing Array Object as it is
: Referring to the Array Object directly leads to printing out the memory address of Array, resulting in output like arr = [I@776ec8df
import java.util.Arrays;
public class ArrayProblem2 {
public static void main(String[] args) {
int[] arr = {1,5,3,2,7};
int[] sorted = solution(arr);
System.out.println("arr = " + arr);// arr = [I@776ec8df
System.out.println("sorted = " + sorted);// arr = [I@776ec8df
}
private static int[] solution(int[] arr) {
int[] clone = arr;
Arrays.sort(arr);
return clone;
}
}
# Neither of these codes can print the specific values of the array,
because they refer to the memory address of Array Object.
# To print the specific values of the array,
You need to use Arrays.toString();
#Arrays.copyOf(); , arr.clone();
import java.util.Arrays;
public class ArrayCopyOfExample {
public static void main(String[] args) {
int[] originalArray = {1,2,3,4,5};
// Create a copy of originalArray with the same length
int[] copiedArrayWithArraylength =
Arrays.copyOf(originalArray, originalArray.length);
// Create a shallow copy of originalArray using ".clone();"
int[] clonedArray = originalArray.clone();
// Create a copy of originalArray with a customized length with 3
int[] copiedArrayWithDifferentLength = Arrays.copyOf(originalArray, 3);
System.out.println("copiedArrayWithArraylength = "
+ Arrays.toString(copiedArrayWithArraylength));
System.out.println("clonedArray = " + Arrays.toString(clonedArray));
System.out.println("copiedArrayWithDifferentLength = "
+ Arrays.toString(copiedArrayWithDifferentLength));
}
}
# Arrays.copyOf(); and Array.clone(); have something in common,
and they are a shallow copy. But, Technically, They are different.
As you see above code snipets, the outstanding difference is that
"Arrays.copyOf();" can customize the length of arrays and return
new array with the length we set.
#One Demensional Array (normal)
public class ArrayPractice {
public static void main(String[] args) {
//Below code can be seen as different, but identical.
int[] arr = {2, 8, 7, 6, 0}; // array that has length 5.
int[] arr2 = new int[5]; // array that has length 5,
// both have the same length.
}
}
( when Array is Initialized in Java (the initial value sets as 0 automatically))
#Two Dimensional Array
public static void main(String[] args) {
int[][] arr = new int[3][3];
System.out.println("arr[0][0] = " + arr[0][0]); // 0
System.out.println("arr[0][1] = " + arr[0][1]); // 0
System.out.println("arr[2][2] = " + arr[2][2]); // 0
/* The value of an array is initialized as 0 without initializing
the particular value (int - primitive datatype)*/
arr[2][2] = 6; // change the value of arr[2][2] from 0 to 6
System.out.println("arr[2][2] = " + arr[2][2]); // 6
}
#arr[2][2] _ Size of Array
#Reference Point to use Array or ArrayList (Time Complexity)
: An array is a fundamental data structure where each value corresponds to an index. This means that accessing data in an array involves directly accessing it via its index number.
Tip 1 - ArrayList vs Array:
when you are not sure the size -> ArrayList, if not -> Array
When the size of the data structure needs to be dynamic and may change over time, it's advisable to use ArrayList. Unlike arrays, ArrayLists can dynamically adjust their size.
Tip 2 - Time Complexity for Removing Data in Array:
When removing data from an array, particularly from the middle or other indices, the time complexity can increase from O(1) to O(N). This is because removing elements can result in shifting elements to fill the gap, which operates N times.
+
public static void main(String[] args) {
int[] answer = {1, 2, 3, 4, 5};
System.out.println("answer[0] = " + answer[0]);
System.out.println("answer = " + answer);
}
Subscribe to my newsletter
Read articles from Byung Joo Jeong directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
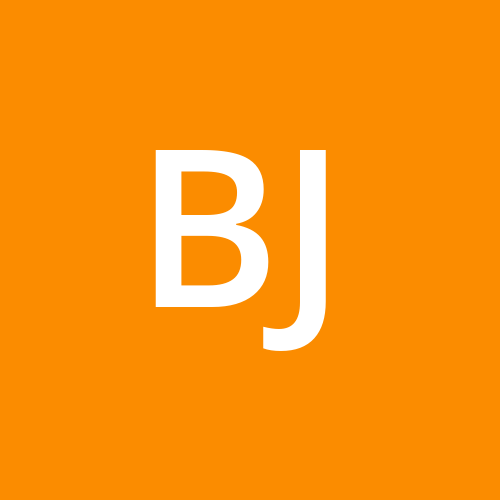