Why Spring? | Loose coupling
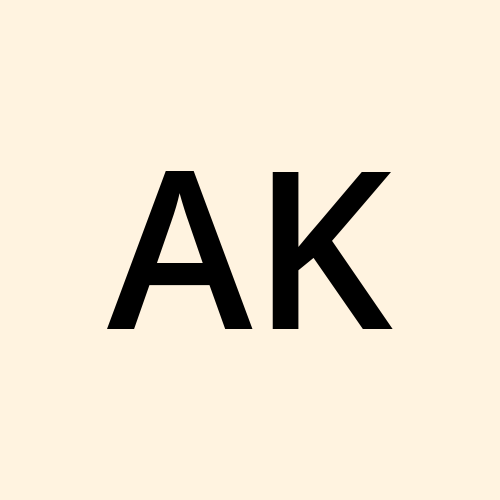
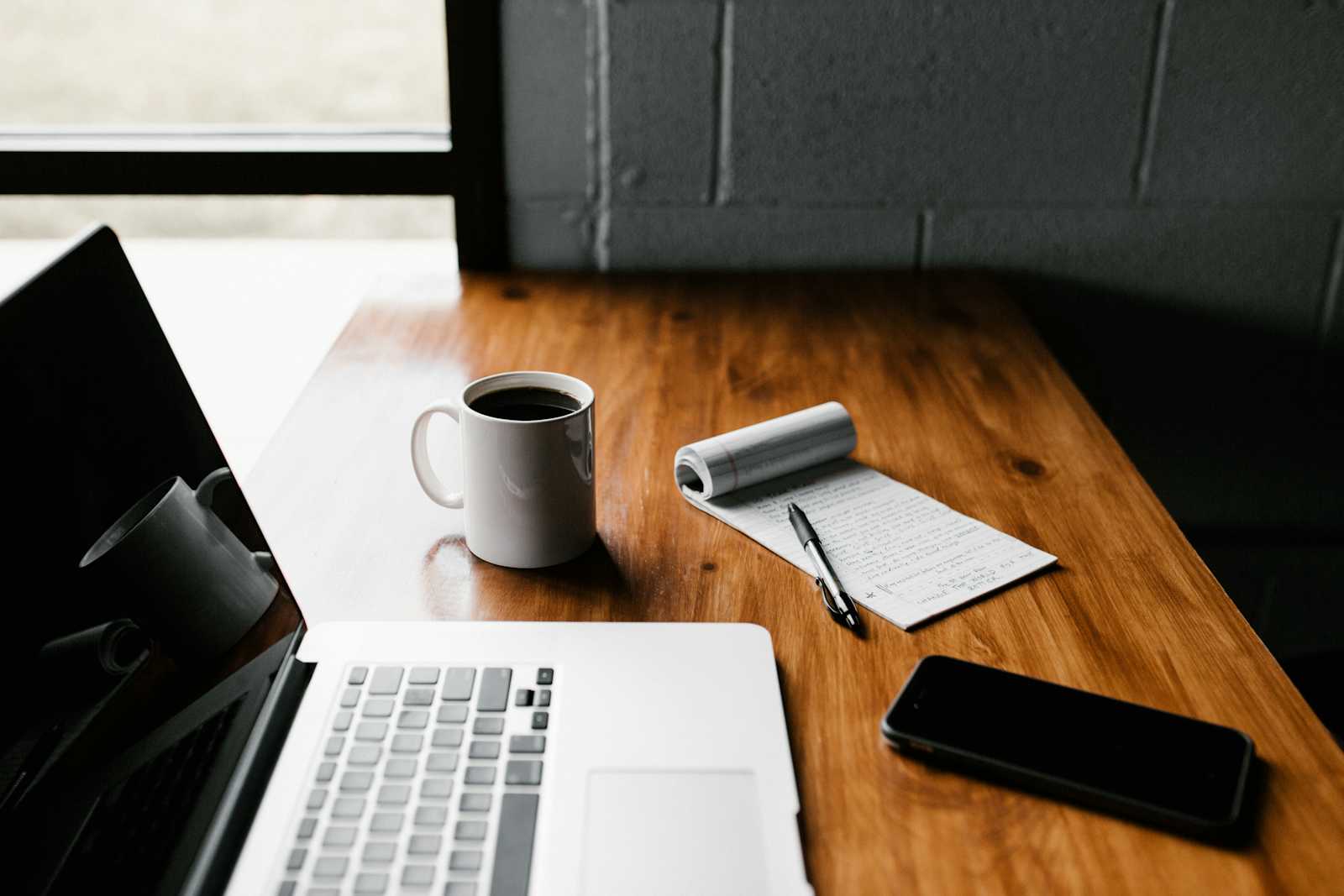
Keywords
- Tight Coupling
- Loose Coupling
- Target class
- Dependent object
- Dependency Injection
- Inversion of control
- Spring
- SpringBoot
Tight Coupling :- If we did any changes in one class then if it will affect in another class then we can say like these two classes are tightly coupled to each other
Loose Coupling :- If we did any changes in one class then if it will not affect in another class then we can say like these two classes are loosely coupled to each other
To implement loose coupling we should follow
->Code to interface
abstraction, providing the specifications what needs to be done,
here we are not writing any business logic.
public interface Course {
boolean getTheCourse(double amount);
}
Business logic we will write in implememtation calss
public class SpringBoot implements Course{
@Override
public boolean getTheCourse(double amount) {
System.out.println("SpringBoot course purchased and fee paid is "+amount);
return true;
}
}
In the Following class we will not make object of springBoot class
public class LaunchCourse {
private Course course;
public void setCourse(Course course) {
this.course = course;
}
boolean buyTheCourse(double amount){
return course.getTheCourse(amount);
}
}
This we will do by Dependency Injection
Target class:- target class is a such a class where services of other classes are being used e.g LaunchCourse class
Dependent object:- dependent object whose service will be used in target class e.g SpringBoot class
Dependency Injection :- In the target class injecting dependent object is called as depency injection.
public class Main {
public static void main(String[] args) {
System.out.println("Welcome");
LaunchCourse launchCourse=new LaunchCourse();
launchCourse.setCourse(new SpringBoot());
boolean status = launchCourse.buyTheCourse(699.9);
if(status)
System.out.println("Course purchased successfully");
else
System.out.println("Course purchased Failed");
}
}
Inversion of control:- If all the object being maintain and dependency injecting by spring framework then this is called inversion of control
Spring :-Spring is a framework. It is help us to gain 100 % loose coupling
SpringBoot:- Using spring framework and make auto configuration easily then we go with springboot.
Subscribe to my newsletter
Read articles from Ajeet Kumar directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
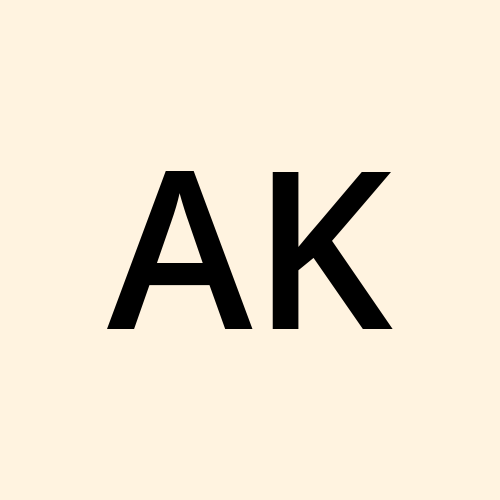