Language Translation with Python NLP (Use Case - 4)
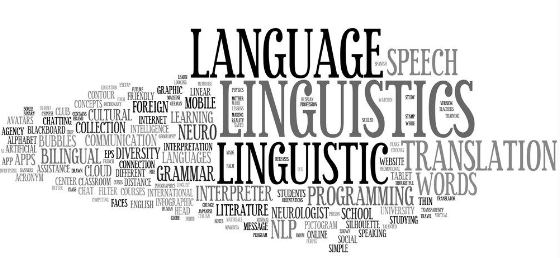
Language translation plays a vital role in breaking down communication barriers and fostering global connectivity. With advancements in Natural Language Processing (NLP) and machine learning, automated language translation has become more accurate and accessible than ever. In this blog post, we'll dive into the world of language translation using Python and NLP techniques, covering key concepts, implementation steps, and real-world examples.
Understanding Language Translation in NLP
Language translation involves converting text from one language to another while preserving meaning and context. NLP techniques enable machines to understand and translate human languages, facilitating cross-cultural communication and knowledge exchange.
Key Components of Language Translation
Tokenization: Breaking text into tokens or words for processing.
Sequence-to-Sequence Models: Models that map input sequences to output sequences, such as the Transformer model.
Encoder-Decoder Architecture: A framework where an encoder processes input text and a decoder generates translated output.
Attention Mechanism: Mechanisms that allow models to focus on relevant parts of the input text during translation.
Evaluation Metrics: Metrics like BLEU (Bilingual Evaluation Understudy) used to evaluate translation quality.
Implementing Language Translation with Python
Let's explore how to implement language translation functionalities using Python and popular NLP libraries such as Hugging Face's Transformers and spaCy.
Step 1: Install Required Libraries
Install the necessary libraries for language translation and NLP tasks.
bashCopy codepip install transformers spacy
Step 2: Preprocessing and Tokenization
Preprocess text data and tokenize it for language translation tasks.
pythonCopy codefrom transformers import MarianMTModel, MarianTokenizer
# Load MarianMT model and tokenizer
model_name = 'Helsinki-NLP/opus-mt-en-ro' # Example: English to Romanian translation
model = MarianMTModel.from_pretrained(model_name)
tokenizer = MarianTokenizer.from_pretrained(model_name)
Step 3: Translate Text
Translate text from one language to another using the loaded model and tokenizer.
pythonCopy codedef translate_text(text, model, tokenizer):
inputs = tokenizer(text, return_tensors='pt')
outputs = model.generate(**inputs)
translated_text = tokenizer.decode(outputs[0], skip_special_tokens=True)
return translated_text
# Example translation
input_text = "Hello, how are you?"
translated_text = translate_text(input_text, model, tokenizer)
print(translated_text)
Step 4: Evaluate Translation Quality
Evaluate translation quality using metrics like BLEU score to assess the similarity between human-generated translations and machine translations.
pythonCopy codefrom nltk.translate.bleu_score import sentence_bleu
# Example evaluation
reference = "Salut, cum îți merge?"
bleu_score = sentence_bleu([reference.split()], translated_text.split())
print(f"BLEU Score: {bleu_score}")
Real-Life Example: Multi-Language Chat Application
Imagine developing a multi-language chat application where users can communicate in different languages seamlessly. The application leverages language translation APIs and NLP models to translate messages in real-time, enabling cross-language conversations.
Conclusion
Language translation using Python NLP empowers applications with multilingual capabilities, facilitating global communication and collaboration. By leveraging state-of-the-art NLP models and evaluation metrics, developers can build robust language translation systems that deliver accurate and contextually relevant translations across various languages.
Subscribe to my newsletter
Read articles from Prakhar Kumar directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by