SAAS service Resend to send emails whenever a new form is created.
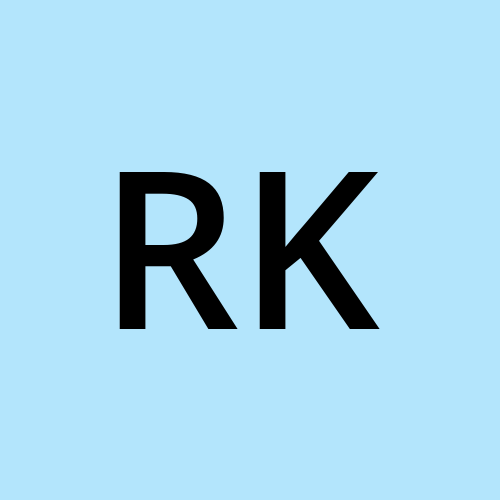
Overview
Portfolio website for a corporation which provides a contact us form along with the basic information about the company.
Technology Used:-
React, Node, Typescript, Tailwind CSS
Let's Quick Setup The Project
React:-
Use the following commands to setup the React Project
npm create vite@latest my-vite-app - - template react-ts
npm install -D tailwindcss postcss autoprefixer
npx tailwindcss init -p
Install some basic react library like react-router-dom and react-hook-form.
Project Structure
Comming to important feature designing of contact.tsx page.
Contact.tsx page
import { useForm } from "react-hook-form";
import axios from "axios";
type FormValues = {
name: string;
email: string;
companyName: string;
feedback: string;
}
const Contact = () => {
const {
register,
handleSubmit,
formState: { errors },
reset,
} = useForm<FormValues>();
const submitHandler = async (data: FormValues) => {
try {
await axios.post("http://localhost:8001/send", {
from: "onboarding@resend.dev",
to: data.email,
subject: data.companyName,
html: data.feedback
})
alert("message is sent");
reset();
} catch (error) {
alert("message not sent");
}
};
return (
<section>
<div className="flex justify-around mt-16 py-5">
<div>
<h3 className="text-xl font-semibold">Contact us</h3>
<h1 className="text-6xl font-bold mt-5">Let's Talk!</h1>
<h4 className="text-xl text-slate-600 mt-5">We will reach out to you within 24 hours</h4>
<h4 className="text-lg text-slate-700 font-semibold mt-7">Don't like filling up forms? Email us directly at-</h4>
<div> <p className="text-slate-600 mt-1">hi@truedax.io</p></div>
</div>
<div className="">
<form onSubmit={handleSubmit(submitHandler)} className="w-[400px] max-w-md space-y-8">
<div>
<input
{...register('name',{ required: true })}
type="text"
className="w-full py-3 px-4 placeholder-gray-500 text-sm border-gray-300 rounded-md"
placeholder="Name"
/>
{errors.name && <p className="text-red-600">Name is required</p>}
</div>
<div>
<input
{...register('email',{ required: true })}
type="email"
className="w-full py-3 px-4 placeholder-gray-500 text-sm border-gray-300 rounded-md"
placeholder="Email"
/>
{errors.email && <p className="text-red-600">Please enter a valid email</p>}
</div>
<div>
<input
{...register('companyName',{ required: true })}
type="text"
className="w-full py-3 px-4 placeholder-gray-500 focus:ring-indigo-500 focus:border-indigo-500 text-sm border-gray-300 rounded-md"
placeholder="Company Name"
/>
{errors.companyName && <p className="text-red-600">Company name is required</p>}
</div>
<div>
<input
{...register('feedback',{ required: true })}
type="text"
className="w-full py-3 px-4 placeholder-gray-500 focus:ring-indigo-500 focus:border-indigo-500 text-sm border-gray-300 rounded-md"
placeholder="How can we help you? Feel free to get in touch!"
/>
{errors.feedback && <p className="text-red-600">Feedback is required</p>}
</div>
<div>
<button
type="submit"
className="group w-1/2 flex justify-center py-2 px-4 border border-transparent text-xl font-medium rounded-full text-white bg-green-600 hover:bg-green-900 focus:outline-none focus:ring-2 focus:ring-offset-2 focus:ring-green-500"
>
Submit
</button>
</div>
</form>
</div>
</div>
</section>
);
};
export default Contact;
Setting up the backend Server form resend.
Create a backend server using the following command.
yarn init
yarn add typescript -D
yarn add tsc-watch -D
yarn add express
yarn add resend
Make sure that you create a api Key from resend to setup the backend.
server.ts file
import express from 'express';
import bodyParser from 'body-parser';
import { Resend } from 'resend';
import cors from 'cors';
const resend = new Resend('RESEND API KEY ENTER HERE');
const app = express();
app.use(cors());
app.use(bodyParser.json());
app.post("/send", async (req, res) => {
const { from, to, subject, html } = req.body;
try {
const { data, error } = await resend.emails.send({ from, to, subject, html });
console.log(data);
if (error) {
console.error('Error sending email:', error);
res.status(500).json({ error: 'Error sending email' });
return;
}
res.status(200).json({ message: 'Email sent successfully', data });
} catch (error) {
console.error(error);
}
})
app.get("/", (req, res) => {
res.send({
name: "get is working"
})
})
app.listen(8001, () =>
console.log('Example app listening on port 8001!'),
);
To Start the server command Yarn dev
now everything is setup the project is ready
Enjoy Happy Coding
Subscribe to my newsletter
Read articles from Rahul Kumar directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
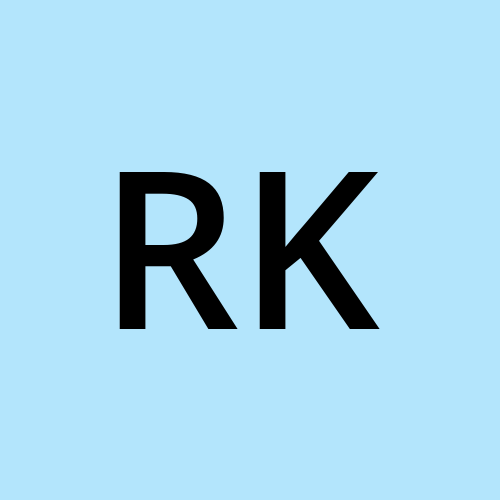