if(answer[i] == pattern[j][i %pattern[j].length]){ } : a single line of code that got me bursted
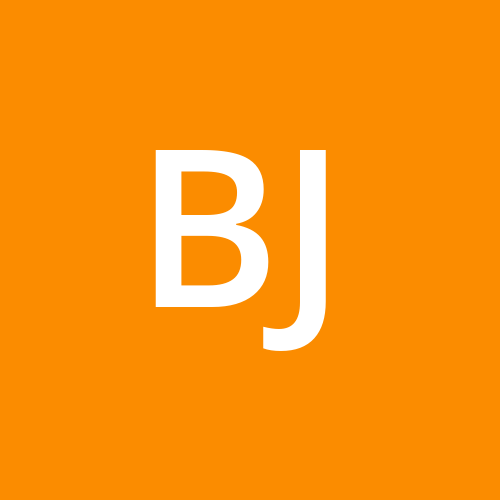
#Foreword
My goal is very simple, I want to understand this Code, Although I am endeavoring to understand and analyze this line of code, I never understand this. sometimes, when I don't face some codes that I never comprehend, I tend to type the code without thoughts. Sometimes, before understanding it, I can accept that code as it is intuitively. But......This Line of code snippets is really hard to accept, It seems a single line of simple code, but I don't know how much several concetps are related, Therefore, I am going to try to walk through this line of code by myself. (I want to ensure I am not a professional programmer, so this code is easy for other people, it's just my logging for my future use.)
if(answer[i] == pattern[j][i % pattern[j].length]) { scores[j]++; }
#Problem
Below Code got me confused. I don't understand how it works.
for(int i = 0; i < answers.length; i++) {
for(int j = 0; j < pattern.length; j++) {
if (answers[i] = pattern[j][i % pattern[j].length]) {
scores[j]++
}
}
}
#Printing 2-dimensional Array's Value 'j < pattern.length; , i < pattern[j].length'
public class Q2ForUnderstandingTwoDimensionalArrayValues {
public static void main(String[] args) {
int[] answer = {1, 2, 3, 4, 5};
int[][] pattern = {
{1, 2, 3, 4, 5},
{2, 2, 3, 3, 4, 4, 5, 5, 1, 1},
{3, 1, 3, 2, 3, 3, 3, 4, 3, 5},
{5, 4, 3, 2, 1}
};
// pattern Array Row Length
System.out.println("pattern.length = " + pattern.length);
// pattern Array Each Row's Column Length.
System.out.println("pattern[0].length = " + pattern[0].length);
System.out.println("pattern[1].length = " + pattern[1].length);
System.out.println("pattern[2].length = " + pattern[2].length);
System.out.println("pattern[3].length = " + pattern[3].length);
for (int i = 0; i < answer.length; i++) {
System.out.println("answer[i] = " + answer[i] + " ,");
}
for (int j = 0; j < pattern.length; j++) {
for (int i = 0; i < pattern[j].length; i++) {
System.out.println(
"pattern[" + j + "][" + i + "] = " + pattern[j][i] + ", "
);
}
}
}
}
Above Code is printing out array's whole row length and each Row's colum length
and every single value in 2 dimensional array.
pattern.length => Row's length : 4, Indicies : 4
pattern[j].length => each Row's length :
ex) pattern[0].length => 5
pattern[1].length => 10
pattern[2].length => 10
pattern[3].length => 5
#Modulus Operator - For Remainder
'a % b' : When a < b , a = b , a > b,
public class Q2ForUnderstadningModulusOperator {
public static void main(String[] args) {
// Exception in thread "main" java.lang.ArithmeticException: / by zero
// System.out.println("3 % 0 = " + modulusMethod(3, 0)); <- ArithmeticException with / by zero.
System.out.println("'a > b' -> 3 % 1 = " + modulusMethod(3, 1));
System.out.println("'a > b' -> 3 % 2 = " + modulusMethod(3, 2));
System.out.println("'a = b' -> 3 % 3 = " + modulusMethod(3, 3));
System.out.println("'a < b' -> 3 % 4 = " + modulusMethod(3, 4));
System.out.println("'a < b' -> 3 % 5 = " + modulusMethod(3, 5));
System.out.println("'a < b' -> 3 % 6 = " + modulusMethod(3, 6));
System.out.println("'a < b' -> 3 % 7 = " + modulusMethod(3, 7));
}
public static int modulusMethod(int a, int b) {
int result = a % b;
return result;
}
}
Modulus Operating
a > b -> remainder is Incremented 3 % 1 => 3 / 1 = 1 * 3 + 0 (remainder)
a > b -> remainder is Incremented 3 % 2 => 3 / 2 = 2 * 1 + 1 (remainder)
a = b -> remainder is '0' => 3 % 3 => 3 / 3 = 3 * 1 + 0 (remainder)
a < b -> remainder is fixed as "a" => 3 / 7 = 7 * 0 + 3 (remainder)
#Understadning Problem
for(int i = 0; i < answers.length; i++) {
for(int j = 0; j < pattern.length; j++) {
if (answers[i] == pattern[j][i % pattern[j].length]) {
scores[j]++
}
}
}
"answers[i] == pattern[j][i % pattern[j].length]"
We can understand this code, i % pattern[j].length
-> 1. i > pattern[j].length ==> remainder is incrementing by one
2. i = pattern[j].length ==> remainder is fixed as 0
3. i < pattern[j].length ==> remainder is fixed as 'i' number.
:: In other words, pattern[j] row's column number -> pattern[j][columnN]
is incrementing only when "i > pattern[j].length".
In Conclusion:
pattern[j][i % pattern[j].length]:
Accesses the element at position (i % pattern[j].length) within the j-th row
of the pattern array. Here, % is the modulus operator, which calculates
the remainder of i divided by pattern[j].length (the length of the j-th row
of pattern.) This operation ensures that the index stays within the bounds
of the pattern[j] array even if 'i' exceeds the length of the row."
Cf)
#Code Explanation (Whole Code Snippet)
(I saw that code from Coding Test Problems, I won't use that problem as it is, I will change the several conditions and I will post whole code for the reference)
"int[] answers" is given as answer sheet, it has specific answers pattern.
(answers number is among '1,2,3,4,5')
There're four test-takers that use specific pattern number for answer. (A,B,C,D)
We are seeking for test-taker who has max scores, If there're test-takers who have the same scores, it's sorted in ascending order.
#Test-taker's Pattern
A : 1,2,3,4,5,1,2,3,4,5
B : 2,2,3,3,4,4,5,5,1,1,2,2,3,3,4,4,5,5,1,1
C : 3,1,3,2,3,3,3,4,3,5,3,1,3,2,3,3,3,4,3,5
D : 5,4,3,2,1,5,4,3,2,1
#Input and output examples
answers(pattern of answer sheet) / return (test taker that has max scroes)
[1,2,3,4,5] [1]
[1,3,2,4,2] [1,2,3]
#Resolving Problem
figure out each test-taker's answer pattern.
A : 1,2,3,4,5 (repeat)
B : 2,2,3,3,4,4,5,5,1,1 (repeat)
C : 3,1,3,2,3,3,3,4,3,5 (repeat)
D : 5,4,3,2,1 (repeat)
public static int[] solution(int[] answers) {
}
/* Normally, We don't do Hard Coding, but as i heard
,it's useful depending on situation. See below (when it's clear to use)*/
// Test-taker's answer pattern
int[][] pattern = {
{1,2,3,4,5},
{2,2,3,3,4,4,5,5,1,1},
{3,1,3,2,3,3,3,4,3,5},
{5,4,3,2,1}
};
// Array for saving test-taker's score. (4 test-taker)
int[] scores = new int[4];
// Matching Answer Sheet Pattern with each Test-Taker's Answer pattern.
for(int i = 0; i < answers.length; i++) {
for(int j = 0; j < pattern.length; j++) {
if (answers[i] = pattern[j][i % pattern[j].length]) {
scores[j]++
}
}
}
// save max Score (from "int[]" into "int".)
int maxScore = Arrays.stream(scores).max().getAsInt();
// the number of test-taker that is max score is added into ArrayList.
ArrayList<Integer> answer = new ArrayList<>();
for (int i = 0; i < scores.length; i++) {
if (scores[i] == maxScore) {
/* test taker's index number starts from 0, by writing (i + 1) this
,it starts from 1, which is for Human Index, for readability */
answer.add(i + 1);
}
}
int[] result = answer.stream().mapToInt(Integer::intValue).toArray();
return result;
}
}
Subscribe to my newsletter
Read articles from Byung Joo Jeong directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
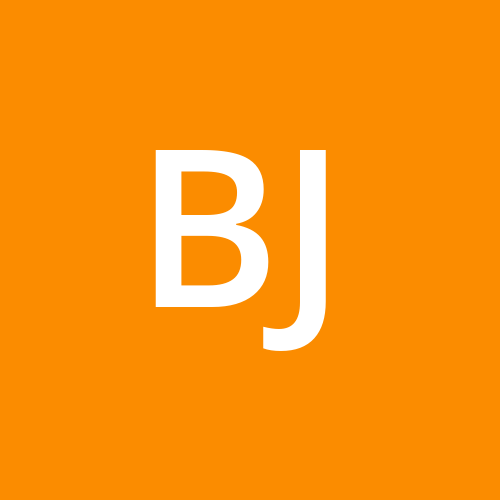