Transforming Meeting Transcripts into Actionable Insights: A Guide to Automated Minutes of Meeting Using NLP in Python (Use Case - 1)
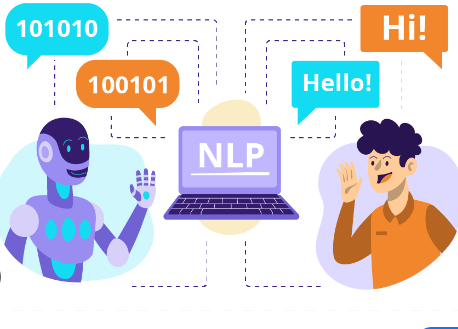
In today's fast-paced business environment, efficient communication and decision-making are paramount. Meetings play a crucial role in fostering collaboration and driving initiatives forward. However, capturing and summarizing meeting discussions accurately can be time-consuming and error-prone. This is where Natural Language Processing (NLP) and Python come to the rescue.
In this article, we'll explore how to leverage NLP techniques in Python to transform meeting transcripts into actionable insights, creating automated Minutes of Meeting (MoM) effortlessly.
Understanding the Process
Automating MoM creation involves several key steps:
Text Preprocessing: Clean and prepare the meeting transcript data.
Topic Extraction: Identify key topics discussed during the meeting.
Summarization: Generate a concise summary of the meeting discussions.
Let's dive into each step with Python code examples using popular NLP libraries like NLTK, Gensim, and spaCy.
Step 1: Text Preprocessing
The first step is to preprocess the meeting transcript text. We'll tokenize the text into sentences, remove stopwords and punctuation, and perform lemmatization for word normalization.
pythonCopy codeimport nltk
from nltk.tokenize import sent_tokenize, word_tokenize
from nltk.corpus import stopwords
from nltk.stem import WordNetLemmatizer
# Sample meeting transcript
meeting_transcript = "..."
sentences = sent_tokenize(meeting_transcript)
stop_words = set(stopwords.words('english'))
lemmatizer = WordNetLemmatizer()
processed_sentences = []
for sentence in sentences:
words = word_tokenize(sentence)
filtered_words = [lemmatizer.lemmatize(word.lower()) for word in words if word.isalnum() and word.lower() not in stop_words]
processed_sentences.append(filtered_words)
Step 2: Topic Extraction
Next, we'll use topic modeling techniques, such as Latent Dirichlet Allocation (LDA), to extract key topics from the meeting transcript.
pythonCopy codefrom gensim.models import LdaModel
from gensim import corpora
dictionary = corpora.Dictionary(processed_sentences)
corpus = [dictionary.doc2bow(text) for text in processed_sentences]
lda_model = LdaModel(corpus, num_topics=2, id2word=dictionary)
topics = lda_model.print_topics(num_words=5)
Step 3: Summarization
Finally, we'll summarize the meeting discussions using spaCy for extractive summarization.
pythonCopy codeimport spacy
nlp = spacy.load('en_core_web_sm')
doc = nlp(meeting_transcript)
summary_sentences = []
for sentence in doc.sents:
if any(word in sentence.text.lower() for word in ['review', 'challenge', 'campaign']):
summary_sentences.append(sentence.text)
Bringing It All Together
With the text preprocessing, topic extraction, and summarization steps completed, we can now create the automated Minutes of Meeting.
pythonCopy codeprint("Minutes of Meeting:")
print("Topics Discussed:")
for topic in topics:
print(topic)
print("\nSummary:")
print('\n'.join(summary_sentences))
Benefits of Automated MoM Creation
Time-Saving: Eliminate manual effort in summarizing meeting content.
Accuracy: Reduce errors and ensure accurate capture of key discussions.
Actionable Insights: Extract actionable insights and action items from meetings.
Conclusion
Automating Minutes of Meeting creation using NLP techniques in Python streamlines the process, enhances accuracy, and provides actionable insights from your meetings. By leveraging text preprocessing, topic extraction, and summarization, you can transform raw meeting transcripts into valuable assets for decision-making and collaboration.
Start implementing NLP automation in your meeting processes today and unlock the full potential of your team's discussions.
Subscribe to my newsletter
Read articles from Prakhar Kumar directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by