Mastering JavaScript: Higher-Order Functions
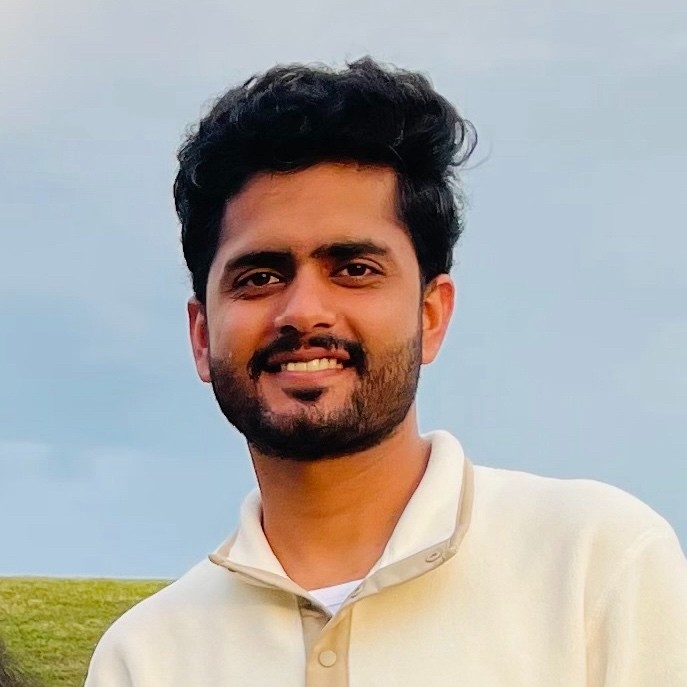
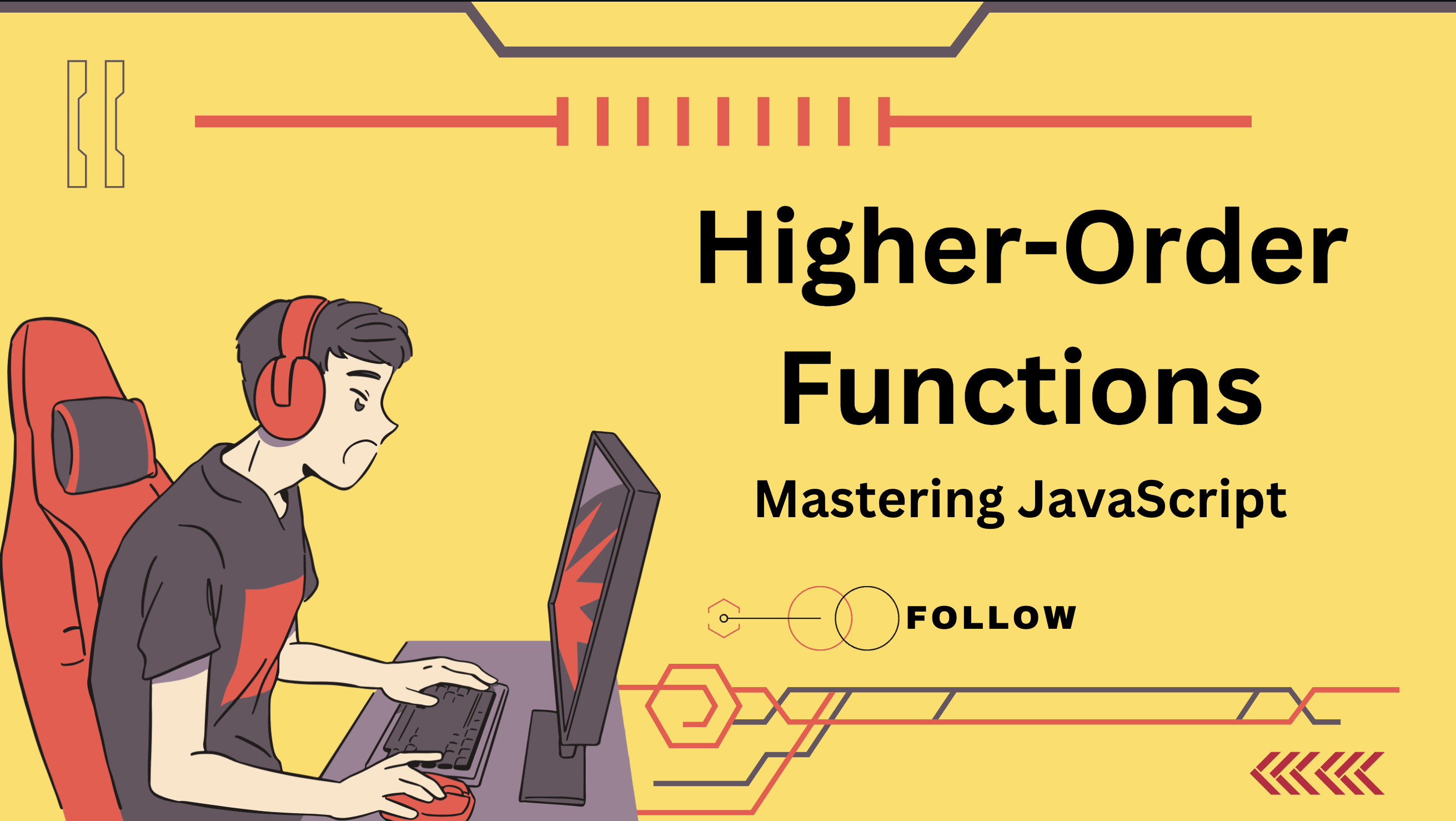
Higher-order functions in JavaScript are functions that can accept other functions as arguments or return functions as output. They enable a functional programming style, allowing developers to write concise, expressive code that is both flexible and powerful.
Exploring Higher-Order Functions: Let’s dive into the world of higher-order functions and discover the array of possibilities they offer for simplifying and enhancing your JavaScript code.
- map(): Transforming Array Elements The map() method iterates over each element of an array, applies a transformation function to each element, and returns a new array containing the transformed elements.
const numbers = [1, 2, 3, 4, 5];
const squaredNumbers = numbers.map(function(number) {
return number * number;
});
console.log(squaredNumbers); // Output: [1, 4, 9, 16, 25]
2. filter(): Selecting Relevant Data The filter() method creates a new array containing only the elements that satisfy a specified condition defined by a filtering function.
const numbers = [1, 2, 3, 4, 5];
const evenNumbers = numbers.filter(function(number) {
return number % 2 === 0;
});
console.log(evenNumbers); // Output: [2, 4]
3. forEach(): Performing Actions on Array Elements The forEach() method executes a provided function once for each array element, allowing you to perform actions on each element.
const colors = ['red', 'green', 'blue'];
colors.forEach(function(color) {
console.log(color);
});
// Output:
// red
// green
// blue
map() vs forEach()
map()
Iterates over each element of an array and applies a transformation function to each element.
Returns a new array containing the results of applying the transformation function to each element.
Does not modify the original array; it creates a new array with transformed elements.
Suitable when you need to transform each element of an array and create a new array with the transformed values.
Allows chaining with other array methods since it returns a new array.
forEach()
Iterates over each element of an array and executes a provided callback function for each element.
Does not return anything; it simply executes the provided callback function for each element.
Does not create a new array or modify the existing array; it only executes the callback function.
Useful when you want to execute a function for each element of an array but do not need to create a new array.
Cannot be chained with other array methods as it does not return anything. Typically used inside a loop or chain with other methods.
Conclusion:
Congratulations on mastering JavaScript higher-order functions! With the map(), filter(), and forEach() methods at your disposal, you now have powerful tools for transforming, filtering, and iterating over arrays with ease.
Happy Coding!!
Subscribe to my newsletter
Read articles from Vinay Chhabra directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
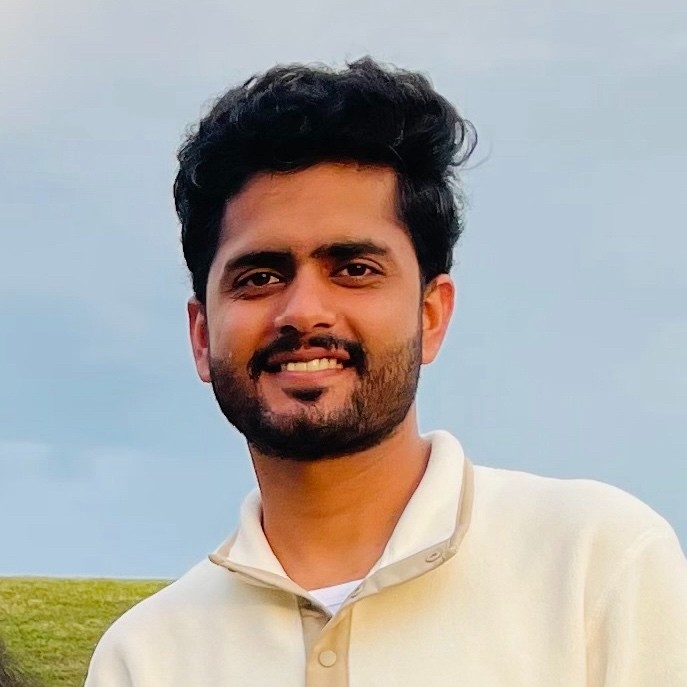
Vinay Chhabra
Vinay Chhabra
I'm Vinay Chhabra, a Full-Stack Developer and Software Engineer specializing in React.js, Node.js, and Magento 2. On Hashnode, I share insights on tech trends, development strategies, and e-commerce solutions.