Fluent Interface in PHP
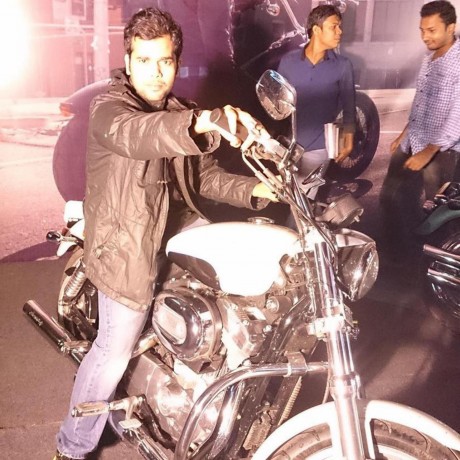
Purpose: To write code that is easily readable like sentences in a natural language (like English).
The Fluent Interface is an Interface Design Pattern that allows us to chain method calls together in a readable and intuitive manner. We must declare methods that return objects from the same class to implement it. As a result, we’ll be able to chain together multiple method calls. The pattern is often used in building QueryBuilder in ORM.
Here is a simple example of a calculator using the method chaining / Fluent Interface
class Calculator
{
public $value = 0;
public function Fluently()
{
return $this;
}
public function Add( $value )
{
$this->value = $value;
return $this;
}
public function Subtract($value)
{
$this->value -= $value;
return $this;
}
public function Multiply($value)
{
$this->value *= $value;
return $this;
}
public function Divide($value)
{
$this->value /= $value;
return $this;
}
public function Result()
{
return $this;
}
}
$MyCalculator = new Calculator();
$result = $MyCalculator->Fluently()
->Add(5)
->Multiply(3)
->Subtract(3)
->Divide(4)
->Result();
var_dump($result); // result ((5 * 3) — 3) / 4 = 3
Now if we have to write the above example without Fluent Interface.
class Calculator2
{
public $value = 0;
public function Add( $value )
{
$this->value += $value;
}
public function Subtract($value)
{
$this->value -= $value;
}
public function Multiply($value)
{
$this->value *= $value;
}
public function Divide($value)
{
$this->value /= $value;
}
public function Result()
{
return $this;
}
}
$MyCalculator2 = new Calculator2();
$MyCalculator2->add(5);
$MyCalculator2->Multiply(3);
$MyCalculator2->Subtract(3);
$MyCalculator2->Divide(4);
var_dump($MyCalculator2->Result()); // result ((5 * 3) — 3) / 4 = 3
The code looks cleaner and more readable with Fluent Interface in the previous example than this one.
Conclusion
A fluent interface is a design pattern that can be used to create an easy-to-use and read API. It is often used to provide a simple, intuitive way for developers to interact with it.
Some examples of scenarios where the fluent interface design pattern may be useful include:
Creating a QueryBuilder for a database
Creating an Interface for a Web Service
Creating an Builder Interface for a web form
Reference:
https://designpatternsphp.readthedocs.io/en/latest/Structural/FluentInterface/README.html
https://justgokus.medium.com/what-is-the-fluent-interface-design-pattern-177b9cc93c75
https://programmingdive.com/how-to-use-method-chaining-in-php/
Subscribe to my newsletter
Read articles from Kishore Chandra Sahoo directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
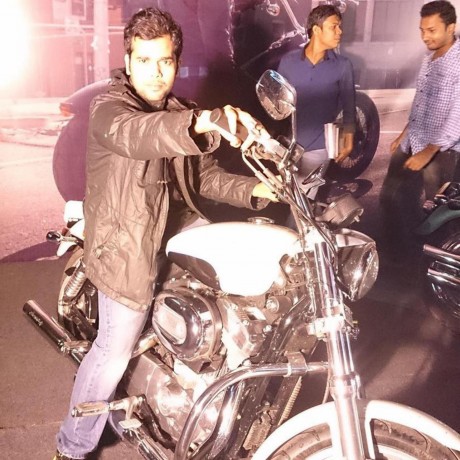
Kishore Chandra Sahoo
Kishore Chandra Sahoo
ଓଡ଼ିଆ, Trekkie, Developer, Founder & CEO of @upnrunnHQ 🚀 , @wpdrift.