What are DTOs in software systems?

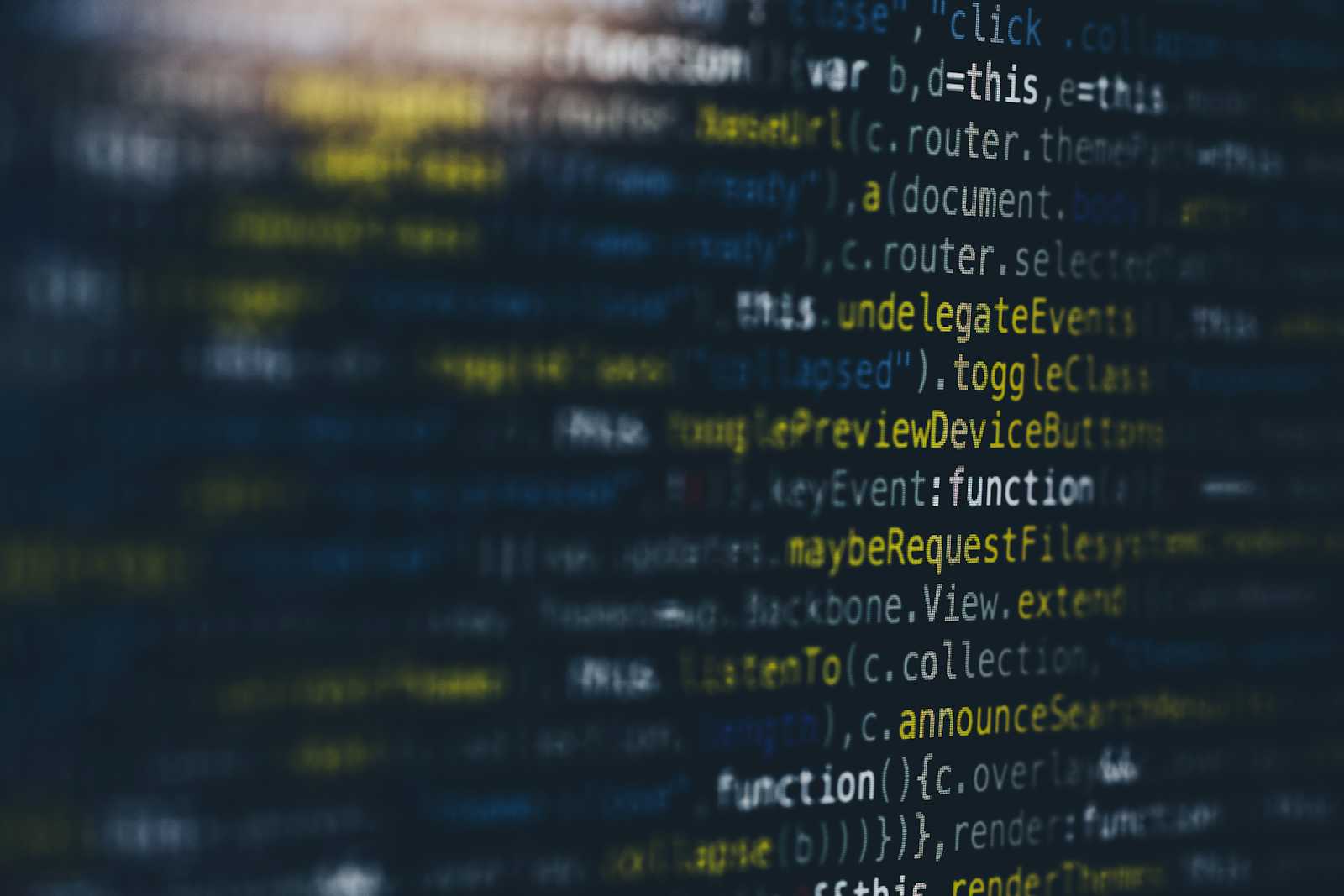
DTOs, or Data Transfer Objects, are used to move data between different parts of a software application. They are especially useful in service-oriented architectures (SOA) or microservices. The main goal of DTOs is to bundle and carry data across the system's parts while reducing dependencies and boosting efficiency.
Usually, DTOs prevent the need to expose the internal data structures of one part of the system to another. This separation makes the system easier to maintain and adapt, as changes in one part's data structures don't affect the others. In the example below, a client
sends data to service A
using DTO A
, and service A
communicates with service B
using DTO B
.
DTOs often contain only the necessary data fields without business logic. They act as a container for data that needs to be passed across different layers, such as between a client and a server or between different microservices in a distributed system.
In the subsequent sections, we will learn about some key aspects of DTOs and see an example of how to serialize a data transfer object into a JSON string in Python and deserialize the JSON string back into a data transfer object in C#.
Key characteristics of DTO
Some of the key characteristics of a DTO include the following:
Data Structure: DTOs are essentially objects that represent a set of data fields. They don't contain any business logic; their main purpose is to hold and transfer data.
No Behavior: Unlike domain objects or entities, DTOs typically do not have methods or behavior. They are focused on carrying data.
Boundary Crossing: DTOs are commonly used when data needs to be passed between layers of an application or between different components. For example, when transferring data between the frontend and backend of a web application.
Reducing Coupling: DTOs help reduce the coupling between different parts of a system. By using DTOs, changes in the data structure of one component do not directly affect the other components as long as the DTO interface remains unchanged.
Serialization: DTOs are often used in the context of serialization and deserialization. They provide a structured way to package and send data over a network or between different parts of a distributed system.
Serialization/Deserialization of DTO
As seen in the previous section, serialization and deserialization of DTOs are important so that we can maintain data contracts across different layers of the software system. In this section, we will see examples of how we can serialize a DTO in Python and then deserialize it in C#.
Python (Serialization):
Below is sample code snippet to serialize a python object.
import json
class UserDTO:
def __init__(self, username, email):
self.username = username
self.email = email
# Create an instance of the UserDTO class
user_data = UserDTO(username="JohnDoe", email="johndoe@example.com")
# Serialize the UserDTO instance to a JSON string
json_string = json.dumps(user_data.__dict__)
# Print the serialized JSON string
print(json_string)
The above code gives us a serialized JSON string output like below:-
{"username": "JohnDoe", "email": "johndoe@example.com"}
C# (Deserialization):
Below is sample code snippet to deserialize a JSON string into a C# object.
using System;
using Newtonsoft.Json;
class Program
{
static void Main()
{
// The JSON string serialized in Python
string jsonString = "{\"username\":\"JohnDoe\",\"email\":\"johndoe@example.com\"}";
// Deserialize the JSON string to a UserDTO object
UserDTO userDto = JsonConvert.DeserializeObject<UserDTO>(jsonString);
// Print the deserialized UserDTO object
Console.WriteLine($"Username: {userDto.Username}, Email: {userDto.Email}");
}
}
class UserDTO
{
public string Username { get; set; }
public string Email { get; set; }
}
The output of the deserialization in C# is below:-
Username: JohnDoe, Email: johndoe@example.com
In the above code examples:
The
UserDTO
class is defined in both Python and C# with similar structures.In Python, an instance of
UserDTO
is created and serialized to a JSON string usingjson.dumps()
.In C#, the JSON string is deserialized back into a
UserDTO
object usingJsonConvert.DeserializeObject
from theNewtonsoft.Json
library.
Subscribe to my newsletter
Read articles from Gaurav Gupta directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
